Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / Utility / ReadOnlyDictionary.cs / 1305600 / ReadOnlyDictionary.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class defines a generic Read Only dictionary // // History: // 04/19/2005: LGolding: Initial implementation. // 03/06/2006: IgorBel: Switch from the RM specific Use license dictionary to a generic Read Only dictionary that can // be share across multiple scenarios // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; //using System.Collections.Specialized; using System.Windows; // for resources using MS.Internal; // for invariant using SR=MS.Internal.WindowsBase.SR; using SRID=MS.Internal.WindowsBase.SRID; namespace MS.Internal.Utility { ////// This is a generic Read Only Dictionary based on the implementation of the Generic Dictionary /// ////// internal class ReadOnlyDictionary/// The generic Dictionary object exposes six interfaces, so this class exposes the /// same interfaces. The methods and properties in this file are sorted by which /// interface they come from. /// ////// The only reason for most of the code in this class is to ensure that the dictionary /// behaves as read-only. All the read methods just delegate to the underlying generic /// Dictionary object. All the write methods just throw. /// ///: IEnumerable >, ICollection >, IDictionary , IEnumerable, ICollection, IDictionary { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Constructor. /// internal ReadOnlyDictionary(Dictionarydict) { Invariant.Assert(dict != null); _dict = dict; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods //------------------------------------------------------ // IEnumerable > Methods //------------------------------------------------------ #region IEnumerable > Public Methods /// /// Returns an enumerator that iterates through the collection. /// IEnumerator> IEnumerable >.GetEnumerator() { return ((IEnumerable >)_dict).GetEnumerator(); } #endregion IEnumerable > Public Methods //----------------------------------------------------- // ICollection > Methods //------------------------------------------------------ #region ICollection > Methods /// /// Adds a new entry to the collection. /// /// /// The pair to be added. /// ////// Always, because the collection is read-only. /// public void Add(KeyValuePairpair ) { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } /// /// Removes all items from the collection. /// ////// Always, because the collection is read-only. /// public void Clear() { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } ////// Determines whether the collection contains a specified pair. /// /// /// The pair being sought. /// public bool Contains(KeyValuePairpair ) { return ((ICollection >)_dict).Contains(pair); } /// /// Copies the elements of the collection to an array, starting at the specified /// array index. /// public void CopyTo( KeyValuePair[] array, int arrayIndex ) { ((ICollection >)_dict).CopyTo(array, arrayIndex); } /// /// Removes the first occurrence of the specified pair from the /// collection. /// /// /// The pair to be removed. /// ////// Always, because the collection is read-only. /// public bool Remove( KeyValuePairpair ) { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } #endregion ICollection > Methods //----------------------------------------------------- // IDictionary Methods //----------------------------------------------------- #region IDictionary Methods /// /// Adds an entry with the specified key ( ///) and value /// ( ) to the dictionary. /// /// Always, because the dictionary is read-only. /// public void Add(K key, V value) { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } ////// Determines whether the dictionary contains entry fo the specified key. /// ////// true if the dictionary contains an entry for the specified user, otherwise false. /// public bool ContainsKey(K key) { return _dict.ContainsKey(key); } ////// Remove the entry with the specified key from the dictionary. /// ////// true if the element is successfully removed; otherwise, false. This method also returns false /// if key was not found in the dictionary. /// ////// Always, because the dictionary is read-only. /// public bool Remove(K key) { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } ////// Retrieve the entry associated with the specified key. /// ////// true if the dictionary contains an entry for the specified key; /// otherwise false. /// public bool TryGetValue(K key, out V value) { return _dict.TryGetValue(key, out value); } #endregion IDictionaryMethods //----------------------------------------------------- // IEnumerable Methods //------------------------------------------------------ #region IEnumerable Methods /// /// Returns an enumerator that iterates through the collection. /// IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable)_dict).GetEnumerator(); } #endregion IEnumerable Methods //----------------------------------------------------- // ICollection Methods //------------------------------------------------------ #region ICollection Methods ////// Copies the elements of the collection to an array, starting at the specified /// array index. /// public void CopyTo(Array array, int index ) { ((ICollection)_dict).CopyTo(array, index); } #endregion ICollection Methods //------------------------------------------------------ // IDictionary Methods //----------------------------------------------------- #region IDictionary Methods ////// Adds an element with the specified key and value to the dictionary. /// ////// Always, because the dictionary is read-only. /// public void Add(object key, object value) { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } ////// Determines whether the dictionary