Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / DataOracleClient / System / Data / OracleClient / TracedNativeMethods.cs / 1 / TracedNativeMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Text; using System.Runtime.ConstrainedExecution; static internal class TracedNativeMethods { //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OraMTSEnlCtxGet ( byte[] userName, byte[] password, byte[] serverName, OciHandle pOCISvc, OciHandle pOCIErr, out IntPtr pCtxt // can't return a HandleRef ) { int rc; RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { if (Bid.AdvancedOn){ Bid.Trace("userName=..., password=..., serverName=..., pOCISvc=0x%-07Ix pOCIErr=0x%-07Ix dwFlags=0x%08X\n", OciHandle.HandleValueToTrace(pOCISvc), OciHandle.HandleValueToTrace(pOCIErr), 0 ); } rc = UnsafeNativeMethods.OraMTSEnlCtxGet(userName, password, serverName, pOCISvc, pOCIErr, 0, out pCtxt ); if (Bid.AdvancedOn){ Bid.Trace(" pCtxt=0x%-07Ix rc=%d\n", pCtxt, rc); } } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OraMTSEnlCtxRel ( IntPtr pCtxt ) { int rc; RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { if (Bid.AdvancedOn){ Bid.Trace(" pCtxt=%Id\n", pCtxt); } rc = UnsafeNativeMethods.OraMTSEnlCtxRel(pCtxt); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } } return rc; } //---------------------------------------------------------------------- static internal int OraMTSOCIErrGet ( ref int dwErr, NativeBuffer lpcEMsg, ref int lpdLen ) { if (Bid.AdvancedOn){ Bid.Trace(" dwErr=%08X, lpcEMsg=0x%-07Ix lpdLen=%d\n", dwErr, NativeBuffer.HandleValueToTrace(lpcEMsg), lpdLen ); } int rc = UnsafeNativeMethods.OraMTSOCIErrGet(ref dwErr,lpcEMsg,ref lpdLen); if (Bid.AdvancedOn){ if (0 == rc) { Bid.Trace(" rc=%d\n", rc); } else { string message = lpcEMsg.PtrToStringAnsi(0, lpdLen); Bid.Trace(" rd=%d message='%ls', lpdLen=%d\n", rc, message, lpdLen); } } return rc; } //--------------------------------------------------------------------- static internal int OraMTSJoinTxn ( OciEnlistContext pCtxt, System.Transactions.IDtcTransaction pTrans ) { if (Bid.AdvancedOn){ Bid.Trace(" pCtxt=0x%-07Ix pTrans=...\n", OciEnlistContext.HandleValueToTrace(pCtxt)); } int rc = UnsafeNativeMethods.OraMTSJoinTxn(pCtxt, pTrans); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int oermsg ( short rcode, NativeBuffer buf ) { if (Bid.AdvancedOn){ Bid.Trace(" rcode=%d\n", (int)rcode); } int rc = UnsafeNativeMethods.oermsg(rcode, buf); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } #if ADVANCEDTRACING //---------------------------------------------------------------------- static internal string GetAttributeName ( OciHandle handle, OCI.ATTR atype ) { if (OCI.HTYPE.OCI_DTYPE_PARAM == handle.HandleType) return ((OCI.PATTR)atype).ToString(); return atype.ToString(); } #endif //ADVANCEDTRACING //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, ref IntPtr attributep, // Used to get strings; can't return a handle ref! ref uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, ref attributep, ref sizep, attrtype, errhp); if (Bid.AdvancedOn) { if (OCI.ATTR.OCI_ATTR_NAME == attrtype) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20ls sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, trgthndlp.PtrToString(attributep, checked((int)sizep)), sizep, rc ); } else { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=0x%-18Ix sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, attributep, sizep, rc ); } } return rc; } //---------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, out byte attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int attribute = 