Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / CachedRequestParams.cs / 2 / CachedRequestParams.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Caches the value of all request headers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ////// Enum to represent various http methods /// internal enum AstoriaVerbs { #region Values. ///Not Initialized. None, ///Represents the GET http method. GET, ///Represents the PUT http method. PUT, ///Represents the POST http method. POST, ///Represents the DELETE http method. DELETE, ///Represents the MERGE http method. MERGE #endregion Values. } ////// Use this class to hold on to request parameters that may be inaccessible after a response writing begins. /// [DebuggerDisplay("{HttpMethod} {AbsoluteRequestUri}")] internal class CachedRequestParams { #region Fields. ///Gets the absolute resource upon which to apply the request. internal readonly Uri AbsoluteRequestUri; ///Gets the absolute URI to the service. internal readonly Uri AbsoluteServiceUri; ///Value for the Accept header. internal readonly string Accept; ///Value for the Accept-Charset header. internal readonly string AcceptCharset; ///Value of the Content-Type header. internal readonly string ContentType; ///HTTP method used for the request. internal readonly string HttpMethod; ///Value for the If-Match header. internal readonly string IfMatch; ///Value for the If-None-Match header. internal readonly string IfNoneMatch; ///Value for the MaxDataServiceVersion header. internal readonly string MaxVersion; ///Value for the DataServiceVersion header. internal readonly string Version; ///enum indicating http method used on the request, private AstoriaVerbs astoriaHttpVerb; #endregion Fields. #region Constructors. ///Initializes a new /// Value for the Accept header. /// Value for the Accept-Charset header. /// Value of the Content-Type header. /// HTTP method used for the request. /// Value for the If-Match header. /// Value for the If-None-Match header. /// Value for the DataServiceVersion header. /// Value for the MaxDataServiceVersion header. /// The absolute URI to the resource upon which to apply the request. /// URI to the service. internal CachedRequestParams( string accept, string acceptCharset, string contentType, string httpMethod, string headerIfMatch, string headerIfNoneMatch, string version, string maxVersion, Uri absoluteRequestUri, Uri serviceUri) { Debug.Assert(absoluteRequestUri != null, "absoluteRequestUri != null"); Debug.Assert(serviceUri != null, "serviceUri != null"); Debug.Assert(absoluteRequestUri.IsAbsoluteUri, "absoluteRequestUri.IsAbsoluteUri(" + absoluteRequestUri + ")"); Debug.Assert(serviceUri.IsAbsoluteUri, "serviceUri.IsAbsoluteUri(" + serviceUri + ")"); this.Accept = accept; this.AcceptCharset = acceptCharset; this.ContentType = contentType; this.HttpMethod = httpMethod; this.IfMatch = headerIfMatch; this.IfNoneMatch = headerIfNoneMatch; this.AbsoluteRequestUri = absoluteRequestUri; this.AbsoluteServiceUri = serviceUri; this.MaxVersion = maxVersion; this.Version = version; this.astoriaHttpVerb = AstoriaVerbs.None; } #endregion Constructors. #region Properties. ///instance. /// Get the enum representing the http method name. /// /// http method used for the request. ///enum representing the http method name. internal AstoriaVerbs AstoriaHttpVerb { get { if (this.astoriaHttpVerb == AstoriaVerbs.None) { if (XmlConstants.HttpMethodGet == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.GET; } else if (XmlConstants.HttpMethodPost == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.POST; } else if (XmlConstants.HttpMethodPut == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.PUT; } else