Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / WorkflowViewManager.cs / 1407647 / WorkflowViewManager.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Documents; using System.Activities.Presentation; using System.Activities.Presentation.View; using System.Activities.Presentation.Hosting; using System.Activities.Presentation.Model; using System.Activities.Presentation.Documents; using System.Activities.Presentation.Services; using System.Collections.ObjectModel; using System.Collections; using System.Collections.Generic; using System.Runtime; using System.ComponentModel.Design; internal class WorkflowViewManager : ViewManager { const string KeywordForWorkflowDesignerHomePage = "DefaultWorkflowDesigner"; EditingContext context; Selection oldSelection; ModelService modelService; System.Activities.Presentation.View.DesignerView view; AttachedPropertyisPrimarySelectionProperty; AttachedProperty isSelectionProperty; IIntegratedHelpService helpService; public override System.Windows.Media.Visual View { get { return view; } } public override void Initialize(EditingContext context) { this.context = context; AttachedPropertiesService propertiesService = this.context.Services.GetService (); helpService = this.context.Services.GetService (); oldSelection = this.context.Items.GetValue (); isPrimarySelectionProperty = new AttachedProperty () { Getter = (modelItem) => (this.context.Items.GetValue ().PrimarySelection == modelItem), Name = "IsPrimarySelection", OwnerType = typeof(Object) }; isSelectionProperty = new AttachedProperty () { Getter = (modelItem) => (((IList)this.context.Items.GetValue ().SelectedObjects).Contains(modelItem)), Name = "IsSelection", OwnerType = typeof(Object) }; propertiesService.AddProperty(isPrimarySelectionProperty); propertiesService.AddProperty(isSelectionProperty); if (this.context.Services.GetService () == null) { view = new System.Activities.Presentation.View.DesignerView(this.context); WorkflowViewService viewService = new WorkflowViewService(context); WorkflowViewStateService viewStateService = new WorkflowViewStateService(context); this.context.Services.Publish (viewService); this.context.Services.Publish (new VirtualizedContainerService(this.context)); this.context.Services.Publish (viewStateService); this.context.Services.Publish (view); this.context.Services.Subscribe (delegate(ModelService modelService) { this.modelService = modelService; if (modelService.Root != null) { view.MakeRootDesigner(modelService.Root); } view.RestoreDesignerStates(); this.context.Items.Subscribe (new SubscribeContextCallback (OnItemSelected)); }); } if (helpService != null) { helpService.AddContextAttribute(string.Empty, KeywordForWorkflowDesignerHomePage, HelpKeywordType.F1Keyword); } } internal static string GetF1HelpTypeKeyword(Type type) { Fx.Assert(type != null, "type is null"); if (type.IsGenericType) { Type genericTypeDefinition = type.GetGenericTypeDefinition(); return genericTypeDefinition.FullName; } return type.FullName; } void OnItemSelected(Selection newSelection) { Fx.Assert(newSelection != null, "newSelection is null"); IList newSelectionObjects = newSelection.SelectedObjects as IList ; IList oldSelectionObjects = oldSelection.SelectedObjects as IList ; //Call notifyPropertyChanged for IsPrimarySelection attached property. if (newSelection.PrimarySelection != null && !newSelection.PrimarySelection.Equals(oldSelection.PrimarySelection)) { isPrimarySelectionProperty.NotifyPropertyChanged(oldSelection.PrimarySelection); isPrimarySelectionProperty.NotifyPropertyChanged(newSelection.PrimarySelection); } else if (newSelection.PrimarySelection == null) { isPrimarySelectionProperty.NotifyPropertyChanged(oldSelection.PrimarySelection); } //call NotifyPropertyChanged for IsSelection property on ModelItems