Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / Util / TemplateFactory.cs / 1305376 / TemplateFactory.cs
using System.Collections; using System.Diagnostics; using System.Globalization; using System.Web.Compilation; using System.Web.Hosting; using System.Web.Resources; namespace System.Web.DynamicData { internal class TemplateFactory { // Use Hashtable instead of Dictionary<,> because it is more thread safe private Hashtable _fieldTemplateVirtualPathCache = new Hashtable(); private string _defaultLocation; private string _templateFolderVirtualPath; internal MetaModel Model { get; set; } private bool _needToResolveVirtualPath; private bool _trackFolderChanges; private bool _registeredForChangeNotifications; private VirtualPathProvider _vpp; private bool _usingCustomVpp; internal TemplateFactory(string defaultLocation) : this(defaultLocation, true) { } internal TemplateFactory(string defaultLocation, bool trackFolderChanges) { Debug.Assert(!String.IsNullOrEmpty(defaultLocation)); _defaultLocation = defaultLocation; _trackFolderChanges = trackFolderChanges; } internal string TemplateFolderVirtualPath { get { if (_templateFolderVirtualPath == null) { // If not set, set its default location TemplateFolderVirtualPath = _defaultLocation; } if (_needToResolveVirtualPath) { // Make sure it ends with a slash _templateFolderVirtualPath = VirtualPathUtility.AppendTrailingSlash(_templateFolderVirtualPath); // If it's relative, make it relative to the Model's path // Note can be null under Unit Testing if (Model != null) { _templateFolderVirtualPath = VirtualPathUtility.Combine(Model.DynamicDataFolderVirtualPath, _templateFolderVirtualPath); } _needToResolveVirtualPath = false; } return _templateFolderVirtualPath; } set { _templateFolderVirtualPath = value; // Make sure we register for change notifications, since we just got a new path _registeredForChangeNotifications = false; // It may be relative and need resolution, but let's not do it until we need it _needToResolveVirtualPath = true; } } internal VirtualPathProvider VirtualPathProvider { get { if (_vpp == null) { _vpp = HostingEnvironment.VirtualPathProvider; } return _vpp; } set { _vpp = value; _usingCustomVpp = value != null; } } internal string GetTemplatePath(long cacheKey, FunctemplatePathFactoryFunction) { // Check if we already have it cached string virtualPath = this[cacheKey]; // null is a valid value, so we also need to check whether the key exists if (virtualPath == null && !ContainsKey(cacheKey)) { // It's not cached, so compute it and cache it. Make sure multiple writers are serialized virtualPath = templatePathFactoryFunction(); this[cacheKey] = virtualPath; } return virtualPath; } private string this[long cacheKey] { get { EnsureRegisteredForChangeNotifications(); return (string)_fieldTemplateVirtualPathCache[cacheKey]; } set { EnsureRegisteredForChangeNotifications(); lock (_fieldTemplateVirtualPathCache) { _fieldTemplateVirtualPathCache[cacheKey] = value; } } } private bool ContainsKey(long cacheKey) { EnsureRegisteredForChangeNotifications(); return _fieldTemplateVirtualPathCache.ContainsKey(cacheKey); } private void EnsureRegisteredForChangeNotifications() { if (!_trackFolderChanges) { return; } if (!_registeredForChangeNotifications) { lock (this) { if (!_registeredForChangeNotifications) { // Make sure the folder exists if (!VirtualPathProvider.DirectoryExists(TemplateFolderVirtualPath)) { throw new InvalidOperationException(String.Format( CultureInfo.CurrentCulture, DynamicDataResources.FieldTemplateFactory_FolderNotFound, TemplateFolderVirtualPath)); } // Register for notifications if anything in that folder changes FileChangeNotifier.Register(TemplateFolderVirtualPath, delegate(string path) { // Something has changed, so clear our cache lock (_fieldTemplateVirtualPathCache) { _fieldTemplateVirtualPathCache.Clear(); } }); _registeredForChangeNotifications = true; } } } } internal bool FileExists(string virtualPath) { #if ORYX_VNEXT // Checking file existence is tricky, because in non-updatable precompiled apps, the ascx files are not // there at all. In that