Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Abstractions / HttpContextBase.cs / 1305376 / HttpContextBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Security.Principal; using System.Web.Caching; using System.Web.Profile; using System.Web.SessionState; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public abstract class HttpContextBase : IServiceProvider { [SuppressMessage("Microsoft.Performance", "CA1819:PropertiesShouldNotReturnArrays", Justification = "Matches HttpContext class")] public virtual Exception[] AllErrors { get { throw new NotImplementedException(); } } public virtual HttpApplicationStateBase Application { get { throw new NotImplementedException(); } } public virtual HttpApplication ApplicationInstance { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Cache Cache { get { throw new NotImplementedException(); } } public virtual IHttpHandler CurrentHandler { get { throw new NotImplementedException(); } } public virtual RequestNotification CurrentNotification { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Error", Justification = "Matches HttpContext class")] public virtual Exception Error { get { throw new NotImplementedException(); } } public virtual IHttpHandler Handler { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool IsCustomErrorEnabled { get { throw new NotImplementedException(); } } public virtual bool IsDebuggingEnabled { get { throw new NotImplementedException(); } } public virtual bool IsPostNotification { get { throw new NotImplementedException(); } } public virtual IDictionary Items { get { throw new NotImplementedException(); } } public virtual IHttpHandler PreviousHandler { get { throw new NotImplementedException(); } } public virtual ProfileBase Profile { get { throw new NotImplementedException(); } } public virtual HttpRequestBase Request { get { throw new NotImplementedException(); } } public virtual HttpResponseBase Response { get { throw new NotImplementedException(); } } public virtual HttpServerUtilityBase Server { get { throw new NotImplementedException(); } } public virtual HttpSessionStateBase Session { get { throw new NotImplementedException(); } } public virtual bool SkipAuthorization { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual DateTime Timestamp { get { throw new NotImplementedException(); } } public virtual TraceContext Trace { get { throw new NotImplementedException(); } } public virtual IPrincipal User { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void AddError(Exception errorInfo) { throw new NotImplementedException(); } public virtual void ClearError() { throw new NotImplementedException(); } public virtual object GetGlobalResourceObject(string classKey, string resourceKey) { throw new NotImplementedException(); } public virtual object GetGlobalResourceObject(string classKey, string resourceKey, CultureInfo culture) { throw new NotImplementedException(); } public virtual object GetLocalResourceObject(string virtualPath, string resourceKey) { throw new NotImplementedException(); } public virtual object GetLocalResourceObject(string virtualPath, string resourceKey, CultureInfo culture) { throw new NotImplementedException(); } public virtual object GetSection(string sectionName) { throw new NotImplementedException(); } public virtual void RemapHandler(IHttpHandler handler) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string path) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string path, bool rebaseClientPath) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string filePath, string pathInfo, string queryString) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string filePath, string pathInfo, string queryString, bool setClientFilePath) { throw new NotImplementedException(); } public virtual void SetSessionStateBehavior(SessionStateBehavior sessionStateBehavior) { throw new NotImplementedException(); } #region IServiceProvider Members [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual object GetService(Type serviceType) { throw new NotImplementedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Security.Principal; using System.Web.Caching; using System.Web.Profile; using System.Web.SessionState; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public abstract class HttpContextBase : IServiceProvider { [SuppressMessage("Microsoft.Performance", "CA1819:PropertiesShouldNotReturnArrays", Justification = "Matches HttpContext class")] public virtual Exception[] AllErrors { get { throw new NotImplementedException(); } } public virtual HttpApplicationStateBase Application { get { throw new NotImplementedException(); } } public virtual HttpApplication ApplicationInstance { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual Cache Cache { get { throw new NotImplementedException(); } } public virtual IHttpHandler CurrentHandler { get { throw new NotImplementedException(); } } public virtual RequestNotification CurrentNotification { get { throw new NotImplementedException(); } } [SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Error", Justification = "Matches HttpContext class")] public virtual Exception Error { get { throw new NotImplementedException(); } } public virtual IHttpHandler Handler { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual bool IsCustomErrorEnabled { get { throw new NotImplementedException(); } } public