Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Imaging / UnmanagedBitmapWrapper.cs / 1305600 / UnmanagedBitmapWrapper.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation. All Rights Reserved. // // File: UnmanagedBitmapWrapper.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Imaging { internal sealed class UnmanagedBitmapWrapper : BitmapSource { ////// Critical - calls critical code method BitmapSource.UpdateCachedSettings /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] public UnmanagedBitmapWrapper(BitmapSourceSafeMILHandle bitmapSource) : base(true) { _bitmapInit.BeginInit(); // // This constructor is used by BitmapDecoder and BitmapFrameDecode for thumbnails and // previews. The bitmapSource parameter comes from BitmapSource.CreateCachedBitmap // which already calculated memory pressure, so there's no need to do it here. // WicSourceHandle = bitmapSource; _bitmapInit.EndInit(); UpdateCachedSettings(); } #region Protected Methods ////// Critical - eventually access'es critical resources (_wicSource) /// TreatAsSafe - all inputs are checked /// [SecurityCritical, SecurityTreatAsSafe] internal UnmanagedBitmapWrapper(bool initialize) : base(true) { // Call BeginInit and EndInit if initialize is true. if (initialize) { _bitmapInit.BeginInit(); _bitmapInit.EndInit(); } } ////// Implementation of protected override Freezable CreateInstanceCore() { return new UnmanagedBitmapWrapper(false); } private void CopyCommon(UnmanagedBitmapWrapper sourceBitmap) { _bitmapInit.BeginInit(); _bitmapInit.EndInit(); } ///Freezable.CreateInstanceCore . ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { UnmanagedBitmapWrapper sourceBitmap = (UnmanagedBitmapWrapper)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(sourceBitmap); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Freezable.GetCurrentValueAsFrozenCore . ///
Link Menu
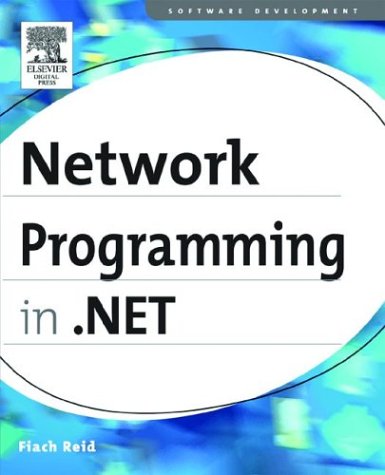
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListView.cs
- DesignerTransactionCloseEvent.cs
- BitmapFrameEncode.cs
- WebMessageEncoderFactory.cs
- ParallelEnumerable.cs
- SamlConstants.cs
- DesigntimeLicenseContextSerializer.cs
- ToolStripItemCollection.cs
- Serializer.cs
- Material.cs
- MonthCalendarDesigner.cs
- IApplicationTrustManager.cs
- LabelDesigner.cs
- CodeExpressionCollection.cs
- XmlSerializerVersionAttribute.cs
- TextDecoration.cs
- LongMinMaxAggregationOperator.cs
- Trace.cs
- RawStylusInputCustomDataList.cs
- followingsibling.cs
- WmpBitmapDecoder.cs
- LoginName.cs
- ContextMenu.cs
- DetailsViewInsertEventArgs.cs
- CodeGotoStatement.cs
- GenericAuthenticationEventArgs.cs
- ScaleTransform.cs
- _RequestCacheProtocol.cs
- FileVersionInfo.cs
- DotExpr.cs
- ProtocolsConfigurationEntry.cs
- AssemblyUtil.cs
- LogicalExpressionEditor.cs
- DataGridViewCellValueEventArgs.cs
- TextWriterTraceListener.cs
- ActivityMetadata.cs
- AttributeProviderAttribute.cs
- Span.cs
- ColumnClickEvent.cs
- JsonFaultDetail.cs
- CheckableControlBaseAdapter.cs
- XmlCharacterData.cs
- ValuePattern.cs
- LinqDataSourceInsertEventArgs.cs
- TraceLog.cs
- SBCSCodePageEncoding.cs
- SafeThreadHandle.cs
- DecoderBestFitFallback.cs
- CompositeKey.cs
- oledbmetadatacolumnnames.cs
- ConfigurationManagerInternal.cs
- ForeignConstraint.cs
- ConfigurationFileMap.cs
- JapaneseCalendar.cs
- AdornerDecorator.cs
- PreProcessor.cs
- PipeStream.cs
- ChannelTraceRecord.cs
- Oid.cs
- PriorityItem.cs
- LineUtil.cs
- ExtendedProperty.cs
- SortDescription.cs
- AppDomainInfo.cs
- DependencyStoreSurrogate.cs
- MediaCommands.cs
- MetaType.cs
- Bezier.cs
- MouseEventArgs.cs
- TextServicesLoader.cs
- Queue.cs
- Invariant.cs
- XsltLoader.cs
- HttpAsyncResult.cs
- Typography.cs
- TopClause.cs
- FormViewCommandEventArgs.cs
- FileSystemEventArgs.cs
- ButtonBaseDesigner.cs
- EntitySqlQueryBuilder.cs
- TableLayoutCellPaintEventArgs.cs
- CompositeActivityTypeDescriptorProvider.cs
- HttpException.cs
- ImageConverter.cs
- NotifyInputEventArgs.cs
- ListViewTableRow.cs
- HashAlgorithm.cs
- AsyncPostBackErrorEventArgs.cs
- WindowsScrollBarBits.cs
- HtmlShimManager.cs
- MetaForeignKeyColumn.cs
- PagedDataSource.cs
- PersonalizationAdministration.cs
- EventlogProvider.cs
- TextElementCollection.cs
- SqlParameterCollection.cs
- ObjectAssociationEndMapping.cs
- ToolBarPanel.cs
- WebPartHeaderCloseVerb.cs
- KoreanCalendar.cs