Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Markup / XamlTemplateSerializer.cs / 1 / XamlTemplateSerializer.cs
//---------------------------------------------------------------------------- // // File: XamlTemplateSerializer.cs // // Description: // Class that serializes and deserializes Templates. // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Collections; using System.Globalization; using System.IO; using System.Reflection; using System.Text; using System.Xml; using System.Security.Permissions; using MS.Utility; #if !PBTCOMPILER using System.Windows.Data; using System.Windows.Controls; using System.Windows.Documents; #endif #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ////// Class that knows how to serialize and deserialize Template objects /// internal class XamlTemplateSerializer : XamlSerializer { #region Construction ////// Constructor for XamlTemplateSerializer /// public XamlTemplateSerializer() : base() { } internal XamlTemplateSerializer(ParserHooks parserHooks) : base() { _parserHooks = parserHooks; } #endregion Construction #region OtherConversions ////// Convert from Xaml read by a token reader into baml being written /// out by a record writer. The context gives mapping information. /// #if !PBTCOMPILER //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] #endif internal override void ConvertXamlToBaml ( XamlReaderHelper tokenReader, ParserContext context, XamlNode xamlNode, BamlRecordWriter bamlWriter) { TemplateXamlParser templateParser = new TemplateXamlParser(tokenReader, context); templateParser.ParserHooks = _parserHooks; templateParser.BamlRecordWriter = bamlWriter; // Process the xamlNode that is passed in so that the element is written to baml templateParser.WriteElementStart((XamlElementStartNode)xamlNode); // Parse the entire Template section now, writing everything out directly to BAML. templateParser.Parse(); } #if !PBTCOMPILER ////// Convert from Xaml read by a token reader into a live /// object tree. The context gives mapping information. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override void ConvertXamlToObject ( XamlReaderHelper tokenReader, ReadWriteStreamManager streamManager, ParserContext context, XamlNode xamlNode, BamlRecordReader reader) { TemplateXamlTreeBuilder treeBuilder = new TemplateXamlTreeBuilder(context, tokenReader, streamManager, reader.ContextStack, reader.RootList); // Process the xamlNode that is passed in so that the element is written to baml treeBuilder.ProcessXamlNode(xamlNode); // Parse the entire Template section now. This should exit voluntarily at the end of the // Template block. In the future we may limit how much the tokenReader reads to prevent // inadvertent reading past the end of the block. treeBuilder.ParseFragment(); } ////// Convert from Baml read by a baml reader into an object tree. /// The context gives mapping information. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override void ConvertBamlToObject ( BamlRecordReader reader, // Current reader that is processing records BamlRecord bamlRecord, // Record read in that triggered serializer ParserContext context) // Context { Debug.Assert(reader.MapTable==context.MapTable); TemplateTreeBuilderBamlTranslator treeBuilder = new TemplateTreeBuilderBamlTranslator(context, reader.BamlStream, reader.PreParsedRecordsStart, reader.PreParsedCurrentRecord, reader.ContextStack, reader.RootList, reader.XamlParseMode, reader.MaxAsyncRecords); // Process the passed record, which is presumably the start tag. This // must be processed out-of-line, since the previous reader has already retrieved it // from the baml stream treeBuilder.RecordReader.ReadRecord(bamlRecord); // Now process the contents of the Template block after the start tag. treeBuilder.Parse(); // Have the original TreeBuilder catch up with the parsed records, since we used a // new treebuilder for this portion of the parsing. reader.PreParsedCurrentRecord = treeBuilder.RecordReader.PreParsedCurrentRecord; } ////// If the Template represented by a group of baml records is stored in a dictionary, this /// method will extract the key used for this dictionary from the passed /// collection of baml records. For ControlTemplate, this is the styleTargetType. /// For DataTemplate, this is the DataTemplateKey containing the DataType. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override object GetDictionaryKey(BamlRecord startRecord, ParserContext parserContext) { object key = null; int numberOfElements = 0; BamlRecord record = startRecord; short ownerTypeId = 0; while (record != null) { if (record.RecordType == BamlRecordType.ElementStart) { BamlElementStartRecord elementStart = record as BamlElementStartRecord; if (++numberOfElements == 1) { // save the type ID of the first element (i.e.) ownerTypeId = elementStart.TypeId; } else { // We didn't find the key before a reading the // VisualTree nodes of the template break; } } else if (record.RecordType == BamlRecordType.Property && numberOfElements == 1) { // look for the TargetType property on the element // or the DataType property on the tag or // TableTemplate.Tree or the tag break; } record = record.Next; } if (key == null) { ThrowException(SRID.StyleNoDictionaryKey, parserContext.LineNumber, parserContext.LinePosition, null); } return key; } // Helper to insert line and position numbers into message, if they are present void ThrowException( string id, int lineNumber, int linePosition, Exception innerException) { string message = SR.Get(id); XamlParseException parseException; // Throw the appropriate execption. If we have line numbers, then we are // parsing a xaml file, so throw a xaml exception. Otherwise were are // parsing a baml file. if (lineNumber > 0) { message += " "; message += SR.Get(SRID.ParserLineAndOffset, lineNumber.ToString(CultureInfo.CurrentUICulture), linePosition.ToString(CultureInfo.CurrentUICulture)); parseException = new XamlParseException(message, lineNumber, linePosition); } else { parseException = new XamlParseException(message); } throw parseException; } #endif // !PBTCOMPILER #endregion OtherConversions #region Data private ParserHooks _parserHooks = null; // Constants used for emitting specific properties and attributes for a Style internal const string ControlTemplateTagName = "ControlTemplate"; internal const string DataTemplateTagName = "DataTemplate"; internal const string HierarchicalDataTemplateTagName = "HierarchicalDataTemplate"; internal const string ItemsPanelTemplateTagName = "ItemsPanelTemplate"; internal const string TargetTypePropertyName = "TargetType"; internal const string DataTypePropertyName = "DataType"; internal const string TriggersPropertyName = "Triggers"; internal const string ResourcesPropertyName = "Resources"; internal const string SettersPropertyName = "Setters"; internal const string ItemsSourcePropertyName = "ItemsSource"; internal const string ItemTemplatePropertyName = "ItemTemplate"; internal const string ItemTemplateSelectorPropertyName = "ItemTemplateSelector"; internal const string ItemContainerStylePropertyName = "ItemContainerStyle"; internal const string ItemContainerStyleSelectorPropertyName = "ItemContainerStyleSelector"; internal const string ItemStringFormatPropertyName = "ItemStringFormat"; internal const string ItemBindingGroupPropertyName = "ItemBindingGroup"; internal const string AlternationCountPropertyName = "AlternationCount"; internal const string ControlTemplateTriggersFullPropertyName = ControlTemplateTagName + "." + TriggersPropertyName; internal const string ControlTemplateResourcesFullPropertyName = ControlTemplateTagName + "." + ResourcesPropertyName; internal const string DataTemplateTriggersFullPropertyName = DataTemplateTagName + "." + TriggersPropertyName; internal const string DataTemplateResourcesFullPropertyName = DataTemplateTagName + "." + ResourcesPropertyName; internal const string HierarchicalDataTemplateTriggersFullPropertyName = HierarchicalDataTemplateTagName + "." + TriggersPropertyName; internal const string HierarchicalDataTemplateItemsSourceFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemsSourcePropertyName; internal const string HierarchicalDataTemplateItemTemplateFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemTemplatePropertyName; internal const string HierarchicalDataTemplateItemTemplateSelectorFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemTemplateSelectorPropertyName; internal const string HierarchicalDataTemplateItemContainerStyleFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemContainerStylePropertyName; internal const string HierarchicalDataTemplateItemContainerStyleSelectorFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemContainerStyleSelectorPropertyName; internal const string HierarchicalDataTemplateItemStringFormatFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemStringFormatPropertyName; internal const string HierarchicalDataTemplateItemBindingGroupFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemBindingGroupPropertyName; internal const string HierarchicalDataTemplateAlternationCountFullPropertyName = HierarchicalDataTemplateTagName + "." + AlternationCountPropertyName; internal const string PropertyTriggerPropertyName = "Property"; internal const string PropertyTriggerValuePropertyName = "Value"; internal const string PropertyTriggerSourceName = "SourceName"; internal const string PropertyTriggerEnterActions = "EnterActions"; internal const string PropertyTriggerExitActions = "ExitActions"; internal const string DataTriggerBindingPropertyName = "Binding"; internal const string EventTriggerEventName = "RoutedEvent"; internal const string EventTriggerSourceName = "SourceName"; internal const string EventTriggerActions = "Actions"; internal const string MultiPropertyTriggerConditionsPropertyName = "Conditions"; internal const string SetterTagName = "Setter"; internal const string SetterPropertyAttributeName = "Property"; internal const string SetterValueAttributeName = "Value"; internal const string SetterTargetAttributeName = "TargetName"; internal const string SetterEventAttributeName = "Event"; internal const string SetterHandlerAttributeName = "Handler"; #if HANDLEDEVENTSTOO internal const string SetterHandledEventsTooAttributeName = "HandledEventsToo"; #endif #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlTemplateSerializer.cs // // Description: // Class that serializes and deserializes Templates. // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Collections; using System.Globalization; using System.IO; using System.Reflection; using System.Text; using System.Xml; using System.Security.Permissions; using MS.Utility; #if !PBTCOMPILER using System.Windows.Data; using System.Windows.Controls; using System.Windows.Documents; #endif #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { ///element BamlPropertyRecord propertyRecord = record as BamlPropertyRecord; short attributeOwnerTypeId; string attributeName; BamlAttributeUsage attributeUsage; parserContext.MapTable.GetAttributeInfoFromId(propertyRecord.AttributeId, out attributeOwnerTypeId, out attributeName, out attributeUsage); if (attributeOwnerTypeId == ownerTypeId) { if (attributeName == TargetTypePropertyName) { key = parserContext.XamlTypeMapper.GetDictionaryKey(propertyRecord.Value, parserContext); } else if (attributeName == DataTypePropertyName) { object dataType = parserContext.XamlTypeMapper.GetDictionaryKey(propertyRecord.Value, parserContext); Exception ex = TemplateKey.ValidateDataType(dataType, null); if (ex != null) { ThrowException(SRID.TemplateBadDictionaryKey, parserContext.LineNumber, parserContext.LinePosition, ex); } // key = new DataTemplateKey(dataType); } } } else if (record.RecordType == BamlRecordType.PropertyComplexStart || record.RecordType == BamlRecordType.PropertyIListStart || record.RecordType == BamlRecordType.ElementEnd) { // We didn't find the targetType before a complex property like // FrameworkTemplate.VisualTree or the /// Class that knows how to serialize and deserialize Template objects /// internal class XamlTemplateSerializer : XamlSerializer { #region Construction ////// Constructor for XamlTemplateSerializer /// public XamlTemplateSerializer() : base() { } internal XamlTemplateSerializer(ParserHooks parserHooks) : base() { _parserHooks = parserHooks; } #endregion Construction #region OtherConversions ////// Convert from Xaml read by a token reader into baml being written /// out by a record writer. The context gives mapping information. /// #if !PBTCOMPILER //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] #endif internal override void ConvertXamlToBaml ( XamlReaderHelper tokenReader, ParserContext context, XamlNode xamlNode, BamlRecordWriter bamlWriter) { TemplateXamlParser templateParser = new TemplateXamlParser(tokenReader, context); templateParser.ParserHooks = _parserHooks; templateParser.BamlRecordWriter = bamlWriter; // Process the xamlNode that is passed in so that the element is written to baml templateParser.WriteElementStart((XamlElementStartNode)xamlNode); // Parse the entire Template section now, writing everything out directly to BAML. templateParser.Parse(); } #if !PBTCOMPILER ////// Convert from Xaml read by a token reader into a live /// object tree. The context gives mapping information. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override void ConvertXamlToObject ( XamlReaderHelper tokenReader, ReadWriteStreamManager streamManager, ParserContext context, XamlNode xamlNode, BamlRecordReader reader) { TemplateXamlTreeBuilder treeBuilder = new TemplateXamlTreeBuilder(context, tokenReader, streamManager, reader.ContextStack, reader.RootList); // Process the xamlNode that is passed in so that the element is written to baml treeBuilder.ProcessXamlNode(xamlNode); // Parse the entire Template section now. This should exit voluntarily at the end of the // Template block. In the future we may limit how much the tokenReader reads to prevent // inadvertent reading past the end of the block. treeBuilder.ParseFragment(); } ////// Convert from Baml read by a baml reader into an object tree. /// The context gives mapping information. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override void ConvertBamlToObject ( BamlRecordReader reader, // Current reader that is processing records BamlRecord bamlRecord, // Record read in that triggered serializer ParserContext context) // Context { Debug.Assert(reader.MapTable==context.MapTable); TemplateTreeBuilderBamlTranslator treeBuilder = new TemplateTreeBuilderBamlTranslator(context, reader.BamlStream, reader.PreParsedRecordsStart, reader.PreParsedCurrentRecord, reader.ContextStack, reader.RootList, reader.XamlParseMode, reader.MaxAsyncRecords); // Process the passed record, which is presumably the start tag. This // must be processed out-of-line, since the previous reader has already retrieved it // from the baml stream treeBuilder.RecordReader.ReadRecord(bamlRecord); // Now process the contents of the Template block after the start tag. treeBuilder.Parse(); // Have the original TreeBuilder catch up with the parsed records, since we used a // new treebuilder for this portion of the parsing. reader.PreParsedCurrentRecord = treeBuilder.RecordReader.PreParsedCurrentRecord; } ////// If the Template represented by a group of baml records is stored in a dictionary, this /// method will extract the key used for this dictionary from the passed /// collection of baml records. For ControlTemplate, this is the styleTargetType. /// For DataTemplate, this is the DataTemplateKey containing the DataType. /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.InheritanceDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal override object GetDictionaryKey(BamlRecord startRecord, ParserContext parserContext) { object key = null; int numberOfElements = 0; BamlRecord record = startRecord; short ownerTypeId = 0; while (record != null) { if (record.RecordType == BamlRecordType.ElementStart) { BamlElementStartRecord elementStart = record as BamlElementStartRecord; if (++numberOfElements == 1) { // save the type ID of the first element (i.e.) ownerTypeId = elementStart.TypeId; } else { // We didn't find the key before a reading the // VisualTree nodes of the template break; } } else if (record.RecordType == BamlRecordType.Property && numberOfElements == 1) { // look for the TargetType property on the element // or the DataType property on the tag or // TableTemplate.Tree or the tag break; } record = record.Next; } if (key == null) { ThrowException(SRID.StyleNoDictionaryKey, parserContext.LineNumber, parserContext.LinePosition, null); } return key; } // Helper to insert line and position numbers into message, if they are present void ThrowException( string id, int lineNumber, int linePosition, Exception innerException) { string message = SR.Get(id); XamlParseException parseException; // Throw the appropriate execption. If we have line