0; int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, out attribute, out sizep, attrtype, errhp); attributep = unchecked((byte)attribute); if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20d sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, (int)attributep, sizep, rc ); } return rc; } //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, out short attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int attribute = 0; int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, out attribute, out sizep, attrtype, errhp); attributep = unchecked((short)attribute); if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20d sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, (int)attributep, sizep, rc ); } return rc; } //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, out int attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, out attributep, out sizep, attrtype, errhp); if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20d sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, attributep, sizep, rc ); } return rc; } //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, OciHandle attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { // NOTE: we're updating the data that the handle points to, not the // handle value itself. int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, attributep, out sizep, attrtype, errhp); if (Bid.AdvancedOn) { // Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=0x%-18Ix sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, OciHandle.HandleValueToTrace(attributep), sizep, rc ); } return rc; } //---------------------------------------------------------------------- static internal int OCIAttrSet ( OciHandle trgthndlp, ref int attributep, uint size, OCI.ATTR attrtype, OciHandle errhp ) { if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attributep=%-9d size=%-2d attrtype=%-22ls errhp=0x%-07Ix\n", trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); } int rc = UnsafeNativeMethods.OCIAttrSet(trgthndlp, trgthndlp.HandleType, ref attributep, size, attrtype, errhp); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //--------------------------------------------------------------------- static internal int OCIAttrSet ( OciHandle trgthndlp, OciHandle attributep, uint size, OCI.ATTR attrtype, OciHandle errhp ) { if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attributep=0x%-07Ix size=%d attrtype=%-22ls errhp=0x%-07Ix\n", trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); } int rc = UnsafeNativeMethods.OCIAttrSet(trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- static internal int OCIAttrSet ( OciHandle trgthndlp, byte[] attributep, uint size, OCI.ATTR attrtype, OciHandle errhp ) { if (Bid.AdvancedOn) { string attributes; if (OCI.ATTR.OCI_ATTR_EXTERNAL_NAME == attrtype || OCI.ATTR.OCI_ATTR_INTERNAL_NAME == attrtype) { char[] temp = System.Text.Encoding.UTF8.GetChars(attributep, 0, checked((int)size)); attributes = new string(temp); } else { attributes = attributep.ToString(); } Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attributep='%ls' size=%d attrtype=%-22ls errhp=0x%-07Ix\n", trgthndlp, trgthndlp.HandleType, attributes, size, attrtype, errhp); } int rc = UnsafeNativeMethods.OCIAttrSet(trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- static internal int OCIBindByName ( OciHandle stmtp, out IntPtr bindpp, // can't return a handle ref! OciHandle errhp, string placeholder, int placeh_len, IntPtr valuep, int value_sz, OCI.DATATYPE dty, IntPtr indp, IntPtr alenp, //ub2* OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix placeholder=%-20ls placeh_len=%-2d