Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / ProxyManager.cs / 1 / ProxyManager.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.ServiceModel.Channels; using System.Runtime.InteropServices; using System.Collections.Generic; using System.ServiceModel; using System.Runtime.Remoting.Proxies; using System.Threading; internal class ProxyManager : IProxyManager { IProxyCreator proxyCreator; internal ProxyManager (IProxyCreator proxyCreator) { this.proxyCreator = proxyCreator; InterfaceIDToComProxy = new Dictionary(); } bool IsIntrinsic (ref Guid riid) { if ( (riid == typeof (IChannelOptions).GUID) || (riid == typeof (IChannelCredentials).GUID) ) return true; return false; } void IProxyManager.TearDownChannels () { lock (this) { IEnumerator > enumeratorInterfaces = InterfaceIDToComProxy.GetEnumerator (); while (enumeratorInterfaces.MoveNext ()) { KeyValuePair current = enumeratorInterfaces.Current; IDisposable comProxy = current.Value as IDisposable; if (comProxy == null) DiagnosticUtility.DebugAssert("comProxy should not be null"); else comProxy.Dispose (); } InterfaceIDToComProxy.Clear (); proxyCreator.Dispose (); enumeratorInterfaces.Dispose (); proxyCreator = null; } } Dictionary InterfaceIDToComProxy ; ComProxy CreateServiceChannel (IntPtr outerProxy, ref Guid riid) { return proxyCreator.CreateProxy (outerProxy, ref riid); } ComProxy GenerateIntrinsic (IntPtr outerProxy, ref Guid riid) { if (proxyCreator.SupportsIntrinsics ()) { if (riid == typeof (IChannelOptions).GUID) return ChannelOptions.Create (outerProxy, proxyCreator as IProvideChannelBuilderSettings) ; else if (riid == typeof (IChannelCredentials).GUID) return ChannelCredentials.Create (outerProxy, proxyCreator as IProvideChannelBuilderSettings) ; else { DiagnosticUtility.DebugAssert("Given IID is not an intrinsic"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } else { DiagnosticUtility.DebugAssert("proxyCreator does not support intrinsic"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } void FindOrCreateProxyInternal (IntPtr outerProxy, ref Guid riid, out ComProxy comProxy) { comProxy = null; lock (this) { InterfaceIDToComProxy.TryGetValue(riid, out comProxy); if (comProxy == null) { if (IsIntrinsic(ref riid)) comProxy = GenerateIntrinsic(outerProxy, ref riid); else comProxy = CreateServiceChannel(outerProxy, ref riid); InterfaceIDToComProxy[riid] = comProxy; } } if (comProxy == null) { DiagnosticUtility.DebugAssert("comProxy should not be null at this point"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } int IProxyManager.FindOrCreateProxy (IntPtr outerProxy, ref Guid riid, out IntPtr tearOff) { tearOff = IntPtr.Zero; try { ComProxy comProxy = null; FindOrCreateProxyInternal (outerProxy, ref riid, out comProxy); comProxy.QueryInterface (ref riid, out tearOff); return HR.S_OK; } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; e = e.GetBaseException (); return Marshal.GetHRForException (e); } } int IProxyManager.InterfaceSupportsErrorInfo (ref Guid riid) { if (IsIntrinsic (ref riid)) return HR.S_OK; else return proxyCreator.SupportsErrorInfo (ref riid) ? HR.S_OK : HR.S_FALSE; } void IProxyManager.GetIDsOfNames( [MarshalAs(UnmanagedType.LPWStr)] string name, IntPtr pDispID) { Int32 dispID = -1; switch (name) { case "ChannelOptions": dispID = 1; break; case "ChannelCredentials": dispID = 2; break; } Marshal.WriteInt32 (pDispID, (int) dispID); } int IProxyManager.Invoke( UInt32 dispIdMember, IntPtr outerProxy, IntPtr pVarResult, IntPtr pExcepInfo ) { try { ComProxy comProxy = null; Guid riid; if ((dispIdMember == 1)) riid = typeof (IChannelOptions).GUID; else if ((dispIdMember == 2)) riid = typeof (IChannelCredentials).GUID; else return HR.DISP_E_MEMBERNOTFOUND; FindOrCreateProxyInternal (outerProxy, ref riid, out comProxy); TagVariant variant = new TagVariant (); variant.vt = (ushort)VarEnum.VT_DISPATCH; IntPtr tearOffDispatch = IntPtr.Zero; comProxy.QueryInterface (ref riid, out tearOffDispatch); variant.ptr = tearOffDispatch; Marshal.StructureToPtr (variant, pVarResult, true); return HR.S_OK; } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; if (pExcepInfo != IntPtr.Zero) { System.Runtime.InteropServices.ComTypes.EXCEPINFO exceptionInfo = new System.Runtime.InteropServices.ComTypes.EXCEPINFO () ; e = e.GetBaseException (); exceptionInfo.bstrDescription = e.Message; exceptionInfo.bstrSource = e.Source; exceptionInfo.scode = Marshal.GetHRForException (e); Marshal.StructureToPtr (exceptionInfo, pExcepInfo, false); } return HR.DISP_E_EXCEPTION; } } int IProxyManager.SupportsDispatch () { if (proxyCreator.SupportsDispatch ()) return HR.S_OK; else return HR.E_FAIL; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
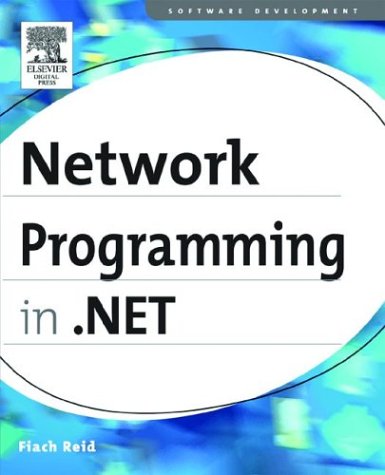
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiscoveryClientProtocol.cs
- CellParagraph.cs
- DataGrid.cs
- Assert.cs
- KnownBoxes.cs
- CodeBlockBuilder.cs
- WindowProviderWrapper.cs
- SplineKeyFrames.cs
- HybridWebProxyFinder.cs
- QilXmlReader.cs
- PublisherMembershipCondition.cs
- TraceSection.cs
- MetadataArtifactLoaderCompositeResource.cs
- ButtonColumn.cs
- DbMetaDataFactory.cs
- XmlHierarchicalEnumerable.cs
- KnownTypesProvider.cs
- NonBatchDirectoryCompiler.cs
- ApplicationInfo.cs
- MenuItem.cs
- FixedSchema.cs
- FileSystemEventArgs.cs
- UInt32Converter.cs
- WebAdminConfigurationHelper.cs
- IUnknownConstantAttribute.cs
- externdll.cs
- ServiceModelEnumValidator.cs
- AutoCompleteStringCollection.cs
- DataGridViewRowsAddedEventArgs.cs
- datacache.cs
- ExecutionScope.cs
- FloaterBaseParaClient.cs
- CodeAttributeArgument.cs
- ValidationHelper.cs
- BaseTypeViewSchema.cs
- StatusStrip.cs
- CompModSwitches.cs
- basecomparevalidator.cs
- NativeMethods.cs
- ServiceHttpModule.cs
- ProfileInfo.cs
- StorageComplexTypeMapping.cs
- XmlUTF8TextReader.cs
- ipaddressinformationcollection.cs
- WebPartEventArgs.cs
- XmlNamespaceMapping.cs
- DbConnectionHelper.cs
- ServiceDebugElement.cs
- SqlConnectionManager.cs
- ResXResourceWriter.cs
- DataColumnPropertyDescriptor.cs
- TransactionCache.cs
- ColumnWidthChangedEvent.cs
- FileDialogCustomPlace.cs
- DataGridViewComboBoxEditingControl.cs
- ExtendedPropertyCollection.cs
- SqlDelegatedTransaction.cs
- TypeConverter.cs
- FlowLayout.cs
- InputLanguage.cs
- XmlComment.cs
- StorageRoot.cs
- Overlapped.cs
- ScriptRegistrationManager.cs
- GeneralTransform3DTo2DTo3D.cs
- RegistrationServices.cs
- InkCollectionBehavior.cs
- SoapSchemaExporter.cs
- OdbcTransaction.cs
- SplashScreen.cs
- SmiEventStream.cs
- Size.cs
- FilteredAttributeCollection.cs
- HtmlInputControl.cs
- QilStrConcatenator.cs
- HtmlInputImage.cs
- ViewPort3D.cs
- DesignConnectionCollection.cs
- ConnectionStringsExpressionBuilder.cs
- DbSetClause.cs
- IDQuery.cs
- JsonDataContract.cs
- ChannelCredentials.cs
- PageOutputColor.cs
- future.cs
- Lock.cs
- AsmxEndpointPickerExtension.cs
- WindowsFormsSectionHandler.cs
- LinkLabelLinkClickedEvent.cs
- ConfigurationErrorsException.cs
- ChannelBinding.cs
- HitTestParameters3D.cs
- SudsWriter.cs
- IndicFontClient.cs
- XmlConvert.cs
- DefaultValidator.cs
- PerformanceCountersElement.cs
- Renderer.cs
- PrintController.cs
- ComplexPropertyEntry.cs