Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / FontSizeConverter.cs / 1 / FontSizeConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FontSizeConverter.cs // // Description: Converter for FontSize to Double // // History: // 01/12/05 : GHermann - Ported from FontSize // //--------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; // for ConstructorInfo using System.Globalization; // for CultureInfo using MS.Utility; // For ExceptionStringTable using System.Windows.Media; // for Typeface using System.Windows.Controls; // for ReadingPanel using System.Windows.Documents; using System.Windows.Markup; using MS.Internal; using MS.Internal.Text; using MS.Internal.Documents; namespace System.Windows { ////// TypeConverter to convert FontSize to/from other types. /// Currently: string, int, float, and double are supported. /// // Used by Parser to parse BoxUnits from markup. public class FontSizeConverter : TypeConverter { ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert from ///true if conversion is possible public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return (sourceType == typeof(string) || sourceType == typeof(int) || sourceType == typeof(float) || sourceType == typeof(double)); } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// Current culture (see CLR specs) /// value to convert from ///value that is result of conversion public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } // NB: The culture passed from Avalon parser should always be the invariant culture. // Do we care about usage in other circumstances? string text = value as string; if (text != null) { double amount; FromString(text, culture, out amount); return amount; } if (value is System.Int32 || value is System.Single || value is System.Double) { return (double)value; } // Can't convert, wrong type return null; } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } double fs = (double)value; if (destinationType == typeof(string)) { return fs.ToString(culture); } if (destinationType == typeof(int)) { return (int)fs; } if (destinationType == typeof(float)) { return (float)fs; } if (destinationType == typeof(double)) { return fs; } return base.ConvertTo(context, culture, value, destinationType); } ////// Convert a string into an amount and a font size type that can be used to create /// and instance of a Fontsize, or to serialize in a more compact representation to /// a baml stream. /// internal static void FromString( string text, CultureInfo culture, out double amount) { amount = LengthConverter.FromString(text, culture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FontSizeConverter.cs // // Description: Converter for FontSize to Double // // History: // 01/12/05 : GHermann - Ported from FontSize // //--------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; // for ConstructorInfo using System.Globalization; // for CultureInfo using MS.Utility; // For ExceptionStringTable using System.Windows.Media; // for Typeface using System.Windows.Controls; // for ReadingPanel using System.Windows.Documents; using System.Windows.Markup; using MS.Internal; using MS.Internal.Text; using MS.Internal.Documents; namespace System.Windows { ////// TypeConverter to convert FontSize to/from other types. /// Currently: string, int, float, and double are supported. /// // Used by Parser to parse BoxUnits from markup. public class FontSizeConverter : TypeConverter { ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert from ///true if conversion is possible public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return (sourceType == typeof(string) || sourceType == typeof(int) || sourceType == typeof(float) || sourceType == typeof(double)); } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// Current