Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / MetaChildrenColumn.cs / 1305376 / MetaChildrenColumn.cs
using System.Security.Permissions; using System.Collections.Generic; using System.Diagnostics; using System.Web.DynamicData.ModelProviders; using System.Collections; using System.Web.Routing; namespace System.Web.DynamicData { ////// A special column representing 1-many relationships /// public class MetaChildrenColumn : MetaColumn, IMetaChildrenColumn { public MetaChildrenColumn(MetaTable table, ColumnProvider entityMember) : base(table, entityMember) { } ////// Perform initialization logic for this column /// internal protected override void Initialize() { base.Initialize(); AssociationProvider a = this.Provider.Association; ChildTable = Model.GetTable(a.ToTable.Name, Table.DataContextType); if (a.ToColumn != null) { ColumnInOtherTable = ChildTable.GetColumn(a.ToColumn.Name); } } ////// Returns whether this entity set column is in a Many To Many relationship /// public bool IsManyToMany { get { return Provider.Association != null && Provider.Association.Direction == AssociationDirection.ManyToMany; } } ////// The child table (e.g. Products in Categories<-Products) /// public MetaTable ChildTable { get; private set; } ////// A pointer to the MetaColumn in the other table /// public MetaColumn ColumnInOtherTable { get; private set; } ////// Override disabling sorting /// internal override string SortExpressionInternal { get { // children columns are not sortable return String.Empty; } } /*protected*/ internal override bool ScaffoldNoCache { get { // always display 1-many associations return true; } } ////// Shortcut for getting the path to the list action for all entities in the child table that have the given row as a parent. /// /// ///public string GetChildrenListPath(object row) { return GetChildrenPath(PageAction.List, row); } public string GetChildrenPath(string action, object row) { // If there is no row, we can't get a path if (row == null) return String.Empty; return ChildTable.GetActionPath(action, GetRouteValues(row)); } public string GetChildrenPath(string action, object row, string path) { // If there is no row, we can't get a path if (row == null) return String.Empty; if (String.IsNullOrEmpty(path)) { return GetChildrenPath(action, row); } // Build a query string param with our primary key RouteValueDictionary routeValues = GetRouteValues(row); // Add it to the path return QueryStringHandler.AddFiltersToPath(path, routeValues); } private RouteValueDictionary GetRouteValues(object row) { var routeValues = new RouteValueDictionary(); IList
Link Menu
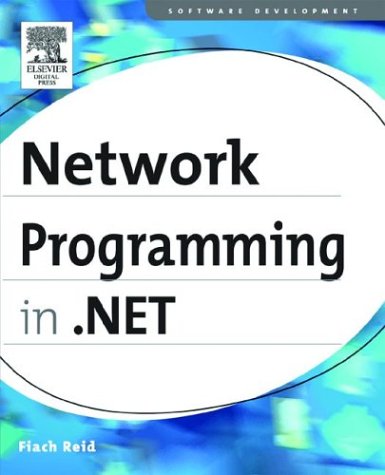
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringHandle.cs
- DrawingGroupDrawingContext.cs
- Attachment.cs
- ListDictionary.cs
- DataGridViewComboBoxColumn.cs
- CurrentChangingEventArgs.cs
- ObjectParameterCollection.cs
- NavigationWindow.cs
- DataError.cs
- ColorContext.cs
- ReceiveCompletedEventArgs.cs
- ContainerAction.cs
- DataQuery.cs
- ListMarkerSourceInfo.cs
- WebEventTraceProvider.cs
- TerminatingOperationBehavior.cs
- SessionIDManager.cs
- PropertyChangeTracker.cs
- ProcessInputEventArgs.cs
- ApplyImportsAction.cs
- RelationshipSet.cs
- Win32KeyboardDevice.cs
- CqlQuery.cs
- FileUpload.cs
- XmlSerializerObjectSerializer.cs
- SingleStorage.cs
- AsymmetricSignatureDeformatter.cs
- KeyToListMap.cs
- XNameConverter.cs
- StreamSecurityUpgradeInitiatorAsyncResult.cs
- OneOfConst.cs
- UriTemplateQueryValue.cs
- SpeechAudioFormatInfo.cs
- WebProxyScriptElement.cs
- GridViewUpdatedEventArgs.cs
- ConfigurationFileMap.cs
- CodeDomConfigurationHandler.cs
- BindingExpression.cs
- AsyncResult.cs
- SqlDataSourceSelectingEventArgs.cs
- SelectionBorderGlyph.cs
- InputBindingCollection.cs
- ByteStream.cs
- OleDbFactory.cs
- XmlLanguageConverter.cs
- WS2007FederationHttpBindingElement.cs
- IInstanceTable.cs
- ProfileSettingsCollection.cs
- WebBrowserEvent.cs
- EmptyControlCollection.cs
- EnumConverter.cs
- WriteableBitmap.cs
- TrackBarRenderer.cs
- ToolStripDropTargetManager.cs
- XmlChildEnumerator.cs
- ConnectionInterfaceCollection.cs
- SByteStorage.cs
- MobileUserControl.cs
- Literal.cs
- EndpointIdentity.cs
- ImageCodecInfoPrivate.cs
- EdmMember.cs
- Debug.cs
- DefaultPrintController.cs
- ByteFacetDescriptionElement.cs
- _NegotiateClient.cs
- Double.cs
- SourceInterpreter.cs
- Highlights.cs
- XPathAncestorQuery.cs
- IntegerValidatorAttribute.cs
- LogicalExpr.cs
- GraphicsState.cs
- TableLayoutPanelCodeDomSerializer.cs
- RoleGroupCollection.cs
- DocumentOrderQuery.cs
- UpdateEventArgs.cs
- AgileSafeNativeMemoryHandle.cs
- InputGestureCollection.cs
- CardSpaceException.cs
- SineEase.cs
- CustomTypeDescriptor.cs
- DbCommandTree.cs
- Int64AnimationUsingKeyFrames.cs
- PrincipalPermission.cs
- Label.cs
- CodePrimitiveExpression.cs
- TypeConverterAttribute.cs
- ControlAdapter.cs
- TypedColumnHandler.cs
- TimelineCollection.cs
- DataSourceXmlElementAttribute.cs
- DbgCompiler.cs
- InkCanvasAutomationPeer.cs
- StyleTypedPropertyAttribute.cs
- PathFigureCollection.cs
- XamlReaderHelper.cs
- HttpListenerException.cs
- Property.cs
- TransformCollection.cs