Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activities / Activation / WorkflowServiceHostFactory.cs / 1305376 / WorkflowServiceHostFactory.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activities.Activation { using System.Activities; using System.Activities.XamlIntegration; using System.IO; using System.Globalization; using System.Reflection; using System.Runtime; using System.Runtime.DurableInstancing; using System.Security; using System.Security.Permissions; using System.ServiceModel.Activation; using System.Web; using System.Web.Compilation; using System.Web.Hosting; using System.Xaml; using System.Xml; using System.Xml.Linq; public class WorkflowServiceHostFactory : ServiceHostFactoryBase { public override ServiceHostBase CreateServiceHost(string constructorString, Uri[] baseAddresses) { WorkflowServiceHost serviceHost = null; if (string.IsNullOrEmpty(constructorString)) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.WorkflowServiceHostFactoryConstructorStringNotProvided)); } if (baseAddresses == null) { throw FxTrace.Exception.ArgumentNull("baseAddresses"); } if (baseAddresses.Length == 0) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.BaseAddressesNotProvided)); } if (!HostingEnvironment.IsHosted) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.Hosting_ProcessNotExecutingUnderHostedContext("WorkflowServiceHostFactory.CreateServiceHost"))); } // We expect most users will use .xamlx file instead of precompiled assembly // Samples of xamlVirtualPath under all scenarios // constructorString XamlFileBaseLocation xamlVirtualPath // 1. Xamlx direct ~/sub/a.xamlx ~/sub/ ~/sub/a.xamlx // 2. CBA with precompiled servicetypeinfo ~/sub/servicetypeinfo ~/sub/servicetypeinfo * no file will be found // 3. CBA with xamlx sub/a.xamlx ~/ ~/sub/a.xamlx // 4. Svc with precompiled servicetypeinfo ~/sub/servicetypeinfo ~/sub/servicetypeinfo * no file will be found // 5. Svc with Xamlx ../a.xamlx ~/sub/ ~/a.xamlx string xamlVirtualPath = VirtualPathUtility.Combine(AspNetEnvironment.Current.XamlFileBaseLocation, constructorString); Stream activityStream; string compiledCustomString; if (GetServiceFileStreamOrCompiledCustomString(xamlVirtualPath, baseAddresses, out activityStream, out compiledCustomString)) { object serviceObject; using (activityStream) { // BuildManager.GetReferencedAssemblies(); //XmlnsMappingHelper.EnsureMappingPassed(); XamlXmlReaderSettings xamlXmlReaderSettings = new XamlXmlReaderSettings(); xamlXmlReaderSettings.ProvideLineInfo = true; XamlReader wrappedReader = ActivityXamlServices.CreateReader(new XamlXmlReader(XmlReader.Create(activityStream), xamlXmlReaderSettings)); if (TD.XamlServicesLoadStartIsEnabled()) { TD.XamlServicesLoadStart(); } serviceObject = XamlServices.Load(wrappedReader); if (TD.XamlServicesLoadStopIsEnabled()) { TD.XamlServicesLoadStop(); } } WorkflowService service = null; if (serviceObject is Activity) { service = new WorkflowService { Body = (Activity)serviceObject }; } else if (serviceObject is WorkflowService) { service = (WorkflowService)serviceObject; } // If name of the service is not set // service name = xaml file name with extension // service namespace = IIS virtual path // service config name = Activity.DisplayName if (service != null) { if (service.Name == null) { string serviceName = VirtualPathUtility.GetFileName(xamlVirtualPath); string serviceNamespace = String.Format(CultureInfo.InvariantCulture, "/{0}{1}", ServiceHostingEnvironment.SiteName, VirtualPathUtility.GetDirectory(ServiceHostingEnvironment.FullVirtualPath)); service.Name = XName.Get(XmlConvert.EncodeLocalName(serviceName), serviceNamespace); if (service.ConfigurationName == null && service.Body != null) { service.ConfigurationName = XmlConvert.EncodeLocalName(service.Body.DisplayName); } } serviceHost = CreateWorkflowServiceHost(service, baseAddresses); } } else { Type activityType = this.GetTypeFromAssembliesInCurrentDomain(constructorString); if (null == activityType) { activityType = GetTypeFromCompileCustomString(compiledCustomString, constructorString); } if (null == activityType) { //for file-less cases, try in referenced assemblies as CompileCustomString assemblies are empty. BuildManager.GetReferencedAssemblies(); activityType = this.GetTypeFromAssembliesInCurrentDomain(constructorString); } if (null != activityType) { if (!TypeHelper.AreTypesCompatible(activityType, typeof(Activity))) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.TypeNotActivity(activityType.FullName))); } Activity activity = (Activity)Activator.CreateInstance(activityType); serviceHost = CreateWorkflowServiceHost(activity, baseAddresses); } } if (serviceHost == null) { throw FxTrace.Exception.AsError( new InvalidOperationException(SR.CannotResolveConstructorStringToWorkflowType(constructorString))); } //The Description.Name and Description.NameSpace aren't included intentionally - because //in farm scenarios the sole and unique identifier is the service deployment URL ((IDurableInstancingOptions)serviceHost.DurableInstancingOptions).SetScopeName( XName.Get(XmlConvert.EncodeLocalName(VirtualPathUtility.GetFileName(ServiceHostingEnvironment.FullVirtualPath)), String.Format(CultureInfo.InvariantCulture, "/{0}{1}", ServiceHostingEnvironment.SiteName, VirtualPathUtility.GetDirectory(ServiceHostingEnvironment.FullVirtualPath)))); return serviceHost; } Type GetTypeFromAssembliesInCurrentDomain(string typeString) { Type activityType = Type.GetType(typeString, false); if (null == activityType) { foreach (Assembly assembly in AppDomain.CurrentDomain.GetAssemblies()) { activityType = assembly.GetType(typeString, false); if (null != activityType) { break; } } } return activityType; } Type GetTypeFromCompileCustomString(string compileCustomString, string typeString) { if (string.IsNullOrEmpty(compileCustomString)) { return null; } string[] components = compileCustomString.Split('|'); if (components.Length < 3) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.InvalidCompiledString(compileCustomString))); } Type activityType = null; for (int i = 3; i < components.Length; i++) { Assembly assembly = Assembly.Load(components[i]); activityType = assembly.GetType(typeString, false); if (activityType != null) { break; } } return activityType; } protected virtual WorkflowServiceHost CreateWorkflowServiceHost(Activity activity, Uri[] baseAddresses) { return new WorkflowServiceHost(activity, baseAddresses); } protected virtual WorkflowServiceHost CreateWorkflowServiceHost(WorkflowService service, Uri[] baseAddresses) { return new WorkflowServiceHost(service, baseAddresses); } // The code is optimized for reducing impersonation // true means serviceFileStream has been set; false means CompiledCustomString has been set [Fx.Tag.SecurityNote(Critical = "Calls SecurityCritical method HostingEnvironmentWrapper.UnsafeImpersonate().", Safe = "Demands unmanaged code