Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / CollectionEditVerbManager.cs / 1 / CollectionEditVerbManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using Accessibility; using System.Runtime.Serialization.Formatters; using System.Threading; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using System.Drawing.Design; using Microsoft.Win32; ////// Class for sharing code for launching the ToolStripItemsCollectionEditor from a verb. /// This class implments the IWindowsFormsEditorService and ITypeDescriptorContext to /// display the dialog. /// /// internal class CollectionEditVerbManager : IWindowsFormsEditorService, ITypeDescriptorContext { private ComponentDesigner _designer; private IComponentChangeService _componentChangeSvc; private PropertyDescriptor _targetProperty; private DesignerVerb _editItemsVerb; ////// Create one of these things... /// internal CollectionEditVerbManager(string text, ComponentDesigner designer, PropertyDescriptor prop, bool addToDesignerVerbs) { Debug.Assert(designer != null, "Can't have a CollectionEditVerbManager without an associated designer"); this._designer = designer; this._targetProperty = prop; if (prop == null) { prop = TypeDescriptor.GetDefaultProperty(designer.Component); if (prop != null && typeof(ICollection).IsAssignableFrom(prop.PropertyType)) { _targetProperty = prop; } } Debug.Assert(_targetProperty != null, "Need PropertyDescriptor for ICollection property to associate collectoin edtior with."); if (text == null) { text = SR.GetString(SR.ToolStripItemCollectionEditorVerb); } _editItemsVerb = new DesignerVerb(text, new EventHandler(this.OnEditItems)); if (addToDesignerVerbs) { _designer.Verbs.Add(_editItemsVerb); } } ////// Our caching property for the IComponentChangeService /// private IComponentChangeService ChangeService { get { if (_componentChangeSvc == null) { _componentChangeSvc = (IComponentChangeService)((IServiceProvider)this).GetService(typeof(IComponentChangeService)); } return _componentChangeSvc; } } ////// Self-explanitory interface impl. /// IContainer ITypeDescriptorContext.Container { get { if (_designer.Component.Site != null) { return _designer.Component.Site.Container; } return null; } } public DesignerVerb EditItemsVerb { get { return _editItemsVerb; } } ////// Self-explanitory interface impl. /// void ITypeDescriptorContext.OnComponentChanged() { ChangeService.OnComponentChanged(_designer.Component, _targetProperty, null, null); } ////// Self-explanitory interface impl. /// bool ITypeDescriptorContext.OnComponentChanging() { try { ChangeService.OnComponentChanging(_designer.Component, _targetProperty); } catch(CheckoutException checkoutException) { if (checkoutException == CheckoutException.Canceled) { return false; } throw; } return true; } ////// Self-explanitory interface impl. /// object ITypeDescriptorContext.Instance { get { return _designer.Component; } } ////// Self-explanitory interface impl. /// PropertyDescriptor ITypeDescriptorContext.PropertyDescriptor { get { return _targetProperty; } } ////// Self-explanitory interface impl. /// object IServiceProvider.GetService(Type serviceType) { if (serviceType == typeof(ITypeDescriptorContext) || serviceType == typeof(IWindowsFormsEditorService)) { return this; } if (_designer.Component.Site != null) { return _designer.Component.Site.GetService(serviceType); } return null; } ////// Self-explanitory interface impl. /// void IWindowsFormsEditorService.CloseDropDown() { // we'll never be called to do this. // Debug.Fail("NOTIMPL"); return; } ////// Self-explanitory interface impl. /// void IWindowsFormsEditorService.DropDownControl(Control control) { // nope, sorry // Debug.Fail("NOTIMPL"); return; } ////// Self-explanitory interface