that were added or removed from selection. HashSet selectionChangeSet = new HashSet (oldSelectionObjects); selectionChangeSet.SymmetricExceptWith(newSelectionObjects); foreach (ModelItem selectionChangeMI in selectionChangeSet) { isSelectionProperty.NotifyPropertyChanged(selectionChangeMI); } if (helpService != null) { if (oldSelection.PrimarySelection != null) { helpService.RemoveContextAttribute(string.Empty, GetF1HelpTypeKeyword(oldSelection.PrimarySelection.ItemType)); } if (newSelection.PrimarySelection != null) { helpService.AddContextAttribute(string.Empty, GetF1HelpTypeKeyword(newSelection.PrimarySelection.ItemType), HelpKeywordType.F1Keyword); } } oldSelection = newSelection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Documents; using System.Activities.Presentation; using System.Activities.Presentation.View; using System.Activities.Presentation.Hosting; using System.Activities.Presentation.Model; using System.Activities.Presentation.Documents; using System.Activities.Presentation.Services; using System.Collections.ObjectModel; using System.Collections; using System.Collections.Generic; using System.Runtime; using System.ComponentModel.Design; internal class WorkflowViewManager : ViewManager { const string KeywordForWorkflowDesignerHomePage = "DefaultWorkflowDesigner"; EditingContext context; Selection oldSelection; ModelService modelService; System.Activities.Presentation.View.DesignerView view; AttachedProperty isPrimarySelectionProperty; AttachedProperty isSelectionProperty; IIntegratedHelpService helpService; public override System.Windows.Media.Visual View { get { return view; } } public override void Initialize(EditingContext context) { this.context = context; AttachedPropertiesService propertiesService = this.context.Services.GetService (); helpService = this.context.Services.GetService (); oldSelection = this.context.Items.GetValue (); isPrimarySelectionProperty = new AttachedProperty () { Getter = (modelItem) => (this.context.Items.GetValue ().PrimarySelection == modelItem), Name = "IsPrimarySelection", OwnerType = typeof(Object) }; isSelectionProperty = new AttachedProperty () { Getter = (modelItem) => (((IList)this.context.Items.GetValue ().SelectedObjects).Contains(modelItem)), Name = "IsSelection", OwnerType = typeof(Object) }; propertiesService.AddProperty(isPrimarySelectionProperty); propertiesService.AddProperty(isSelectionProperty); if (this.context.Services.GetService () == null) { view = new System.Activities.Presentation.View.DesignerView(this.context); WorkflowViewService viewService = new WorkflowViewService(context); WorkflowViewStateService viewStateService = new WorkflowViewStateService(context); this.context.Services.Publish (viewService); this.context.Services.Publish (new VirtualizedContainerService(this.context)); this.context.Services.Publish (viewStateService); this.context.Services.Publish (view); this.context.Services.Subscribe (delegate(ModelService modelService) { this.modelService = modelService; if (modelService.Root != null) { view.MakeRootDesigner(modelService.Root); } view.RestoreDesignerStates(); this.context.Items.Subscribe (new SubscribeContextCallback (OnItemSelected)); }); } if (helpService != null) { helpService.AddContextAttribute(string.Empty, KeywordForWorkflowDesignerHomePage, HelpKeywordType.F1Keyword); } } internal static string GetF1HelpTypeKeyword(Type type) { Fx.Assert(type != null, "type is null"); if (type.IsGenericType) { Type genericTypeDefinition = type.GetGenericTypeDefinition(); return genericTypeDefinition.FullName; } return type.FullName; } void OnItemSelected(Selection newSelection) { Fx.Assert(newSelection != null, "newSelection is null"); IList newSelectionObjects = newSelection.SelectedObjects as IList ; IList oldSelectionObjects = oldSelection.SelectedObjects as IList ; //Call notifyPropertyChanged for IsPrimarySelection attached property. if (newSelection.PrimarySelection != null && !newSelection.PrimarySelection.Equals(oldSelection.PrimarySelection)) { isPrimarySelectionProperty.NotifyPropertyChanged(oldSelection.PrimarySelection); isPrimarySelectionProperty.NotifyPropertyChanged(newSelection.PrimarySelection); } else if (newSelection.PrimarySelection == null) { isPrimarySelectionProperty.NotifyPropertyChanged(oldSelection.PrimarySelection); } //call NotifyPropertyChanged for IsSelection property on ModelItems that were added or removed from selection. HashSet selectionChangeSet = new HashSet (oldSelectionObjects); selectionChangeSet.SymmetricExceptWith(newSelectionObjects); foreach (ModelItem selectionChangeMI in selectionChangeSet) { isSelectionProperty.NotifyPropertyChanged(selectionChangeMI); } if (helpService != null) { if (oldSelection.PrimarySelection != null) { helpService.RemoveContextAttribute(string.Empty, GetF1HelpTypeKeyword(oldSelection.PrimarySelection.ItemType)); } if (newSelection.PrimarySelection != null) { helpService.AddContextAttribute(string.Empty, GetF1HelpTypeKeyword(newSelection.PrimarySelection.ItemType), HelpKeywordType.F1Keyword); } } oldSelection = newSelection; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
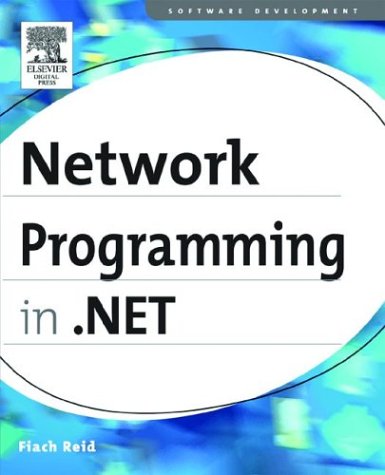
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _NestedMultipleAsyncResult.cs
- CharEnumerator.cs
- JulianCalendar.cs
- ListViewInsertionMark.cs
- OdbcConnection.cs
- SolidColorBrush.cs
- SystemUnicastIPAddressInformation.cs
- Speller.cs
- OperationFormatUse.cs
- JsonEncodingStreamWrapper.cs
- PagerStyle.cs
- DocumentCollection.cs
- WebServiceHandlerFactory.cs
- IndentTextWriter.cs
- entityreference_tresulttype.cs
- AtlasWeb.Designer.cs
- SafeBitVector32.cs
- BamlRecords.cs
- ForceCopyBuildProvider.cs
- ExpandableObjectConverter.cs
- CustomExpressionEventArgs.cs
- ToolboxService.cs
- CapabilitiesAssignment.cs
- VideoDrawing.cs
- ColorConverter.cs
- WorkerRequest.cs
- DeadLetterQueue.cs
- AutomationPeer.cs
- ValidationSummary.cs
- ArgumentDesigner.xaml.cs
- ToolZone.cs
- RequiredFieldValidator.cs
- PhysicalAddress.cs
- MarkupObject.cs
- WebControlsSection.cs
- ResetableIterator.cs
- DeviceContext.cs
- VectorCollection.cs
- ServiceHostFactory.cs
- StorageFunctionMapping.cs
- VBIdentifierNameEditor.cs
- WebHttpElement.cs
- TextEffectCollection.cs
- ProcessModuleCollection.cs
- TypeDescriptionProvider.cs
- ViewGenerator.cs
- SchemaNames.cs
- SspiNegotiationTokenAuthenticatorState.cs
- TextCompositionManager.cs
- Range.cs
- GridViewSelectEventArgs.cs
- ContentFilePart.cs
- PropertyEmitterBase.cs
- ToolStripArrowRenderEventArgs.cs
- XmlProcessingInstruction.cs
- BaseParaClient.cs
- AttributeQuery.cs
- IISUnsafeMethods.cs
- ToolStripProgressBar.cs
- TripleDES.cs
- PeerEndPoint.cs
- LinqDataSourceDeleteEventArgs.cs
- MenuItemStyle.cs
- NotificationContext.cs
- ServiceHostFactory.cs
- MobileRedirect.cs
- XsdDuration.cs
- LogStore.cs
- WebCategoryAttribute.cs
- StringReader.cs
- MultipleViewPattern.cs
- TextBoxLine.cs
- ViewManager.cs
- DataControlPagerLinkButton.cs
- SoapTypeAttribute.cs
- TransactionOptions.cs
- WebPartConnectionsCancelVerb.cs
- NullRuntimeConfig.cs
- LogicalExpr.cs
- LinqDataSourceView.cs
- OdbcInfoMessageEvent.cs
- TemplateBuilder.cs
- ExternalException.cs
- ValueType.cs
- ValidatedMobileControlConverter.cs
- ResourcePool.cs
- TableLayoutCellPaintEventArgs.cs
- EventProviderClassic.cs
- GuidTagList.cs
- COM2IDispatchConverter.cs
- KeyPressEvent.cs
- PositiveTimeSpanValidator.cs
- XmlSchemaGroupRef.cs
- GraphicsContext.cs
- ClrPerspective.cs
- SafeLocalMemHandle.cs
- LassoHelper.cs
- ListViewAutomationPeer.cs
- MultiBinding.cs
- AnnotationAuthorChangedEventArgs.cs