Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Command / ApplicationCommands.cs / 2 / ApplicationCommands.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The ApplicationCommands class defines a standard set of commands that are required in most applications. // The goal of these commands is to unify input, programming model and UI for the most common actions in // Windows applications thus providing a standard interface for such common commands. // // See spec at : [....]/CoreUI/Specs%20%20Eventing%20and%20Commanding/CommandLibrarySpec.mht // // // History: // 03/31/2004 : [....] - Created // 04/28/2004 : Added Accelerator table loading from Resource // 02/02/2005 : Created ApplicationCommands class from CommandLibrary class. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Collections; using System.ComponentModel; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using MS.Internal; // CommandHelper namespace System.Windows.Input { ////// ApplicationCommands - Set of Standard Commands /// public static class ApplicationCommands { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// CutCommand - action to cut selection /// public static RoutedUICommand Cut { get { return _EnsureCommand(CommandId.Cut); } } ////// CopyCommand /// public static RoutedUICommand Copy { get { return _EnsureCommand(CommandId.Copy); } } ////// PasteCommand /// public static RoutedUICommand Paste { get { return _EnsureCommand(CommandId.Paste); } } ////// DeleteCommand /// public static RoutedUICommand Delete { get { return _EnsureCommand(CommandId.Delete); } } ////// UndoCommand /// public static RoutedUICommand Undo { get { return _EnsureCommand(CommandId.Undo); } } ////// RedoCommand /// public static RoutedUICommand Redo { get { return _EnsureCommand(CommandId.Redo); } } ////// SelectAllCommand /// public static RoutedUICommand Find { get { return _EnsureCommand(CommandId.Find); } } ////// ReplaceCommand /// public static RoutedUICommand Replace { get { return _EnsureCommand(CommandId.Replace); } } ////// SelectAllCommand /// public static RoutedUICommand SelectAll { get { return _EnsureCommand(CommandId.SelectAll); } } ////// HelpCommand /// public static RoutedUICommand Help { get { return _EnsureCommand(CommandId.Help); } } ////// NewCommand /// public static RoutedUICommand New { get { return _EnsureCommand(CommandId.New); } } ////// OpenCommand /// public static RoutedUICommand Open { get { return _EnsureCommand(CommandId.Open); } } ////// CloseCommand /// public static RoutedUICommand Close { get { return _EnsureCommand(CommandId.Close); } } ////// SaveCommand /// public static RoutedUICommand Save { get { return _EnsureCommand(CommandId.Save); } } ////// SaveAsCommand /// public static RoutedUICommand SaveAs { get { return _EnsureCommand(CommandId.SaveAs); } } ////// PrintCommand /// public static RoutedUICommand Print { get { return _EnsureCommand(CommandId.Print); } } ////// CancelPrintCommand /// public static RoutedUICommand CancelPrint { get { return _EnsureCommand(CommandId.CancelPrint); } } ////// PrintPreviewCommand /// public static RoutedUICommand PrintPreview { get { return _EnsureCommand(CommandId.PrintPreview); } } ////// PropertiesCommand /// public static RoutedUICommand Properties { get { return _EnsureCommand(CommandId.Properties); } } ////// ContextMenuCommand /// public static RoutedUICommand ContextMenu { get { return _EnsureCommand(CommandId.ContextMenu); } } ////// StopCommand /// public static RoutedUICommand Stop { get { return _EnsureCommand(CommandId.Stop); } } ////// CorrectionListCommand /// public static RoutedUICommand CorrectionList { get { return _EnsureCommand(CommandId.CorrectionList); } } ////// NotACommand command. /// ////// This "command" is always ignored, without handling the input event /// that caused it. This provides a way to turn off an input binding /// built into an existing control. /// public static RoutedUICommand NotACommand { get { return _EnsureCommand(CommandId.NotACommand); } } #endregion Public Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Critical - determines the permission to be applied for a command /// TreatAsSafe - callers don't need to worry, but we need to review /// any changes to this. /// [SecurityCritical, SecurityTreatAsSafe] private static PermissionSet GetRequiredPermissions(CommandId commandId) { PermissionSet permissions; switch (commandId) { case CommandId.Paste: permissions = new PermissionSet(PermissionState.None); permissions.AddPermission(new UIPermission(UIPermissionClipboard.AllClipboard)); break; default: permissions = null; break; } return permissions; } private static string GetPropertyName(CommandId commandId) { string propertyName = String.Empty; switch (commandId) { case CommandId.Cut: propertyName = "Cut"; break; case CommandId.Copy: propertyName = "Copy"; break; case CommandId.Paste: propertyName = "Paste"; break; case CommandId.Undo: propertyName = "Undo"; break; case CommandId.Redo: propertyName = "Redo"; break; case CommandId.Delete: propertyName = "Delete"; break; case CommandId.Find: propertyName = "Find"; break; case CommandId.Replace: propertyName = "Replace"; break; case CommandId.Help: propertyName = "Help"; break; case CommandId.New: propertyName = "New"; break; case CommandId.Open: propertyName = "Open"; break; case CommandId.Save: propertyName = "Save"; break; case CommandId.SaveAs: propertyName = "SaveAs"; break; case CommandId.Close: propertyName = "Close"; break; case CommandId.Print: propertyName = "Print"; break; case CommandId.CancelPrint: propertyName = "CancelPrint"; break; case CommandId.PrintPreview: propertyName = "PrintPreview"; break; case CommandId.Properties: propertyName = "Properties"; break; case CommandId.ContextMenu: propertyName = "ContextMenu"; break; case CommandId.CorrectionList: propertyName = "CorrectionList"; break; case CommandId.SelectAll: propertyName = "SelectAll"; break; case CommandId.Stop: propertyName = "Stop"; break; case CommandId.NotACommand: propertyName = "NotACommand"; break; } return propertyName; } internal static string GetUIText(byte commandId) { string uiText = String.Empty; switch ((CommandId)commandId) { case CommandId.Cut: uiText = SR.Get(SRID.CutText); break; case CommandId.Copy: uiText = SR.Get(SRID.CopyText);break; case CommandId.Paste: uiText = SR.Get(SRID.PasteText);break; case CommandId.Undo: uiText = SR.Get(SRID.UndoText);break; case CommandId.Redo: uiText = SR.Get(SRID.RedoText); break; case CommandId.Delete: uiText = SR.Get(SRID.DeleteText); break; case CommandId.Find: uiText = SR.Get(SRID.FindText); break; case CommandId.Replace: uiText = SR.Get(SRID.ReplaceText); break; case CommandId.SelectAll: uiText = SR.Get(SRID.SelectAllText); break; case CommandId.Help: uiText = SR.Get(SRID.HelpText); break; case CommandId.New: uiText = SR.Get(SRID.NewText); break; case CommandId.Open: uiText = SR.Get(SRID.OpenText); break; case CommandId.Save: uiText = SR.Get(SRID.SaveText); break; case CommandId.SaveAs: uiText = SR.Get(SRID.SaveAsText); break; case CommandId.Print: uiText = SR.Get(SRID.PrintText); break; case CommandId.CancelPrint: uiText = SR.Get(SRID.CancelPrintText); break; case CommandId.PrintPreview: uiText = SR.Get(SRID.PrintPreviewText); break; case CommandId.Close: uiText = SR.Get(SRID.CloseText); break; case CommandId.ContextMenu: uiText = SR.Get(SRID.ContextMenuText); break; case CommandId.CorrectionList: uiText = SR.Get(SRID.CorrectionListText); break; case CommandId.Properties: uiText = SR.Get(SRID.PropertiesText); break; case CommandId.Stop: uiText = SR.Get(SRID.StopText); break; case CommandId.NotACommand: uiText = SR.Get(SRID.NotACommandText); break; } return uiText; } internal static InputGestureCollection LoadDefaultGestureFromResource(byte commandId) { InputGestureCollection gestures = new InputGestureCollection(); //Standard Commands switch ((CommandId)commandId) { case CommandId.Cut: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.CutKey), SR.Get(SRID.CutKeyDisplayString), gestures); break; case CommandId.Copy: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.CopyKey), SR.Get(SRID.CopyKeyDisplayString), gestures); break; case CommandId.Paste: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.PasteKey), SR.Get(SRID.PasteKeyDisplayString), gestures); break; case CommandId.Undo: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.UndoKey), SR.Get(SRID.UndoKeyDisplayString), gestures); break; case CommandId.Redo: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.RedoKey), SR.Get(SRID.RedoKeyDisplayString), gestures); break; case CommandId.Delete: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.DeleteKey), SR.Get(SRID.DeleteKeyDisplayString), gestures); break; case CommandId.Find: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.FindKey), SR.Get(SRID.FindKeyDisplayString), gestures); break; case CommandId.Replace: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.ReplaceKey), SR.Get(SRID.ReplaceKeyDisplayString), gestures); break; case CommandId.SelectAll: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.SelectAllKey), SR.Get(SRID.SelectAllKeyDisplayString), gestures); break; case CommandId.Help: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.HelpKey), SR.Get(SRID.HelpKeyDisplayString), gestures); break; case CommandId.New: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.NewKey), SR.Get(SRID.NewKeyDisplayString), gestures); break; case CommandId.Open: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.OpenKey), SR.Get(SRID.OpenKeyDisplayString), gestures); break; case CommandId.Save: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.SaveKey), SR.Get(SRID.SaveKeyDisplayString), gestures); break; case CommandId.SaveAs: break; // there are no default bindings for CommandId.SaveAs case CommandId.Print: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.PrintKey), SR.Get(SRID.PrintKeyDisplayString), gestures); break; case CommandId.CancelPrint: break; // there are no default bindings for CommandId.CancelPrint case CommandId.PrintPreview: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.PrintPreviewKey), SR.Get(SRID.PrintPreviewKeyDisplayString), gestures); break; case CommandId.Close: break; // there are no default bindings for CommandId.Close case CommandId.ContextMenu: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.ContextMenuKey), SR.Get(SRID.ContextMenuKeyDisplayString), gestures); break; case CommandId.CorrectionList: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.CorrectionListKey), SR.Get(SRID.CorrectionListKeyDisplayString), gestures); break; case CommandId.Properties: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.PropertiesKey), SR.Get(SRID.PropertiesKeyDisplayString), gestures); break; case CommandId.Stop: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.StopKey), SR.Get(SRID.StopKeyDisplayString), gestures); break; case CommandId.NotACommand: break; // there are no default bindings for CommandId.NotACommand } return gestures; } private static RoutedUICommand _EnsureCommand(CommandId idCommand) { if (idCommand >= 0 && idCommand < CommandId.Last) { lock (_internalCommands.SyncRoot) { if (_internalCommands[(int)idCommand] == null) { RoutedUICommand newCommand = CommandLibraryHelper.CreateUICommand( GetPropertyName(idCommand), typeof(ApplicationCommands), (byte)idCommand, GetRequiredPermissions(idCommand)); _internalCommands[(int)idCommand] = newCommand; } } return _internalCommands[(int)idCommand]; } return null; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // these constants will go away in future, its just to index into the right one. private enum CommandId : byte { Cut=0, Copy=1, Paste=2, Undo=3, Redo=4, Delete=5, Find=6, Replace=7, Help=8, SelectAll=9, New=10, Open=11, Save=12, SaveAs=13, Print = 14, CancelPrint = 15, PrintPreview = 16, Close = 17, Properties=18, ContextMenu=19, CorrectionList=20, Stop=21, NotACommand=22, // Last Last=23 } private static RoutedUICommand[] _internalCommands = new RoutedUICommand[(int)CommandId.Last]; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
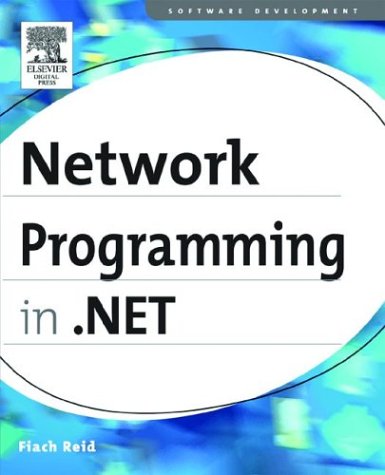
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConditionalAttribute.cs
- StateWorkerRequest.cs
- EntityDataSourceValidationException.cs
- ComAdminWrapper.cs
- SqlDependencyUtils.cs
- ScopedMessagePartSpecification.cs
- ManagementOptions.cs
- ListViewUpdatedEventArgs.cs
- InfoCardArgumentException.cs
- ValidatingReaderNodeData.cs
- ControlBuilderAttribute.cs
- Icon.cs
- HttpHandlerAction.cs
- TextLineBreak.cs
- LowerCaseStringConverter.cs
- LocalizabilityAttribute.cs
- HandledEventArgs.cs
- Inline.cs
- DataGridViewCell.cs
- CollectionViewGroupInternal.cs
- Root.cs
- SmiEventSink_Default.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- TriState.cs
- ValidationPropertyAttribute.cs
- DocumentViewer.cs
- ZipPackagePart.cs
- RangeValidator.cs
- ObjectParameterCollection.cs
- NgenServicingAttributes.cs
- FileCodeGroup.cs
- DiagnosticTraceSource.cs
- ButtonBaseAdapter.cs
- InvalidMessageContractException.cs
- SqlProviderManifest.cs
- XmlTextEncoder.cs
- AdRotatorDesigner.cs
- HttpModuleActionCollection.cs
- TableCellCollection.cs
- ResXDataNode.cs
- AssemblyInfo.cs
- WsatEtwTraceListener.cs
- Stacktrace.cs
- MissingFieldException.cs
- SafeRightsManagementSessionHandle.cs
- LicenseProviderAttribute.cs
- URLString.cs
- GeneralTransform.cs
- Rect.cs
- HostElement.cs
- Encoding.cs
- InputEventArgs.cs
- ZoneButton.cs
- DataGridRowAutomationPeer.cs
- TransformerInfoCollection.cs
- ArrangedElementCollection.cs
- BreadCrumbTextConverter.cs
- ModuleConfigurationInfo.cs
- DesignTimeVisibleAttribute.cs
- FixedSOMPageElement.cs
- DecimalConstantAttribute.cs
- ScrollBarRenderer.cs
- WebRequestModulesSection.cs
- Blend.cs
- SessionStateModule.cs
- ConfigurationElementCollection.cs
- VirtualPathProvider.cs
- ConfigurationStrings.cs
- ToolboxComponentsCreatingEventArgs.cs
- ItemList.cs
- JapaneseLunisolarCalendar.cs
- ParameterEditorUserControl.cs
- ContextActivityUtils.cs
- ToolStripItemImageRenderEventArgs.cs
- AsyncSerializedWorker.cs
- WebPart.cs
- Pair.cs
- NameValueCollection.cs
- RenderOptions.cs
- PageThemeParser.cs
- ObjectIDGenerator.cs
- ScrollChrome.cs
- WorkItem.cs
- StackSpiller.Bindings.cs
- ColumnResizeAdorner.cs
- StrokeSerializer.cs
- RequestNavigateEventArgs.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ExecutionContext.cs
- QuaternionRotation3D.cs
- EntitySetBaseCollection.cs
- SaveFileDialog.cs
- DeploymentExceptionMapper.cs
- BindingList.cs
- ClientEventManager.cs
- SortExpressionBuilder.cs
- TimeStampChecker.cs
- BrowserDefinitionCollection.cs
- StreamSecurityUpgradeAcceptorBase.cs
- odbcmetadatacollectionnames.cs