Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / Printing / PreviewPrintController.cs / 1 / PreviewPrintController.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Internal; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Runtime.InteropServices; using CodeAccessPermission = System.Security.CodeAccessPermission; ////// /// A PrintController which "prints" to a series of images. /// public class PreviewPrintController : PrintController { private IList list = new ArrayList(); // list of PreviewPageInfo private System.Drawing.Graphics graphics; private DeviceContext dc; private bool antiAlias; private void CheckSecurity() { IntSecurity.SafePrinting.Demand(); } ////// /// public override bool IsPreview { get { return true; } } ////// This is new public property which notifies if this controller is used for PrintPreview. /// ////// /// public override void OnStartPrint(PrintDocument document, PrintEventArgs e) { Debug.Assert(dc == null && graphics == null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); base.OnStartPrint(document, e); try { if (!document.PrinterSettings.IsValid) throw new InvalidPrinterException(document.PrinterSettings); IntSecurity.AllPrintingAndUnmanagedCode.Assert(); // We need a DC as a reference; we don't actually draw on it. // We make sure to reuse the same one to improve performance. dc = document.PrinterSettings.CreateInformationContext(modeHandle); } finally { CodeAccessPermission.RevertAssert(); } } ////// Implements StartPrint for generating print preview information. /// ////// /// public override Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { Debug.Assert(dc != null && graphics == null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); base.OnStartPage(document, e); try { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); e.PageSettings.CopyToHdevmode(modeHandle); Size size = e.PageBounds.Size; // Metafile framing rectangles apparently use hundredths of mm as their unit of measurement, // instead of the GDI+ standard hundredth of an inch. Size metafileSize = PrinterUnitConvert.Convert(size, PrinterUnit.Display, PrinterUnit.HundredthsOfAMillimeter); // Create a Metafile which accepts only GDI+ commands since we are the ones creating // and using this ... // Framework creates a dual-mode EMF for each page in the preview. // When these images are displayed in preview, // they are added to the dual-mode EMF. However, // GDI+ breaks during this process if the image // is sufficiently large and has more than 254 colors. // This code path can easily be avoided by requesting // an EmfPlusOnly EMF.. Metafile metafile = new Metafile(dc.Hdc, new Rectangle(0,0, metafileSize.Width, metafileSize.Height), MetafileFrameUnit.GdiCompatible, EmfType.EmfPlusOnly); PreviewPageInfo info = new PreviewPageInfo(metafile, size); list.Add(info); PrintPreviewGraphics printGraphics = new PrintPreviewGraphics(document, e); graphics = Graphics.FromImage(metafile); if (graphics != null && document.OriginAtMargins) { // Adjust the origin of the graphics object to be at the // user-specified margin location // int dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSX); int dpiY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSY); int hardMarginX_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETX); int hardMarginY_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETY); float hardMarginX = hardMarginX_DU * 100 / dpiX; float hardMarginY = hardMarginY_DU * 100 / dpiY; graphics.TranslateTransform(-hardMarginX, -hardMarginY); graphics.TranslateTransform(document.DefaultPageSettings.Margins.Left, document.DefaultPageSettings.Margins.Top); } graphics.PrintingHelper = printGraphics; if (antiAlias) { graphics.TextRenderingHint = TextRenderingHint.AntiAlias; graphics.SmoothingMode = SmoothingMode.AntiAlias; } } finally { CodeAccessPermission.RevertAssert(); } return graphics; } ////// Implements StartEnd for generating print preview information. /// ////// /// public override void OnEndPage(PrintDocument document, PrintPageEventArgs e) { Debug.Assert(dc != null && graphics != null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); graphics.Dispose(); graphics = null; base.OnEndPage(document, e); } ////// Implements EndPage for generating print preview information. /// ////// /// public override void OnEndPrint(PrintDocument document, PrintEventArgs e) { Debug.Assert(dc != null && graphics == null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods are called in any particular order CheckSecurity(); dc.Dispose(); dc = null; base.OnEndPrint(document, e); } ////// Implements EndPrint for generating print preview information. /// ////// /// public PreviewPageInfo[] GetPreviewPageInfo() { // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); PreviewPageInfo[] temp = new PreviewPageInfo[list.Count]; list.CopyTo(temp, 0); return temp; } ////// The "printout". /// ///public virtual bool UseAntiAlias { get { return antiAlias; } set { antiAlias = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Internal; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Runtime.InteropServices; using CodeAccessPermission = System.Security.CodeAccessPermission; ////// /// A PrintController which "prints" to a series of images. /// public class PreviewPrintController : PrintController { private IList list = new ArrayList(); // list of PreviewPageInfo private System.Drawing.Graphics graphics; private DeviceContext dc; private bool antiAlias; private void CheckSecurity() { IntSecurity.SafePrinting.Demand(); } ////// /// public override bool IsPreview { get { return true; } } ////// This is new public property which notifies if this controller is used for PrintPreview. /// ////// /// public override void OnStartPrint(PrintDocument document, PrintEventArgs e) { Debug.Assert(dc == null && graphics == null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); base.OnStartPrint(document, e); try { if (!document.PrinterSettings.IsValid) throw new InvalidPrinterException(document.PrinterSettings); IntSecurity.AllPrintingAndUnmanagedCode.Assert(); // We need a DC as a reference; we don't actually draw on it. // We make sure to reuse the same one to improve performance. dc = document.PrinterSettings.CreateInformationContext(modeHandle); } finally { CodeAccessPermission.RevertAssert(); } } ////// Implements StartPrint for generating print preview information. /// ////// /// public override Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { Debug.Assert(dc != null && graphics == null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); base.OnStartPage(document, e); try { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); e.PageSettings.CopyToHdevmode(modeHandle); Size size = e.PageBounds.Size; // Metafile framing rectangles apparently use hundredths of mm as their unit of measurement, // instead of the GDI+ standard hundredth of an inch. Size metafileSize = PrinterUnitConvert.Convert(size, PrinterUnit.Display, PrinterUnit.HundredthsOfAMillimeter); // Create a Metafile which accepts only GDI+ commands since we are the ones creating // and using this ... // Framework creates a dual-mode EMF for each page in the preview. // When these images are displayed in preview, // they are added to the dual-mode EMF. However, // GDI+ breaks during this process if the image // is sufficiently large and has more than 254 colors. // This code path can easily be avoided by requesting // an EmfPlusOnly EMF.. Metafile metafile = new Metafile(dc.Hdc, new Rectangle(0,0, metafileSize.Width, metafileSize.Height), MetafileFrameUnit.GdiCompatible, EmfType.EmfPlusOnly); PreviewPageInfo info = new PreviewPageInfo(metafile, size); list.Add(info); PrintPreviewGraphics printGraphics = new PrintPreviewGraphics(document, e); graphics = Graphics.FromImage(metafile); if (graphics != null && document.OriginAtMargins) { // Adjust the origin of the graphics object to be at the // user-specified margin location // int dpiX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSX); int dpiY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.LOGPIXELSY); int hardMarginX_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETX); int hardMarginY_DU = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(dc, dc.Hdc), SafeNativeMethods.PHYSICALOFFSETY); float