Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / DnsPermission.cs / 1305376 / DnsPermission.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Security; using System.Security.Permissions; using System.Globalization; //NOTE: While DnsPermissionAttribute resides in System.DLL, // no classes from that DLL are able to make declarative usage of DnsPermission. [ AttributeUsage( AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] [Serializable()] sealed public class DnsPermissionAttribute : CodeAccessSecurityAttribute { public DnsPermissionAttribute ( SecurityAction action ): base( action ) { } public override IPermission CreatePermission() { if (Unrestricted) { return new DnsPermission( PermissionState.Unrestricted); } else { return new DnsPermission( PermissionState.None); } } } ////// [Serializable] public sealed class DnsPermission : CodeAccessPermission, IUnrestrictedPermission { private bool m_noRestriction; ////// ////// public DnsPermission(PermissionState state) { m_noRestriction = (state==PermissionState.Unrestricted); } internal DnsPermission(bool free) { m_noRestriction = free; } // IUnrestrictedPermission interface methods ////// Creates a new instance of the ////// class that passes all demands or that fails all demands. /// /// public bool IsUnrestricted() { return m_noRestriction; } // IPermission interface methods ////// Checks the overall permission state of the object. /// ////// public override IPermission Copy () { return new DnsPermission(m_noRestriction); } ////// Creates a copy of a ///instance. /// /// public override IPermission Union(IPermission target) { // Pattern suggested by Security engine if (target==null) { return this.Copy(); } DnsPermission other = target as DnsPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } return new DnsPermission(m_noRestriction || other.m_noRestriction); } ///Returns the logical union between two ///instances. /// public override IPermission Intersect(IPermission target) { // Pattern suggested by Security engine if (target==null) { return null; } DnsPermission other = target as DnsPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } // return null if resulting permission is restricted and empty // Hence, the only way for a bool permission will be. if (this.m_noRestriction && other.m_noRestriction) { return new DnsPermission(true); } return null; } ///Returns the logical intersection between two ///instances. /// public override bool IsSubsetOf(IPermission target) { // Pattern suggested by Security engine if (target == null) { return m_noRestriction == false; } DnsPermission other = target as DnsPermission; if (other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } //Here is the matrix of result based on m_noRestriction for me and she // me.noRestriction she.noRestriction me.isSubsetOf(she) // 0 0 1 // 0 1 1 // 1 0 0 // 1 1 1 return (!m_noRestriction || other.m_noRestriction); } ///Compares two ///instances. /// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { // // null SecurityElement // throw new ArgumentNullException("securityElement"); } if (!securityElement.Tag.Equals("IPermission")) { // // SecurityElement must be a permission element // throw new ArgumentException(SR.GetString(SR.net_no_classname), "securityElement"); } string className = securityElement.Attribute( "class" ); if (className == null) { // // SecurityElement must be a permission element for this type // throw new ArgumentException(SR.GetString(SR.net_no_classname), "securityElement"); } if (className.IndexOf( this.GetType().FullName ) < 0) { // // SecurityElement must be a permission element for this type // throw new ArgumentException(SR.GetString(SR.net_no_typename), "securityElement"); } string str = securityElement.Attribute( "Unrestricted" ); m_noRestriction = (str!=null?(0 == string.Compare( str, "true", StringComparison.OrdinalIgnoreCase)):false); } ////// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement( "IPermission" ); securityElement.AddAttribute( "class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace( '\"', '\'' ) ); securityElement.AddAttribute( "version", "1" ); if (m_noRestriction) { securityElement.AddAttribute( "Unrestricted", "true" ); } return securityElement; } } // class DnsPermission } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Security; using System.Security.Permissions; using System.Globalization; //NOTE: While DnsPermissionAttribute resides in System.DLL, // no classes from that DLL are able to make declarative usage of DnsPermission. [ AttributeUsage( AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false )] [Serializable()] sealed public class DnsPermissionAttribute : CodeAccessSecurityAttribute { public DnsPermissionAttribute ( SecurityAction action ): base( action ) { } public override IPermission CreatePermission() { if (Unrestricted) { return new DnsPermission( PermissionState.Unrestricted); } else { return new DnsPermission( PermissionState.None); } } } ////// [Serializable] public sealed class DnsPermission : CodeAccessPermission, IUnrestrictedPermission { private bool m_noRestriction; ////// ////// public DnsPermission(PermissionState state) { m_noRestriction = (state==PermissionState.Unrestricted); } internal DnsPermission(bool free) { m_noRestriction = free; } // IUnrestrictedPermission interface methods ////// Creates a new instance of the ////// class that passes all demands or that fails all demands. /// /// public bool IsUnrestricted() { return m_noRestriction; } // IPermission interface methods ////// Checks the overall