permission, disposes impersonation context in a finally, and tightly scopes the usage of the umpersonation token.")] [SecurityPermission(SecurityAction.Demand, UnmanagedCode = true)] [SecuritySafeCritical] bool GetServiceFileStreamOrCompiledCustomString(string virtualPath, Uri[] baseAddresses, out Stream serviceFileStream, out string compiledCustomString) { IDisposable unsafeImpersonate = null; compiledCustomString = null; serviceFileStream = null; try { try { try { } finally { unsafeImpersonate = HostingEnvironmentWrapper.UnsafeImpersonate(); } if (HostingEnvironment.VirtualPathProvider.FileExists(virtualPath)) { serviceFileStream = HostingEnvironment.VirtualPathProvider.GetFile(virtualPath).Open(); return true; } else { if (!AspNetEnvironment.Current.IsConfigurationBased) { compiledCustomString = BuildManager.GetCompiledCustomString(baseAddresses[0].AbsolutePath); } return false; } } finally { if (null != unsafeImpersonate) { unsafeImpersonate.Dispose(); } } } catch { throw; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
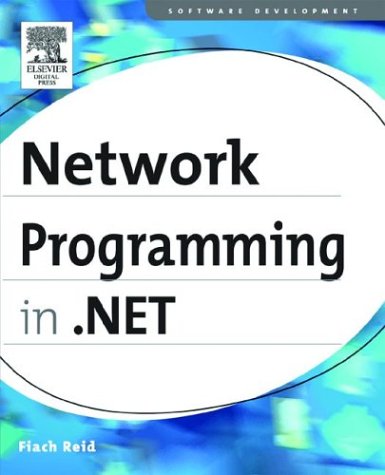
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssemblyGen.cs
- TextBoxView.cs
- PageBuildProvider.cs
- UriWriter.cs
- CompositeCollection.cs
- LocalizabilityAttribute.cs
- References.cs
- MulticastDelegate.cs
- HtmlLabelAdapter.cs
- RenameRuleObjectDialog.cs
- EditBehavior.cs
- HTTPNotFoundHandler.cs
- GlobalEventManager.cs
- VirtualPathUtility.cs
- util.cs
- ChameleonKey.cs
- BooleanStorage.cs
- AppDomainShutdownMonitor.cs
- JoinTreeNode.cs
- ComponentTray.cs
- SQLBinaryStorage.cs
- CallbackException.cs
- DocumentPageHost.cs
- NotifyIcon.cs
- InternalBufferManager.cs
- SHA1Cng.cs
- RtfControls.cs
- XmlNamedNodeMap.cs
- TextEndOfLine.cs
- ToolStrip.cs
- WCFServiceClientProxyGenerator.cs
- CryptoProvider.cs
- basecomparevalidator.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- GeneralTransform3DCollection.cs
- WebPartPersonalization.cs
- SharedPerformanceCounter.cs
- AsymmetricKeyExchangeFormatter.cs
- EventLogTraceListener.cs
- SystemColors.cs
- TdsParserSessionPool.cs
- ApplicationContext.cs
- TypeNameConverter.cs
- DocobjHost.cs
- MethodImplAttribute.cs
- NetworkStream.cs
- SByteStorage.cs
- ExpressionBuilder.cs
- ConversionContext.cs
- ReliableSessionBindingElement.cs
- GroupItem.cs
- BamlVersionHeader.cs
- MD5HashHelper.cs
- ListenerElementsCollection.cs
- ProfileGroupSettings.cs
- DataBindingExpressionBuilder.cs
- JsonWriterDelegator.cs
- MergeFilterQuery.cs
- AssemblyBuilder.cs
- HeaderPanel.cs
- ServiceRouteHandler.cs
- XmlQuerySequence.cs
- XMLSchema.cs
- SchemaEntity.cs
- NumberFormatInfo.cs
- PngBitmapEncoder.cs
- DeadCharTextComposition.cs
- PaginationProgressEventArgs.cs
- IUnknownConstantAttribute.cs
- HttpContext.cs
- SerialReceived.cs
- FontFamily.cs
- XmlIncludeAttribute.cs
- ConfigurationErrorsException.cs
- WindowsGrip.cs
- Dictionary.cs
- FixUpCollection.cs
- FunctionCommandText.cs
- LineMetrics.cs
- StyleModeStack.cs
- SSmlParser.cs
- CodeBlockBuilder.cs
- InfoCardKeyedHashAlgorithm.cs
- ModifierKeysValueSerializer.cs
- MulticastDelegate.cs
- Accessible.cs
- DesignTimeHTMLTextWriter.cs
- LambdaCompiler.cs
- QilIterator.cs
- SrgsSubset.cs
- WebPartConnectVerb.cs
- RootDesignerSerializerAttribute.cs
- COM2ComponentEditor.cs
- FloaterBaseParagraph.cs
- RepeaterItem.cs
- EnumMemberAttribute.cs
- PermissionRequestEvidence.cs
- diagnosticsswitches.cs
- ObjectConverter.cs
- DataContractAttribute.cs