Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Principal / WindowsImpersonationContext.cs / 1 / WindowsImpersonationContext.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // WindowsImpersonationContext.cs // // Representation of an impersonation context. // namespace System.Security.Principal { using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Runtime.ConstrainedExecution; [System.Runtime.InteropServices.ComVisible(true)] public class WindowsImpersonationContext : IDisposable { private SafeTokenHandle m_safeTokenHandle = SafeTokenHandle.InvalidHandle; private WindowsIdentity m_wi; private FrameSecurityDescriptor m_fsd; private WindowsImpersonationContext () {} internal WindowsImpersonationContext (SafeTokenHandle safeTokenHandle, WindowsIdentity wi, bool isImpersonating, FrameSecurityDescriptor fsd) { // make this a no-op on Win98 so calling code does not have to special case down-level platforms. if (WindowsIdentity.RunningOnWin2K) { if (safeTokenHandle.IsInvalid) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidImpersonationToken")); if (isImpersonating) { if (!Win32Native.DuplicateHandle(Win32Native.GetCurrentProcess(), safeTokenHandle, Win32Native.GetCurrentProcess(), ref m_safeTokenHandle, 0, true, Win32Native.DUPLICATE_SAME_ACCESS)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); m_wi = wi; } m_fsd = fsd; } } // Revert to previous impersonation (the only public method). public void Undo () { // make this a no-op on Win98 so calling code does not have to special case down-level platforms. if (!WindowsIdentity.RunningOnWin2K) return; int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); } else { hr = Win32.RevertToSelf(); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); if (hr < 0) throw new SecurityException(Win32Native.GetMessage(hr)); } WindowsIdentity.UpdateThreadWI(m_wi); if (m_fsd != null) m_fsd.SetTokenHandles(null, null); } // Non-throwing version that does not new any exception objects. To be called when reliability matters [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] internal bool UndoNoThrow() { bool bRet = false; try{ // make this a no-op on Win98 so calling code does not have to special case down-level platforms. if (!WindowsIdentity.RunningOnWin2K) return true; int hr = 0; if (m_safeTokenHandle.IsInvalid) { // the thread was not initially impersonating hr = Win32.RevertToSelf(); } else { hr = Win32.RevertToSelf(); if (hr >= 0) hr = Win32.ImpersonateLoggedOnUser(m_safeTokenHandle); } bRet = (hr >= 0); if (m_fsd != null) m_fsd.SetTokenHandles(null,null); } catch { bRet = false; } return bRet; } // // IDisposable interface. // [ComVisible(false)] protected virtual void Dispose(bool disposing) { if (disposing) { if (m_safeTokenHandle != null && !m_safeTokenHandle.IsClosed) { Undo(); m_safeTokenHandle.Dispose(); } } } [ComVisible(false)] public void Dispose () { Dispose(true); } } }
Link Menu
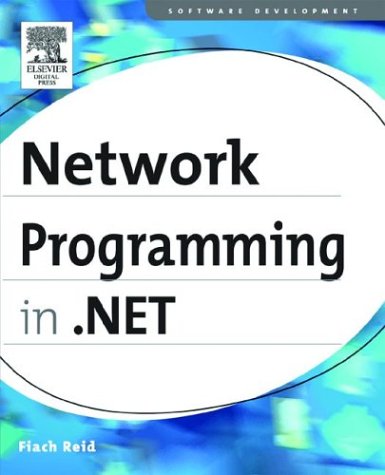
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProtectedConfigurationSection.cs
- SimpleLine.cs
- Floater.cs
- NativeMethods.cs
- GZipDecoder.cs
- VisualBasicReference.cs
- ServiceBusyException.cs
- DbMetaDataColumnNames.cs
- RangeValueProviderWrapper.cs
- DataGridViewImageCell.cs
- ImageList.cs
- ProfileSettingsCollection.cs
- Attachment.cs
- SHA512.cs
- InputLanguageManager.cs
- ProgressPage.cs
- Adorner.cs
- RestHandlerFactory.cs
- PageThemeParser.cs
- RMPermissions.cs
- PopupControlService.cs
- CompModSwitches.cs
- DataListDesigner.cs
- DataControlButton.cs
- ServicePoint.cs
- SignedXml.cs
- UnsafeNativeMethodsMilCoreApi.cs
- TextTreeUndoUnit.cs
- IImplicitResourceProvider.cs
- MobileControl.cs
- ContextProperty.cs
- PartBasedPackageProperties.cs
- QueryStringHandler.cs
- MethodInfo.cs
- ValidationHelper.cs
- ProcessProtocolHandler.cs
- GridItemProviderWrapper.cs
- DataMemberConverter.cs
- TableLayoutPanelBehavior.cs
- CombinedGeometry.cs
- DragEventArgs.cs
- StyleSheet.cs
- PathFigureCollectionValueSerializer.cs
- SchemaImporterExtension.cs
- SoapRpcMethodAttribute.cs
- Point3D.cs
- BitmapEffectGroup.cs
- SafePEFileHandle.cs
- HttpCachePolicy.cs
- InternalDispatchObject.cs
- InvalidProgramException.cs
- WebConfigurationFileMap.cs
- ScopedKnownTypes.cs
- TriggerCollection.cs
- ConfigUtil.cs
- SafeUserTokenHandle.cs
- MarginsConverter.cs
- WebBrowsableAttribute.cs
- DataServiceQueryException.cs
- Rect3D.cs
- Object.cs
- HorizontalAlignConverter.cs
- SmtpReplyReaderFactory.cs
- InkCanvasFeedbackAdorner.cs
- TypographyProperties.cs
- OverflowException.cs
- ToolboxSnapDragDropEventArgs.cs
- TableParaClient.cs
- SafeSystemMetrics.cs
- DictionaryCustomTypeDescriptor.cs
- ComPlusSynchronizationContext.cs
- WebConfigurationFileMap.cs
- BmpBitmapEncoder.cs
- SchemaImporterExtensionElement.cs
- XmlAttributeCollection.cs
- CustomAttribute.cs
- AutomationProperties.cs
- HtmlEncodedRawTextWriter.cs
- _ChunkParse.cs
- DebugView.cs
- PerformanceCounterManager.cs
- SoapSchemaMember.cs
- SqlCacheDependency.cs
- OperandQuery.cs
- panel.cs
- ProtocolsConfiguration.cs
- Exception.cs
- EntityDataSourceQueryBuilder.cs
- ApplicationSecurityInfo.cs
- NavigationService.cs
- ReadOnlyCollectionBuilder.cs
- UnionExpr.cs
- InputMethod.cs
- URI.cs
- ProtocolsConfigurationEntry.cs
- HttpValueCollection.cs
- Array.cs
- XmlBindingWorker.cs
- CodeCompiler.cs
- StructuredProperty.cs