permission state of the object. /// ////// public override IPermission Copy () { return new DnsPermission(m_noRestriction); } ////// Creates a copy of a ///instance. /// /// public override IPermission Union(IPermission target) { // Pattern suggested by Security engine if (target==null) { return this.Copy(); } DnsPermission other = target as DnsPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } return new DnsPermission(m_noRestriction || other.m_noRestriction); } ///Returns the logical union between two ///instances. /// public override IPermission Intersect(IPermission target) { // Pattern suggested by Security engine if (target==null) { return null; } DnsPermission other = target as DnsPermission; if(other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } // return null if resulting permission is restricted and empty // Hence, the only way for a bool permission will be. if (this.m_noRestriction && other.m_noRestriction) { return new DnsPermission(true); } return null; } ///Returns the logical intersection between two ///instances. /// public override bool IsSubsetOf(IPermission target) { // Pattern suggested by Security engine if (target == null) { return m_noRestriction == false; } DnsPermission other = target as DnsPermission; if (other == null) { throw new ArgumentException(SR.GetString(SR.net_perm_target), "target"); } //Here is the matrix of result based on m_noRestriction for me and she // me.noRestriction she.noRestriction me.isSubsetOf(she) // 0 0 1 // 0 1 1 // 1 0 0 // 1 1 1 return (!m_noRestriction || other.m_noRestriction); } ///Compares two ///instances. /// public override void FromXml(SecurityElement securityElement) { if (securityElement == null) { // // null SecurityElement // throw new ArgumentNullException("securityElement"); } if (!securityElement.Tag.Equals("IPermission")) { // // SecurityElement must be a permission element // throw new ArgumentException(SR.GetString(SR.net_no_classname), "securityElement"); } string className = securityElement.Attribute( "class" ); if (className == null) { // // SecurityElement must be a permission element for this type // throw new ArgumentException(SR.GetString(SR.net_no_classname), "securityElement"); } if (className.IndexOf( this.GetType().FullName ) < 0) { // // SecurityElement must be a permission element for this type // throw new ArgumentException(SR.GetString(SR.net_no_typename), "securityElement"); } string str = securityElement.Attribute( "Unrestricted" ); m_noRestriction = (str!=null?(0 == string.Compare( str, "true", StringComparison.OrdinalIgnoreCase)):false); } ////// public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement( "IPermission" ); securityElement.AddAttribute( "class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace( '\"', '\'' ) ); securityElement.AddAttribute( "version", "1" ); if (m_noRestriction) { securityElement.AddAttribute( "Unrestricted", "true" ); } return securityElement; } } // class DnsPermission } // namespace System.Net // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
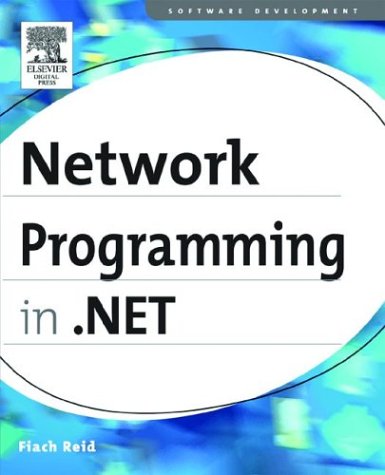
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlMethodCallConverter.cs
- CodeObject.cs
- NonPrimarySelectionGlyph.cs
- HtmlInputText.cs
- AdornerLayer.cs
- securitycriticaldataClass.cs
- BinaryWriter.cs
- Variant.cs
- OleDbRowUpdatingEvent.cs
- WebConfigurationHostFileChange.cs
- InternalConfigHost.cs
- ComPlusSynchronizationContext.cs
- DictionaryBase.cs
- NodeInfo.cs
- SHA256Managed.cs
- CodeExporter.cs
- EdmEntityTypeAttribute.cs
- DataControlField.cs
- ComboBoxRenderer.cs
- LayoutInformation.cs
- BoundsDrawingContextWalker.cs
- codemethodreferenceexpression.cs
- Method.cs
- BasicAsyncResult.cs
- LifetimeServices.cs
- DecryptRequest.cs
- RSAOAEPKeyExchangeDeformatter.cs
- XPathDocumentNavigator.cs
- AnonymousIdentificationSection.cs
- AppDomainUnloadedException.cs
- SoapEnumAttribute.cs
- Timer.cs
- WebConfigurationManager.cs
- Win32.cs
- SelectedCellsChangedEventArgs.cs
- HitTestResult.cs
- BamlVersionHeader.cs
- InstanceCreationEditor.cs
- SafeRightsManagementPubHandle.cs
- MaskDescriptors.cs
- EntitySetBaseCollection.cs
- WindowsFormsHostAutomationPeer.cs
- ShutDownListener.cs
- CroppedBitmap.cs
- EpmCustomContentDeSerializer.cs
- HandlerWithFactory.cs
- BrowserCapabilitiesCodeGenerator.cs
- InputChannelAcceptor.cs
- ReferenceSchema.cs
- GregorianCalendarHelper.cs
- Pen.cs
- TypeNameHelper.cs
- AstTree.cs
- WebPartVerb.cs
- TextOnlyOutput.cs
- LogEntryHeaderDeserializer.cs
- SystemInfo.cs
- ReaderOutput.cs
- TemplateComponentConnector.cs
- Pts.cs
- SchemaObjectWriter.cs
- HtmlInputPassword.cs
- SqlConnection.cs
- SetterBaseCollection.cs
- IdnElement.cs
- UidPropertyAttribute.cs
- EastAsianLunisolarCalendar.cs
- DataGridLinkButton.cs
- DataBoundControlParameterTarget.cs
- Canvas.cs
- AnnotationHighlightLayer.cs
- XmlNodeReader.cs
- Span.cs
- SqlFactory.cs
- IndexedEnumerable.cs
- FolderNameEditor.cs
- TiffBitmapEncoder.cs
- RSAProtectedConfigurationProvider.cs
- CodeSubDirectoriesCollection.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- JapaneseLunisolarCalendar.cs
- TreeWalkHelper.cs
- ObjectQuery.cs
- DateTimePicker.cs
- LinkConverter.cs
- IdentityValidationException.cs
- GPPOINT.cs
- HttpRawResponse.cs
- SimpleWorkerRequest.cs
- PolygonHotSpot.cs
- PartialCachingControl.cs
- RelationshipNavigation.cs
- StreamFormatter.cs
- infer.cs
- PackWebResponse.cs
- MediaScriptCommandRoutedEventArgs.cs
- SplitterCancelEvent.cs
- TimeSpan.cs
- MissingMemberException.cs
- GridViewDeleteEventArgs.cs