valuep=0x%-07Ix value_sz=%-4d dty=%d{OCI.DATATYPE} indp=0x%-07Ix *indp=%-3d alenp=0x%-07Ix *alenp=%-4d rcodep=0x%-07Ix maxarr_len=%-4d curelap=0x%-07Ix mode=0x%x{OCI.MODE}\n", OciHandle.HandleValueToTrace(stmtp), OciHandle.HandleValueToTrace(errhp), placeholder, placeh_len, valuep, value_sz, (int)dty, indp, (int)(IntPtr.Zero == (IntPtr)indp ? (short)0 : Marshal.ReadInt16((IntPtr)indp)), alenp, (int)(IntPtr.Zero == (IntPtr)alenp? (short)0 : Marshal.ReadInt16((IntPtr)alenp)), IntPtr.Zero, 0, IntPtr.Zero, (int)mode ); } byte[] placeholderName = stmtp.GetBytes(placeholder); int placeholderNameLength = placeholderName.Length; int rc = UnsafeNativeMethods.OCIBindByName(stmtp, out bindpp, errhp, placeholderName, placeholderNameLength, valuep, value_sz, dty, indp, alenp, IntPtr.Zero, 0, IntPtr.Zero, mode); if (Bid.AdvancedOn){ Bid.Trace(" bindpp=0x%-07Ix rc=%d\n", bindpp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIDefineByPos ( OciHandle stmtp, out IntPtr hndlpp, // can't return a handle ref! OciHandle errhp, uint position, IntPtr valuep, int value_sz, OCI.DATATYPE dty, IntPtr indp, IntPtr rlenp, //ub2* IntPtr rcodep, //ub2* OCI.MODE mode ) { int rc = UnsafeNativeMethods.OCIDefineByPos(stmtp, out hndlpp, errhp, position, valuep, value_sz, dty, indp, rlenp, rcodep, mode); if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix position=%-2d valuep=0x%-07Ix value_sz=%-4d dty=%-3d %-14s indp=0x%-07Ix rlenp=0x%-07Ix rcodep=0x%-07Ix mode=0x%x{OCI.MODE} hndlpp=0x%-07Ix rc=%d\n", stmtp, errhp, position, valuep, value_sz, (int)dty, dty, indp, rlenp, rcodep, (int)mode, hndlpp, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIDefineArrayOfStruct ( OciHandle defnp, OciHandle errhp, uint pvskip, uint indskip, uint rlskip, uint rcskip ) { if (Bid.AdvancedOn){ Bid.Trace(" defnp=0x%-07Ix errhp=0x%-07Ix pvskip=%-4d indskip=%-4d rlskip=%-4d rcskip=%-4d\n", defnp, errhp, pvskip, indskip, rlskip, rcskip ); } int rc = UnsafeNativeMethods.OCIDefineArrayOfStruct(defnp, errhp, pvskip, indskip, rlskip, rcskip); if (0 != rc && Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIDefineDynamic ( OciHandle defnp, OciHandle errhp, IntPtr octxp, OCI.Callback.OCICallbackDefine ocbfp ) { if (Bid.AdvancedOn){ Bid.Trace(" defnp=0x%-07Ix errhp=0x%-07Ix octxp=0x%-07Ix ocbfp=...\n", OciHandle.HandleValueToTrace(defnp), OciHandle.HandleValueToTrace(errhp), octxp); // note: can't trace OCI.Callback.OCICallbackDefine } int rc = UnsafeNativeMethods.OCIDefineDynamic(defnp, errhp, octxp, ocbfp); if (0 != rc && Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIDescriptorAlloc ( OciHandle parenth, out IntPtr hndlpp, // can't return a handle ref! OCI.HTYPE type ) { int rc = UnsafeNativeMethods.OCIDescriptorAlloc(parenth, out hndlpp, type, 0, IntPtr.Zero); if (Bid.AdvancedOn) { Bid.Trace(" parenth=0x%-07Ix type=%3d xtramemsz=%d usrmempp=0x%-07Ix hndlpp=0x%-07Ix rc=%d\n", parenth, (int)type, 0, IntPtr.Zero, hndlpp, rc ); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OCIDescriptorFree ( IntPtr hndlp, OCI.HTYPE type ) { if (Bid.AdvancedOn) { Bid.Trace(" hndlp=0x%Id type=%3d\n", hndlp, (int)type ); } int rc = UnsafeNativeMethods.OCIDescriptorFree(hndlp, type); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIEnvCreate ( out IntPtr envhpp, // can't return a handle ref! OCI.MODE mode ) { if (Bid.AdvancedOn) { Bid.Trace(" mode=0x%x{OCI.MODE} ctxp=0x%-07Ix malocfp=0x%-07Ix ralocfp=0x%-07Ix mfreefp=0x%-07Ix xtramemsz=%d usrmempp=0x%-07Ix", (int)mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero ); } int rc = UnsafeNativeMethods.OCIEnvCreate(out envhpp, mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero); if (Bid.AdvancedOn) { Bid.Trace(" envhpp=0x%-07Ix, rc=%d\n", envhpp, rc ); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIEnvNlsCreate ( out IntPtr envhpp, // can't return a handle ref! OCI.MODE mode, ushort charset, ushort ncharset ) { if (Bid.AdvancedOn) { Bid.Trace(" mode=0x%x{OCI.MODE} ctxp=0x%-07Ix malocfp=0x%-07Ix ralocfp=0x%-07Ix mfreefp=0x%-07Ix xtramemsz=%d usrmempp=0x%-07Ix charset=%d ncharset=%d", (int)mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero, (int)charset, (int)ncharset ); } int rc = UnsafeNativeMethods.OCIEnvNlsCreate(out envhpp, mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero, charset, ncharset); if (Bid.AdvancedOn) { Bid.Trace(" envhpp=0x%-07Ix rc=%d\n", envhpp, rc ); } return rc; } //---------------------------------------------------------------------- static internal int OCIErrorGet ( OciHandle hndlp, int recordno, out int errcodep, NativeBuffer bufp ) { if (Bid.AdvancedOn){ Bid.Trace(" hndlp=0x%-07Ix recordno=%d sqlstate=0x%-07Ix bufp=0x%-07Ix bufsiz=%d type=%d{OCI.HTYPE}\n", OciHandle.HandleValueToTrace(hndlp), recordno, IntPtr.Zero, NativeBuffer.HandleValueToTrace(bufp), bufp.Length, (int)hndlp.HandleType ); } int rc = UnsafeNativeMethods.OCIErrorGet(hndlp, checked((uint)recordno), IntPtr.Zero, out errcodep, bufp, unchecked((uint)bufp.Length), hndlp.HandleType); if (Bid.AdvancedOn){ Bid.Trace(" errcodep=%d rc=%d\n\t%ls\n\n", errcodep, rc, hndlp.PtrToString(bufp)); } return rc; } //---------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIHandleAlloc ( OciHandle parenth, out IntPtr hndlpp, // can't return a handle ref! OCI.HTYPE type ) { int rc = UnsafeNativeMethods.OCIHandleAlloc(parenth, out hndlpp, type, 0, IntPtr.Zero); if (Bid.AdvancedOn) { Bid.Trace(" parenth=0x%-07Ix type=%3d xtramemsz=%d usrmempp=0x%-07Ix hndlpp=0x%-07Ix rc=%d\n", parenth, (int)type, 0, IntPtr.Zero, hndlpp, rc ); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OCIHandleFree ( IntPtr hndlp, OCI.HTYPE type ) { if (Bid.AdvancedOn) { Bid.Trace(" hndlp=0x%-07Ix type=%3d\n", hndlp, (int)type); } int rc = UnsafeNativeMethods.OCIHandleFree(hndlp, type); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- static internal int OCILobAppend ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix dst_locp=0x%-07Ix src_locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(dst_locp), OciHandle.HandleValueToTrace(src_locp) ); } int rc = UnsafeNativeMethods.OCILobAppend(svchp, errhp, dst_locp, src_locp); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobClose ( OciHandle svchp, OciHandle errhp, OciHandle locp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobClose(svchp, errhp, locp); if (Bid.AdvancedOn){ Bid.Trace(" %d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobCopy ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ) { if (Bid.AdvancedOn){ Bid.Trace(" " +" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" dst_locp=0x%-07Ix" +" src_locp=0x%-07Ix" +" amount=%u" +" dst_offset=%u" +" src_offset=%u\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(dst_locp), OciHandle.HandleValueToTrace(src_locp), amount, dst_offset, src_offset ); } int rc = UnsafeNativeMethods.OCILobCopy(svchp, errhp, dst_locp, src_locp, amount, dst_offset, src_offset); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobCreateTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp, [In, MarshalAs(UnmanagedType.U2)] ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm, [In, MarshalAs(UnmanagedType.U1)] OCI.LOB_TYPE lobtype, int cache, [In, MarshalAs(UnmanagedType.U2)] OCI.DURATION duration ) { if (Bid.AdvancedOn){ Bid.Trace(" " +" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" locp=0x%-07Ix" +" csid=%d" +" csfrm=%d{OCI.CHARSETFORM}" +" lobtype=%d{OCI.LOB_TYPE}" +" cache=%d" +" duration=%d{OCI.DURATION}\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), (int)csid, (int)csfrm, (int)lobtype, cache, (int)duration ); } int rc = UnsafeNativeMethods.OCILobCreateTemporary(svchp, errhp, locp, csid, csfrm, lobtype, cache, duration); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobErase ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amount, uint offset ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=0x%-07Ix amount=%d, offset=%d\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), amount, offset ); } int rc = UnsafeNativeMethods.OCILobErase(svchp, errhp, locp, ref amount, offset); if (Bid.AdvancedOn){ Bid.Trace(" amount=%u, rc=%d\n", amount, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobFileExists ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobFileExists(svchp, errhp, locp, out flag); if (Bid.AdvancedOn){ Bid.Trace(" flag=%u, rc=%d\n", flag, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobFileGetName ( OciHandle envhp, OciHandle errhp, OciHandle filep, IntPtr dir_alias, ref ushort d_length, IntPtr filename, ref ushort f_length ) { if (Bid.AdvancedOn){ Bid.Trace(" envhp=0x%-07Ix errhp=0x%-07Ix filep=%Id\n", OciHandle.HandleValueToTrace(envhp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(filep) ); } int rc = UnsafeNativeMethods.OCILobFileGetName(envhp, errhp, filep, dir_alias, ref d_length, filename, ref f_length); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d, dir_alias='%ls', d_lenght=%d, filename='%ls', f_length=%d\n", rc, envhp.PtrToString(dir_alias, d_length), (int)d_length, envhp.PtrToString(filename, f_length), (int)f_length ); } return rc; } //---------------------------------------------------------------------- static internal int OCILobFileSetName ( OciHandle envhp, OciHandle errhp, OciFileDescriptor filep, string dir_alias, string filename ) { if (Bid.AdvancedOn){ Bid.Trace(" envhp=0x%-07Ix errhp=0x%-07Ix filep=0x%-07Ix dir_alias='%ls', d_length=%d, filename='%ls', f_length=%d\n", OciHandle.HandleValueToTrace(envhp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(filep), dir_alias, dir_alias.Length, filename, filename.Length ); } byte[] dirAlias = envhp.GetBytes(dir_alias); ushort dirAliasLength = checked((ushort)dirAlias.Length); byte[] fileName = envhp.GetBytes(filename); ushort fileNameLength = checked((ushort)fileName.Length); int rc = filep.OCILobFileSetNameWrapper(envhp, errhp, dirAlias, dirAliasLength, fileName, fileNameLength); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobFreeTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobFreeTemporary(svchp, errhp, locp); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobGetChunkSize ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobGetChunkSize(svchp, errhp, locp, out lenp); if (Bid.AdvancedOn){ Bid.Trace(" len=%u, rc=%d\n", lenp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobGetLength ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobGetLength(svchp, errhp, locp, out lenp); if (Bid.AdvancedOn){ Bid.Trace(" len=%u, rc=%d\n", lenp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobIsOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ) { if (Bid.AdvancedOn) Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); int rc = UnsafeNativeMethods.OCILobIsOpen(svchp, errhp, locp, out flag); if (Bid.AdvancedOn) Bid.Trace(" flag=%d, rc=%d\n", flag, rc); return rc; } //--------------------------------------------------------------------- static