contains an element with the specified key. /// public bool Contains(object key) { return ((IDictionary)_dict).Contains(key); } ////// Returns an IDictionaryEnumerator for the dictionary. /// IDictionaryEnumerator IDictionary.GetEnumerator() { return ((IDictionary)_dict).GetEnumerator(); } ////// Removes the element with the specified key from the dictionary. /// ////// Always, because the dictionary is read-only. /// public void Remove(object key) { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } #endregion IDictionary Methods #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties //----------------------------------------------------- // ICollection> Properties //----------------------------------------------------- #region ICollection > Properties /// /// Returns the number of elements in the collection. /// public int Count { get { return _dict.Count; } } ////// Gets a value indicating whether the dictionary is read-only. /// public bool IsReadOnly { get { return true; } } #endregion ICollection> Properties //------------------------------------------------------ // IDictionary Properties //----------------------------------------------------- #region IDictionary Properties /// /// Gets the use license associated with the user specified by public V this[K key] { get { return _dict[key]; } set { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } } ///. /// /// Returns an ICollection containing the keys of the dictionary. /// public ICollectionKeys { get { return ((IDictionary )_dict).Keys; } } /// /// Returns an ICollection containing the values in the dictionary. /// public ICollectionValues { get { return ((IDictionary )_dict).Values; } } #endregion IDictionary Properties //------------------------------------------------------ // ICollection Properties //------------------------------------------------------ #region ICollection Properties public bool IsSynchronized { get { return ((ICollection)_dict).IsSynchronized; } } /// /// Gets an object that can be used to synchronize access to the collection. /// public object SyncRoot { get { return ((ICollection)_dict).SyncRoot; } } #endregion ICollection Properties //----------------------------------------------------- // IDictionary Properties //------------------------------------------------------ #region IDictionary Properties ////// Gets a value indicating whether the dictionary has a fixed size. /// public bool IsFixedSize { get { return true; } } ////// Returns an ICollection containing the keys of the dictionary. /// ICollection IDictionary.Keys { get { return ((IDictionary)_dict).Keys; } } ////// Returns an ICollection containing the values in the dictionary. /// ICollection IDictionary.Values { get { return ((IDictionary)_dict).Values; } } ////// Gets the value associated with the specified key>. /// public object this[object key] { get { return ((IDictionary)_dict)[key]; } set { throw new NotSupportedException(SR.Get(SRID.DictionaryIsReadOnly)); } } #endregion IDictionary Properties #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields // // The object that provides the implementation of the IDictionary methods. // private Dictionary_dict; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
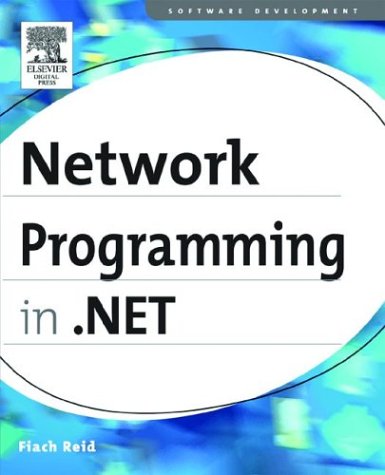
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GiveFeedbackEvent.cs
- AutoScrollHelper.cs
- MissingFieldException.cs
- PriorityRange.cs
- FakeModelItemImpl.cs
- DoubleConverter.cs
- DataBindingCollectionEditor.cs
- CustomCategoryAttribute.cs
- Form.cs
- DecoratedNameAttribute.cs
- FixedDocument.cs
- DATA_BLOB.cs
- CryptoConfig.cs
- CodeVariableReferenceExpression.cs
- AssemblyResourceLoader.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- dsa.cs
- ProfileWorkflowElement.cs
- BuildProvider.cs
- X509RawDataKeyIdentifierClause.cs
- XmlParserContext.cs
- XmlCharCheckingReader.cs
- TPLETWProvider.cs
- ToolStripOverflowButton.cs
- DependencyPropertyAttribute.cs
- ModulesEntry.cs
- ExtentCqlBlock.cs
- AssemblyBuilder.cs
- VersionConverter.cs
- EventLogEntry.cs
- ClockGroup.cs
- PanelStyle.cs
- PackageStore.cs
- SettingsAttributes.cs
- StretchValidation.cs
- UserUseLicenseDictionaryLoader.cs
- HttpResponseHeader.cs
- CompositeScriptReference.cs
- ICspAsymmetricAlgorithm.cs
- SharedConnectionWorkflowTransactionService.cs
- Content.cs
- OracleInternalConnection.cs
- StylusTip.cs
- EventData.cs
- ConstraintConverter.cs
- ServiceDescriptionContext.cs
- MustUnderstandSoapException.cs
- XPathNavigatorKeyComparer.cs
- FaultImportOptions.cs
- SqlSelectClauseBuilder.cs
- SystemDiagnosticsSection.cs
- SoapWriter.cs
- FontWeightConverter.cs
- SystemSounds.cs
- SQLInt32Storage.cs
- InsufficientMemoryException.cs
- ConfigPathUtility.cs
- path.cs
- StringFormat.cs
- EditableLabelControl.cs
- BaseParaClient.cs
- Int16AnimationUsingKeyFrames.cs
- TypeCollectionPropertyEditor.cs
- RegexNode.cs
- SqlServer2KCompatibilityAnnotation.cs
- GenericArgumentsUpdater.cs
- DesignSurfaceEvent.cs
- DispatcherExceptionFilterEventArgs.cs
- DoubleAnimationUsingPath.cs
- Button.cs
- documentsequencetextcontainer.cs
- CircleEase.cs
- ADMembershipProvider.cs
- ParseElementCollection.cs
- Vector3DIndependentAnimationStorage.cs
- OdbcCommand.cs
- RenderDataDrawingContext.cs
- ContentElement.cs
- Subtree.cs
- StringWriter.cs
- XmlWriterSettings.cs
- LinkedResourceCollection.cs
- TdsParserHelperClasses.cs
- XomlCompilerResults.cs
- ProcessManager.cs
- WasAdminWrapper.cs
- SortKey.cs
- DirectoryRedirect.cs
- ThemeableAttribute.cs
- CacheEntry.cs
- TabItemWrapperAutomationPeer.cs
- VisualStyleTypesAndProperties.cs
- XmlQueryRuntime.cs
- XmlEncoding.cs
- Unit.cs
- Timer.cs
- ToolBarOverflowPanel.cs
- BitmapEffectGroup.cs
- PerfCounters.cs
- EventMappingSettingsCollection.cs