if (XmlConstants.HttpMethodMerge == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.MERGE; } else if (XmlConstants.HttpMethodDelete == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.DELETE; } else if (String.IsNullOrEmpty(this.HttpMethod)) { throw new InvalidOperationException(Strings.DataService_EmptyHttpMethod); } else { // 501: Not Implemented (rather than 405 - Method Not Allowed, // which implies it was understood and rejected). throw DataServiceException.CreateMethodNotImplemented(Strings.DataService_NotImplementedException); } } return this.astoriaHttpVerb; } } #endregion Properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Caches the value of all request headers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Diagnostics; #endregion Namespaces. ////// Enum to represent various http methods /// internal enum AstoriaVerbs { #region Values. ///Not Initialized. None, ///Represents the GET http method. GET, ///Represents the PUT http method. PUT, ///Represents the POST http method. POST, ///Represents the DELETE http method. DELETE, ///Represents the MERGE http method. MERGE #endregion Values. } ////// Use this class to hold on to request parameters that may be inaccessible after a response writing begins. /// [DebuggerDisplay("{HttpMethod} {AbsoluteRequestUri}")] internal class CachedRequestParams { #region Fields. ///Gets the absolute resource upon which to apply the request. internal readonly Uri AbsoluteRequestUri; ///Gets the absolute URI to the service. internal readonly Uri AbsoluteServiceUri; ///Value for the Accept header. internal readonly string Accept; ///Value for the Accept-Charset header. internal readonly string AcceptCharset; ///Value of the Content-Type header. internal readonly string ContentType; ///HTTP method used for the request. internal readonly string HttpMethod; ///Value for the If-Match header. internal readonly string IfMatch; ///Value for the If-None-Match header. internal readonly string IfNoneMatch; ///Value for the MaxDataServiceVersion header. internal readonly string MaxVersion; ///Value for the DataServiceVersion header. internal readonly string Version; ///enum indicating http method used on the request, private AstoriaVerbs astoriaHttpVerb; #endregion Fields. #region Constructors. ///Initializes a new /// Value for the Accept header. /// Value for the Accept-Charset header. /// Value of the Content-Type header. /// HTTP method used for the request. /// Value for the If-Match header. /// Value for the If-None-Match header. /// Value for the DataServiceVersion header. /// Value for the MaxDataServiceVersion header. /// The absolute URI to the resource upon which to apply the request. /// URI to the service. internal CachedRequestParams( string accept, string acceptCharset, string contentType, string httpMethod, string headerIfMatch, string headerIfNoneMatch, string version, string maxVersion, Uri absoluteRequestUri, Uri serviceUri) { Debug.Assert(absoluteRequestUri != null, "absoluteRequestUri != null"); Debug.Assert(serviceUri != null, "serviceUri != null"); Debug.Assert(absoluteRequestUri.IsAbsoluteUri, "absoluteRequestUri.IsAbsoluteUri(" + absoluteRequestUri + ")"); Debug.Assert(serviceUri.IsAbsoluteUri, "serviceUri.IsAbsoluteUri(" + serviceUri + ")"); this.Accept = accept; this.AcceptCharset = acceptCharset; this.ContentType = contentType; this.HttpMethod = httpMethod; this.IfMatch = headerIfMatch; this.IfNoneMatch = headerIfNoneMatch; this.AbsoluteRequestUri = absoluteRequestUri; this.AbsoluteServiceUri = serviceUri; this.MaxVersion = maxVersion; this.Version = version; this.astoriaHttpVerb = AstoriaVerbs.None; } #endregion Constructors. #region