Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Serialization / XmlAttributes.cs / 1305376 / XmlAttributes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System; using System.Reflection; using System.Collections; using System.ComponentModel; internal enum XmlAttributeFlags { Enum = 0x1, Array = 0x2, Text = 0x4, ArrayItems = 0x8, Elements = 0x10, Attribute = 0x20, Root = 0x40, Type = 0x80, AnyElements = 0x100, AnyAttribute = 0x200, ChoiceIdentifier = 0x400, XmlnsDeclarations = 0x800, } ////// /// public class XmlAttributes { XmlElementAttributes xmlElements = new XmlElementAttributes(); XmlArrayItemAttributes xmlArrayItems = new XmlArrayItemAttributes(); XmlAnyElementAttributes xmlAnyElements = new XmlAnyElementAttributes(); XmlArrayAttribute xmlArray; XmlAttributeAttribute xmlAttribute; XmlTextAttribute xmlText; XmlEnumAttribute xmlEnum; bool xmlIgnore; bool xmlns; object xmlDefaultValue = null; XmlRootAttribute xmlRoot; XmlTypeAttribute xmlType; XmlAnyAttributeAttribute xmlAnyAttribute; XmlChoiceIdentifierAttribute xmlChoiceIdentifier; static Type ignoreAttributeType; ///[To be supplied.] ////// /// public XmlAttributes() { } internal XmlAttributeFlags XmlFlags { get { XmlAttributeFlags flags = 0; if (xmlElements.Count > 0) flags |= XmlAttributeFlags.Elements; if (xmlArrayItems.Count > 0) flags |= XmlAttributeFlags.ArrayItems; if (xmlAnyElements.Count > 0) flags |= XmlAttributeFlags.AnyElements; if (xmlArray != null) flags |= XmlAttributeFlags.Array; if (xmlAttribute != null) flags |= XmlAttributeFlags.Attribute; if (xmlText != null) flags |= XmlAttributeFlags.Text; if (xmlEnum != null) flags |= XmlAttributeFlags.Enum; if (xmlRoot != null) flags |= XmlAttributeFlags.Root; if (xmlType != null) flags |= XmlAttributeFlags.Type; if (xmlAnyAttribute != null) flags |= XmlAttributeFlags.AnyAttribute; if (xmlChoiceIdentifier != null) flags |= XmlAttributeFlags.ChoiceIdentifier; if (xmlns) flags |= XmlAttributeFlags.XmlnsDeclarations; return flags; } } private static Type IgnoreAttribute { get { if (ignoreAttributeType == null) { ignoreAttributeType = typeof(object).Assembly.GetType("System.XmlIgnoreMemberAttribute"); if (ignoreAttributeType == null) { ignoreAttributeType = typeof(XmlIgnoreAttribute); } } return ignoreAttributeType; } } ///[To be supplied.] ////// /// public XmlAttributes(ICustomAttributeProvider provider) { object[] attrs = provider.GetCustomAttributes(false); // most generic[To be supplied.] ///matches everithig XmlAnyElementAttribute wildcard = null; for (int i = 0; i < attrs.Length; i++) { if (attrs[i] is XmlIgnoreAttribute || attrs[i] is ObsoleteAttribute || attrs[i].GetType() == IgnoreAttribute) { xmlIgnore = true; break; } else if (attrs[i] is XmlElementAttribute) { this.xmlElements.Add((XmlElementAttribute)attrs[i]); } else if (attrs[i] is XmlArrayItemAttribute) { this.xmlArrayItems.Add((XmlArrayItemAttribute)attrs[i]); } else if (attrs[i] is XmlAnyElementAttribute) { XmlAnyElementAttribute any = (XmlAnyElementAttribute)attrs[i]; if ((any.Name == null || any.Name.Length == 0) && any.NamespaceSpecified && any.Namespace == null) { // ignore duplicate wildcards wildcard = any; } else { this.xmlAnyElements.Add((XmlAnyElementAttribute)attrs[i]); } } else if (attrs[i] is DefaultValueAttribute) { this.xmlDefaultValue = ((DefaultValueAttribute)attrs[i]).Value; } else if (attrs[i] is XmlAttributeAttribute) { this.xmlAttribute = (XmlAttributeAttribute)attrs[i]; } else if (attrs[i] is XmlArrayAttribute) { this.xmlArray = (XmlArrayAttribute)attrs[i]; } else if (attrs[i] is XmlTextAttribute) { this.xmlText = (XmlTextAttribute)attrs[i]; } else if (attrs[i] is XmlEnumAttribute) { this.xmlEnum = (XmlEnumAttribute)attrs[i]; } else if (attrs[i] is XmlRootAttribute) { this.xmlRoot = (XmlRootAttribute)attrs[i]; } else if (attrs[i] is XmlTypeAttribute) { this.xmlType = (XmlTypeAttribute)attrs[i]; } else if (attrs[i] is XmlAnyAttributeAttribute) { this.xmlAnyAttribute = (XmlAnyAttributeAttribute)attrs[i]; } else if (attrs[i] is XmlChoiceIdentifierAttribute) { this.xmlChoiceIdentifier = (XmlChoiceIdentifierAttribute)attrs[i]; } else if (attrs[i] is XmlNamespaceDeclarationsAttribute) { this.xmlns = true; } } if (xmlIgnore) { this.xmlElements.Clear(); this.xmlArrayItems.Clear(); this.xmlAnyElements.Clear(); this.xmlDefaultValue = null; this.xmlAttribute = null; this.xmlArray = null; this.xmlText = null; this.xmlEnum = null; this.xmlType = null; this.xmlAnyAttribute = null; this.xmlChoiceIdentifier = null; this.xmlns = false; } else { if (wildcard != null) { this.xmlAnyElements.Add(wildcard); } } } internal static object GetAttr(ICustomAttributeProvider provider, Type attrType) { object[] attrs = provider.GetCustomAttributes(attrType, false); if (attrs.Length == 0) return null; return attrs[0]; } /// /// /// public XmlElementAttributes XmlElements { get { return xmlElements; } } ///[To be supplied.] ////// /// public XmlAttributeAttribute XmlAttribute { get { return xmlAttribute; } set { xmlAttribute = value; } } ///[To be supplied.] ////// /// public XmlEnumAttribute XmlEnum { get { return xmlEnum; } set { xmlEnum = value; } } ///[To be supplied.] ////// /// public XmlTextAttribute XmlText { get { return xmlText; } set { xmlText = value; } } ///[To be supplied.] ////// /// public XmlArrayAttribute XmlArray { get { return xmlArray; } set { xmlArray = value; } } ///[To be supplied.] ////// /// public XmlArrayItemAttributes XmlArrayItems { get { return xmlArrayItems; } } ///[To be supplied.] ////// /// public object XmlDefaultValue { get { return xmlDefaultValue; } set { xmlDefaultValue = value; } } ///[To be supplied.] ////// /// public bool XmlIgnore { get { return xmlIgnore; } set { xmlIgnore = value; } } ///[To be supplied.] ////// /// public XmlTypeAttribute XmlType { get { return xmlType; } set { xmlType = value; } } ///[To be supplied.] ////// /// public XmlRootAttribute XmlRoot { get { return xmlRoot; } set { xmlRoot = value; } } ///[To be