case, it's still possible to check existence by calling BuildManager.GetCompiledType // and seeing if it throws, but this can cause a lot of first chance exceptions, which affect the debugging experience. // As a compromise, we only use the BuildManager.GetCompiledType code path if we're in the non-updatable precomp case. // if (!Misc.IsNonUpdatablePrecompiledApp()) return VirtualPathProvider.FileExists(virtualPath); try { BuildManager.GetCompiledType(virtualPath); } catch { return false; } return true; #else if (_usingCustomVpp) { // for unit testing return VirtualPathProvider.FileExists(virtualPath); } else { // Use GetObjectFactory instead of GetCompiledType because it will not throw, which improves the debugging experience return BuildManager.GetObjectFactory(virtualPath, /* throwIfNotFound */ false) != null; } #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections; using System.Diagnostics; using System.Globalization; using System.Web.Compilation; using System.Web.Hosting; using System.Web.Resources; namespace System.Web.DynamicData { internal class TemplateFactory { // Use Hashtable instead of Dictionary<,> because it is more thread safe private Hashtable _fieldTemplateVirtualPathCache = new Hashtable(); private string _defaultLocation; private string _templateFolderVirtualPath; internal MetaModel Model { get; set; } private bool _needToResolveVirtualPath; private bool _trackFolderChanges; private bool _registeredForChangeNotifications; private VirtualPathProvider _vpp; private bool _usingCustomVpp; internal TemplateFactory(string defaultLocation) : this(defaultLocation, true) { } internal TemplateFactory(string defaultLocation, bool trackFolderChanges) { Debug.Assert(!String.IsNullOrEmpty(defaultLocation)); _defaultLocation = defaultLocation; _trackFolderChanges = trackFolderChanges; } internal string TemplateFolderVirtualPath { get { if (_templateFolderVirtualPath == null) { // If not set, set its default location TemplateFolderVirtualPath = _defaultLocation; } if (_needToResolveVirtualPath) { // Make sure it ends with a slash _templateFolderVirtualPath = VirtualPathUtility.AppendTrailingSlash(_templateFolderVirtualPath); // If it's relative, make it relative to the Model's path // Note can be null under Unit Testing if (Model != null) { _templateFolderVirtualPath = VirtualPathUtility.Combine(Model.DynamicDataFolderVirtualPath, _templateFolderVirtualPath); } _needToResolveVirtualPath = false; } return _templateFolderVirtualPath; } set { _templateFolderVirtualPath = value; // Make sure we register for change notifications, since we just got a new path _registeredForChangeNotifications = false; // It may be relative and need resolution, but let's not do it until we need it _needToResolveVirtualPath = true; } } internal VirtualPathProvider VirtualPathProvider { get { if (_vpp == null) { _vpp = HostingEnvironment.VirtualPathProvider; } return _vpp; } set { _vpp = value; _usingCustomVpp = value != null; } } internal string GetTemplatePath(long cacheKey, Func templatePathFactoryFunction) { // Check if we already have it cached string virtualPath = this[cacheKey]; // null is a valid value, so we also need to check whether the key exists if (virtualPath == null && !ContainsKey(cacheKey)) { // It's not cached, so compute it and cache it. Make sure multiple writers are serialized virtualPath = templatePathFactoryFunction(); this[cacheKey] = virtualPath; } return virtualPath; } private string this[long cacheKey] { get { EnsureRegisteredForChangeNotifications(); return (string)_fieldTemplateVirtualPathCache[cacheKey]; } set { EnsureRegisteredForChangeNotifications(); lock (_fieldTemplateVirtualPathCache) { _fieldTemplateVirtualPathCache[cacheKey] = value; } } } private bool ContainsKey(long cacheKey) { EnsureRegisteredForChangeNotifications(); return _fieldTemplateVirtualPathCache.ContainsKey(cacheKey); } private void EnsureRegisteredForChangeNotifications() { if (!_trackFolderChanges) { return; } if (!_registeredForChangeNotifications) { lock (this) { if (!