Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / Material.cs / 1 / Material.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { abstract partial class Material : Animatable, IFormattable, DUCE.IResource { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Material Clone() { return (Material)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Material CloneCurrentValue() { return (Material)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal abstract DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel); ////// AddRefOnChannel /// DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { return AddRefOnChannelCore(channel); } } internal abstract void ReleaseOnChannelCore(DUCE.Channel channel); ////// ReleaseOnChannel /// void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { ReleaseOnChannelCore(channel); } } internal abstract DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel); ////// GetHandle /// DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle handle; using (CompositionEngineLock.Acquire()) { handle = GetHandleCore(channel); } return handle; } internal abstract int GetChannelCountCore(); ////// GetChannelCount /// int DUCE.IResource.GetChannelCount() { // must already be in composition lock here return GetChannelCountCore(); } internal abstract DUCE.Channel GetChannelCore(int index); ////// GetChannel /// DUCE.Channel DUCE.IResource.GetChannel(int index) { // must already be in composition lock here return GetChannelCore(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal virtual string ConvertToString(string format, IFormatProvider provider) { return base.ToString(); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { abstract partial class Material : Animatable, IFormattable, DUCE.IResource { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new Material Clone() { return (Material)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new Material CloneCurrentValue() { return (Material)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal abstract DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel); ////// AddRefOnChannel /// DUCE.ResourceHandle DUCE.IResource.AddRefOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { return AddRefOnChannelCore(channel); } } internal abstract void ReleaseOnChannelCore(DUCE.Channel channel); ////// ReleaseOnChannel /// void DUCE.IResource.ReleaseOnChannel(DUCE.Channel channel) { // Reconsider the need for this lock when removing the MultiChannelResource. using (CompositionEngineLock.Acquire()) { ReleaseOnChannelCore(channel); } } internal abstract DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel); ////// GetHandle /// DUCE.ResourceHandle DUCE.IResource.GetHandle(DUCE.Channel channel) { DUCE.ResourceHandle handle; using (CompositionEngineLock.Acquire()) { handle = GetHandleCore(channel); } return handle; } internal abstract int GetChannelCountCore(); ////// GetChannelCount /// int DUCE.IResource.GetChannelCount() { // must already be in composition lock here return GetChannelCountCore(); } internal abstract DUCE.Channel GetChannelCore(int index); ////// GetChannel /// DUCE.Channel DUCE.IResource.GetChannel(int index) { // must already be in composition lock here return GetChannelCore(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { ReadPreamble(); // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal virtual string ConvertToString(string format, IFormatProvider provider) { return base.ToString(); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
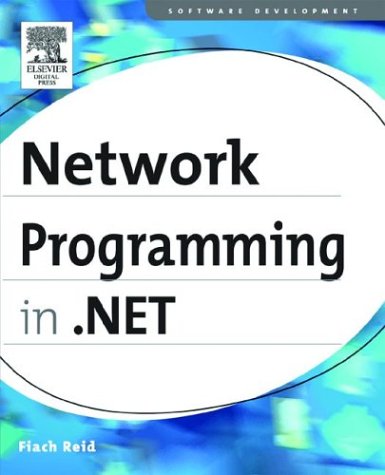
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionHelper.cs
- XmlAttributeCollection.cs
- TypedReference.cs
- HttpCookieCollection.cs
- PeerReferralPolicy.cs
- WindowsGrip.cs
- ImageFormat.cs
- HttpVersion.cs
- PasswordTextNavigator.cs
- XslTransform.cs
- HandledEventArgs.cs
- PersonalizableTypeEntry.cs
- SqlWebEventProvider.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- HttpListenerResponse.cs
- SoapDocumentServiceAttribute.cs
- ColumnMapCopier.cs
- FileDataSourceCache.cs
- ExtensionSimplifierMarkupObject.cs
- SqlDataSourceStatusEventArgs.cs
- XmlSchemaSubstitutionGroup.cs
- DataGridViewRowCollection.cs
- ToolboxItemCollection.cs
- SamlAudienceRestrictionCondition.cs
- InsufficientMemoryException.cs
- FixedSOMGroup.cs
- EdmType.cs
- AssemblyHash.cs
- FormsIdentity.cs
- UrlUtility.cs
- Debug.cs
- InkCollectionBehavior.cs
- NegationPusher.cs
- Optimizer.cs
- CacheSection.cs
- ArrayList.cs
- AssemblyName.cs
- OperationCanceledException.cs
- GreaterThanOrEqual.cs
- GridViewRowCollection.cs
- _BaseOverlappedAsyncResult.cs
- UidManager.cs
- ResourcePool.cs
- ObjectPropertyMapping.cs
- ImageIndexEditor.cs
- TextElementCollectionHelper.cs
- SystemFonts.cs
- RuntimeHandles.cs
- SqlFunctionAttribute.cs
- OdbcDataReader.cs
- IRCollection.cs
- LayeredChannelFactory.cs
- SEHException.cs
- Console.cs
- BinaryFormatterWriter.cs
- ListItemCollection.cs
- ServiceErrorHandler.cs
- FunctionUpdateCommand.cs
- WebPartZoneCollection.cs
- HtmlTitle.cs
- CompositeActivityMarkupSerializer.cs
- ToolStripTextBox.cs
- UpdateTracker.cs
- DataGridParentRows.cs
- AttachedAnnotation.cs
- ProxyWebPartConnectionCollection.cs
- AddIn.cs
- DataObjectPastingEventArgs.cs
- VisualProxy.cs
- WindowsListViewScroll.cs
- WindowsFormsSynchronizationContext.cs
- Matrix3D.cs
- GPPOINTF.cs
- RenderCapability.cs
- DynamicExpression.cs
- DeleteMemberBinder.cs
- ClientSponsor.cs
- LinqDataSourceEditData.cs
- NoneExcludedImageIndexConverter.cs
- XmlObjectSerializer.cs
- PermissionAttributes.cs
- ResourceSet.cs
- NameValueFileSectionHandler.cs
- RtfToXamlReader.cs
- TypeValidationEventArgs.cs
- __FastResourceComparer.cs
- PriorityBinding.cs
- TypeConverter.cs
- EntityDataSourceContextCreatingEventArgs.cs
- ToolStripDropTargetManager.cs
- IFlowDocumentViewer.cs
- SignatureHelper.cs
- StorageConditionPropertyMapping.cs
- DocumentReferenceCollection.cs
- EditorZoneAutoFormat.cs
- DocumentPageHost.cs
- ProfileManager.cs
- ToolBarOverflowPanel.cs
- CheckBoxBaseAdapter.cs
- NegationPusher.cs