internal int OCILobIsTemporary ( OciHandle envhp, OciHandle errhp, OciHandle locp, out int flag ) { if (Bid.AdvancedOn){ Bid.Trace(" envhp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(envhp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobIsTemporary(envhp, errhp, locp, out flag); if (Bid.AdvancedOn){ Bid.Trace(" flag=%d, rc=%d\n", flag, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobLoadFromFile ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix dst_locp=0x%-07Ix src_locp=0x%-07Ix amount=%u dst_offset=%u src_offset=%u\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(dst_locp), OciHandle.HandleValueToTrace(src_locp), amount, dst_offset, src_offset ); } int rc = UnsafeNativeMethods.OCILobLoadFromFile(svchp, errhp, dst_locp, src_locp, amount, dst_offset, src_offset); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, byte mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=0x%-07Ix mode=%d\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), (int)mode ); } int rc = UnsafeNativeMethods.OCILobOpen(svchp, errhp, locp, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobRead ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref int amtp, uint offset, IntPtr bufp, // using pinned memory, IntPtr is OK uint bufl, ushort csid, OCI.CHARSETFORM csfrm ) { uint uintAmtp = checked((uint)amtp); if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" locp=0x%-07Ix" +" amt=%-4d" +" offset=%-6u" +" bufp=0x%-07Ix" +" bufl=%-4d" +" ctxp=0x%-07Ix" +" cbfp=0x%-07Ix" +" csid=%-4d" +" csfrm=%d{OCI.CHARSETFORM}\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), amtp, offset, bufp, unchecked((int)bufl), IntPtr.Zero, IntPtr.Zero, (int)csid, (int)csfrm ); } int rc = UnsafeNativeMethods.OCILobRead(svchp, errhp, locp, ref uintAmtp, offset, bufp, bufl, IntPtr.Zero, IntPtr.Zero, csid, csfrm); amtp = checked((int)uintAmtp); if (Bid.AdvancedOn) Bid.Trace(" amt=%-4d rc=%d\n", amtp, rc); return rc; } //---------------------------------------------------------------------- static internal int OCILobTrim ( OciHandle svchp, OciHandle errhp, OciHandle locp, uint newlen ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=0x%-07Ix newlen=%d\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), newlen ); } int rc = UnsafeNativeMethods.OCILobTrim(svchp, errhp, locp, newlen); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobWrite ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref int amtp, uint offset, IntPtr bufp, // using pinned memory, IntPtr is OK uint buflen, byte piece, ushort csid, OCI.CHARSETFORM csfrm ) { uint uintAmtp = checked((uint)amtp); if (Bid.AdvancedOn){ Bid.Trace(" " +" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" locp=0x%-07Ix" +" amt=%d" +" offset=%u" +" bufp=0x%-07Ix" +" buflen=%d" +" piece=%d{Byte}" +" ctxp=0x%-07Ix" +" cbfp=0x%-07Ix" +" csid=%d" +" csfrm=%d{OCI.CHARSETFORM}\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), amtp, offset, bufp, unchecked((int)buflen), (int)piece, IntPtr.Zero, IntPtr.Zero, (int)csid, (int)csfrm ); } int rc = UnsafeNativeMethods.OCILobWrite(svchp, errhp, locp, ref uintAmtp, offset, bufp, buflen, piece, IntPtr.Zero, IntPtr.Zero, csid, csfrm); amtp = checked((int)uintAmtp); if (Bid.AdvancedOn){ Bid.Trace(" amt=%d, rc=%d\n", amtp, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIParamGet ( OciHandle hndlp, OCI.HTYPE hType, OciHandle errhp, out IntPtr paramdpp, // can't return a handle ref! int pos ) { int rc = UnsafeNativeMethods.OCIParamGet(hndlp, hType, errhp, out paramdpp, checked((uint)pos)); if (Bid.AdvancedOn){ Bid.Trace(" hndlp=0x%-07Ix