Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / TypeSystem / EventInfo.cs / 1305376 / EventInfo.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.Reflection; #region DesignTimeEventInfo internal sealed class DesignTimeEventInfo: EventInfo { #region Members and Constructors private string name; private DesignTimeMethodInfo addMethod = null; private DesignTimeMethodInfo removeMethod = null; private Attribute[] attributes = null; private MemberAttributes memberAttributes; private DesignTimeType declaringType; private CodeMemberEvent codeDomEvent; internal DesignTimeEventInfo(DesignTimeType declaringType, CodeMemberEvent codeDomEvent) { if (declaringType == null) { throw new ArgumentNullException("Declaring Type"); } if (codeDomEvent == null) { throw new ArgumentNullException("codeDomEvent"); } this.declaringType = declaringType; this.codeDomEvent = codeDomEvent; this.name = Helper.EnsureTypeName(codeDomEvent.Name); this.memberAttributes = codeDomEvent.Attributes; this.addMethod = null; this.removeMethod = null; } #endregion #region Event Info overrides public override MethodInfo GetAddMethod(bool nonPublic) { if (this.addMethod == null) { Type handlerType = declaringType.ResolveType(DesignTimeType.GetTypeNameFromCodeTypeReference(this.codeDomEvent.Type, declaringType)); if (handlerType != null) { CodeMemberMethod codeAddMethod = new CodeMemberMethod(); codeAddMethod.Name = "add_" + this.name; codeAddMethod.ReturnType = new CodeTypeReference(typeof(void)); codeAddMethod.Parameters.Add(new CodeParameterDeclarationExpression(this.codeDomEvent.Type, "Handler")); codeAddMethod.Attributes = this.memberAttributes; this.addMethod = new DesignTimeMethodInfo(this.declaringType, codeAddMethod, true); } } return this.addMethod; } public override MethodInfo GetRemoveMethod(bool nonPublic) { if (this.removeMethod == null) { Type handlerType = declaringType.ResolveType(DesignTimeType.GetTypeNameFromCodeTypeReference(this.codeDomEvent.Type, declaringType)); if (handlerType != null) { CodeMemberMethod codeRemoveMethod = new CodeMemberMethod(); codeRemoveMethod.Name = "remove_" + this.name; codeRemoveMethod.ReturnType = new CodeTypeReference(typeof(void)); codeRemoveMethod.Parameters.Add(new CodeParameterDeclarationExpression(handlerType, "Handler")); codeRemoveMethod.Attributes = this.memberAttributes; this.removeMethod = new DesignTimeMethodInfo(declaringType, codeRemoveMethod, true); } } return this.removeMethod; } public override MethodInfo GetRaiseMethod(bool nonPublic) { return null; } public override EventAttributes Attributes { //We're not interested in this flag get { return default(EventAttributes); } } #endregion #region MemberInfo Overrides public override string Name { get { return this.name; } } public override Type DeclaringType { get { return this.declaringType; } } public override Type ReflectedType { get { return this.declaringType; } } public override object[] GetCustomAttributes(bool inherit) { return GetCustomAttributes(typeof(object), inherit); } public override object[] GetCustomAttributes(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); if (this.attributes == null) this.attributes = Helper.LoadCustomAttributes(this.codeDomEvent.CustomAttributes, this.DeclaringType as DesignTimeType); return