_registeredForChangeNotifications) { // Make sure the folder exists if (!VirtualPathProvider.DirectoryExists(TemplateFolderVirtualPath)) { throw new InvalidOperationException(String.Format( CultureInfo.CurrentCulture, DynamicDataResources.FieldTemplateFactory_FolderNotFound, TemplateFolderVirtualPath)); } // Register for notifications if anything in that folder changes FileChangeNotifier.Register(TemplateFolderVirtualPath, delegate(string path) { // Something has changed, so clear our cache lock (_fieldTemplateVirtualPathCache) { _fieldTemplateVirtualPathCache.Clear(); } }); _registeredForChangeNotifications = true; } } } } internal bool FileExists(string virtualPath) { #if ORYX_VNEXT // Checking file existence is tricky, because in non-updatable precompiled apps, the ascx files are not // there at all. In that case, it's still possible to check existence by calling BuildManager.GetCompiledType // and seeing if it throws, but this can cause a lot of first chance exceptions, which affect the debugging experience. // As a compromise, we only use the BuildManager.GetCompiledType code path if we're in the non-updatable precomp case. // if (!Misc.IsNonUpdatablePrecompiledApp()) return VirtualPathProvider.FileExists(virtualPath); try { BuildManager.GetCompiledType(virtualPath); } catch { return false; } return true; #else if (_usingCustomVpp) { // for unit testing return VirtualPathProvider.FileExists(virtualPath); } else { // Use GetObjectFactory instead of GetCompiledType because it will not throw, which improves the debugging experience return BuildManager.GetObjectFactory(virtualPath, /* throwIfNotFound */ false) != null; } #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
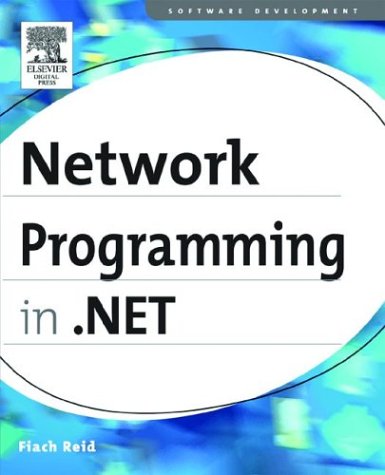
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlMethods.cs
- SoapFault.cs
- TdsParserSessionPool.cs
- SqlClientMetaDataCollectionNames.cs
- TraceData.cs
- SyndicationLink.cs
- FixedHyperLink.cs
- GroupLabel.cs
- AutomationFocusChangedEventArgs.cs
- SchemaTableColumn.cs
- HyperLinkField.cs
- BamlTreeUpdater.cs
- SrgsDocumentParser.cs
- BindingCompleteEventArgs.cs
- JournalEntryStack.cs
- Point3DKeyFrameCollection.cs
- HelpEvent.cs
- VariableDesigner.xaml.cs
- SqlBooleanMismatchVisitor.cs
- WebReferenceOptions.cs
- TableLayoutColumnStyleCollection.cs
- FaultContractAttribute.cs
- FileRecordSequenceHelper.cs
- BinaryMethodMessage.cs
- MatrixAnimationBase.cs
- QilName.cs
- ScrollViewerAutomationPeer.cs
- SettingsProperty.cs
- TreeIterator.cs
- XmlSerializerFactory.cs
- GeometryDrawing.cs
- UrlParameterWriter.cs
- MetafileHeaderWmf.cs
- SQLGuid.cs
- X509Extension.cs
- EncoderExceptionFallback.cs
- DatatypeImplementation.cs
- TextFormatterHost.cs
- BackgroundWorker.cs
- DynamicScriptObject.cs
- CustomMenuItemCollection.cs
- SubqueryRules.cs
- SqlNotificationEventArgs.cs
- HtmlTableCellCollection.cs
- ButtonBaseDesigner.cs
- RawTextInputReport.cs
- MessageOperationFormatter.cs
- WizardPanel.cs
- GeometryCombineModeValidation.cs
- LayoutEngine.cs
- TemplateXamlTreeBuilder.cs
- configsystem.cs
- HttpProfileGroupBase.cs
- ByteKeyFrameCollection.cs
- Calendar.cs
- HiddenFieldPageStatePersister.cs
- SecUtil.cs
- CatalogZoneBase.cs
- _HelperAsyncResults.cs
- XPathDescendantIterator.cs
- Expressions.cs
- Color.cs
- PriorityBinding.cs
- FixUp.cs
- PersonalizablePropertyEntry.cs
- SystemException.cs
- TCPClient.cs
- TextParagraphView.cs
- ThreadInterruptedException.cs
- BatchParser.cs
- TripleDES.cs
- SymbolPair.cs
- HandlerBase.cs
- SafeReversePInvokeHandle.cs
- HttpValueCollection.cs
- XmlSchemaAnyAttribute.cs
- Comparer.cs
- ScriptResourceHandler.cs
- DeflateEmulationStream.cs
- CanonicalFormWriter.cs
- SqlNotificationRequest.cs
- ProxyHwnd.cs
- RegistryDataKey.cs
- EntityDataSourceStatementEditorForm.cs
- Symbol.cs
- WebSysDescriptionAttribute.cs
- CommonRemoteMemoryBlock.cs
- _WebProxyDataBuilder.cs
- AsyncStreamReader.cs
- ReachSerializer.cs
- EFDataModelProvider.cs
- ResXDataNode.cs
- SharedStatics.cs
- DoubleMinMaxAggregationOperator.cs
- JournalEntryListConverter.cs
- RegistryKey.cs
- webbrowsersite.cs
- VSWCFServiceContractGenerator.cs
- StreamWithDictionary.cs
- CodeAccessPermission.cs