Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / StrongNameSignatureInformation.cs / 1305376 / StrongNameSignatureInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Security.Cryptography; namespace System.Security.Cryptography { ////// Details about a strong name signature /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class StrongNameSignatureInformation { private SignatureVerificationResult m_verificationResult; private AsymmetricAlgorithm m_publicKey; // All strong name signatures use SHA1 as their hash algorithm private static readonly string StrongNameHashAlgorithm = CapiNative.GetAlgorithmName(CapiNative.AlgorithmId.Sha1); internal StrongNameSignatureInformation(AsymmetricAlgorithm publicKey) { Debug.Assert(publicKey != null, "publicKey != null"); m_verificationResult = SignatureVerificationResult.Valid; m_publicKey = publicKey; } internal StrongNameSignatureInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid, "error != SignatureVerificationResult.Valid"); m_verificationResult = error; } ////// Hash algorithm used in calculating the strong name signature /// public string HashAlgorithm { get { return StrongNameHashAlgorithm; } } ////// HRESULT version of the result code /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// Is the strong name signature valid, or was there some form of error /// public bool IsValid { get { return m_verificationResult == SignatureVerificationResult.Valid; } } ////// Public key used to create the signature /// public AsymmetricAlgorithm PublicKey { get { return m_publicKey; } } ////// Results of verifying the strong name signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
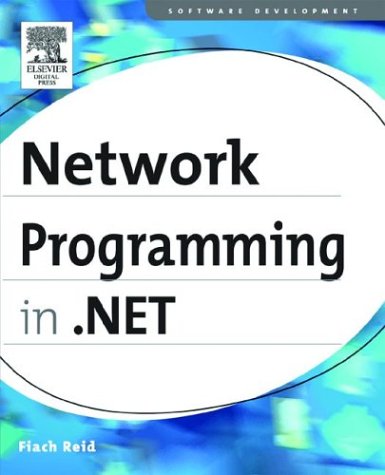
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbFactory.cs
- DbDataAdapter.cs
- TextBoxView.cs
- Clause.cs
- ShellProvider.cs
- SqlCommand.cs
- ByteStreamGeometryContext.cs
- XmlAtomicValue.cs
- Baml2006Reader.cs
- WebResourceAttribute.cs
- HTTPNotFoundHandler.cs
- DocumentSequenceHighlightLayer.cs
- TextBounds.cs
- Gdiplus.cs
- CacheEntry.cs
- _ListenerRequestStream.cs
- QualifierSet.cs
- SqlDataSourceCommandEventArgs.cs
- LoginName.cs
- PermissionSetEnumerator.cs
- IndexerNameAttribute.cs
- LineGeometry.cs
- DupHandleConnectionReader.cs
- HttpDebugHandler.cs
- TableLayoutPanel.cs
- RightsManagementPermission.cs
- IPPacketInformation.cs
- FocusWithinProperty.cs
- URLMembershipCondition.cs
- DataGridColumnsPage.cs
- Timer.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- CreateUserErrorEventArgs.cs
- exports.cs
- SortableBindingList.cs
- HybridDictionary.cs
- PenContexts.cs
- OleDbCommandBuilder.cs
- XmlConvert.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- XDRSchema.cs
- DbConnectionStringBuilder.cs
- DataGridBeginningEditEventArgs.cs
- GetPageNumberCompletedEventArgs.cs
- HighlightVisual.cs
- TableDetailsRow.cs
- safemediahandle.cs
- StorageComplexTypeMapping.cs
- TileBrush.cs
- SqlProvider.cs
- IisTraceListener.cs
- SortAction.cs
- ParallelTimeline.cs
- DrawingServices.cs
- ContentIterators.cs
- SrgsRuleRef.cs
- RtfToXamlReader.cs
- ByteStreamBufferedMessageData.cs
- BuildManagerHost.cs
- ImageSource.cs
- ToolStripControlHost.cs
- FlowDocumentFormatter.cs
- MediaPlayerState.cs
- RegexGroupCollection.cs
- Attachment.cs
- PropertyDescriptor.cs
- VisualState.cs
- RoutedEventConverter.cs
- StructureChangedEventArgs.cs
- TileBrush.cs
- ProviderConnectionPointCollection.cs
- GridViewHeaderRowPresenter.cs
- LogicalMethodInfo.cs
- LocatorPart.cs
- Matrix3DStack.cs
- BitmapEffectInput.cs
- EventLogPermissionEntry.cs
- PauseStoryboard.cs
- FocusChangedEventArgs.cs
- TraceSource.cs
- ExpressionEditorAttribute.cs
- Geometry3D.cs
- ObjectMemberMapping.cs
- UrlParameterWriter.cs
- ParameterCollection.cs
- ConnectorDragDropGlyph.cs
- DbConnectionOptions.cs
- QueryResponse.cs
- CSharpCodeProvider.cs
- PageAction.cs
- ImmutableObjectAttribute.cs
- DbConnectionPoolGroupProviderInfo.cs
- IndexOutOfRangeException.cs
- ScriptModule.cs
- BitmapMetadata.cs
- ImageList.cs
- GridViewColumnHeader.cs
- DataGridViewCellStyle.cs
- RegexWorker.cs
- ParentControlDesigner.cs