impl. /// System.Windows.Forms.DialogResult IWindowsFormsEditorService.ShowDialog(Form dialog) { IUIService uiSvc = (IUIService)((IServiceProvider)this).GetService(typeof(IUIService)); if (uiSvc != null) { return uiSvc.ShowDialog(dialog); } else { return dialog.ShowDialog(_designer.Component as IWin32Window); } } ////// When the verb is invoked, use all the stuff above to show the dialog, etc. /// private void OnEditItems(object sender, EventArgs e) { // Hide the Chrome.. DesignerActionUIService actionUIService = (DesignerActionUIService)((IServiceProvider)this).GetService(typeof(DesignerActionUIService)); if (actionUIService != null) { actionUIService.HideUI(_designer.Component); } object propertyValue = _targetProperty.GetValue(_designer.Component); if (propertyValue == null) { return; } CollectionEditor itemsEditor = TypeDescriptor.GetEditor(propertyValue, typeof(UITypeEditor)) as CollectionEditor; Debug.Assert(itemsEditor != null, "Didn't get a collection editor for type '" + _targetProperty.PropertyType.FullName + "'"); if (itemsEditor != null) { itemsEditor.EditValue(this, this, propertyValue); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
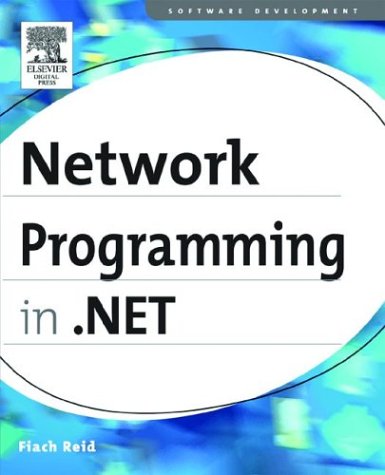
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLoader.cs
- Deserializer.cs
- PLINQETWProvider.cs
- SystemEvents.cs
- RawUIStateInputReport.cs
- MaskInputRejectedEventArgs.cs
- XamlStream.cs
- DependencyStoreSurrogate.cs
- StringFreezingAttribute.cs
- TdsParameterSetter.cs
- CodeParameterDeclarationExpressionCollection.cs
- WinInet.cs
- SingleObjectCollection.cs
- JoinTreeNode.cs
- StatusBarItemAutomationPeer.cs
- SerializationEventsCache.cs
- CollectionBase.cs
- FontStyles.cs
- GetRecipientRequest.cs
- ProcessManager.cs
- EntityDataSourceContainerNameItem.cs
- DataGridItemEventArgs.cs
- CodeRegionDirective.cs
- MarkupProperty.cs
- XNodeNavigator.cs
- HwndStylusInputProvider.cs
- Normalization.cs
- KeyValueConfigurationElement.cs
- AliasGenerator.cs
- TextEffectCollection.cs
- XamlReader.cs
- HttpChannelBindingToken.cs
- PagedDataSource.cs
- PerspectiveCamera.cs
- ClientApiGenerator.cs
- AssemblySettingAttributes.cs
- selecteditemcollection.cs
- SqlAliaser.cs
- FormsAuthenticationTicket.cs
- SecurityTokenContainer.cs
- RemotingAttributes.cs
- PrivilegedConfigurationManager.cs
- OutOfMemoryException.cs
- HtmlTableRow.cs
- TimeIntervalCollection.cs
- SHA1.cs
- AppearanceEditorPart.cs
- ProviderConnectionPointCollection.cs
- DbMetaDataColumnNames.cs
- LinkAreaEditor.cs
- RegisteredScript.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- URLIdentityPermission.cs
- XmlRawWriter.cs
- SqlReferenceCollection.cs
- OpenTypeCommon.cs
- ObjectTag.cs
- PageParser.cs
- WebPartManagerInternals.cs
- CellParagraph.cs
- ColorBuilder.cs
- WebPartZoneBaseDesigner.cs
- UnsafeNativeMethods.cs
- SqlServer2KCompatibilityAnnotation.cs
- DbDataAdapter.cs
- ArrayItemValue.cs
- DictionaryEntry.cs
- EntityWithKeyStrategy.cs
- BehaviorEditorPart.cs
- GraphicsState.cs
- EditingMode.cs
- EventMappingSettings.cs
- RowUpdatedEventArgs.cs
- XmlLinkedNode.cs
- MLangCodePageEncoding.cs
- SendKeys.cs
- IdentityNotMappedException.cs
- ToolStripScrollButton.cs
- WindowsRebar.cs
- XmlNamespaceMapping.cs
- RoleManagerEventArgs.cs
- EntityDataSourceReferenceGroup.cs
- FlowDocumentFormatter.cs
- OleTxTransaction.cs
- StorageAssociationTypeMapping.cs
- XmlSchemaExporter.cs
- InboundActivityHelper.cs
- TextPatternIdentifiers.cs
- AxisAngleRotation3D.cs
- SequentialActivityDesigner.cs
- SchemaNamespaceManager.cs
- PropertyItem.cs
- RuntimeHelpers.cs
- StateWorkerRequest.cs
- ThreadStateException.cs
- EditorPartChrome.cs
- AnonymousIdentificationSection.cs
- MouseGestureValueSerializer.cs
- Profiler.cs
- CodeDirectoryCompiler.cs