Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / SSDLGenerator / RelationshipDetailsRow.cs / 1305376 / RelationshipDetailsRow.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Common; using System.Globalization; using System.Data; using System.Data.Entity.Design.Common; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed RelationshipDetail row /// internal sealed class RelationshipDetailsRow : System.Data.DataRow { private RelationshipDetailsCollection _tableRelationshipDetails; [System.Diagnostics.DebuggerNonUserCodeAttribute()] internal RelationshipDetailsRow(System.Data.DataRowBuilder rb) : base(rb) { this._tableRelationshipDetails = ((RelationshipDetailsCollection)(base.Table)); } ////// Gets a strongly typed table /// public new RelationshipDetailsCollection Table { get { return _tableRelationshipDetails; } } ////// Gets the PkCatalog column value /// public string PKCatalog { get { try { return ((string)(this[this._tableRelationshipDetails.PKCatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKCatalogColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKCatalogColumn] = value; } } ////// Gets the PkSchema column value /// public string PKSchema { get { try { return ((string)(this[this._tableRelationshipDetails.PKSchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKSchemaColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKSchemaColumn] = value; } } ////// Gets the PkTable column value /// public string PKTable { get { try { return ((string)(this[this._tableRelationshipDetails.PKTableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKTableColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKTableColumn] = value; } } ////// Gets the PkColumn column value /// public string PKColumn { get { try { return ((string)(this[this._tableRelationshipDetails.PKColumnColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKColumnColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKColumnColumn] = value; } } ////// Gets the FkCatalog column value /// public string FKCatalog { get { try { return ((string)(this[this._tableRelationshipDetails.FKCatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKCatalogColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKCatalogColumn] = value; } } ////// Gets the FkSchema column value /// public string FKSchema { get { try { return ((string)(this[this._tableRelationshipDetails.FKSchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKSchemaColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKSchemaColumn] = value; } } ////// Gets the FkTable column value /// public string FKTable { get { try { return ((string)(this[this._tableRelationshipDetails.FKTableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKTableColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKTableColumn] = value; } } ////// Gets the FkColumn column value /// public string FKColumn { get { try { return ((string)(this[this._tableRelationshipDetails.FKColumnColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKColumnColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKColumnColumn] = value; } } ////// Gets the Ordinal column value /// public int Ordinal { get { try { return ((int)(this[this._tableRelationshipDetails.OrdinalColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.OrdinalColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.OrdinalColumn] = value; } } ////// Gets the RelationshipName column value /// public string RelationshipName { get { try { return ((string)(this[this._tableRelationshipDetails.RelationshipNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.RelationshipNameColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.RelationshipNameColumn] = value; } } ////// Gets the RelationshipName column value /// public string RelationshipId { get { try { return ((string)(this[this._tableRelationshipDetails.RelationshipIdColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.RelationshipIdColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.RelationshipIdColumn] = value; } } ////// Gets the IsCascadeDelete column value /// public bool RelationshipIsCascadeDelete { get { try { return ((bool)(this[this._tableRelationshipDetails.RelationshipIsCascadeDeleteColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.RelationshipIsCascadeDeleteColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.RelationshipIsCascadeDeleteColumn] = value; } } ////// Determines if the PkCatalog column value is null /// ///true if the value is null, otherwise false. public bool IsPKCatalogNull() { return this.IsNull(this._tableRelationshipDetails.PKCatalogColumn); } ////// Determines if the PkSchema column value is null /// ///true if the value is null, otherwise false. public bool IsPKSchemaNull() { return this.IsNull(this._tableRelationshipDetails.PKSchemaColumn); } ////// Determines if the PkTable column value is null /// ///true if the value is null, otherwise false. public bool IsPKTableNull() { return this.IsNull(this._tableRelationshipDetails.PKTableColumn); } ////// Determines if the PkColumn column value is null /// ///true if the value is null, otherwise false. public bool IsPKColumnNull() { return