Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / IO / Zip / ZipFileInfo.cs / 1305600 / ZipFileInfo.cs
//------------------------------------------------------------------------------ //------------- *** WARNING *** //------------- This file is part of a legally monitored development project. //------------- Do not check in changes to this project. Do not raid bugs on this //------------- code in the main PS database. Do not contact the owner of this //------------- code directly. Contact the legal team at ‘ZSLegal’ for assistance. //------------- *** WARNING *** //----------------------------------------------------------------------------- //----------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is an internal class that enables interactions with Zip archives // for OPC scenarios // // History: // 11/19/2004: IgorBel: Initial creation. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; namespace MS.Internal.IO.Zip { internal sealed class ZipFileInfo { //------------------------------------------------------ // // Public Members // //----------------------------------------------------- // None //------------------------------------------------------ // // Internal Constructors // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal API Methods (although these methods are marked as // Internal they are part of the internal ZIP IO API surface // //------------------------------------------------------ internal Stream GetStream(FileMode mode, FileAccess access) { CheckDisposed(); return _fileBlock.GetStream(mode, access); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal string Name { get { CheckDisposed(); return _fileBlock.FileName; } } internal ZipArchive ZipArchive { get { CheckDisposed(); return _zipArchive; } } internal CompressionMethodEnum CompressionMethod { get { CheckDisposed(); return _fileBlock.CompressionMethod; } } internal DateTime LastModFileDateTime { get { CheckDisposed(); return ZipIOBlockManager.FromMsDosDateTime(_fileBlock.LastModFileDateTime); } } #if false internal bool EncryptedFlag { get { CheckDisposed(); return _fileBlock.EncryptedFlag; } } #endif internal DeflateOptionEnum DeflateOption { get { CheckDisposed(); return _fileBlock.DeflateOption; } } #if false internal bool StreamingCreationFlag { get { CheckDisposed(); return _fileBlock.StreamingCreationFlag; } } #endif // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool FolderFlag { get { CheckDisposed(); return _fileBlock.FolderFlag; } } // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool VolumeLabelFlag { get { CheckDisposed(); return _fileBlock.VolumeLabelFlag; } } //----------------------------------------------------- // Internal NON API Constructor (this constructor is marked as internal // and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipFileInfo(ZipArchive zipArchive, ZipIOLocalFileBlock fileBlock) { Debug.Assert((fileBlock != null) && (zipArchive != null)); _fileBlock = fileBlock; _zipArchive = zipArchive; #if DEBUG // validate that date time is legal DateTime dt = LastModFileDateTime; #endif } //----------------------------------------------------- // Internal NON API property to be used to map FileInfo back to a block that needs to be deleted // (this prperty is marked as internal and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipIOLocalFileBlock LocalFileBlock { get { return _fileBlock; } } //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { _fileBlock.CheckDisposed(); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private ZipIOLocalFileBlock _fileBlock; private ZipArchive _zipArchive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //------------- *** WARNING *** //------------- This file is part of a legally monitored development project. //------------- Do not check in changes to this project. Do not raid bugs on this //------------- code in the main PS database. Do not contact the owner of this //------------- code directly. Contact the legal team at ‘ZSLegal’ for assistance. //------------- *** WARNING *** //----------------------------------------------------------------------------- //----------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is an internal class that enables interactions with Zip archives // for OPC scenarios // // History: // 11/19/2004: IgorBel: Initial creation. // //----------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; namespace MS.Internal.IO.Zip { internal sealed class ZipFileInfo { //------------------------------------------------------ // // Public Members // //----------------------------------------------------- // None //------------------------------------------------------ // // Internal Constructors // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal API Methods (although these methods are marked as // Internal they are part of the internal ZIP IO API surface // //------------------------------------------------------ internal Stream GetStream(FileMode mode, FileAccess