virtual bool IsDebuggingEnabled { get { throw new NotImplementedException(); } } public virtual bool IsPostNotification { get { throw new NotImplementedException(); } } public virtual IDictionary Items { get { throw new NotImplementedException(); } } public virtual IHttpHandler PreviousHandler { get { throw new NotImplementedException(); } } public virtual ProfileBase Profile { get { throw new NotImplementedException(); } } public virtual HttpRequestBase Request { get { throw new NotImplementedException(); } } public virtual HttpResponseBase Response { get { throw new NotImplementedException(); } } public virtual HttpServerUtilityBase Server { get { throw new NotImplementedException(); } } public virtual HttpSessionStateBase Session { get { throw new NotImplementedException(); } } public virtual bool SkipAuthorization { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual DateTime Timestamp { get { throw new NotImplementedException(); } } public virtual TraceContext Trace { get { throw new NotImplementedException(); } } public virtual IPrincipal User { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void AddError(Exception errorInfo) { throw new NotImplementedException(); } public virtual void ClearError() { throw new NotImplementedException(); } public virtual object GetGlobalResourceObject(string classKey, string resourceKey) { throw new NotImplementedException(); } public virtual object GetGlobalResourceObject(string classKey, string resourceKey, CultureInfo culture) { throw new NotImplementedException(); } public virtual object GetLocalResourceObject(string virtualPath, string resourceKey) { throw new NotImplementedException(); } public virtual object GetLocalResourceObject(string virtualPath, string resourceKey, CultureInfo culture) { throw new NotImplementedException(); } public virtual object GetSection(string sectionName) { throw new NotImplementedException(); } public virtual void RemapHandler(IHttpHandler handler) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string path) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string path, bool rebaseClientPath) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string filePath, string pathInfo, string queryString) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters", Justification = "Matches HttpContext class")] public virtual void RewritePath(string filePath, string pathInfo, string queryString, bool setClientFilePath) { throw new NotImplementedException(); } public virtual void SetSessionStateBehavior(SessionStateBehavior sessionStateBehavior) { throw new NotImplementedException(); } #region IServiceProvider Members [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public virtual object GetService(Type serviceType) { throw new NotImplementedException(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
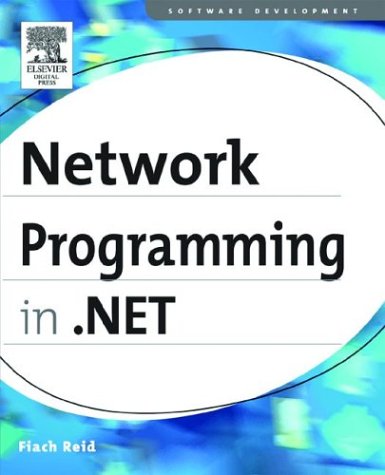
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextReturnReader.cs
- NativeActivityMetadata.cs
- CodeSnippetCompileUnit.cs
- ZoneIdentityPermission.cs
- ReadOnlyObservableCollection.cs
- ConfigurationException.cs
- StaticExtensionConverter.cs
- manifestimages.cs
- ToolStripControlHost.cs
- ObjectAssociationEndMapping.cs
- ToolStripDropDownItem.cs
- SafeNativeMethodsCLR.cs
- SecurityRuntime.cs
- CompilerScope.Storage.cs
- ISCIIEncoding.cs
- SoapExtensionReflector.cs
- CatalogPart.cs
- _NTAuthentication.cs
- TextShapeableCharacters.cs
- IdentifierElement.cs
- FontUnit.cs
- EntityCommandDefinition.cs
- EditorPartCollection.cs
- DbDataRecord.cs
- ResolveMatchesMessage11.cs
- HtmlContainerControl.cs
- ReadWriteObjectLock.cs
- Converter.cs
- TlsnegoTokenProvider.cs
- Calendar.cs
- FileUtil.cs
- MetadataItemSerializer.cs
- XsdBuildProvider.cs
- MimeMultiPart.cs
- TableRow.cs
- XmlSchemaSubstitutionGroup.cs
- DataRecordObjectView.cs
- WebPartsPersonalizationAuthorization.cs
- StateItem.cs
- SQLRoleProvider.cs
- TextSearch.cs
- DataBoundControlHelper.cs
- EntityContainerEmitter.cs
- VerificationAttribute.cs
- WebRequestModuleElementCollection.cs
- PrtCap_Base.cs
- FormCollection.cs
- FileLevelControlBuilderAttribute.cs
- MenuTracker.cs
- EventPropertyMap.cs
- DuplicateWaitObjectException.cs
- DomainUpDown.cs
- CompiledELinqQueryState.cs
- DurableInstanceProvider.cs
- UnescapedXmlDiagnosticData.cs
- TargetInvocationException.cs
- DataKeyCollection.cs
- ChannelBinding.cs
- ToolStripManager.cs
- ToolStripLabel.cs
- ElementMarkupObject.cs
- TabControlToolboxItem.cs
- Canvas.cs
- GridItemProviderWrapper.cs
- InheritanceContextHelper.cs
- BinaryObjectReader.cs
- TransactionScope.cs
- AbsoluteQuery.cs
- ProjectionPruner.cs
- ProxySimple.cs
- ObjectRef.cs
- ConfigurationSectionGroup.cs
- IntegerFacetDescriptionElement.cs
- ResXResourceWriter.cs
- ProtocolViolationException.cs
- _SpnDictionary.cs
- Subtree.cs
- ObservableCollection.cs
- FormViewDeletedEventArgs.cs
- _ContextAwareResult.cs
- EntityModelBuildProvider.cs
- LogLogRecordHeader.cs
- OracleBinary.cs
- CustomAttribute.cs
- LineUtil.cs
- ConfigurationLocation.cs
- TextureBrush.cs
- COM2PropertyDescriptor.cs
- TransformationRules.cs
- Animatable.cs
- ColumnClickEvent.cs
- CatalogZone.cs
- LocalServiceSecuritySettings.cs
- GlyphShapingProperties.cs
- DragStartedEventArgs.cs
- XmlNotation.cs
- SHA1Cng.cs
- FileLevelControlBuilderAttribute.cs
- MetadataArtifactLoaderFile.cs
- WebException.cs