numbers, then we are // parsing a xaml file, so throw a xaml exception. Otherwise were are // parsing a baml file. if (lineNumber > 0) { message += " "; message += SR.Get(SRID.ParserLineAndOffset, lineNumber.ToString(CultureInfo.CurrentUICulture), linePosition.ToString(CultureInfo.CurrentUICulture)); parseException = new XamlParseException(message, lineNumber, linePosition); } else { parseException = new XamlParseException(message); } throw parseException; } #endif // !PBTCOMPILER #endregion OtherConversions #region Data private ParserHooks _parserHooks = null; // Constants used for emitting specific properties and attributes for a Style internal const string ControlTemplateTagName = "ControlTemplate"; internal const string DataTemplateTagName = "DataTemplate"; internal const string HierarchicalDataTemplateTagName = "HierarchicalDataTemplate"; internal const string ItemsPanelTemplateTagName = "ItemsPanelTemplate"; internal const string TargetTypePropertyName = "TargetType"; internal const string DataTypePropertyName = "DataType"; internal const string TriggersPropertyName = "Triggers"; internal const string ResourcesPropertyName = "Resources"; internal const string SettersPropertyName = "Setters"; internal const string ItemsSourcePropertyName = "ItemsSource"; internal const string ItemTemplatePropertyName = "ItemTemplate"; internal const string ItemTemplateSelectorPropertyName = "ItemTemplateSelector"; internal const string ItemContainerStylePropertyName = "ItemContainerStyle"; internal const string ItemContainerStyleSelectorPropertyName = "ItemContainerStyleSelector"; internal const string ItemStringFormatPropertyName = "ItemStringFormat"; internal const string ItemBindingGroupPropertyName = "ItemBindingGroup"; internal const string AlternationCountPropertyName = "AlternationCount"; internal const string ControlTemplateTriggersFullPropertyName = ControlTemplateTagName + "." + TriggersPropertyName; internal const string ControlTemplateResourcesFullPropertyName = ControlTemplateTagName + "." + ResourcesPropertyName; internal const string DataTemplateTriggersFullPropertyName = DataTemplateTagName + "." + TriggersPropertyName; internal const string DataTemplateResourcesFullPropertyName = DataTemplateTagName + "." + ResourcesPropertyName; internal const string HierarchicalDataTemplateTriggersFullPropertyName = HierarchicalDataTemplateTagName + "." + TriggersPropertyName; internal const string HierarchicalDataTemplateItemsSourceFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemsSourcePropertyName; internal const string HierarchicalDataTemplateItemTemplateFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemTemplatePropertyName; internal const string HierarchicalDataTemplateItemTemplateSelectorFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemTemplateSelectorPropertyName; internal const string HierarchicalDataTemplateItemContainerStyleFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemContainerStylePropertyName; internal const string HierarchicalDataTemplateItemContainerStyleSelectorFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemContainerStyleSelectorPropertyName; internal const string HierarchicalDataTemplateItemStringFormatFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemStringFormatPropertyName; internal const string HierarchicalDataTemplateItemBindingGroupFullPropertyName = HierarchicalDataTemplateTagName + "." + ItemBindingGroupPropertyName; internal const string HierarchicalDataTemplateAlternationCountFullPropertyName = HierarchicalDataTemplateTagName + "." + AlternationCountPropertyName; internal const string PropertyTriggerPropertyName = "Property"; internal const string PropertyTriggerValuePropertyName = "Value"; internal const string PropertyTriggerSourceName = "SourceName"; internal const string PropertyTriggerEnterActions = "EnterActions"; internal const string PropertyTriggerExitActions = "ExitActions"; internal const string DataTriggerBindingPropertyName = "Binding"; internal const string EventTriggerEventName = "RoutedEvent"; internal const string EventTriggerSourceName = "SourceName"; internal const string EventTriggerActions = "Actions"; internal const string MultiPropertyTriggerConditionsPropertyName = "Conditions"; internal