Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / SystemKeyConverter.cs / 1 / SystemKeyConverter.cs
//---------------------------------------------------------------------------- // // File: SystemKeyConverter.cs // // Description: // TypeConverter for SystemResourceKey and SystemThemeKey. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Globalization; using System.ComponentModel.Design.Serialization; using System.Windows.Controls; using System.Windows.Controls.Primitives; namespace System.Windows.Markup { ////// Common TypeConverter functionality SystemThemeKey and SystemResourceKey; each /// is an internal type, so gets serialized as an {x:Static} reference. /// internal class SystemKeyConverter : TypeConverter { ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert from /// ////// true if conversion is possible /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == null) { throw new ArgumentNullException("sourceType"); } return base.CanConvertFrom(context, sourceType); } ////// TypeConverter method override. /// /// /// ITypeDescriptorContext /// /// /// Type to convert to /// ////// true if conversion is possible /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // Validate Input Arguments if (destinationType == null) { throw new ArgumentNullException("destinationType"); } else if( destinationType == typeof(MarkupExtension) && context is IValueSerializerContext ) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// ////// value that is result of conversion /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { return base.ConvertFrom(context, culture, value); } ////// TypeConverter method implementation. /// /// /// ITypeDescriptorContext /// /// /// current culture (see CLR specs) /// /// /// value to convert from /// /// /// Type to convert to /// ////// converted value /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { // Input validation if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(MarkupExtension) && CanConvertTo(context, destinationType) ) { SystemResourceKeyID keyId; // Get the SystemResourceKeyID if( value is SystemResourceKey ) { keyId = (value as SystemResourceKey).InternalKey; } else if( value is SystemThemeKey ) { keyId = (value as SystemThemeKey).InternalKey; } else { throw new ArgumentException(SR.Get(SRID.MustBeOfType, "value", "SystemResourceKey or SystemThemeKey")); } // System resource keys can be converted into a MarkupExtension (StaticExtension) Type keyType = SystemKeyConverter.GetSystemClassType(keyId); // Get the value serialization context IValueSerializerContext valueSerializerContext = context as IValueSerializerContext; if( valueSerializerContext != null ) { // And from that get a System.Type serializer ValueSerializer typeSerializer = valueSerializerContext.GetValueSerializerFor(typeof(Type)); if( typeSerializer != null ) { // And use that to create the string-ized class name string systemClassName = typeSerializer.ConvertToString(keyType, valueSerializerContext); // And finally create the StaticExtension. return new StaticExtension( systemClassName + "." + GetSystemKeyName(keyId) ); } } } return base.CanConvertTo(context, destinationType); } internal static Type GetSystemClassType(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemColorsEnd)) { return typeof(SystemColors); } else if ((SystemResourceKeyID.InternalSystemFontsStart < id) && (id < SystemResourceKeyID.InternalSystemFontsEnd)) { return typeof(SystemFonts); } else if ((SystemResourceKeyID.InternalSystemParametersStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return typeof(SystemParameters); } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id) { return typeof(MenuItem); } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { return typeof(ToolBar); } else if (SystemResourceKeyID.StatusBarSeparatorStyle == id) { return typeof(StatusBar); } else if ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle)) { return typeof(GridView); } return null; } internal static string GetSystemClassName(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemColorsEnd)) { return "SystemColors"; } else if ((SystemResourceKeyID.InternalSystemFontsStart < id) && (id < SystemResourceKeyID.InternalSystemFontsEnd)) { return "SystemFonts"; } else if ((SystemResourceKeyID.InternalSystemParametersStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return "SystemParameters"; } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id) { return "MenuItem"; } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { return "ToolBar"; } else if (SystemResourceKeyID.StatusBarSeparatorStyle == id) { return "StatusBar"; } else if ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle)) { return "GridView"; } return String.Empty; } internal static string GetSystemKeyName(SystemResourceKeyID id) { if (((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) || ((SystemResourceKeyID.GridViewScrollViewerStyle <= id) && (id <= SystemResourceKeyID.GridViewItemContainerStyle))) { return Enum.GetName(typeof(SystemResourceKeyID), id) + "Key"; } else if (SystemResourceKeyID.MenuItemSeparatorStyle == id || SystemResourceKeyID.StatusBarSeparatorStyle == id) { return "SeparatorStyleKey"; } else if ((SystemResourceKeyID.ToolBarButtonStyle <= id) && (id <= SystemResourceKeyID.ToolBarMenuStyle)) { string propName = Enum.GetName(typeof(SystemResourceKeyID), id) + "Key"; return propName.Remove(0, 7); // Remove the "ToolBar" prefix } return String.Empty; } internal static string GetSystemPropertyName(SystemResourceKeyID id) { if ((SystemResourceKeyID.InternalSystemColorsStart < id) && (id < SystemResourceKeyID.InternalSystemParametersEnd)) { return Enum.GetName(typeof(SystemResourceKeyID), id); } return String.Empty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
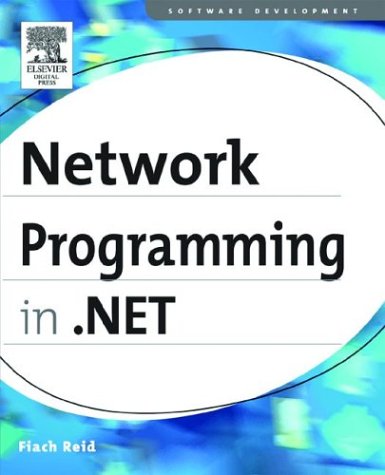
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Facet.cs
- EntitySet.cs
- UidManager.cs
- SafeViewOfFileHandle.cs
- WithStatement.cs
- nulltextcontainer.cs
- sitestring.cs
- MaterialGroup.cs
- _StreamFramer.cs
- SectionInput.cs
- WindowsSolidBrush.cs
- WrapperEqualityComparer.cs
- AdjustableArrowCap.cs
- SafeFileMappingHandle.cs
- BitmapFrameEncode.cs
- CommonDialog.cs
- _OSSOCK.cs
- ColumnWidthChangingEvent.cs
- EmptyElement.cs
- DataGridViewEditingControlShowingEventArgs.cs
- ParserStack.cs
- RegistryConfigurationProvider.cs
- FlowDocumentReader.cs
- Image.cs
- CacheChildrenQuery.cs
- TextFormatter.cs
- CodeAttributeDeclaration.cs
- ItemContainerGenerator.cs
- ProfileGroupSettingsCollection.cs
- ResourceDictionary.cs
- DateBoldEvent.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- GetPageCompletedEventArgs.cs
- DispatcherExceptionEventArgs.cs
- FixedDocumentPaginator.cs
- ContentAlignmentEditor.cs
- RegexCaptureCollection.cs
- Method.cs
- TcpTransportElement.cs
- ToolStripDropDownClosedEventArgs.cs
- HyperLinkField.cs
- WorkflowMarkupSerializer.cs
- WebPartConnectionsDisconnectVerb.cs
- EditorZoneBase.cs
- SubpageParaClient.cs
- CssStyleCollection.cs
- regiisutil.cs
- DataBoundControl.cs
- Geometry3D.cs
- LinqToSqlWrapper.cs
- UnsafePeerToPeerMethods.cs
- EmptyElement.cs
- TdsParser.cs
- SHA1Cng.cs
- RichTextBox.cs
- CheckBox.cs
- QualifiedId.cs
- CompiledRegexRunnerFactory.cs
- SelectionService.cs
- PerfCounters.cs
- Int32CAMarshaler.cs
- EmissiveMaterial.cs
- CompilerScopeManager.cs
- MultiSelectRootGridEntry.cs
- ProfilePropertySettings.cs
- EncoderFallback.cs
- ADConnectionHelper.cs
- HwndSource.cs
- SoapUnknownHeader.cs
- PrintingPermissionAttribute.cs
- SubpageParaClient.cs
- PropertyGridCommands.cs
- NameValueCollection.cs
- TypedReference.cs
- ComponentTray.cs
- IResourceProvider.cs
- Vector3DIndependentAnimationStorage.cs
- FixedSOMContainer.cs
- ColorAnimationUsingKeyFrames.cs
- PartitionResolver.cs
- HttpCacheParams.cs
- Icon.cs
- TextContainerChangeEventArgs.cs
- RenamedEventArgs.cs
- ActionItem.cs
- SerializerWriterEventHandlers.cs
- MaskedTextProvider.cs
- TargetControlTypeAttribute.cs
- ByteStream.cs
- CornerRadius.cs
- WorkflowOperationErrorHandler.cs
- Point.cs
- MatrixAnimationUsingKeyFrames.cs
- SourceSwitch.cs
- SapiGrammar.cs
- XmlHierarchicalEnumerable.cs
- BooleanExpr.cs
- XmlSchemaAll.cs
- VirtualizingStackPanel.cs
- XmlAttributes.cs