Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / __ComObject.cs / 1 / __ComObject.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: __ComObject ** ** ** __ComObject is the root class for all COM wrappers. This class ** defines only the basics. This class is used for wrapping COM objects ** accessed from COM+ ** ** ===========================================================*/ namespace System { using System; using System.Collections; using System.Threading; using System.Runtime.InteropServices; using System.Reflection; using System.Security.Permissions; internal class __ComObject : MarshalByRefObject { private Hashtable m_ObjectToDataMap; /*=========================================================== ** default constructor ** can't instantiate this directly =============================================================*/ private __ComObject () { } internal IntPtr GetIUnknown(out bool fIsURTAggregated) { fIsURTAggregated = !GetType().IsDefined(typeof(ComImportAttribute), false); return System.Runtime.InteropServices.Marshal.GetIUnknownForObject(this); } //=================================================================== // This method retrieves the data associated with the specified // key if any such data exists for the current __ComObject. //=================================================================== internal Object GetData(Object key) { Object data = null; // Synchronize access to the map. lock(this) { // If the map hasn't been allocated, then there can be no data for the specified key. if (m_ObjectToDataMap != null) { // Look up the data in the map. data = m_ObjectToDataMap[key]; } } return data; } //==================================================================== // This method sets the data for the specified key on the current // __ComObject. //=================================================================== internal bool SetData(Object key, Object data) { bool bAdded = false; // Synchronize access to the map. lock(this) { // If the map hasn't been allocated yet, allocate it. if (m_ObjectToDataMap == null) m_ObjectToDataMap = new Hashtable(); // If there isn't already data in the map then add it. if (m_ObjectToDataMap[key] == null) { m_ObjectToDataMap[key] = data; bAdded = true; } } return bAdded; } //==================================================================== // This method is called from within the EE and releases all the // cached data for the __ComObject. //==================================================================== internal void ReleaseAllData() { // Synchronize access to the map. lock(this) { // If the map hasn't been allocated, then there is nothing to do. if (m_ObjectToDataMap != null) { foreach (Object o in m_ObjectToDataMap.Values) { // If the object implements IDisposable, then call Dispose on it. IDisposable DisposableObj = o as IDisposable; if (DisposableObj != null) DisposableObj.Dispose(); // If the object is a derived from __ComObject, then call Marshal.ReleaseComObject on it. __ComObject ComObj = o as __ComObject; if (ComObj != null) Marshal.ReleaseComObject(ComObj); } // Set the map to null to indicate it has been cleaned up. m_ObjectToDataMap = null; } } } //=================================================================== // This method is called from within the EE and is used to handle // calls on methods of event interfaces. //==================================================================== internal Object GetEventProvider(Type t) { // Check to see if we already have a cached event provider for this type. Object EvProvider = GetData(t); // If we don't then we need to create one. if (EvProvider == null) EvProvider = CreateEventProvider(t); return EvProvider; } internal int ReleaseSelf() { return Marshal.InternalReleaseComObject(this); } internal void FinalReleaseSelf() { Marshal.InternalFinalReleaseComObject(this); } [ReflectionPermissionAttribute(SecurityAction.Assert, MemberAccess=true)] private Object CreateEventProvider(Type t) { // Create the event provider for the specified type. Object EvProvider = Activator.CreateInstance(t, Activator.ConstructorDefault | BindingFlags.NonPublic, null, new Object[]{this}, null); // Attempt to cache the wrapper on the object. if (!SetData(t, EvProvider)) { // Dispose the event provider if it implements IDisposable. IDisposable DisposableEvProv = EvProvider as IDisposable; if (DisposableEvProv != null) DisposableEvProv.Dispose(); // Another thead already cached the wrapper so use that one instead. EvProvider = GetData(t); } return EvProvider; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: __ComObject ** ** ** __ComObject is the root class for all COM wrappers. This class ** defines only the basics. This class is used for wrapping COM objects ** accessed from COM+ ** ** ===========================================================*/ namespace System { using System; using System.Collections; using System.Threading; using System.Runtime.InteropServices; using System.Reflection; using System.Security.Permissions; internal class __ComObject : MarshalByRefObject { private Hashtable m_ObjectToDataMap; /*=========================================================== ** default constructor ** can't instantiate this directly =============================================================*/ private __ComObject () { } internal IntPtr GetIUnknown(out bool fIsURTAggregated) { fIsURTAggregated = !GetType().IsDefined(typeof(ComImportAttribute), false); return System.Runtime.InteropServices.Marshal.GetIUnknownForObject(this); } //=================================================================== // This method retrieves the data associated with the specified // key if any such data exists for the current __ComObject. //=================================================================== internal Object GetData(Object