hardMarginX = hardMarginX_DU * 100 / dpiX; float hardMarginY = hardMarginY_DU * 100 / dpiY; graphics.TranslateTransform(-hardMarginX, -hardMarginY); graphics.TranslateTransform(document.DefaultPageSettings.Margins.Left, document.DefaultPageSettings.Margins.Top); } graphics.PrintingHelper = printGraphics; if (antiAlias) { graphics.TextRenderingHint = TextRenderingHint.AntiAlias; graphics.SmoothingMode = SmoothingMode.AntiAlias; } } finally { CodeAccessPermission.RevertAssert(); } return graphics; } ////// Implements StartEnd for generating print preview information. /// ////// /// public override void OnEndPage(PrintDocument document, PrintPageEventArgs e) { Debug.Assert(dc != null && graphics != null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); graphics.Dispose(); graphics = null; base.OnEndPage(document, e); } ////// Implements EndPage for generating print preview information. /// ////// /// public override void OnEndPrint(PrintDocument document, PrintEventArgs e) { Debug.Assert(dc != null && graphics == null, "PrintController methods called in the wrong order?"); // For security purposes, don't assume our public methods are called in any particular order CheckSecurity(); dc.Dispose(); dc = null; base.OnEndPrint(document, e); } ////// Implements EndPrint for generating print preview information. /// ////// /// public PreviewPageInfo[] GetPreviewPageInfo() { // For security purposes, don't assume our public methods methods are called in any particular order CheckSecurity(); PreviewPageInfo[] temp = new PreviewPageInfo[list.Count]; list.CopyTo(temp, 0); return temp; } ////// The "printout". /// ///public virtual bool UseAntiAlias { get { return antiAlias; } set { antiAlias = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
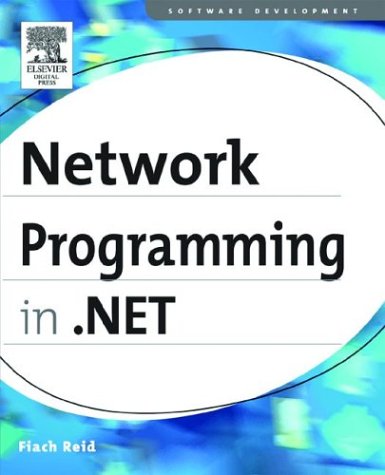
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewRowHeaderCell.cs
- StrokeIntersection.cs
- SqlClientPermission.cs
- Utils.cs
- TypeUtils.cs
- ActivityCodeDomSerializer.cs
- ProcessHostConfigUtils.cs
- SurrogateEncoder.cs
- UIElementHelper.cs
- SetterBaseCollection.cs
- RequestCachingSection.cs
- IndexerNameAttribute.cs
- OleDbPermission.cs
- Tuple.cs
- PageCopyCount.cs
- SystemResourceHost.cs
- DeferredReference.cs
- AccessText.cs
- InternalConfigRoot.cs
- QueryStringParameter.cs
- webbrowsersite.cs
- ClientTarget.cs
- DynamicMetaObject.cs
- XmlSchemaChoice.cs
- SortedList.cs
- ContentElement.cs
- PageAdapter.cs
- AspNetRouteServiceHttpHandler.cs
- COM2Properties.cs
- KeyFrames.cs
- DataGridViewRow.cs
- SettingsBase.cs
- AutomationPropertyInfo.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- TagMapInfo.cs
- DesignerActionService.cs
- ReceiveParametersContent.cs
- ColorAnimation.cs
- ResetableIterator.cs
- ListDictionary.cs
- SrgsSubset.cs
- FakeModelItemImpl.cs
- NotSupportedException.cs
- SystemFonts.cs
- GeometryModel3D.cs
- DesignConnection.cs
- Underline.cs
- MorphHelper.cs
- PiiTraceSource.cs
- SspiHelper.cs
- SelectionProviderWrapper.cs
- TextElement.cs
- SamlSerializer.cs
- AddInServer.cs
- DbProviderSpecificTypePropertyAttribute.cs
- OutputScope.cs
- RenderData.cs
- ListViewInsertEventArgs.cs
- ExitEventArgs.cs
- ChtmlPhoneCallAdapter.cs
- CompilerGlobalScopeAttribute.cs
- DataServicePagingProviderWrapper.cs
- MemberMaps.cs
- IntSecurity.cs
- DoWorkEventArgs.cs
- PersonalizablePropertyEntry.cs
- FrameworkObject.cs
- OdbcTransaction.cs
- Package.cs
- OdbcFactory.cs
- SystemIcmpV4Statistics.cs
- HtmlForm.cs
- PathFigureCollection.cs
- XAMLParseException.cs
- BaseDataBoundControl.cs
- PkcsMisc.cs
- Typography.cs
- XmlCDATASection.cs
- MouseActionValueSerializer.cs
- SqlParameterizer.cs
- COM2Enum.cs
- UInt64Storage.cs
- LogicalTreeHelper.cs
- NameValueFileSectionHandler.cs
- PrimitiveXmlSerializers.cs
- TableCell.cs
- TemplateInstanceAttribute.cs
- UseLicense.cs
- ServiceHttpHandlerFactory.cs
- LayoutUtils.cs
- recordstatescratchpad.cs
- BinaryObjectWriter.cs
- EmbeddedMailObject.cs
- RedirectionProxy.cs
- DataControlImageButton.cs
- Substitution.cs
- SystemException.cs
- XmlMembersMapping.cs
- WebPartDeleteVerb.cs
- ProxyElement.cs