const string SetterTagName = "Setter"; internal const string SetterPropertyAttributeName = "Property"; internal const string SetterValueAttributeName = "Value"; internal const string SetterTargetAttributeName = "TargetName"; internal const string SetterEventAttributeName = "Event"; internal const string SetterHandlerAttributeName = "Handler"; #if HANDLEDEVENTSTOO internal const string SetterHandledEventsTooAttributeName = "HandledEventsToo"; #endif #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.element BamlPropertyRecord propertyRecord = record as BamlPropertyRecord; short attributeOwnerTypeId; string attributeName; BamlAttributeUsage attributeUsage; parserContext.MapTable.GetAttributeInfoFromId(propertyRecord.AttributeId, out attributeOwnerTypeId, out attributeName, out attributeUsage); if (attributeOwnerTypeId == ownerTypeId) { if (attributeName == TargetTypePropertyName) { key = parserContext.XamlTypeMapper.GetDictionaryKey(propertyRecord.Value, parserContext); } else if (attributeName == DataTypePropertyName) { object dataType = parserContext.XamlTypeMapper.GetDictionaryKey(propertyRecord.Value, parserContext); Exception ex = TemplateKey.ValidateDataType(dataType, null); if (ex != null) { ThrowException(SRID.TemplateBadDictionaryKey, parserContext.LineNumber, parserContext.LinePosition, ex); } // key = new DataTemplateKey(dataType); } } } else if (record.RecordType == BamlRecordType.PropertyComplexStart || record.RecordType == BamlRecordType.PropertyIListStart || record.RecordType == BamlRecordType.ElementEnd) { // We didn't find the targetType before a complex property like // FrameworkTemplate.VisualTree or the
Link Menu
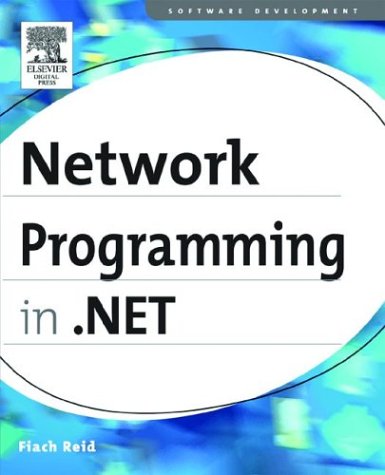
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlHostingConfiguration.cs
- PopupControlService.cs
- SqlConnectionHelper.cs
- DBAsyncResult.cs
- ListControl.cs
- SystemSounds.cs
- ParsedAttributeCollection.cs
- StylusPointPropertyInfo.cs
- TransactionValidationBehavior.cs
- WebPartsSection.cs
- DbParameterCollection.cs
- TitleStyle.cs
- RegistryPermission.cs
- DesignTimeXamlWriter.cs
- ExternalFile.cs
- DragAssistanceManager.cs
- PromptEventArgs.cs
- QuestionEventArgs.cs
- ByteArrayHelperWithString.cs
- XmlValidatingReaderImpl.cs
- MatrixAnimationUsingPath.cs
- OleDbRowUpdatingEvent.cs
- ImportedNamespaceContextItem.cs
- XmlSchemaSimpleTypeRestriction.cs
- XmlValidatingReaderImpl.cs
- MsmqOutputChannel.cs
- DependencyPropertyKey.cs
- UriScheme.cs
- ProcessModuleDesigner.cs
- SimplePropertyEntry.cs
- GenericEnumerator.cs
- SelectedDatesCollection.cs
- _Win32.cs
- AnnotationHighlightLayer.cs
- TextElementAutomationPeer.cs
- XmlSerializerOperationFormatter.cs
- DbConnectionPoolIdentity.cs
- SequentialUshortCollection.cs
- SamlAction.cs
- TreeNodeCollection.cs
- WebColorConverter.cs
- CalendarDateRange.cs
- ObjectDataSource.cs
- HttpServerUtilityWrapper.cs
- HWStack.cs
- DistinctQueryOperator.cs
- RequestStatusBarUpdateEventArgs.cs
- DataError.cs
- DataGridViewImageColumn.cs
- CookieProtection.cs
- IsolatedStorageSecurityState.cs
- CssClassPropertyAttribute.cs
- PenCursorManager.cs
- DataMemberAttribute.cs
- HtmlTableRow.cs
- EntityCommand.cs
- SimplePropertyEntry.cs
- XmlName.cs
- x509utils.cs
- DesignBindingConverter.cs
- SystemResources.cs
- DetailsViewRowCollection.cs
- ManifestResourceInfo.cs
- CertificateManager.cs
- CapabilitiesState.cs
- SqlConnection.cs
- UnaryExpression.cs
- ToolStripRenderEventArgs.cs
- ItemsChangedEventArgs.cs
- EventLogPermissionEntry.cs
- CodeConstructor.cs
- ComponentChangingEvent.cs
- DataGridViewMethods.cs
- PasswordBoxAutomationPeer.cs
- Rfc4050KeyFormatter.cs
- AppliedDeviceFiltersEditor.cs
- HttpVersion.cs
- SqlTransaction.cs
- LoginUtil.cs
- ActivityBindForm.cs
- ClientType.cs
- RegistrySecurity.cs
- BuildProvidersCompiler.cs
- InstancePersistenceException.cs
- ObsoleteAttribute.cs
- Transform3DCollection.cs
- NullableDoubleMinMaxAggregationOperator.cs
- RepeaterDesigner.cs
- InlineUIContainer.cs
- DrawingState.cs
- WebControl.cs
- DBCommand.cs
- WeakHashtable.cs
- TypeCollectionPropertyEditor.cs
- ConfigurationSectionGroupCollection.cs
- initElementDictionary.cs
- XamlParser.cs
- _NegoStream.cs
- CommentEmitter.cs
- IHttpResponseInternal.cs