this.IsNull(this._tableRelationshipDetails.PKColumnColumn); } ////// Determines if the FkCatalog column value is null /// ///true if the value is null, otherwise false. public bool IsFKCatalogNull() { return this.IsNull(this._tableRelationshipDetails.FKCatalogColumn); } ////// Determines if the FkSchema column value is null /// ///true if the value is null, otherwise false. public bool IsFKSchemaNull() { return this.IsNull(this._tableRelationshipDetails.FKSchemaColumn); } ////// Determines if the FkTable column value is null /// ///true if the value is null, otherwise false. public bool IsFKTableNull() { return this.IsNull(this._tableRelationshipDetails.FKTableColumn); } ////// Determines if the FkColumn column value is null /// ///true if the value is null, otherwise false. public bool IsFKColumnNull() { return this.IsNull(this._tableRelationshipDetails.FKColumnColumn); } ////// Determines if the Ordinal column value is null /// ///true if the value is null, otherwise false. public bool IsOrdinalNull() { return this.IsNull(this._tableRelationshipDetails.OrdinalColumn); } ////// Determines if the RelationshipName column value is null /// ///true if the value is null, otherwise false. public bool IsRelationshipNameNull() { return this.IsNull(this._tableRelationshipDetails.RelationshipNameColumn); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Common; using System.Globalization; using System.Data; using System.Data.Entity.Design.Common; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed RelationshipDetail row /// internal sealed class RelationshipDetailsRow : System.Data.DataRow { private RelationshipDetailsCollection _tableRelationshipDetails; [System.Diagnostics.DebuggerNonUserCodeAttribute()] internal RelationshipDetailsRow(System.Data.DataRowBuilder rb) : base(rb) { this._tableRelationshipDetails = ((RelationshipDetailsCollection)(base.Table)); } ////// Gets a strongly typed table /// public new RelationshipDetailsCollection Table { get { return _tableRelationshipDetails; } } ////// Gets the PkCatalog column value /// public string PKCatalog { get { try { return ((string)(this[this._tableRelationshipDetails.PKCatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKCatalogColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKCatalogColumn] = value; } } ////// Gets the PkSchema column value /// public string PKSchema { get { try { return ((string)(this[this._tableRelationshipDetails.PKSchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKSchemaColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKSchemaColumn] = value; } } ////// Gets the PkTable column value /// public string PKTable { get { try { return ((string)(this[this._tableRelationshipDetails.PKTableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKTableColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKTableColumn] = value; } } ////// Gets the PkColumn column value /// public string PKColumn { get { try { return ((string)(this[this._tableRelationshipDetails.PKColumnColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.PKColumnColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.PKColumnColumn] = value; } } ////// Gets the FkCatalog column value /// public string FKCatalog { get { try { return ((string)(this[this._tableRelationshipDetails.FKCatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKCatalogColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKCatalogColumn] = value; } } ////// Gets the FkSchema column value /// public string FKSchema { get { try { return ((string)(this[this._tableRelationshipDetails.FKSchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKSchemaColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKSchemaColumn] = value; } } ////// Gets the FkTable column value /// public string FKTable { get { try { return ((string)(this[this._tableRelationshipDetails.FKTableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKTableColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKTableColumn] = value; } } ////// Gets the FkColumn column value /// public string FKColumn { get { try { return ((string)(this[this._tableRelationshipDetails.FKColumnColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.FKColumnColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.FKColumnColumn] = value; } } ////// Gets the Ordinal column value /// public int Ordinal { get { try { return ((int)(this[this._tableRelationshipDetails.OrdinalColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.OrdinalColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.OrdinalColumn] = value; } } ////// Gets the RelationshipName column value /// public string RelationshipName { get { try { return ((string)(this[this._tableRelationshipDetails.RelationshipNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.RelationshipNameColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.RelationshipNameColumn] = value; } } ////// Gets the RelationshipName column value /// public string RelationshipId { get { try { return ((string)(this[this._tableRelationshipDetails.RelationshipIdColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.RelationshipIdColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.RelationshipIdColumn] = value; } } ////// Gets