Properties. ///instance. /// Get the enum representing the http method name. /// /// http method used for the request. ///enum representing the http method name. internal AstoriaVerbs AstoriaHttpVerb { get { if (this.astoriaHttpVerb == AstoriaVerbs.None) { if (XmlConstants.HttpMethodGet == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.GET; } else if (XmlConstants.HttpMethodPost == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.POST; } else if (XmlConstants.HttpMethodPut == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.PUT; } else if (XmlConstants.HttpMethodMerge == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.MERGE; } else if (XmlConstants.HttpMethodDelete == this.HttpMethod) { this.astoriaHttpVerb = AstoriaVerbs.DELETE; } else if (String.IsNullOrEmpty(this.HttpMethod)) { throw new InvalidOperationException(Strings.DataService_EmptyHttpMethod); } else { // 501: Not Implemented (rather than 405 - Method Not Allowed, // which implies it was understood and rejected). throw DataServiceException.CreateMethodNotImplemented(Strings.DataService_NotImplementedException); } } return this.astoriaHttpVerb; } } #endregion Properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
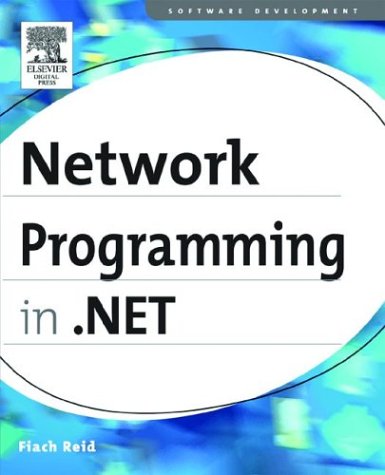
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PingOptions.cs
- SqlBuffer.cs
- SettingsPropertyValueCollection.cs
- DesignTimeSiteMapProvider.cs
- FamilyMapCollection.cs
- XmlChildNodes.cs
- CryptoKeySecurity.cs
- UnknownBitmapDecoder.cs
- DrawListViewColumnHeaderEventArgs.cs
- GraphicsPathIterator.cs
- LinqDataSourceDisposeEventArgs.cs
- MessageQueueAccessControlEntry.cs
- PrimarySelectionGlyph.cs
- LinqToSqlWrapper.cs
- Visitor.cs
- HtmlHead.cs
- LinqDataSourceValidationException.cs
- PersonalizationProvider.cs
- HtmlTableCellCollection.cs
- ControlValuePropertyAttribute.cs
- SqlFormatter.cs
- TypeConverterAttribute.cs
- SurrogateEncoder.cs
- Frame.cs
- CursorEditor.cs
- InvalidPipelineStoreException.cs
- sqlstateclientmanager.cs
- SoapExtensionTypeElementCollection.cs
- Configuration.cs
- QueryStringParameter.cs
- Byte.cs
- TemplateControl.cs
- OleTxTransaction.cs
- EncoderParameters.cs
- DBSchemaRow.cs
- XmlQueryOutput.cs
- PartDesigner.cs
- TypeGeneratedEventArgs.cs
- MetadataCache.cs
- LeftCellWrapper.cs
- SqlDataAdapter.cs
- ConditionValidator.cs
- AppSettingsExpressionBuilder.cs
- Msmq3PoisonHandler.cs
- BuildManager.cs
- LinqDataSource.cs
- ButtonColumn.cs
- ConfigErrorGlyph.cs
- MergePropertyDescriptor.cs
- Pkcs7Recipient.cs
- KeyGestureConverter.cs
- ThousandthOfEmRealDoubles.cs
- MembershipSection.cs
- HttpCachePolicyBase.cs
- AsnEncodedData.cs
- LocatorBase.cs
- CancellationState.cs
- ObjectQueryExecutionPlan.cs
- AncillaryOps.cs
- ControlsConfig.cs
- ScrollBarRenderer.cs
- LockedAssemblyCache.cs
- HttpPostedFileBase.cs
- CopyOfAction.cs
- GetIndexBinder.cs
- UnitySerializationHolder.cs
- FormViewPagerRow.cs
- ProjectionNode.cs
- BatchParser.cs
- ObjectListCommandsPage.cs
- SchemaMapping.cs
- GetIndexBinder.cs
- CustomPopupPlacement.cs
- GeneralTransformGroup.cs
- SqlConnection.cs
- M3DUtil.cs
- WebRequestModuleElementCollection.cs
- CacheHelper.cs
- NonSerializedAttribute.cs
- SchemaTypeEmitter.cs
- ComboBoxRenderer.cs
- ToolStripSplitButton.cs
- WsdlInspector.cs
- ConstructorNeedsTagAttribute.cs
- KeyFrames.cs
- MiniLockedBorderGlyph.cs
- SchemaNotation.cs
- WebPartCollection.cs
- WebPartCatalogCloseVerb.cs
- StorageRoot.cs
- DeadCharTextComposition.cs
- IndependentAnimationStorage.cs
- Pkcs7Signer.cs
- DynamicDocumentPaginator.cs
- SettingsPropertyNotFoundException.cs
- Grammar.cs
- BitmapEffectInput.cs
- DataSourceControlBuilder.cs
- RecognizedAudio.cs
- DefaultDiscoveryService.cs