Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / MS / Internal / IO / Packaging / DeflateEmulationStream.cs / 1 / DeflateEmulationStream.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of a helper class that provides a fully functional Stream on a restricted functionality // Compression stream (System.IO.Compression.DeflateStream). // // History: // 10/05/2005: BruceMac: Split out from CompressEmulationStream //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; // for DeflateStream using System.Diagnostics; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging { //----------------------------------------------------- // // Internal Members // //----------------------------------------------------- ////// Emulates a fully functional stream using restricted functionality DeflateStream /// internal class DeflateEmulationTransform : IDeflateTransform { ////// Extract from DeflateStream to temp stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Decompress(Stream source, Stream sink) { // for non-empty stream create deflate stream that can // actually decompress using (DeflateStream deflateStream = new DeflateStream( source, // source of compressed data CompressionMode.Decompress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { int bytesRead = 0; do { bytesRead = deflateStream.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) sink.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } } ////// Compress from the temp stream into the base stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Compress(Stream source, Stream sink) { // create deflate stream that can actually compress or decompress using (DeflateStream deflateStream = new DeflateStream( sink, // destination for compressed data CompressionMode.Compress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { // persist to deflated stream from working stream int bytesRead = 0; do { bytesRead = source.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) deflateStream.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } // truncate if necessary and possible if (sink.CanSeek) sink.SetLength(sink.Position); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- private byte[] Buffer { get { if (_buffer == null) _buffer = new byte[0x1000]; // 4k return _buffer; } } //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private byte[] _buffer; // alloc and re-use to reduce memory fragmentation // this is safe because we are not thread-safe } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of a helper class that provides a fully functional Stream on a restricted functionality // Compression stream (System.IO.Compression.DeflateStream). // // History: // 10/05/2005: BruceMac: Split out from CompressEmulationStream //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; // for DeflateStream using System.Diagnostics; using System.IO.Packaging; using System.Windows; namespace MS.Internal.IO.Packaging { //----------------------------------------------------- // // Internal Members // //----------------------------------------------------- ////// Emulates a fully functional stream using restricted functionality DeflateStream /// internal class DeflateEmulationTransform : IDeflateTransform { ////// Extract from DeflateStream to temp stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Decompress(Stream source, Stream sink) { // for non-empty stream create deflate stream that can // actually decompress using (DeflateStream deflateStream = new DeflateStream( source, // source of compressed data CompressionMode.Decompress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { int bytesRead = 0; do { bytesRead = deflateStream.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) sink.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } } ////// Compress from the temp stream into the base stream /// ///Caller is responsible for correctly positioning source and sink stream pointers before calling. public void Compress(Stream source, Stream sink) { // create deflate stream that can actually compress or decompress using (DeflateStream deflateStream = new DeflateStream( sink, // destination for compressed data CompressionMode.Compress, // compress or decompress true)) // leave base stream open when the deflate stream is closed { // persist to deflated stream from working stream int bytesRead = 0; do { bytesRead = source.Read(Buffer, 0, Buffer.Length); if (bytesRead > 0) deflateStream.Write(Buffer, 0, bytesRead); } while (bytesRead > 0); } // truncate if necessary and possible if (sink.CanSeek) sink.SetLength(sink.Position); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- private byte[] Buffer { get { if (_buffer == null) _buffer = new byte[0x1000]; // 4k return _buffer; } } //------------------------------------------------------ // // Private Members // //------------------------------------------------------ private byte[] _buffer; // alloc and re-use to reduce memory fragmentation // this is safe because we are not thread-safe } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
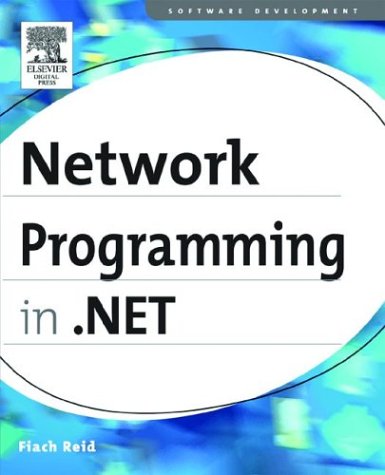
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IIS7UserPrincipal.cs
- ListControlBoundActionList.cs
- HotSpotCollection.cs
- TaskFormBase.cs
- DataFormats.cs
- TimeEnumHelper.cs
- XmlSerializationGeneratedCode.cs
- DiscoveryReferences.cs
- RegexCompilationInfo.cs
- bidPrivateBase.cs
- Int16Animation.cs
- StringArrayEditor.cs
- TextReader.cs
- ReadOnlyCollectionBase.cs
- X509Certificate2Collection.cs
- SolidColorBrush.cs
- NamespaceExpr.cs
- EntityTransaction.cs
- Events.cs
- ColumnWidthChangedEvent.cs
- WindowsFormsHostPropertyMap.cs
- SelectionProviderWrapper.cs
- ListViewItem.cs
- documentsequencetextcontainer.cs
- LabelDesigner.cs
- SqlBulkCopyColumnMapping.cs
- SqlAliaser.cs
- CodeIterationStatement.cs
- SafeArrayRankMismatchException.cs
- ExpandableObjectConverter.cs
- TextTreeTextNode.cs
- COM2Properties.cs
- ObjectQueryExecutionPlan.cs
- XamlGridLengthSerializer.cs
- Byte.cs
- DataGridHeaderBorder.cs
- QueryServiceConfigHandle.cs
- FlowDocumentFormatter.cs
- HttpPostProtocolImporter.cs
- DataGridViewCellCollection.cs
- LockedBorderGlyph.cs
- ListBoxDesigner.cs
- LockedBorderGlyph.cs
- DoubleIndependentAnimationStorage.cs
- QilPatternVisitor.cs
- TreeViewHitTestInfo.cs
- IisTraceWebEventProvider.cs
- TransactionBehavior.cs
- ImageListStreamer.cs
- OdbcConnectionOpen.cs
- TraceXPathNavigator.cs
- OpenTypeCommon.cs
- StoragePropertyMapping.cs
- Debug.cs
- ElementFactory.cs
- GridSplitterAutomationPeer.cs
- AuthenticatedStream.cs
- ListViewSelectEventArgs.cs
- BinarySerializer.cs
- MetadataArtifactLoaderCompositeFile.cs
- ArrayConverter.cs
- xmlglyphRunInfo.cs
- QilVisitor.cs
- DbConnectionFactory.cs
- NativeCompoundFileAPIs.cs
- FormsAuthenticationEventArgs.cs
- LinqDataSource.cs
- TextPenaltyModule.cs
- ImageConverter.cs
- TypedRowGenerator.cs
- RuleSettings.cs
- ChannelSinkStacks.cs
- Equal.cs
- ReflectionPermission.cs
- BitmapFrameDecode.cs
- NameValuePair.cs
- UniqueEventHelper.cs
- DataKeyArray.cs
- DateTimeFormatInfoScanner.cs
- StylusButton.cs
- CommandHelper.cs
- SqlServices.cs
- HostedTransportConfigurationManager.cs
- QilInvokeEarlyBound.cs
- EmptyImpersonationContext.cs
- ConstraintCollection.cs
- DataControlCommands.cs
- ExceptionUtil.cs
- ThreadStateException.cs
- XmlILConstructAnalyzer.cs
- SqlClientFactory.cs
- KeyValuePair.cs
- BitStream.cs
- AffineTransform3D.cs
- PolyLineSegment.cs
- WorkflowTraceTransfer.cs
- ClientTargetCollection.cs
- SQLCharsStorage.cs
- TreeViewHitTestInfo.cs
- HMACRIPEMD160.cs