Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / BinHexDecoder.cs / 1 / BinHexDecoder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; namespace System.Xml { internal class BinHexDecoder : IncrementalReadDecoder { // // Fields // byte[] buffer; int startIndex; int curIndex; int endIndex; bool hasHalfByteCached; byte cachedHalfByte; // // IncrementalReadDecoder interface // internal override int DecodedCount { get { return curIndex - startIndex; } } internal override bool IsFull { get { return curIndex == endIndex; } } internal override unsafe int Decode( char[] chars, int startPos, int len ) { Debug.Assert( chars != null ); Debug.Assert( len >= 0 ); Debug.Assert( startPos >= 0 ); Debug.Assert( chars.Length - startPos >= len ); if ( len == 0 ) { return 0; } int bytesDecoded, charsDecoded; fixed ( char* pChars = &chars[startPos] ) { fixed ( byte* pBytes = &buffer[curIndex] ) { Decode( pChars, pChars + len, pBytes, pBytes + ( endIndex - curIndex ), ref this.hasHalfByteCached, ref this.cachedHalfByte, out charsDecoded, out bytesDecoded ); } } curIndex += bytesDecoded; return charsDecoded; } internal override unsafe int Decode( string str, int startPos, int len ) { Debug.Assert( str != null ); Debug.Assert( len >= 0 ); Debug.Assert( startPos >= 0 ); Debug.Assert( str.Length - startPos >= len ); if ( len == 0 ) { return 0; } int bytesDecoded, charsDecoded; fixed ( char* pChars = str ) { fixed ( byte* pBytes = &buffer[curIndex] ) { Decode( pChars + startPos, pChars + startPos + len, pBytes, pBytes + ( endIndex - curIndex ), ref this.hasHalfByteCached, ref this.cachedHalfByte, out charsDecoded, out bytesDecoded ); } } curIndex += bytesDecoded; return charsDecoded; } internal override void Reset() { this.hasHalfByteCached = false; this.cachedHalfByte = 0; } internal override void SetNextOutputBuffer( Array buffer, int index, int count ) { Debug.Assert( buffer != null ); Debug.Assert( count >= 0 ); Debug.Assert( index >= 0 ); Debug.Assert( buffer.Length - index >= count ); Debug.Assert( ( buffer as byte[] ) != null ); this.buffer = (byte[])buffer; this.startIndex = index; this.curIndex = index; this.endIndex = index + count; } // // Static methods // public static unsafe byte[] Decode( char[] chars, bool allowOddChars ) { if ( chars == null ) { throw new ArgumentException( "chars" ); } int len = chars.Length; if ( len == 0 ) { return new byte[0]; } byte[] bytes = new byte[ ( len + 1 ) / 2 ]; int bytesDecoded, charsDecoded; bool hasHalfByteCached = false; byte cachedHalfByte = 0; fixed ( char* pChars = &chars[0] ) { fixed ( byte* pBytes = &bytes[0] ) { Decode( pChars, pChars + len, pBytes, pBytes + bytes.Length, ref hasHalfByteCached, ref cachedHalfByte, out charsDecoded, out bytesDecoded ); } } if ( hasHalfByteCached && !allowOddChars ) { throw new XmlException( Res.Xml_InvalidBinHexValueOddCount, new string( chars ) ); } if ( bytesDecoded < bytes.Length ) { byte[] tmp = new byte[ bytesDecoded ]; Buffer.BlockCopy( bytes, 0, tmp, 0, bytesDecoded ); bytes = tmp; } return bytes; } // // Private methods // private static unsafe void Decode( char* pChars, char *pCharsEndPos, byte* pBytes, byte* pBytesEndPos, ref bool hasHalfByteCached, ref byte cachedHalfByte, out int charsDecoded, out int bytesDecoded ) { #if DEBUG Debug.Assert( pCharsEndPos - pChars >= 0 ); Debug.Assert( pBytesEndPos - pBytes >= 0 ); #endif char* pChar = pChars; byte* pByte = pBytes; XmlCharType xmlCharType = XmlCharType.Instance; while ( pChar < pCharsEndPos && pByte < pBytesEndPos ) { byte halfByte; char ch = *pChar++; if ( ch >= 'a' && ch <= 'f' ) { halfByte = (byte)(ch - 'a' + 10); } else if ( ch >= 'A' && ch <= 'F' ) { halfByte = (byte)(ch - 'A' + 10); } else if ( ch >= '0' && ch <= '9' ) { halfByte = (byte)(ch - '0'); } else if ( ( xmlCharType.charProperties[ch] & XmlCharType.fWhitespace ) != 0 ) { // else if ( xmlCharType.IsWhiteSpace( ch ) ) { continue; } else { throw new XmlException(Res.Xml_InvalidBinHexValue, new string( pChars, 0, (int)( pCharsEndPos - pChars ) ) ); } if ( hasHalfByteCached ) { *pByte++ = (byte)( ( cachedHalfByte << 4 ) + halfByte ); hasHalfByteCached = false; } else { cachedHalfByte = halfByte; hasHalfByteCached = true; } } bytesDecoded = (int)(pByte - pBytes); charsDecoded = (int)(pChar - pChars); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
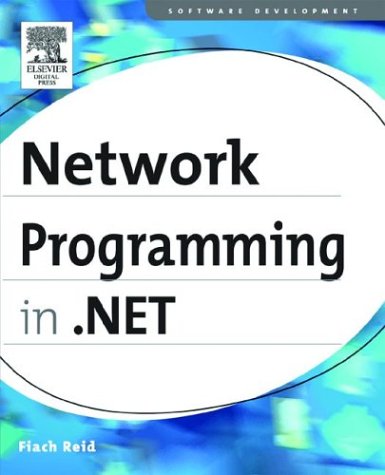
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnimationLayer.cs
- SqlFileStream.cs
- DefaultMemberAttribute.cs
- XmlChoiceIdentifierAttribute.cs
- Parallel.cs
- FilePrompt.cs
- StringPropertyBuilder.cs
- ClientApiGenerator.cs
- CodeConstructor.cs
- ViewBase.cs
- FormViewDeleteEventArgs.cs
- StdValidatorsAndConverters.cs
- BatchParser.cs
- ProxyWebPartConnectionCollection.cs
- FilterElement.cs
- ResourceReferenceExpression.cs
- XmlDocumentFragment.cs
- TableLayoutStyleCollection.cs
- ScriptControlManager.cs
- ObjectTokenCategory.cs
- WindowsRichEditRange.cs
- UnsafeMethods.cs
- ObjectDataSourceFilteringEventArgs.cs
- TypeBuilderInstantiation.cs
- ToolStripComboBox.cs
- StyleReferenceConverter.cs
- DetailsViewRow.cs
- RotateTransform.cs
- XmlSchemaAll.cs
- RouteValueDictionary.cs
- PolyLineSegment.cs
- NoneExcludedImageIndexConverter.cs
- KernelTypeValidation.cs
- XmlArrayItemAttributes.cs
- ObjectItemNoOpAssemblyLoader.cs
- GlobalizationSection.cs
- WmlLabelAdapter.cs
- HtmlInputImage.cs
- XslVisitor.cs
- StyleCollection.cs
- HttpResponse.cs
- PolyQuadraticBezierSegment.cs
- Visitors.cs
- BorderGapMaskConverter.cs
- XmlResolver.cs
- CoreSwitches.cs
- RadioButton.cs
- WindowInteractionStateTracker.cs
- SerializationFieldInfo.cs
- TypeSource.cs
- PageRanges.cs
- ObjectDataSourceMethodEventArgs.cs
- InstanceDataCollection.cs
- AssemblyInfo.cs
- ProgressBar.cs
- DataControlField.cs
- NativeMethods.cs
- CrossAppDomainChannel.cs
- GetKeyedHashRequest.cs
- MetadataItemEmitter.cs
- DiagnosticTrace.cs
- XslTransform.cs
- DesignerTransaction.cs
- ButtonPopupAdapter.cs
- Label.cs
- Cursors.cs
- PrintDialog.cs
- QueryOperationResponseOfT.cs
- ControlPaint.cs
- PointLight.cs
- Set.cs
- Point3DConverter.cs
- Transactions.cs
- QilFactory.cs
- IResourceProvider.cs
- EntityDataSourceReferenceGroup.cs
- util.cs
- IndexOutOfRangeException.cs
- DashStyles.cs
- DataGridViewCellPaintingEventArgs.cs
- Processor.cs
- ModifierKeysValueSerializer.cs
- UnknownBitmapDecoder.cs
- Hyperlink.cs
- MediaPlayer.cs
- MdiWindowListStrip.cs
- ConfigXmlDocument.cs
- BadImageFormatException.cs
- RijndaelManaged.cs
- IteratorFilter.cs
- InternalPermissions.cs
- TimeSpanValidator.cs
- COAUTHINFO.cs
- EntitySet.cs
- EmptyEnumerator.cs
- Odbc32.cs
- MetadataSerializer.cs
- FilterException.cs
- BindingOperations.cs
- ArrangedElement.cs