htype=%-18ls errhp=0x%-07Ix pos=%d paramdpp=0x%-07Ix rc=%d\n", hndlp, hType, errhp, pos, paramdpp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIRowidToChar ( OciHandle rowidDesc, NativeBuffer outbfp, ref int bufferLength, OciHandle errhp ) { ushort outbflp = checked((ushort)bufferLength); if (Bid.AdvancedOn){ Bid.Trace(" rowidDesc=0x%-07Ix outbfp=0x%-07Ix outbflp=%d, errhp=0x%-07Ix\n", OciHandle.HandleValueToTrace(rowidDesc), NativeBuffer.HandleValueToTrace(outbfp), outbfp.Length, OciHandle.HandleValueToTrace(errhp) ); } int rc = UnsafeNativeMethods.OCIRowidToChar(rowidDesc, outbfp, ref outbflp, errhp); bufferLength = outbflp; if (Bid.AdvancedOn){ Bid.Trace(" outbfp='%ls' rc=%d\n", outbfp.PtrToStringAnsi(0, outbflp), rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIServerAttach ( OciHandle srvhp, OciHandle errhp, string dblink, int dblink_len, OCI.MODE mode // Must always be OCI_DEFAULT ) { if (Bid.AdvancedOn){ Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix dblink='%ls' dblink_len=%d mode=0x%x{OCI.MODE}\n", srvhp, errhp, dblink, dblink_len, (int)mode); } byte[] dblinkValue = srvhp.GetBytes(dblink); int dblinkLen = dblinkValue.Length; int rc = UnsafeNativeMethods.OCIServerAttach(srvhp, errhp, dblinkValue, dblinkLen, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OCIServerDetach ( IntPtr srvhp, IntPtr errhp, OCI.MODE mode ) { if (Bid.AdvancedOn) { Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", srvhp, errhp, (int)mode); } int rc = UnsafeNativeMethods.OCIServerDetach(srvhp, errhp, mode); if (Bid.AdvancedOn) { Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIServerVersion ( OciHandle hndlp, OciHandle errhp, NativeBuffer bufp ) { if (Bid.AdvancedOn){ Bid.Trace(" hndlp=0x%-07Ix errhp=0x%-07Ix bufp=0x%-07Ix bufsz=%d hndltype=%d{OCI.HTYPE}\n", OciHandle.HandleValueToTrace(hndlp), OciHandle.HandleValueToTrace(errhp), NativeBuffer.HandleValueToTrace(bufp), bufp.Length, (int)hndlp.HandleType ); } int rc = UnsafeNativeMethods.OCIServerVersion(hndlp, errhp, bufp, unchecked((uint)bufp.Length), (byte)hndlp.HandleType); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n%ls\n\n", rc, hndlp.PtrToString(bufp)); } return rc; } //--------------------------------------------------------------------- static internal int OCISessionBegin ( OciHandle svchp, OciHandle errhp, OciHandle usrhp, OCI.CRED credt, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix usrhp=0x%-07Ix credt=%s mode=0x%x{OCI.MODE}\n", svchp, errhp, usrhp, credt, (int)mode ); } int rc = UnsafeNativeMethods.OCISessionBegin(svchp, errhp, usrhp, credt, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCISessionEnd ( IntPtr svchp, IntPtr errhp, IntPtr usrhp, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix usrhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", svchp, errhp, usrhp, (int)mode ); } int rc = UnsafeNativeMethods.OCISessionEnd(svchp, errhp, usrhp, mode); if (Bid.AdvancedOn) Bid.Trace(" rc=%d\n", rc); return rc; } //---------------------------------------------------------------------- static internal int OCIStmtExecute ( OciHandle svchp, OciHandle stmtp, OciHandle errhp, int iters, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix stmtp=0x%-07Ix errhp=0x%-07Ix iters=%d rowoff=%d snap_in=0x%-07Ix snap_out=0x%-07Ix mode=0x%x{OCI.MODE}\n", svchp, stmtp, errhp, iters, 0, IntPtr.Zero, IntPtr.Zero, (int)mode ); } int rc = UnsafeNativeMethods.OCIStmtExecute(svchp, stmtp, errhp, checked((uint)iters), 0, IntPtr.Zero, IntPtr.Zero, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIStmtFetch ( OciHandle stmtp, OciHandle errhp, int nrows, OCI.FETCH