Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Imaging / InplaceBitmapMetadataWriter.cs / 1 / InplaceBitmapMetadataWriter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved // // File: InPlaceBitmapMetadataWriter.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using MS.Win32.PresentationCore; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.Windows.Media.Imaging; using System.Windows.Threading; using System.Text; using MS.Internal.PresentationCore; // SecurityHelper namespace System.Windows.Media.Imaging { #region InPlaceBitmapMetadataWriter ////// Metadata Class for BitmapImage. /// sealed public partial class InPlaceBitmapMetadataWriter : BitmapMetadata { #region Constructors ////// /// private InPlaceBitmapMetadataWriter() { } ////// /// ////// Critical - Accesses critical resources /// [SecurityCritical] internal InPlaceBitmapMetadataWriter( SafeMILHandle /* IWICFastMetadataEncoder */ fmeHandle, SafeMILHandle /* IWICMetadataQueryWriter */ metadataHandle, object syncObject ) : base(metadataHandle, false, false, syncObject) { _fmeHandle = fmeHandle; } ////// /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] static internal InPlaceBitmapMetadataWriter CreateFromFrameDecode(BitmapSourceSafeMILHandle frameHandle, object syncObject) { Invariant.Assert(frameHandle != null); SafeMILHandle /* IWICFastMetadataEncoder */ fmeHandle = null; SafeMILHandle /* IWICMetadataQueryWriter */ metadataHandle = null; using (FactoryMaker factoryMaker = new FactoryMaker()) { lock (syncObject) { HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateFastMetadataEncoderFromFrameDecode( factoryMaker.ImagingFactoryPtr, frameHandle, out fmeHandle)); } } HRESULT.Check(UnsafeNativeMethods.WICFastMetadataEncoder.GetMetadataQueryWriter( fmeHandle, out metadataHandle)); return new InPlaceBitmapMetadataWriter(fmeHandle, metadataHandle, syncObject); } ////// /// ////// Critical - Accesses unmanaged code /// TreatAsSafe - inputs are verified or safe /// [SecurityCritical, SecurityTreatAsSafe] static internal InPlaceBitmapMetadataWriter CreateFromDecoder(SafeMILHandle decoderHandle, object syncObject) { Invariant.Assert(decoderHandle != null); SafeMILHandle /* IWICFastMetadataEncoder */ fmeHandle = null; SafeMILHandle /* IWICMetadataQueryWriter */ metadataHandle = null; using (FactoryMaker factoryMaker = new FactoryMaker()) { lock (syncObject) { HRESULT.Check(UnsafeNativeMethods.WICImagingFactory.CreateFastMetadataEncoderFromDecoder( factoryMaker.ImagingFactoryPtr, decoderHandle, out fmeHandle)); } } HRESULT.Check(UnsafeNativeMethods.WICFastMetadataEncoder.GetMetadataQueryWriter( fmeHandle, out metadataHandle)); return new InPlaceBitmapMetadataWriter(fmeHandle, metadataHandle, syncObject); } ////// /// ////// Critical - Accesses unmanaged code /// PublicOK - inputs are verified or safe /// [SecurityCritical ] public bool TrySave() { int hr; Invariant.Assert(_fmeHandle != null); lock (SyncObject) { hr = UnsafeNativeMethods.WICFastMetadataEncoder.Commit(_fmeHandle); } return HRESULT.Succeeded(hr); } #endregion #region Freezable ////// Shadows inherited Copy() with a strongly typed /// version for convenience. /// public new InPlaceBitmapMetadataWriter Clone() { return (InPlaceBitmapMetadataWriter)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { throw new InvalidOperationException(SR.Get(SRID.Image_InplaceMetadataNoCopy)); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { throw new InvalidOperationException(SR.Get(SRID.Image_InplaceMetadataNoCopy)); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { throw new InvalidOperationException(SR.Get(SRID.Image_InplaceMetadataNoCopy)); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { throw new InvalidOperationException(SR.Get(SRID.Image_InplaceMetadataNoCopy)); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { throw new InvalidOperationException(SR.Get(SRID.Image_InplaceMetadataNoCopy)); } #endregion ///Freezable.GetCurrentValueAsFrozenCore . ////// Critical - pointer to an unmanaged object that methods are called on. /// [SecurityCritical] private SafeMILHandle _fmeHandle; } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
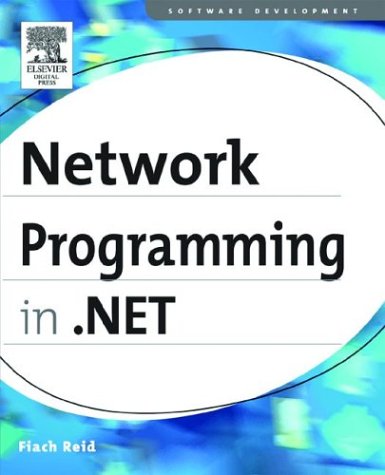
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsGraphics.cs
- Base64Decoder.cs
- Line.cs
- HostedTcpTransportManager.cs
- SystemNetworkInterface.cs
- ChtmlSelectionListAdapter.cs
- SafeRightsManagementPubHandle.cs
- BamlBinaryReader.cs
- XmlDataSourceView.cs
- FontFamily.cs
- ObjectDesignerDataSourceView.cs
- XmlCollation.cs
- CollectionType.cs
- ConfigUtil.cs
- DelegateTypeInfo.cs
- HTMLTagNameToTypeMapper.cs
- BamlWriter.cs
- DataGridViewHitTestInfo.cs
- CounterSampleCalculator.cs
- ApplyImportsAction.cs
- DeviceSpecificChoice.cs
- Metafile.cs
- Array.cs
- DateTimeConverter.cs
- TitleStyle.cs
- UIntPtr.cs
- BaseDataListComponentEditor.cs
- RowParagraph.cs
- HostUtils.cs
- Dynamic.cs
- ConfigurationManagerHelperFactory.cs
- HttpStreamXmlDictionaryReader.cs
- ResourcePermissionBase.cs
- basemetadatamappingvisitor.cs
- NewArrayExpression.cs
- StoreUtilities.cs
- ToolStripMenuItemCodeDomSerializer.cs
- HttpAsyncResult.cs
- WebPartDisplayModeEventArgs.cs
- BackgroundWorker.cs
- NotifyCollectionChangedEventArgs.cs
- ClientSettingsProvider.cs
- LeafCellTreeNode.cs
- SelectionRange.cs
- BitmapFrameDecode.cs
- EndpointConfigContainer.cs
- ManagedFilter.cs
- PathFigureCollection.cs
- MulticastDelegate.cs
- TextRenderer.cs
- BaseComponentEditor.cs
- EmbeddedMailObject.cs
- StringPropertyBuilder.cs
- ListViewDataItem.cs
- TextParentUndoUnit.cs
- cookieexception.cs
- CollectionView.cs
- CodePageUtils.cs
- ListViewItem.cs
- EncryptedType.cs
- ParserOptions.cs
- ParserContext.cs
- DriveInfo.cs
- StrongNameIdentityPermission.cs
- TemplateParser.cs
- DataRelationPropertyDescriptor.cs
- CollectionViewGroupRoot.cs
- CqlLexer.cs
- DirectionalLight.cs
- OperationPerformanceCounters.cs
- KerberosSecurityTokenParameters.cs
- InvokeMethodActivity.cs
- TransformDescriptor.cs
- BaseComponentEditor.cs
- FixedSOMTableRow.cs
- CodeParameterDeclarationExpression.cs
- PerformanceCounterLib.cs
- Pens.cs
- RectangleConverter.cs
- X500Name.cs
- CodeIndexerExpression.cs
- ContractHandle.cs
- ConfigXmlElement.cs
- MenuBase.cs
- ManualResetEvent.cs
- Token.cs
- ObjectSet.cs
- DataSourceSelectArguments.cs
- SpoolingTaskBase.cs
- GridSplitterAutomationPeer.cs
- PathGeometry.cs
- ControlBuilderAttribute.cs
- SynchronizedDispatch.cs
- DataFormats.cs
- ProfileGroupSettingsCollection.cs
- BuildDependencySet.cs
- DesignTimeParseData.cs
- StringValidator.cs
- XPathScanner.cs
- SerialErrors.cs