key) { Object data = null; // Synchronize access to the map. lock(this) { // If the map hasn't been allocated, then there can be no data for the specified key. if (m_ObjectToDataMap != null) { // Look up the data in the map. data = m_ObjectToDataMap[key]; } } return data; } //==================================================================== // This method sets the data for the specified key on the current // __ComObject. //=================================================================== internal bool SetData(Object key, Object data) { bool bAdded = false; // Synchronize access to the map. lock(this) { // If the map hasn't been allocated yet, allocate it. if (m_ObjectToDataMap == null) m_ObjectToDataMap = new Hashtable(); // If there isn't already data in the map then add it. if (m_ObjectToDataMap[key] == null) { m_ObjectToDataMap[key] = data; bAdded = true; } } return bAdded; } //==================================================================== // This method is called from within the EE and releases all the // cached data for the __ComObject. //==================================================================== internal void ReleaseAllData() { // Synchronize access to the map. lock(this) { // If the map hasn't been allocated, then there is nothing to do. if (m_ObjectToDataMap != null) { foreach (Object o in m_ObjectToDataMap.Values) { // If the object implements IDisposable, then call Dispose on it. IDisposable DisposableObj = o as IDisposable; if (DisposableObj != null) DisposableObj.Dispose(); // If the object is a derived from __ComObject, then call Marshal.ReleaseComObject on it. __ComObject ComObj = o as __ComObject; if (ComObj != null) Marshal.ReleaseComObject(ComObj); } // Set the map to null to indicate it has been cleaned up. m_ObjectToDataMap = null; } } } //=================================================================== // This method is called from within the EE and is used to handle // calls on methods of event interfaces. //==================================================================== internal Object GetEventProvider(Type t) { // Check to see if we already have a cached event provider for this type. Object EvProvider = GetData(t); // If we don't then we need to create one. if (EvProvider == null) EvProvider = CreateEventProvider(t); return EvProvider; } internal int ReleaseSelf() { return Marshal.InternalReleaseComObject(this); } internal void FinalReleaseSelf() { Marshal.InternalFinalReleaseComObject(this); } [ReflectionPermissionAttribute(SecurityAction.Assert, MemberAccess=true)] private Object CreateEventProvider(Type t) { // Create the event provider for the specified type. Object EvProvider = Activator.CreateInstance(t, Activator.ConstructorDefault | BindingFlags.NonPublic, null, new Object[]{this}, null); // Attempt to cache the wrapper on the object. if (!SetData(t, EvProvider)) { // Dispose the event provider if it implements IDisposable. IDisposable DisposableEvProv = EvProvider as IDisposable; if (DisposableEvProv != null) DisposableEvProv.Dispose(); // Another thead already cached the wrapper so use that one instead. EvProvider = GetData(t); } return EvProvider; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
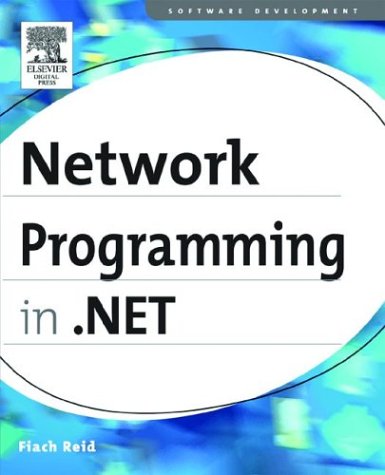
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultValueTypeConverter.cs
- Vector3DKeyFrameCollection.cs
- NoPersistProperty.cs
- AudioFormatConverter.cs
- HtmlMeta.cs
- relpropertyhelper.cs
- ManagementOptions.cs
- HashCodeCombiner.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- ConfigurationManagerHelper.cs
- Attributes.cs
- PolicyImporterElement.cs
- SqlGatherProducedAliases.cs
- QuaternionAnimationUsingKeyFrames.cs
- TextServicesDisplayAttribute.cs
- ListViewHitTestInfo.cs
- EventListener.cs
- BufferedWebEventProvider.cs
- IntSecurity.cs
- ToolStripLabel.cs
- DefaultValueAttribute.cs
- SmuggledIUnknown.cs
- CatalogPartChrome.cs
- XmlParserContext.cs
- Span.cs
- WebRequestModuleElementCollection.cs
- RegexWriter.cs
- XmlSerializationReader.cs
- SByte.cs
- ThrowHelper.cs
- VirtualPathProvider.cs
- SafeThreadHandle.cs
- AsyncCompletedEventArgs.cs
- KeyEvent.cs
- MouseActionValueSerializer.cs
- LinearKeyFrames.cs
- BindingMAnagerBase.cs
- ExpressionHelper.cs
- CompModSwitches.cs
- XmlEnumAttribute.cs
- SqlInternalConnection.cs
- DBBindings.cs
- LocalizableResourceBuilder.cs
- Polygon.cs
- WindowsTooltip.cs
- HtmlTableCell.cs
- _KerberosClient.cs
- WindowsStartMenu.cs
- HttpInputStream.cs
- HtmlInputText.cs
- FormattedText.cs
- ProfileBuildProvider.cs
- sqlpipe.cs
- GridViewRow.cs
- PartialCachingControl.cs
- GenericParameterDataContract.cs
- PathFigureCollection.cs
- ProtectedConfigurationProviderCollection.cs
- DynamicVirtualDiscoSearcher.cs
- InternalUserCancelledException.cs
- PrinterSettings.cs
- RolePrincipal.cs
- XmlWrappingReader.cs
- SecurityKeyIdentifier.cs
- QilPatternFactory.cs
- XmlStreamStore.cs
- CodeDelegateCreateExpression.cs
- HyperlinkAutomationPeer.cs
- RepeaterItemCollection.cs
- TriggerActionCollection.cs
- XmlDataSourceNodeDescriptor.cs
- EventPropertyMap.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- AuthorizationRuleCollection.cs
- QueryInterceptorAttribute.cs
- StreamGeometry.cs
- XsltException.cs
- TemplatePropertyEntry.cs
- DataConnectionHelper.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- ListViewDeleteEventArgs.cs
- XDeferredAxisSource.cs
- ElementProxy.cs
- SqlLiftWhereClauses.cs
- PageDeviceFont.cs
- NonSerializedAttribute.cs
- FileDialog_Vista.cs
- Calendar.cs
- XmlRootAttribute.cs
- LayoutUtils.cs
- HttpValueCollection.cs
- HostingEnvironmentWrapper.cs
- DocumentViewerAutomationPeer.cs
- DSASignatureDeformatter.cs
- TextFormatterHost.cs
- AssemblySettingAttributes.cs
- DataGridViewSortCompareEventArgs.cs
- ListViewSortEventArgs.cs
- SByte.cs
- PerfService.cs