Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / SkinBuilder.cs / 1305376 / SkinBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Classes related to templated control support * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Globalization; using System.IO; using System.Reflection; using System.Web.Compilation; using System.Web.UI.WebControls; using System.Web.Util; #if !FEATURE_PAL using System.Web.UI.Design; #endif // !FEATURE_PAL ////// public sealed class SkinBuilder : ControlBuilder { private ThemeProvider _provider; private Control _control; private ControlBuilder _skinBuilder; private string _themePath; internal static readonly Object[] EmptyParams = new Object[0]; public SkinBuilder(ThemeProvider provider, Control control, ControlBuilder skinBuilder, string themePath) { _provider = provider; _control = control; _skinBuilder = skinBuilder; _themePath = themePath; } private void ApplyTemplateProperties(Control control) { object[] parameters = new object[1]; ICollection entries = GetFilteredPropertyEntrySet(_skinBuilder.TemplatePropertyEntries); foreach (TemplatePropertyEntry entry in entries) { try { object originalValue = FastPropertyAccessor.GetProperty(control, entry.Name, InDesigner); if (originalValue == null) { ControlBuilder controlBuilder = ((TemplatePropertyEntry)entry).Builder; controlBuilder.SetServiceProvider(ServiceProvider); try { object objectValue = controlBuilder.BuildObject(true); parameters[0] = objectValue; } finally { controlBuilder.SetServiceProvider(null); } MethodInfo methodInfo = entry.PropertyInfo.GetSetMethod(); Util.InvokeMethod(methodInfo, control, parameters); } } catch (Exception e) { Debug.Fail(e.Message); } #pragma warning disable 1058 catch { } #pragma warning restore 1058 } } private void ApplyComplexProperties(Control control) { ICollection entries = GetFilteredPropertyEntrySet(_skinBuilder.ComplexPropertyEntries); foreach (ComplexPropertyEntry entry in entries) { ControlBuilder builder = entry.Builder; if (builder != null) { string propertyName = entry.Name; if (entry.ReadOnly) { object objectValue = FastPropertyAccessor.GetProperty(control, propertyName, InDesigner); if (objectValue == null) continue; entry.Builder.SetServiceProvider(ServiceProvider); try { entry.Builder.InitObject(objectValue); } finally { entry.Builder.SetServiceProvider(null); } } else { object childObj; string actualPropName; object value = entry.Builder.BuildObject(true); // Make the UrlProperty based on theme path for control themes(Must be a string) PropertyDescriptor desc = PropertyMapper.GetMappedPropertyDescriptor(control, PropertyMapper.MapNameToPropertyName(propertyName), out childObj, out actualPropName, InDesigner); if (desc != null) { string str = value as string; if (value != null && desc.Attributes[typeof(UrlPropertyAttribute)] != null && UrlPath.IsRelativeUrl(str)) { value = _themePath + str; } } FastPropertyAccessor.SetProperty(childObj, propertyName, value, InDesigner); } } } } private void ApplySimpleProperties(Control control) { ICollection entries = GetFilteredPropertyEntrySet(_skinBuilder.SimplePropertyEntries); foreach (SimplePropertyEntry entry in entries) { try { if (entry.UseSetAttribute) { SetSimpleProperty(entry, control); continue; } string propertyName = PropertyMapper.MapNameToPropertyName(entry.Name); object childObj; string actualPropName; PropertyDescriptor desc = PropertyMapper.GetMappedPropertyDescriptor(control, propertyName, out childObj, out actualPropName, InDesigner); if (desc != null) { DefaultValueAttribute defValAttr = (DefaultValueAttribute)desc.Attributes[typeof(DefaultValueAttribute)]; object currentValue = desc.GetValue(childObj); // Only apply the themed value if different from default value. if (defValAttr != null && !object.Equals(defValAttr.Value, currentValue)) { continue; } object value = entry.Value; // Make the UrlProperty based on theme path for control themes. string str = value as string; if (value != null && desc.Attributes[typeof(UrlPropertyAttribute)] != null && UrlPath.IsRelativeUrl(str)) { value = _themePath + str; } SetSimpleProperty(entry, control); } } catch (Exception e) { Debug.Fail(e.Message); } #pragma warning disable 1058 catch { } #pragma warning restore 1058 } } private void ApplyBoundProperties(Control control) { DataBindingCollection dataBindings = null; IAttributeAccessor