supplied.] ////// /// public XmlAnyElementAttributes XmlAnyElements { get { return xmlAnyElements; } } ///[To be supplied.] ////// /// public XmlAnyAttributeAttribute XmlAnyAttribute { get { return xmlAnyAttribute; } set { xmlAnyAttribute = value; } } ///[To be supplied.] ///public XmlChoiceIdentifierAttribute XmlChoiceIdentifier { get { return xmlChoiceIdentifier; } } /// /// /// public bool Xmlns { get { return xmlns; } set { xmlns = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
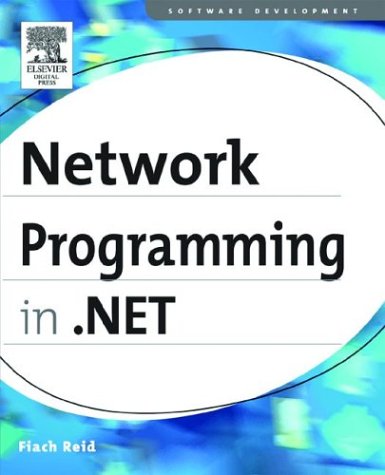
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReliableMessagingHelpers.cs
- RecordManager.cs
- NativeMethods.cs
- AutomationElement.cs
- LocalizationParserHooks.cs
- DesignerView.cs
- PageCatalogPart.cs
- RoleManagerSection.cs
- OleDbConnection.cs
- DataGridRowClipboardEventArgs.cs
- XhtmlBasicLiteralTextAdapter.cs
- InkCanvasAutomationPeer.cs
- ButtonColumn.cs
- KeyEvent.cs
- HashCryptoHandle.cs
- XmlParserContext.cs
- XmlSchemaComplexContent.cs
- OverflowException.cs
- XmlTextReaderImplHelpers.cs
- ListArgumentProvider.cs
- AggregateException.cs
- EventHandlerList.cs
- DynamicPropertyHolder.cs
- IdentityValidationException.cs
- SortedSetDebugView.cs
- ClientTargetSection.cs
- BamlTreeMap.cs
- ContainerParagraph.cs
- XamlHttpHandlerFactory.cs
- BindingsCollection.cs
- GreenMethods.cs
- QueryCoreOp.cs
- VideoDrawing.cs
- SystemInfo.cs
- XMLSyntaxException.cs
- ListenerElementsCollection.cs
- RawStylusInputCustomDataList.cs
- ModelPerspective.cs
- MemoryPressure.cs
- TemplateKey.cs
- AppModelKnownContentFactory.cs
- DelegatingTypeDescriptionProvider.cs
- SQLInt64.cs
- SvcMapFileLoader.cs
- Wizard.cs
- AccessKeyManager.cs
- NativeMethodsOther.cs
- DataGridViewDataErrorEventArgs.cs
- DataControlImageButton.cs
- CacheVirtualItemsEvent.cs
- X509SecurityTokenAuthenticator.cs
- TypeConverterValueSerializer.cs
- TextContainer.cs
- ConfigurationSectionGroup.cs
- Console.cs
- PKCS1MaskGenerationMethod.cs
- FillErrorEventArgs.cs
- DocumentSequence.cs
- CodePrimitiveExpression.cs
- QilCloneVisitor.cs
- input.cs
- Viewport2DVisual3D.cs
- ElementHost.cs
- EventlogProvider.cs
- TypeSchema.cs
- ValueUtilsSmi.cs
- ExpressionBindingCollection.cs
- CompositeDataBoundControl.cs
- CheckoutException.cs
- SystemDropShadowChrome.cs
- ScrollPatternIdentifiers.cs
- SpecialNameAttribute.cs
- HintTextConverter.cs
- FragmentQueryProcessor.cs
- ContentDisposition.cs
- SqlFactory.cs
- ExpandCollapsePattern.cs
- TextEditorTables.cs
- MouseDevice.cs
- MergePropertyDescriptor.cs
- FocusTracker.cs
- DeploymentExceptionMapper.cs
- X509Certificate2Collection.cs
- CaseStatement.cs
- TypeConverterHelper.cs
- CryptoStream.cs
- RepeatBehavior.cs
- DataTablePropertyDescriptor.cs
- ExpressionBuilderCollection.cs
- XsltConvert.cs
- SoapReflectionImporter.cs
- XmlSchemaCollection.cs
- EtwTrace.cs
- VirtualDirectoryMappingCollection.cs
- CheckBox.cs
- XmlHierarchyData.cs
- ProxyManager.cs
- SchemaTypeEmitter.cs
- PropertyManager.cs
- XPathParser.cs