culture (see CLR specs) /// value to convert from ///value that is result of conversion public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } // NB: The culture passed from Avalon parser should always be the invariant culture. // Do we care about usage in other circumstances? string text = value as string; if (text != null) { double amount; FromString(text, culture, out amount); return amount; } if (value is System.Int32 || value is System.Single || value is System.Double) { return (double)value; } // Can't convert, wrong type return null; } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } double fs = (double)value; if (destinationType == typeof(string)) { return fs.ToString(culture); } if (destinationType == typeof(int)) { return (int)fs; } if (destinationType == typeof(float)) { return (float)fs; } if (destinationType == typeof(double)) { return fs; } return base.ConvertTo(context, culture, value, destinationType); } ////// Convert a string into an amount and a font size type that can be used to create /// and instance of a Fontsize, or to serialize in a more compact representation to /// a baml stream. /// internal static void FromString( string text, CultureInfo culture, out double amount) { amount = LengthConverter.FromString(text, culture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
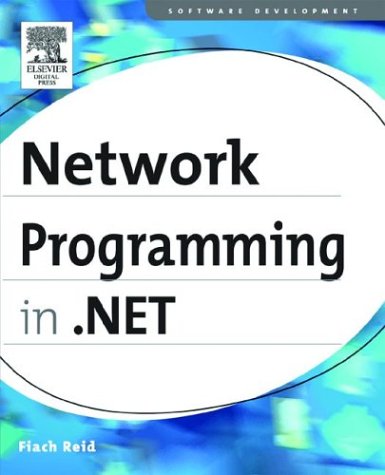
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Dump.cs
- DefinitionBase.cs
- ScalarType.cs
- FormViewInsertEventArgs.cs
- AutomationElement.cs
- ToolStripSeparator.cs
- ButtonColumn.cs
- EasingFunctionBase.cs
- WorkflowEnvironment.cs
- BaseUriHelper.cs
- TreeNodeStyle.cs
- WebReferencesBuildProvider.cs
- XmlSequenceWriter.cs
- ApplicationSettingsBase.cs
- TypeInfo.cs
- StylusEditingBehavior.cs
- ADMembershipProvider.cs
- CaseInsensitiveHashCodeProvider.cs
- MarkupCompilePass2.cs
- KeyValuePairs.cs
- UpdatableWrapper.cs
- SymbolMethod.cs
- XmlCharCheckingReader.cs
- CopyAction.cs
- InternalConfigRoot.cs
- MasterPageCodeDomTreeGenerator.cs
- MessageUtil.cs
- Style.cs
- SiteMembershipCondition.cs
- SplitContainer.cs
- SemanticResultValue.cs
- Registry.cs
- BinHexDecoder.cs
- HttpCacheVary.cs
- NamespaceQuery.cs
- StatusStrip.cs
- InsufficientMemoryException.cs
- WsatTransactionHeader.cs
- Pen.cs
- CodeDomSerializerBase.cs
- UnsafeNativeMethods.cs
- UrlPropertyAttribute.cs
- ListSortDescriptionCollection.cs
- HttpDictionary.cs
- ReliabilityContractAttribute.cs
- Helpers.cs
- UrlMapping.cs
- HttpApplicationFactory.cs
- DashStyles.cs
- GeneralTransform3DGroup.cs
- AutomationElementCollection.cs
- GeometryModel3D.cs
- entitydatasourceentitysetnameconverter.cs
- XmlHierarchicalEnumerable.cs
- PreviewKeyDownEventArgs.cs
- SafeLibraryHandle.cs
- SimpleApplicationHost.cs
- AutomationTextAttribute.cs
- ObjectListGeneralPage.cs
- ImageAnimator.cs
- DataGridViewComponentPropertyGridSite.cs
- CancellationTokenRegistration.cs
- odbcmetadatacolumnnames.cs
- MetafileHeader.cs
- HttpHandlersSection.cs
- PasswordDeriveBytes.cs
- InputManager.cs
- PeerNameRecord.cs
- IApplicationTrustManager.cs
- DirectionalLight.cs
- QilSortKey.cs
- XmlDesigner.cs
- MSAAWinEventWrap.cs
- AuthenticationService.cs
- CounterSetInstance.cs
- BitmapFrameEncode.cs
- _ConnectionGroup.cs
- PathGeometry.cs
- MemberMemberBinding.cs
- RawAppCommandInputReport.cs
- ipaddressinformationcollection.cs
- Stackframe.cs
- DispatcherHooks.cs
- LineProperties.cs
- SerialErrors.cs
- ImageField.cs
- ContextStack.cs
- ZipIOExtraFieldElement.cs
- MediaElement.cs
- ActiveXHelper.cs
- CompilerTypeWithParams.cs
- hwndwrapper.cs
- IIS7WorkerRequest.cs
- Sequence.cs
- PageStatePersister.cs
- ByteStack.cs
- MaskedTextBoxDesignerActionList.cs
- EndGetFileNameFromUserRequest.cs
- SqlParameter.cs
- UnsafeNativeMethodsMilCoreApi.cs