Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / OnOperation.cs / 1305376 / OnOperation.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Objects.DataClasses; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an OnDelete, OnCopy, OnSecure, OnLock or OnSerialize element /// internal sealed class OnOperation : SchemaElement { private Operation _Operation; private Action _Action; ////// /// /// /// public OnOperation(RelationshipEnd parentElement, Operation operation) : base(parentElement) { Operation = operation; } ////// The operation /// public Operation Operation { get { return _Operation; } private set { _Operation = value; } } ////// The action /// public Action Action { get { return _Action; } private set { _Action = value; } } protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.Action)) { HandleActionAttribute(reader); return true; } return false; } ////// Handle the Action attribute /// /// reader positioned at Action attribute private void HandleActionAttribute(XmlReader reader) { Debug.Assert(reader != null); RelationshipKind relationshipKind = ParentElement.ParentElement.RelationshipKind; switch ( reader.Value.Trim() ) { case "None": Action = Action.None; break; case "Cascade": Action = Action.Cascade; break; default: AddError( ErrorCode.InvalidAction, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.InvalidAction(reader.Value, ParentElement.FQName ) ); break; } } ////// the parent element. /// private new RelationshipEnd ParentElement { get { return (RelationshipEnd)base.ParentElement; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Objects.DataClasses; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an OnDelete, OnCopy, OnSecure, OnLock or OnSerialize element /// internal sealed class OnOperation : SchemaElement { private Operation _Operation; private Action _Action; ////// /// /// /// public OnOperation(RelationshipEnd parentElement, Operation operation) : base(parentElement) { Operation = operation; } ////// The operation /// public Operation Operation { get { return _Operation; } private set { _Operation = value; } } ////// The action /// public Action Action { get { return _Action; } private set { _Action = value; } } protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.Action)) { HandleActionAttribute(reader); return true; } return false; } ////// Handle the Action attribute /// /// reader positioned at Action attribute private void HandleActionAttribute(XmlReader reader) { Debug.Assert(reader != null); RelationshipKind relationshipKind = ParentElement.ParentElement.RelationshipKind; switch ( reader.Value.Trim() ) { case "None": Action = Action.None; break; case "Cascade": Action = Action.Cascade; break; default: AddError( ErrorCode.InvalidAction, EdmSchemaErrorSeverity.Error, reader, System.Data.Entity.Strings.InvalidAction(reader.Value, ParentElement.FQName ) ); break; } } ////// the parent element. /// private new RelationshipEnd ParentElement { get { return (RelationshipEnd)base.ParentElement; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
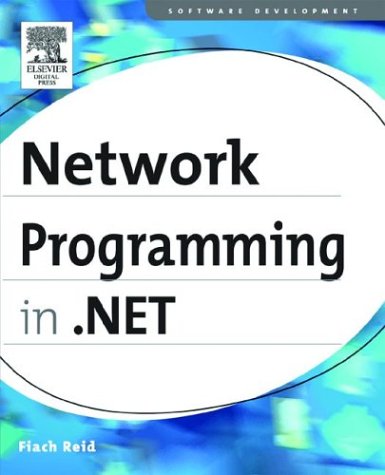
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CngProvider.cs
- HighlightComponent.cs
- Attributes.cs
- TypeInitializationException.cs
- HttpHandlerActionCollection.cs
- RewritingValidator.cs
- x509utils.cs
- ProxyElement.cs
- CorrelationManager.cs
- TextSpanModifier.cs
- DateTimeValueSerializer.cs
- SqlXml.cs
- SqlCrossApplyToCrossJoin.cs
- KnownIds.cs
- cookie.cs
- CircleHotSpot.cs
- PrivilegeNotHeldException.cs
- ServiceOperationHelpers.cs
- SQLDecimalStorage.cs
- SmuggledIUnknown.cs
- DrawingContextWalker.cs
- LazyTextWriterCreator.cs
- PersistenceParticipant.cs
- EventHandlersDesigner.cs
- PrintingPermission.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- DetailsViewDeletedEventArgs.cs
- SafeHandles.cs
- ActivityTypeDesigner.xaml.cs
- WindowsFormsSynchronizationContext.cs
- ModuleElement.cs
- NumericUpDownAcceleration.cs
- MimeParameters.cs
- ApplyHostConfigurationBehavior.cs
- DataStreams.cs
- InstalledFontCollection.cs
- InterleavedZipPartStream.cs
- WindowsAuthenticationEventArgs.cs
- TextMarkerSource.cs
- BindingNavigatorDesigner.cs
- ConstantProjectedSlot.cs
- SqlDataSource.cs
- SymmetricKeyWrap.cs
- followingquery.cs
- Condition.cs
- UncommonField.cs
- HttpConfigurationContext.cs
- EllipticalNodeOperations.cs
- TypeConverter.cs
- SrgsNameValueTag.cs
- EventPrivateKey.cs
- AdCreatedEventArgs.cs
- DataGridViewComboBoxColumn.cs
- NamedElement.cs
- Window.cs
- DependencyPropertyDescriptor.cs
- RoleGroupCollection.cs
- ContentType.cs
- CompositeControl.cs
- ControlBindingsCollection.cs
- SyndicationDeserializer.cs
- XamlWriter.cs
- ListViewSelectEventArgs.cs
- VScrollBar.cs
- DataViewListener.cs
- CompilerGlobalScopeAttribute.cs
- COM2TypeInfoProcessor.cs
- LifetimeServices.cs
- ListChangedEventArgs.cs
- CompilerErrorCollection.cs
- CacheEntry.cs
- AppDomainCompilerProxy.cs
- BamlRecords.cs
- ExpressionConverter.cs
- SystemBrushes.cs
- ExcCanonicalXml.cs
- HwndAppCommandInputProvider.cs
- EncoderFallback.cs
- SelectionRangeConverter.cs
- ToolBarButtonClickEvent.cs
- XXXOnTypeBuilderInstantiation.cs
- EdmProviderManifest.cs
- InfoCardBaseException.cs
- DataReaderContainer.cs
- DefaultSection.cs
- CheckBoxPopupAdapter.cs
- SecurityTokenAuthenticator.cs
- safex509handles.cs
- ProjectionCamera.cs
- DataSourceUtil.cs
- MaskDescriptors.cs
- TransformPattern.cs
- FtpRequestCacheValidator.cs
- HtmlEncodedRawTextWriter.cs
- ContainerVisual.cs
- ButtonRenderer.cs
- DetailsViewInsertedEventArgs.cs
- AssertFilter.cs
- UxThemeWrapper.cs
- FixedStringLookup.cs