Helper.GetCustomAttributes(attributeType, inherit, this.attributes, this); } public override bool IsDefined(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); if (this.attributes == null) this.attributes = Helper.LoadCustomAttributes(this.codeDomEvent.CustomAttributes, this.DeclaringType as DesignTimeType); if (Helper.IsDefined(attributeType, inherit, attributes, this)) return true; return false; } #endregion #region Helpers internal bool IsPublic { get { return ((memberAttributes & MemberAttributes.Public) != 0); } } internal bool IsStatic { get { return ((memberAttributes & MemberAttributes.Static) != 0); } } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
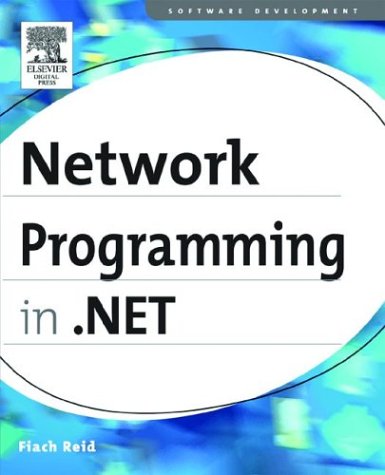
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonFieldBase.cs
- XmlCharType.cs
- XmlSerializerNamespaces.cs
- DefaultBindingPropertyAttribute.cs
- Constraint.cs
- ConfigurationSectionGroup.cs
- PasswordPropertyTextAttribute.cs
- DataGridViewCellStateChangedEventArgs.cs
- CSharpCodeProvider.cs
- BindValidator.cs
- CalendarItem.cs
- PeerNameResolver.cs
- UIElement.cs
- ViewValidator.cs
- DbUpdateCommandTree.cs
- ThemeDirectoryCompiler.cs
- DataReaderContainer.cs
- MenuItem.cs
- EncryptedKey.cs
- FolderLevelBuildProviderCollection.cs
- PasswordBox.cs
- Visual3DCollection.cs
- DataSourceControl.cs
- ConfigXmlSignificantWhitespace.cs
- RtfToXamlLexer.cs
- BlurBitmapEffect.cs
- HandleInitializationContext.cs
- ConfigXmlAttribute.cs
- DependencyObjectValidator.cs
- GridItemPattern.cs
- SqlServer2KCompatibilityCheck.cs
- DataGridViewHeaderCell.cs
- ImageIndexConverter.cs
- TreeNode.cs
- ZoomPercentageConverter.cs
- ReadOnlyPropertyMetadata.cs
- ThousandthOfEmRealPoints.cs
- Object.cs
- InsufficientMemoryException.cs
- smtpconnection.cs
- PenLineJoinValidation.cs
- CodeGroup.cs
- ProcessThreadCollection.cs
- SoapProtocolReflector.cs
- filewebrequest.cs
- SHA384.cs
- AutomationTextAttribute.cs
- QilFactory.cs
- ToolStripOverflowButton.cs
- DiscoveryDocumentReference.cs
- TraceHandlerErrorFormatter.cs
- SelectionUIService.cs
- ExpressionConverter.cs
- AccessControlEntry.cs
- DataTableClearEvent.cs
- ClientFormsAuthenticationCredentials.cs
- KerberosReceiverSecurityToken.cs
- RelationshipSet.cs
- ModelPerspective.cs
- FormViewRow.cs
- StateBag.cs
- UnsafeNativeMethodsMilCoreApi.cs
- RunWorkerCompletedEventArgs.cs
- WindowsStreamSecurityUpgradeProvider.cs
- StrongNameIdentityPermission.cs
- ColorTranslator.cs
- WorkflowApplicationEventArgs.cs
- TransportSecurityProtocol.cs
- MembershipSection.cs
- NetworkAddressChange.cs
- TimeSpan.cs
- VectorConverter.cs
- LineInfo.cs
- AtlasWeb.Designer.cs
- StreamInfo.cs
- Clipboard.cs
- CodeNamespaceCollection.cs
- RegisteredHiddenField.cs
- SqlTransaction.cs
- WinInet.cs
- HttpHeaderCollection.cs
- ScriptingProfileServiceSection.cs
- PersistenceTypeAttribute.cs
- WindowsFormsLinkLabel.cs
- ChildrenQuery.cs
- PageBorderless.cs
- StyleCollection.cs
- XmlUTF8TextReader.cs
- EdmSchemaAttribute.cs
- TextDecoration.cs
- ObjectSet.cs
- BitmapEffectDrawingContextWalker.cs
- EventLogPermissionAttribute.cs
- ToolStripTextBox.cs
- StyleBamlTreeBuilder.cs
- ReaderOutput.cs
- Triplet.cs
- ExtenderProvidedPropertyAttribute.cs
- ImageDrawing.cs
- NextPreviousPagerField.cs