access) { CheckDisposed(); return _fileBlock.GetStream(mode, access); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal string Name { get { CheckDisposed(); return _fileBlock.FileName; } } internal ZipArchive ZipArchive { get { CheckDisposed(); return _zipArchive; } } internal CompressionMethodEnum CompressionMethod { get { CheckDisposed(); return _fileBlock.CompressionMethod; } } internal DateTime LastModFileDateTime { get { CheckDisposed(); return ZipIOBlockManager.FromMsDosDateTime(_fileBlock.LastModFileDateTime); } } #if false internal bool EncryptedFlag { get { CheckDisposed(); return _fileBlock.EncryptedFlag; } } #endif internal DeflateOptionEnum DeflateOption { get { CheckDisposed(); return _fileBlock.DeflateOption; } } #if false internal bool StreamingCreationFlag { get { CheckDisposed(); return _fileBlock.StreamingCreationFlag; } } #endif // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool FolderFlag { get { CheckDisposed(); return _fileBlock.FolderFlag; } } // This ia Directory flag based on the informtion from the central directory // at the moment we have only provide reliable value for the files authored in MS-DOS // The upper byte of version made by indicating (OS) must be == 0 (MS-DOS) // for the other cases (OSes) we will return false internal bool VolumeLabelFlag { get { CheckDisposed(); return _fileBlock.VolumeLabelFlag; } } //----------------------------------------------------- // Internal NON API Constructor (this constructor is marked as internal // and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipFileInfo(ZipArchive zipArchive, ZipIOLocalFileBlock fileBlock) { Debug.Assert((fileBlock != null) && (zipArchive != null)); _fileBlock = fileBlock; _zipArchive = zipArchive; #if DEBUG // validate that date time is legal DateTime dt = LastModFileDateTime; #endif } //----------------------------------------------------- // Internal NON API property to be used to map FileInfo back to a block that needs to be deleted // (this prperty is marked as internal and isNOT part of the ZIP IO API surface) // It supposed to be called only by the ZipArchive class //------------------------------------------------------ internal ZipIOLocalFileBlock LocalFileBlock { get { return _fileBlock; } } //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- private void CheckDisposed() { _fileBlock.CheckDisposed(); } //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private ZipIOLocalFileBlock _fileBlock; private ZipArchive _zipArchive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
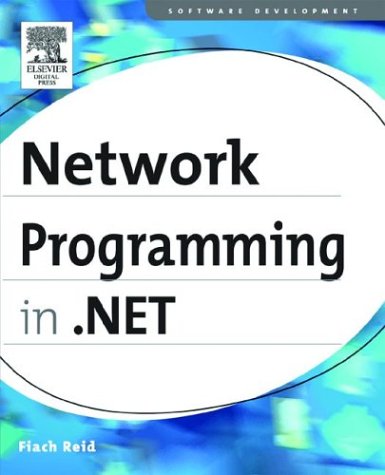
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataItemSerializer.cs
- SqlCacheDependencySection.cs
- DataBoundLiteralControl.cs
- WindowsRebar.cs
- SvcMapFileLoader.cs
- Parameter.cs
- AuthStoreRoleProvider.cs
- WS2007HttpBindingElement.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- DivideByZeroException.cs
- XamlSerializer.cs
- mongolianshape.cs
- WebException.cs
- TextContainer.cs
- ServiceHostFactory.cs
- SiteOfOriginPart.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- LineMetrics.cs
- DataGridViewCellStateChangedEventArgs.cs
- VirtualizedItemProviderWrapper.cs
- DataSourceHelper.cs
- CorruptingExceptionCommon.cs
- DocumentSequenceHighlightLayer.cs
- TraceLevelStore.cs
- FaultContractInfo.cs
- RouteData.cs
- Version.cs
- JoinTreeNode.cs
- LineBreak.cs
- DataSpaceManager.cs
- XmlUrlResolver.cs
- UnitControl.cs
- XmlC14NWriter.cs
- LocatorBase.cs
- XomlCompilerError.cs
- CurrencyWrapper.cs
- ObjectDataSourceWizardForm.cs
- EntityDataSourceValidationException.cs
- CriticalExceptions.cs
- SessionIDManager.cs
- DetailsViewDeletedEventArgs.cs
- XmlExtensionFunction.cs
- EntityClassGenerator.cs
- MimeMultiPart.cs
- WebServiceTypeData.cs
- ExpressionWriter.cs
- AutomationPattern.cs
- DataView.cs
- DataColumnPropertyDescriptor.cs
- MenuItemStyle.cs
- QilNode.cs
- PreviewPageInfo.cs
- DbDataAdapter.cs
- HtmlFormParameterReader.cs
- Single.cs
- SqlPersonalizationProvider.cs
- SafeNativeMethods.cs
- StorageInfo.cs
- AffineTransform3D.cs
- BamlBinaryWriter.cs
- Int16.cs
- Helpers.cs
- AncillaryOps.cs
- DocumentPageViewAutomationPeer.cs
- IntPtr.cs
- OutputScopeManager.cs
- CollaborationHelperFunctions.cs
- IBuiltInEvidence.cs
- SmtpNtlmAuthenticationModule.cs
- SafeCryptContextHandle.cs
- StreamResourceInfo.cs
- ChangeDirector.cs
- XPathLexer.cs
- UrlPropertyAttribute.cs
- SpecialFolderEnumConverter.cs
- ResourceReferenceExpressionConverter.cs
- MaskDesignerDialog.cs
- ColorConvertedBitmap.cs
- SamlAction.cs
- PropertyRef.cs
- HopperCache.cs
- StringArrayConverter.cs
- RowParagraph.cs
- Brush.cs
- SqlUtils.cs
- MemberExpressionHelper.cs
- HtmlTitle.cs
- WebResourceUtil.cs
- CodeAccessSecurityEngine.cs
- SQLStringStorage.cs
- EnumerableRowCollection.cs
- XmlSecureResolver.cs
- Typography.cs
- Pair.cs
- EventItfInfo.cs
- EntityDesignerDataSourceView.cs
- JoinSymbol.cs
- StorageEntityContainerMapping.cs
- TextCharacters.cs
- SchemaType.cs