Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Automation / Peers / TableAutomationPeer.cs / 1 / TableAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TableAutomationPeer.cs // // Description: Automation peer for Table // //--------------------------------------------------------------------------- using System.Windows.Automation.Provider; // IRawElementProviderSimple using System.Windows.Documents; namespace System.Windows.Automation.Peers { /// public class TableAutomationPeer : TextElementAutomationPeer, IGridProvider { ////// Constructor. /// /// Owner of the AutomationPeer. public TableAutomationPeer(Table owner) : base(owner) { _rowCount = GetRowCount(); _columnCount = GetColumnCount(); } ////// public override object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.Grid) { return this; } return null; } ////// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Table; } ////// /// protected override string GetClassNameCore() { return "Table"; } ////// /// protected override bool IsControlElementCore() { return true; } ////// /// protected override bool IsContentElementCore() { return true; } ////// /// Raises property changed events in response to structure changes. /// internal void OnStructureInvalidated() { int rowCount = GetRowCount(); if (rowCount != _rowCount) { RaisePropertyChangedEvent(GridPatternIdentifiers.RowCountProperty, _rowCount, rowCount); _rowCount = rowCount; } int columnCount = GetColumnCount(); if (columnCount != _columnCount) { RaisePropertyChangedEvent(GridPatternIdentifiers.ColumnCountProperty, _columnCount, columnCount); _columnCount = columnCount; } } ////// Returns the number of rows. /// private int GetRowCount() { int rows = 0; foreach (TableRowGroup group in ((Table)Owner).RowGroups) { rows += group.Rows.Count; } return rows; } ////// Returns the number of columns. /// private int GetColumnCount() { return ((Table)Owner).ColumnCount; } private int _rowCount; private int _columnCount; //------------------------------------------------------------------- // // IGridProvider Members // //------------------------------------------------------------------- #region IGridProvider Members ////// Returns the provider for the element that is located at the row and /// column location requested by the client. /// IRawElementProviderSimple IGridProvider.GetItem(int row, int column) { if (row < 0 || row >= ((IGridProvider)this).RowCount) { throw new ArgumentOutOfRangeException("row"); } if (column < 0 || column >= ((IGridProvider)this).ColumnCount) { throw new ArgumentOutOfRangeException("column"); } int currentRow = 0; Table table = (Table)Owner; foreach (TableRowGroup group in table.RowGroups) { if (currentRow + group.Rows.Count < row) { currentRow += group.Rows.Count; } else { foreach (TableRow tableRow in group.Rows) { if (currentRow == row) { foreach (TableCell cell in tableRow.Cells) { if (cell.ColumnIndex <= column && cell.ColumnIndex + cell.ColumnSpan > column) { return ProviderFromPeer(CreatePeerForElement(cell)); } } // check spanned cells foreach (TableCell cell in tableRow.SpannedCells) { if (cell.ColumnIndex <= column && cell.ColumnIndex + cell.ColumnSpan > column) { return ProviderFromPeer(CreatePeerForElement(cell)); } } } else { currentRow++; } } } } return null; } ////// Returns the number of rows in the grid at the time this was requested. /// int IGridProvider.RowCount { get { return _rowCount; } } ////// Returns the number of columns in the grid at the time this was requested. /// int IGridProvider.ColumnCount { get { return _columnCount; } } #endregion IGridProvider Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: TableAutomationPeer.cs // // Description: Automation peer for Table // //--------------------------------------------------------------------------- using System.Windows.Automation.Provider; // IRawElementProviderSimple using System.Windows.Documents; namespace System.Windows.Automation.Peers { /// public class TableAutomationPeer : TextElementAutomationPeer, IGridProvider { ////// Constructor. /// /// Owner of the AutomationPeer. public TableAutomationPeer(Table owner) : base(owner) { _rowCount = GetRowCount(); _columnCount = GetColumnCount(); } ////// public override object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.Grid) { return this; } return null; } ////// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Table; } ////// /// protected override string GetClassNameCore() { return "Table"; } ////// /// protected override bool IsControlElementCore() { return true; } ////// /// protected override bool IsContentElementCore() { return true; } ////// /// Raises property changed events in response to structure changes. /// internal void OnStructureInvalidated() { int rowCount = GetRowCount(); if (rowCount != _rowCount) { RaisePropertyChangedEvent(GridPatternIdentifiers.RowCountProperty, _rowCount, rowCount); _rowCount = rowCount; } int columnCount = GetColumnCount(); if (columnCount != _columnCount) { RaisePropertyChangedEvent(GridPatternIdentifiers.ColumnCountProperty, _columnCount, columnCount); _columnCount = columnCount; } } ////// Returns the number of rows. /// private int GetRowCount() { int rows = 0; foreach (TableRowGroup group in ((Table)Owner).RowGroups) { rows += group.Rows.Count; } return rows; } ////// Returns the number of columns. /// private int GetColumnCount() { return ((Table)Owner).ColumnCount; } private int _rowCount; private int _columnCount; //------------------------------------------------------------------- // // IGridProvider Members // //------------------------------------------------------------------- #region IGridProvider Members ////// Returns the provider for the element that is located at the row and /// column location requested by the client. /// IRawElementProviderSimple IGridProvider.GetItem(int row, int column) { if (row < 0 || row >= ((IGridProvider)this).RowCount) { throw new ArgumentOutOfRangeException("row"); } if (column < 0 || column >= ((IGridProvider)this).ColumnCount) { throw new ArgumentOutOfRangeException("column"); } int currentRow = 0; Table table = (Table)Owner; foreach (TableRowGroup group in table.RowGroups) { if (currentRow + group.Rows.Count < row) { currentRow += group.Rows.Count; } else { foreach (TableRow tableRow in group.Rows) { if (currentRow == row) { foreach (TableCell cell in tableRow.Cells) { if (cell.ColumnIndex <= column && cell.ColumnIndex + cell.ColumnSpan > column) { return ProviderFromPeer(CreatePeerForElement(cell)); } } // check spanned cells foreach (TableCell cell in tableRow.SpannedCells) { if (cell.ColumnIndex <= column && cell.ColumnIndex + cell.ColumnSpan > column) { return ProviderFromPeer(CreatePeerForElement(cell)); } } } else { currentRow++; } } } } return null; } ////// Returns the number of rows in the grid at the time this was requested. /// int IGridProvider.RowCount { get { return _rowCount; } } ////// Returns the number of columns in the grid at the time this was requested. /// int IGridProvider.ColumnCount { get { return _columnCount; } } #endregion IGridProvider Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
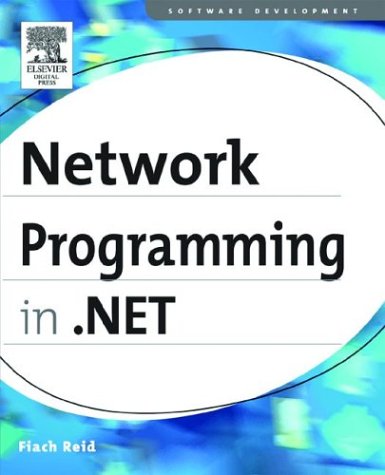
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymDocumentType.cs
- BamlTreeUpdater.cs
- ForEachAction.cs
- UpDownBase.cs
- DataList.cs
- AutomationAttributeInfo.cs
- WebUtil.cs
- TreeIterators.cs
- AvTraceDetails.cs
- BufferedWebEventProvider.cs
- _HTTPDateParse.cs
- Base64Decoder.cs
- TreeViewHitTestInfo.cs
- XmlSerializationGeneratedCode.cs
- SelectedDatesCollection.cs
- ViewValidator.cs
- TypedTableBase.cs
- BehaviorEditorPart.cs
- MonthChangedEventArgs.cs
- HtmlString.cs
- Internal.cs
- codemethodreferenceexpression.cs
- TextTreeText.cs
- HtmlTextArea.cs
- ValidationHelper.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- XmlReader.cs
- PersonalizablePropertyEntry.cs
- BinaryHeap.cs
- WebBrowserUriTypeConverter.cs
- xmlsaver.cs
- AssemblyUtil.cs
- ActivityBuilder.cs
- CngUIPolicy.cs
- TextViewSelectionProcessor.cs
- InputMethodStateChangeEventArgs.cs
- ObjectListItem.cs
- CompilerInfo.cs
- XmlNodeList.cs
- Nullable.cs
- MultiPropertyDescriptorGridEntry.cs
- EmptyReadOnlyDictionaryInternal.cs
- TableParagraph.cs
- TextTreeUndoUnit.cs
- NullExtension.cs
- CompoundFileDeflateTransform.cs
- baseaxisquery.cs
- XmlSerializerNamespaces.cs
- RetrieveVirtualItemEventArgs.cs
- DocumentReferenceCollection.cs
- SequenceFullException.cs
- ParserStreamGeometryContext.cs
- XPathDescendantIterator.cs
- TranslateTransform.cs
- DataGridViewDataErrorEventArgs.cs
- IISMapPath.cs
- VariantWrapper.cs
- TargetException.cs
- MobileCategoryAttribute.cs
- ProxyFragment.cs
- NameValueConfigurationElement.cs
- XLinq.cs
- Font.cs
- PlatformNotSupportedException.cs
- PublisherMembershipCondition.cs
- IIS7WorkerRequest.cs
- StackOverflowException.cs
- DataPagerField.cs
- SQLByteStorage.cs
- MessagePropertyDescriptionCollection.cs
- Metafile.cs
- NameValueConfigurationCollection.cs
- TextFormatterHost.cs
- VisualStyleElement.cs
- MetadataSet.cs
- CompleteWizardStep.cs
- TimeIntervalCollection.cs
- PermissionListSet.cs
- SkinBuilder.cs
- ListViewItemMouseHoverEvent.cs
- EdmConstants.cs
- ApplicationDirectoryMembershipCondition.cs
- ParamArrayAttribute.cs
- ControlPager.cs
- SqlConnectionPoolGroupProviderInfo.cs
- AssemblyBuilder.cs
- InstanceNotReadyException.cs
- remotingproxy.cs
- FunctionDescription.cs
- XamlTypeMapper.cs
- CultureSpecificCharacterBufferRange.cs
- ListItemCollection.cs
- RegexCapture.cs
- CultureInfo.cs
- PropertyDescriptorComparer.cs
- MimeBasePart.cs
- AnimationLayer.cs
- SqlGenerator.cs
- Journal.cs
- RelationshipConverter.cs