attributeAccessor = null; // If there are no filters in the picture, use the entries as is ICollection entries = GetFilteredPropertyEntrySet(_skinBuilder.BoundPropertyEntries); foreach (BoundPropertyEntry entry in entries) { InitBoundProperty(control, entry, ref dataBindings, ref attributeAccessor); } } private void InitBoundProperty(Control control, BoundPropertyEntry entry, ref DataBindingCollection dataBindings, ref IAttributeAccessor attributeAccessor) { string expressionPrefix = entry.ExpressionPrefix; // If we're in the designer, add the bound properties to the collections if (expressionPrefix.Length == 0) { if (dataBindings == null && control is IDataBindingsAccessor) { dataBindings = ((IDataBindingsAccessor)control).DataBindings; } dataBindings.Add(new DataBinding(entry.Name, entry.Type, entry.Expression.Trim())); } else { throw new InvalidOperationException(SR.GetString(SR.ControlBuilder_ExpressionsNotAllowedInThemes)); } } ///[To be supplied.] ////// public Control ApplyTheme() { if (_skinBuilder != null) { ApplySimpleProperties(_control); ApplyComplexProperties(_control); ApplyBoundProperties(_control); ApplyTemplateProperties(_control); } return _control; } } public sealed class ThemeProvider { private IDictionary _skinBuilders; private string[] _cssFiles; private string _themeName; private string _themePath; private int _contentHashCode; private IDesignerHost _host; public ThemeProvider(IDesignerHost host, string name, string themeDefinition, string[] cssFiles, string themePath) { _themeName = name; _themePath = themePath; _cssFiles = cssFiles; _host = host; ControlBuilder themeBuilder = DesignTimeTemplateParser.ParseTheme(host, themeDefinition, themePath); _contentHashCode = themeDefinition.GetHashCode(); ArrayList subBuilders = themeBuilder.SubBuilders; _skinBuilders = new Hashtable(); for (int i=0; i[To be supplied.] ///
Link Menu
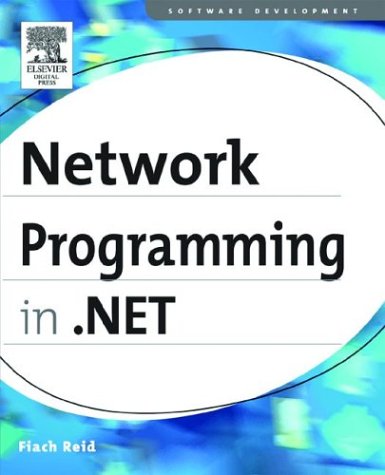
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedReadStream.cs
- Configuration.cs
- MailAddressParser.cs
- ApplicationActivator.cs
- InvalidFilterCriteriaException.cs
- ParserExtension.cs
- ImpersonationContext.cs
- PropagatorResult.cs
- KnownIds.cs
- WebPartVerb.cs
- TriggerActionCollection.cs
- BatchStream.cs
- HtmlTitle.cs
- CheckoutException.cs
- ADConnectionHelper.cs
- StringFunctions.cs
- Decorator.cs
- PaperSize.cs
- ArglessEventHandlerProxy.cs
- RetrieveVirtualItemEventArgs.cs
- Item.cs
- HttpAsyncResult.cs
- ContractReference.cs
- TableCell.cs
- InstanceCreationEditor.cs
- DataBoundControlActionList.cs
- SqlDataSourceCache.cs
- PrivilegeNotHeldException.cs
- PageAction.cs
- FormsAuthenticationConfiguration.cs
- HiddenFieldPageStatePersister.cs
- SourceElementsCollection.cs
- CodeIterationStatement.cs
- Durable.cs
- MethodToken.cs
- StrongNameIdentityPermission.cs
- MouseDevice.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- DynamicResourceExtensionConverter.cs
- ELinqQueryState.cs
- BindingsCollection.cs
- Timer.cs
- FrameworkRichTextComposition.cs
- PropertyChangingEventArgs.cs
- MobileControlDesigner.cs
- ParameterSubsegment.cs
- DNS.cs
- Semaphore.cs
- CodeTypeMemberCollection.cs
- MenuItemAutomationPeer.cs
- RoutedUICommand.cs
- SendKeys.cs
- ZipIOBlockManager.cs
- WebPartDisplayModeCollection.cs
- MailDefinition.cs
- TableLayoutRowStyleCollection.cs
- DynamicValidator.cs
- SmiContextFactory.cs
- StylusButtonCollection.cs
- Verify.cs
- DataSourceDescriptorCollection.cs
- BufferedGraphicsManager.cs
- CacheHelper.cs
- Slider.cs
- isolationinterop.cs
- TypedReference.cs
- CurrencyWrapper.cs
- UserThread.cs
- HasCopySemanticsAttribute.cs
- KnownTypes.cs
- CounterSample.cs
- NetMsmqBinding.cs
- GreenMethods.cs
- SelectionProviderWrapper.cs
- ItemMap.cs
- XamlToRtfWriter.cs
- ColorConverter.cs
- SelectionProcessor.cs
- MethodInfo.cs
- CancelEventArgs.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- WebPartTransformerCollection.cs
- Aes.cs
- XmlEnumAttribute.cs
- Soap11ServerProtocol.cs
- CollectionTypeElement.cs
- DataGridViewCellPaintingEventArgs.cs
- ConfigurationValidatorBase.cs
- Cell.cs
- MailHeaderInfo.cs
- TextEditorMouse.cs
- StringConverter.cs
- HttpFileCollection.cs
- ValueUtilsSmi.cs
- XmlSchemaAll.cs
- WebBrowserUriTypeConverter.cs
- DesignerWebPartChrome.cs
- ConfigXmlCDataSection.cs
- RestClientProxyHandler.cs
- ConfigurationStrings.cs