the IsCascadeDelete column value /// public bool RelationshipIsCascadeDelete { get { try { return ((bool)(this[this._tableRelationshipDetails.RelationshipIsCascadeDeleteColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableRelationshipDetails.RelationshipIsCascadeDeleteColumn.ColumnName, _tableRelationshipDetails.TableName, e); } } set { this[this._tableRelationshipDetails.RelationshipIsCascadeDeleteColumn] = value; } } ////// Determines if the PkCatalog column value is null /// ///true if the value is null, otherwise false. public bool IsPKCatalogNull() { return this.IsNull(this._tableRelationshipDetails.PKCatalogColumn); } ////// Determines if the PkSchema column value is null /// ///true if the value is null, otherwise false. public bool IsPKSchemaNull() { return this.IsNull(this._tableRelationshipDetails.PKSchemaColumn); } ////// Determines if the PkTable column value is null /// ///true if the value is null, otherwise false. public bool IsPKTableNull() { return this.IsNull(this._tableRelationshipDetails.PKTableColumn); } ////// Determines if the PkColumn column value is null /// ///true if the value is null, otherwise false. public bool IsPKColumnNull() { return this.IsNull(this._tableRelationshipDetails.PKColumnColumn); } ////// Determines if the FkCatalog column value is null /// ///true if the value is null, otherwise false. public bool IsFKCatalogNull() { return this.IsNull(this._tableRelationshipDetails.FKCatalogColumn); } ////// Determines if the FkSchema column value is null /// ///true if the value is null, otherwise false. public bool IsFKSchemaNull() { return this.IsNull(this._tableRelationshipDetails.FKSchemaColumn); } ////// Determines if the FkTable column value is null /// ///true if the value is null, otherwise false. public bool IsFKTableNull() { return this.IsNull(this._tableRelationshipDetails.FKTableColumn); } ////// Determines if the FkColumn column value is null /// ///true if the value is null, otherwise false. public bool IsFKColumnNull() { return this.IsNull(this._tableRelationshipDetails.FKColumnColumn); } ////// Determines if the Ordinal column value is null /// ///true if the value is null, otherwise false. public bool IsOrdinalNull() { return this.IsNull(this._tableRelationshipDetails.OrdinalColumn); } ////// Determines if the RelationshipName column value is null /// ///true if the value is null, otherwise false. public bool IsRelationshipNameNull() { return this.IsNull(this._tableRelationshipDetails.RelationshipNameColumn); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
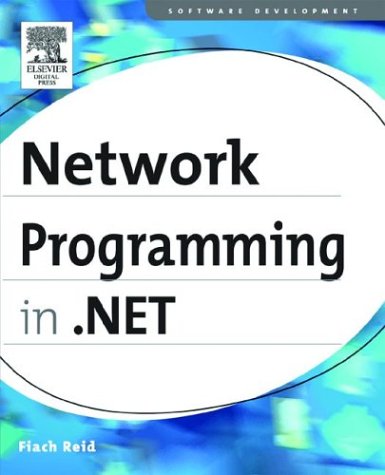
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StrokeRenderer.cs
- BeginStoryboard.cs
- FormattedTextSymbols.cs
- SessionState.cs
- CompositeControl.cs
- PropertyMappingExceptionEventArgs.cs
- milrender.cs
- OptimizedTemplateContentHelper.cs
- MailWebEventProvider.cs
- GeneratedCodeAttribute.cs
- DataGridItemEventArgs.cs
- DesignTimeParseData.cs
- ConnectionConsumerAttribute.cs
- SecondaryIndex.cs
- TemplateColumn.cs
- PointHitTestResult.cs
- XmlSerializationGeneratedCode.cs
- CodeLabeledStatement.cs
- TypeDescriptorFilterService.cs
- DomainUpDown.cs
- ToolStripSeparatorRenderEventArgs.cs
- ConnectionManager.cs
- WinOEToolBoxItem.cs
- SoapAttributeAttribute.cs
- SqlDelegatedTransaction.cs
- ListBoxItemWrapperAutomationPeer.cs
- SpellerHighlightLayer.cs
- regiisutil.cs
- HeaderPanel.cs
- AttributeProviderAttribute.cs
- PrinterUnitConvert.cs
- MessageSecurityOverHttpElement.cs
- DataObjectAttribute.cs
- DetailsViewInsertedEventArgs.cs
- AsyncContentLoadedEventArgs.cs
- DetailsViewDeletedEventArgs.cs
- System.Data.OracleClient_BID.cs
- StylusPointPropertyUnit.cs
- ConstantProjectedSlot.cs
- DataBindingExpressionBuilder.cs
- StylusDevice.cs
- ZipPackagePart.cs
- RenderData.cs
- PrivateFontCollection.cs
- TextContainer.cs
- ConditionValidator.cs
- TransactionTable.cs
- ISO2022Encoding.cs
- ReflectPropertyDescriptor.cs
- RegexReplacement.cs
- FirstQueryOperator.cs
- SrgsSubset.cs
- OutputCacheProfile.cs
- SafeArrayTypeMismatchException.cs
- GenericIdentity.cs
- TreeNodeCollection.cs
- HealthMonitoringSectionHelper.cs
- ExecutionContext.cs
- SqlTypeSystemProvider.cs
- TableDesigner.cs
- ParameterElementCollection.cs
- ListViewUpdatedEventArgs.cs
- RelationshipWrapper.cs
- ColumnMapProcessor.cs
- TextPointerBase.cs
- SHA256.cs
- SafeArchiveContext.cs
- BinaryKeyIdentifierClause.cs
- BindingBase.cs
- NativeMethods.cs
- DesignerCategoryAttribute.cs
- ImageBrush.cs
- FrameworkTextComposition.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- ImageKeyConverter.cs
- VariableQuery.cs
- WebPageTraceListener.cs
- TextParagraphProperties.cs
- ModuleBuilder.cs
- PixelFormat.cs
- ProfileModule.cs
- ItemsControlAutomationPeer.cs
- HttpHeaderCollection.cs
- regiisutil.cs
- AttributeUsageAttribute.cs
- RegionData.cs
- DBCommandBuilder.cs
- ServiceHttpModule.cs
- EntityCommandExecutionException.cs
- CacheOutputQuery.cs
- TypeUsage.cs
- CodeMemberField.cs
- AxHost.cs
- MoveSizeWinEventHandler.cs
- BinHexDecoder.cs
- FontSourceCollection.cs
- EntityDataSourceContextCreatedEventArgs.cs
- NetworkCredential.cs
- RequestNavigateEventArgs.cs
- DeferredElementTreeState.cs