Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Decorator.cs / 1305600 / Decorator.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Border.cs // // Description: Contains the Decorator class. // Spec at http://avalon/layout/Specs/Decorator.xml // // History: // 06/12/2003 : greglett - Added to WCP branch // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Controls; using MS.Utility; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Markup; // IAddChild, ContentPropertyAttribute namespace System.Windows.Controls { ////// Decorator is a base class for elements that apply effects onto or around a single child. /// [Localizability(LocalizationCategory.Ignore, Readability = Readability.Unreadable)] [ContentProperty("Child")] public class Decorator : FrameworkElement, IAddChild { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Decorator() : base() { } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// This method is called to Add the object as a child of the Decorator. This method is used primarily /// by the parser; a more direct way of adding a child to a Decorator is to use the /// /// The object to add as a child; it must be a UIElement. /// void IAddChild.AddChild (Object value) { if (!(value is UIElement)) { throw new ArgumentException (SR.Get(SRID.UnexpectedParameterType, value.GetType(), typeof(UIElement)), "value"); } if (this.Child != null) { throw new ArgumentException(SR.Get(SRID.CanOnlyHaveOneChild, this.GetType(), value.GetType())); } this.Child = (UIElement)value; } ////// property. /// /// This method is called by the parser when text appears under the tag in markup. /// As Decorators do not support text, calling this method has no effect if the text /// is all whitespace. For non-whitespace text, throw an exception. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// The single child of a [DefaultValue(null)] public virtual UIElement Child { get { return _child; } set { if(_child != value) { // notify the visual layer that the old child has been removed. RemoveVisualChild(_child); //need to remove old element from logical tree RemoveLogicalChild(_child); _child = value; AddLogicalChild(value); // notify the visual layer about the new child. AddVisualChild(value); InvalidateMeasure(); } } } ////// /// Returns enumerator to logical children. /// protected internal override IEnumerator LogicalChildren { get { if (_child == null) { return EmptyEnumerator.Instance; } return new SingleChildEnumerator(_child); } } #endregion //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Returns the Visual children count. /// protected override int VisualChildrenCount { get { return (_child == null) ? 0 : 1; } } ////// Returns the child at the specified index. /// protected override Visual GetVisualChild(int index) { if ( (_child == null) || (index != 0)) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return _child; } ////// Updates DesiredSize of the Decorator. Called by parent UIElement. This is the first pass of layout. /// ////// Decorator determines a desired size it needs from the child's sizing properties, margin, and requested size. /// /// Constraint size is an "upper limit" that the return value should not exceed. ///The Decorator's desired size. protected override Size MeasureOverride(Size constraint) { UIElement child = Child; if (child != null) { Helper.SetMeasureDataOnChild(this, child, constraint); // pass along MeasureData so it continues down the tree. child.Measure(constraint); return (child.DesiredSize); } return (new Size()); } ////// Decorator computes the position of its single child inside child's Margin and calls Arrange /// on the child. /// /// Size the Decorator will assume. protected override Size ArrangeOverride(Size arrangeSize) { UIElement child = Child; if (child != null) { child.Arrange(new Rect(arrangeSize)); } return (arrangeSize); } #endregion #region Internal Properties internal UIElement IntChild { get { return _child; } set { _child = value; } } #endregion #region Private Members UIElement _child; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
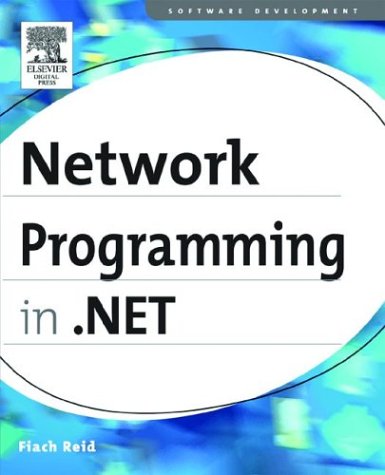
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebMessageEncodingElement.cs
- TriggerCollection.cs
- PackageRelationshipSelector.cs
- EntityKey.cs
- PngBitmapEncoder.cs
- XXXOnTypeBuilderInstantiation.cs
- Application.cs
- Ipv6Element.cs
- X509SecurityTokenAuthenticator.cs
- CheckBox.cs
- DynamicFilter.cs
- XmlSchemaObjectCollection.cs
- UInt16Storage.cs
- ShapeTypeface.cs
- ArrangedElementCollection.cs
- WsdlBuildProvider.cs
- SplitContainerDesigner.cs
- WebControl.cs
- FirstMatchCodeGroup.cs
- SelectionRange.cs
- VectorConverter.cs
- ResponseBodyWriter.cs
- PerformanceCounterManager.cs
- Inflater.cs
- SplitterCancelEvent.cs
- DetailsViewModeEventArgs.cs
- TrackingMemoryStream.cs
- UrlMappingCollection.cs
- PageAsyncTask.cs
- FolderBrowserDialog.cs
- __Filters.cs
- ConfigurationSectionCollection.cs
- FocusTracker.cs
- BufferModesCollection.cs
- SQLDecimalStorage.cs
- EventLogException.cs
- SqlError.cs
- Polygon.cs
- DropShadowEffect.cs
- FastPropertyAccessor.cs
- filewebrequest.cs
- DuplicateWaitObjectException.cs
- Canonicalizers.cs
- ThicknessConverter.cs
- RestHandlerFactory.cs
- RegionInfo.cs
- TakeOrSkipWhileQueryOperator.cs
- AtomContentProperty.cs
- SingleKeyFrameCollection.cs
- InheritedPropertyChangedEventArgs.cs
- PersonalizationAdministration.cs
- ToolStripOverflowButton.cs
- DataReceivedEventArgs.cs
- EventKeyword.cs
- ObjectContext.cs
- Transform3DCollection.cs
- SchemaNames.cs
- ZipIOExtraFieldPaddingElement.cs
- WSHttpBinding.cs
- ColorAnimationBase.cs
- ProcessThreadCollection.cs
- RawStylusSystemGestureInputReport.cs
- Utils.cs
- Tile.cs
- DirectoryNotFoundException.cs
- UnionCodeGroup.cs
- RegexCode.cs
- FacetDescriptionElement.cs
- FixUpCollection.cs
- LinqDataSourceStatusEventArgs.cs
- CellLabel.cs
- SimpleParser.cs
- GenerateTemporaryTargetAssembly.cs
- PasswordTextNavigator.cs
- ChannelPool.cs
- DataList.cs
- TypeHelpers.cs
- HScrollBar.cs
- RowVisual.cs
- BufferedReceiveManager.cs
- TextPattern.cs
- ExceptionRoutedEventArgs.cs
- PropertyDescriptorGridEntry.cs
- WindowVisualStateTracker.cs
- TreeViewBindingsEditorForm.cs
- WorkflowApplicationCompletedException.cs
- PolyBezierSegment.cs
- IERequestCache.cs
- Fonts.cs
- Psha1DerivedKeyGenerator.cs
- CodeExpressionCollection.cs
- SmtpReplyReader.cs
- SiteOfOriginContainer.cs
- DefaultAuthorizationContext.cs
- EnumerableRowCollectionExtensions.cs
- ClientBuildManagerCallback.cs
- SelectionRange.cs
- PageSetupDialog.cs
- RegisteredScript.cs
- ApplicationServicesHostFactory.cs