Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / ResourceReferenceExpressionConverter.cs / 1 / ResourceReferenceExpressionConverter.cs
//---------------------------------------------------------------------------- // // File: ResourceReferenceExpressionConverter.cs // // Description: // TypeConverter for a resource value expression // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Windows; namespace System.Windows.Markup { ////// TypeConverter for a resource value expression /// public class ResourceReferenceExpressionConverter : ExpressionConverter { ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert from /// ////// true if conversion is possible /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return base.CanConvertFrom(context, sourceType); } ////// TypeConverter method override. /// ////// For avalon serialization this converter /// returns true for string types only if the /// target element with the resource /// dictionary for the current resource /// reference is also being serialized. Else /// it returns false and the serialization /// engine must serialize the evaluated /// value of the expression. /// /// /// ITypeDescriptorContext /// /// /// Type to convert to /// ////// true if conversion is possible /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // Validate Input Arguments if (destinationType == null) { throw new ArgumentNullException("destinationType"); } //MarkupExtention if (destinationType == typeof(MarkupExtension)) return true; return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// ////// value that is result of conversion /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { return base.ConvertFrom(context, culture, value); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// /// /// Type to convert to /// ////// converted value /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { // Validate Input Arguments ResourceReferenceExpression expr = value as ResourceReferenceExpression; if (expr == null) { throw new ArgumentException(SR.Get(SRID.MustBeOfType, "value", "ResourceReferenceExpression")); } if (destinationType == null) { throw new ArgumentNullException("destinationType"); } // MarkupExtension if (destinationType == typeof(MarkupExtension)) { return new DynamicResourceExtension(expr.ResourceKey); } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ResourceReferenceExpressionConverter.cs // // Description: // TypeConverter for a resource value expression // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Windows; namespace System.Windows.Markup { ////// TypeConverter for a resource value expression /// public class ResourceReferenceExpressionConverter : ExpressionConverter { ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert from /// ////// true if conversion is possible /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return base.CanConvertFrom(context, sourceType); } ////// TypeConverter method override. /// ////// For avalon serialization this converter /// returns true for string types only if the /// target element with the resource /// dictionary for the current resource /// reference is also being serialized. Else /// it returns false and the serialization /// engine must serialize the evaluated /// value of the expression. /// /// /// ITypeDescriptorContext /// /// /// Type to convert to /// ////// true if conversion is possible /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // Validate Input Arguments if (destinationType == null) { throw new ArgumentNullException("destinationType"); } //MarkupExtention if (destinationType == typeof(MarkupExtension)) return true; return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// ////// value that is result of conversion /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { return base.ConvertFrom(context, culture, value); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// /// /// Type to convert to /// ////// converted value /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { // Validate Input Arguments ResourceReferenceExpression expr = value as ResourceReferenceExpression; if (expr == null) { throw new ArgumentException(SR.Get(SRID.MustBeOfType, "value", "ResourceReferenceExpression")); } if (destinationType == null) { throw new ArgumentNullException("destinationType"); } // MarkupExtension if (destinationType == typeof(MarkupExtension)) { return new DynamicResourceExtension(expr.ResourceKey); } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
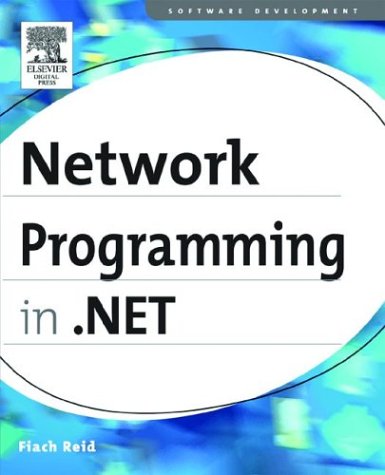
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaSimpleTypeList.cs
- CFGGrammar.cs
- ToolStripComboBox.cs
- Icon.cs
- FtpWebRequest.cs
- RSAPKCS1SignatureFormatter.cs
- Keywords.cs
- HandledMouseEvent.cs
- EntityModelSchemaGenerator.cs
- TableAdapterManagerMethodGenerator.cs
- ControlIdConverter.cs
- ReadOnlyNameValueCollection.cs
- RuntimeIdentifierPropertyAttribute.cs
- SymmetricAlgorithm.cs
- HtmlSelect.cs
- HealthMonitoringSection.cs
- Int64Storage.cs
- HandleRef.cs
- SchemaImporter.cs
- HMAC.cs
- DuplexChannel.cs
- WebPartDeleteVerb.cs
- localization.cs
- DiagnosticsConfiguration.cs
- BamlLocalizableResourceKey.cs
- AuthenticationException.cs
- ContextDataSourceView.cs
- ListViewGroupConverter.cs
- SmtpCommands.cs
- TemplateGroupCollection.cs
- sqlmetadatafactory.cs
- TransformConverter.cs
- PeerNeighborManager.cs
- CodeAccessPermission.cs
- EventItfInfo.cs
- ScriptingScriptResourceHandlerSection.cs
- DiscoveryMessageSequence11.cs
- DataGridViewColumnCollectionDialog.cs
- RayHitTestParameters.cs
- LambdaReference.cs
- ServiceBehaviorElement.cs
- InvokePattern.cs
- FixedSOMContainer.cs
- BufferedOutputStream.cs
- precedingsibling.cs
- DataServiceRequestException.cs
- ValueTypeFixupInfo.cs
- TextFormatter.cs
- DownloadProgressEventArgs.cs
- OdbcCommandBuilder.cs
- SelectorItemAutomationPeer.cs
- ErrorTableItemStyle.cs
- ConfigurationLocation.cs
- CacheSection.cs
- NegatedConstant.cs
- XmlQualifiedName.cs
- Parameter.cs
- SkewTransform.cs
- XmlFormatMapping.cs
- FileClassifier.cs
- ZoneIdentityPermission.cs
- PlainXmlWriter.cs
- BamlReader.cs
- LightweightEntityWrapper.cs
- TextRangeAdaptor.cs
- ObjectAnimationBase.cs
- FactoryRecord.cs
- CompilerWrapper.cs
- UIHelper.cs
- ServiceInstanceProvider.cs
- BooleanSwitch.cs
- RadioButton.cs
- BufferedGraphics.cs
- GridViewCancelEditEventArgs.cs
- TextEditorDragDrop.cs
- BehaviorEditorPart.cs
- FacetValueContainer.cs
- AutomationEvent.cs
- SmtpClient.cs
- ManagementDateTime.cs
- InkCanvasSelectionAdorner.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- TraceSection.cs
- UseManagedPresentationBindingElementImporter.cs
- DataSourceControl.cs
- LicenseManager.cs
- FileDialog_Vista_Interop.cs
- ErrorStyle.cs
- Transform3D.cs
- SqlBulkCopyColumnMappingCollection.cs
- CompilerScopeManager.cs
- TextRange.cs
- PropertyInfoSet.cs
- TextEditorTyping.cs
- SevenBitStream.cs
- _ContextAwareResult.cs
- Rotation3D.cs
- ACL.cs
- HandleRef.cs
- LinqDataSourceView.cs