Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / ActivityTypeDesigner.xaml.cs / 1305376 / ActivityTypeDesigner.xaml.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Linq; using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.Activities.Presentation.Model; using System.Windows.Threading; using System.Activities.Presentation.Services; using System.Activities.Presentation.Xaml; partial class ActivityTypeDesigner : IExpandChild { public ActivityTypeDesigner() { this.InitializeComponent(); } protected override void OnModelItemChanged(object newItem) { base.OnModelItemChanged(newItem); if (this.Context.Services.GetService() == null) { this.Context.Services.Publish (new DisplayNameUpdater(this.Context)); } } protected override void OnContextMenuLoaded(ContextMenu menu) { base.OnContextMenuLoaded(menu); if (null == this.ModelItem.Properties["Implementation"].Value) { var toHide = menu.Items.OfType
Link Menu
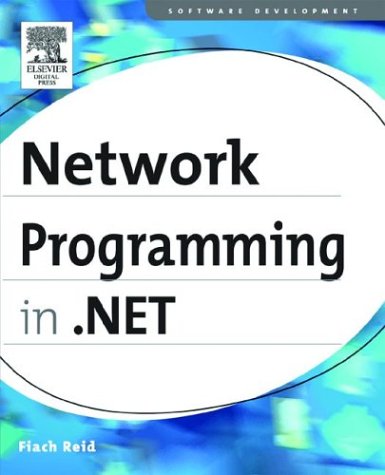
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIntPtr.cs
- EntityParameter.cs
- ProgressBar.cs
- WindowsListViewGroupHelper.cs
- TableCellCollection.cs
- EventLogLink.cs
- ConnectionPoint.cs
- InputReferenceExpression.cs
- MustUnderstandBehavior.cs
- securestring.cs
- CircleHotSpot.cs
- UnsafeNativeMethods.cs
- FontWeights.cs
- SingleAnimation.cs
- XmlSchemaAnyAttribute.cs
- ScopelessEnumAttribute.cs
- EditorZone.cs
- OSEnvironmentHelper.cs
- SerializationInfoEnumerator.cs
- HWStack.cs
- RbTree.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- SelectionProviderWrapper.cs
- MembershipSection.cs
- ServiceModelReg.cs
- SafeEventLogWriteHandle.cs
- PackWebRequestFactory.cs
- QueryComponents.cs
- OrderedDictionary.cs
- CultureMapper.cs
- TransactionFlowProperty.cs
- QilPatternVisitor.cs
- CodeMemberField.cs
- Line.cs
- CodeIdentifiers.cs
- MsmqIntegrationSecurityElement.cs
- ContextMenuStripActionList.cs
- ToolStripMenuItemDesigner.cs
- IdentityNotMappedException.cs
- XmlSchemaIdentityConstraint.cs
- Mutex.cs
- XamlSerializationHelper.cs
- ObjectDataSourceWizardForm.cs
- SequentialUshortCollection.cs
- EntitySqlException.cs
- PageStatePersister.cs
- BindingNavigator.cs
- TraceListener.cs
- TextFormatterHost.cs
- ServiceEndpointElement.cs
- ProvidePropertyAttribute.cs
- CodeDelegateInvokeExpression.cs
- ServiceParser.cs
- TransformPattern.cs
- TraceHandlerErrorFormatter.cs
- Object.cs
- XmlSchemaAppInfo.cs
- SurrogateChar.cs
- MouseEventArgs.cs
- DummyDataSource.cs
- XmlSchemaSimpleType.cs
- HelpProvider.cs
- CheckBoxField.cs
- JournalEntry.cs
- RegularExpressionValidator.cs
- ToolStripItemRenderEventArgs.cs
- FileUtil.cs
- WithParamAction.cs
- CatalogZone.cs
- GridViewDeletedEventArgs.cs
- OutOfProcStateClientManager.cs
- FaultBookmark.cs
- SqlFacetAttribute.cs
- EventDescriptor.cs
- Model3DCollection.cs
- RegistrySecurity.cs
- BamlRecordHelper.cs
- DataRelationPropertyDescriptor.cs
- ByteAnimationBase.cs
- HtmlValidationSummaryAdapter.cs
- CompiledAction.cs
- LockCookie.cs
- DocumentViewerConstants.cs
- WebPartActionVerb.cs
- CqlErrorHelper.cs
- Bitmap.cs
- MissingMemberException.cs
- MessageLoggingElement.cs
- Command.cs
- DataSourceCache.cs
- ReceiveParametersContent.cs
- DataBindingHandlerAttribute.cs
- XdrBuilder.cs
- DbgCompiler.cs
- AttributeProviderAttribute.cs
- HashCodeCombiner.cs
- Base64Decoder.cs
- DeviceSpecific.cs
- Simplifier.cs
- ContentPresenter.cs