orientation, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix nrows=%d orientation=%d{OCI.FETCH}, mode=0x%x{OCI.MODE}\n", stmtp, errhp, nrows, (int)orientation, (int)mode ); } int rc = UnsafeNativeMethods.OCIStmtFetch(stmtp, errhp, checked((uint)nrows), orientation, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIStmtPrepare ( OciHandle stmtp, OciHandle errhp, string stmt, OCI.SYNTAX language, OCI.MODE mode, OracleConnection connection ) { if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix stmt_len=%d language=%d{OCI.SYNTAX} mode=0x%x{OCI.MODE}\n\t\t%ls\n\n", stmtp, errhp, stmt.Length, (int)language, (int)mode, stmt ); } byte[] statementText = connection.GetBytes(stmt, false); uint statementTextLength = unchecked((uint)statementText.Length); int rc = UnsafeNativeMethods.OCIStmtPrepare(stmtp, errhp, statementText, statementTextLength, language, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCITransCommit ( OciHandle srvhp, OciHandle errhp, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", OciHandle.HandleValueToTrace(srvhp), OciHandle.HandleValueToTrace(errhp), (int)mode ); } int rc = UnsafeNativeMethods.OCITransCommit(srvhp, errhp, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCITransRollback ( OciHandle srvhp, OciHandle errhp, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", OciHandle.HandleValueToTrace(srvhp), OciHandle.HandleValueToTrace(errhp), (int)mode ); } int rc = UnsafeNativeMethods.OCITransRollback(srvhp, errhp, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Text; using System.Runtime.ConstrainedExecution; static internal class TracedNativeMethods { //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OraMTSEnlCtxGet ( byte[] userName, byte[] password, byte[] serverName, OciHandle pOCISvc, OciHandle pOCIErr, out IntPtr pCtxt // can't return a HandleRef ) { int rc; RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { if (Bid.AdvancedOn){ Bid.Trace("userName=..., password=..., serverName=..., pOCISvc=0x%-07Ix pOCIErr=0x%-07Ix dwFlags=0x%08X\n", OciHandle.HandleValueToTrace(pOCISvc), OciHandle.HandleValueToTrace(pOCIErr), 0 ); } rc = UnsafeNativeMethods.OraMTSEnlCtxGet(userName, password, serverName, pOCISvc, pOCIErr, 0, out pCtxt ); if (Bid.AdvancedOn){ Bid.Trace(" pCtxt=0x%-07Ix rc=%d\n", pCtxt, rc); } } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OraMTSEnlCtxRel ( IntPtr pCtxt ) { int rc; RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { if (Bid.AdvancedOn){ Bid.Trace(" pCtxt=%Id\n", pCtxt); } rc = UnsafeNativeMethods.OraMTSEnlCtxRel(pCtxt); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } } return rc; } //---------------------------------------------------------------------- static internal int OraMTSOCIErrGet ( ref int dwErr, NativeBuffer lpcEMsg, ref int lpdLen ) { if (Bid.AdvancedOn){ Bid.Trace(" dwErr=%08X, lpcEMsg=0x%-07Ix lpdLen=%d\n", dwErr, NativeBuffer.HandleValueToTrace(lpcEMsg), lpdLen ); } int rc = UnsafeNativeMethods.OraMTSOCIErrGet(ref dwErr,lpcEMsg,ref lpdLen); if (Bid.AdvancedOn){ if (0 == rc) { Bid.Trace(" rc=%d\n", rc); } else { string message = lpcEMsg.PtrToStringAnsi(0, lpdLen); Bid.Trace(" rd=%d message='%ls', lpdLen=%d\n", rc, message, lpdLen); } } return rc; } //--------------------------------------------------------------------- static internal int OraMTSJoinTxn ( OciEnlistContext pCtxt, System.Transactions.IDtcTransaction pTrans ) { if (Bid.AdvancedOn){ Bid.Trace(" pCtxt=0x%-07Ix pTrans=...\n", OciEnlistContext.HandleValueToTrace(pCtxt)); } int rc = UnsafeNativeMethods.OraMTSJoinTxn(pCtxt, pTrans); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int oermsg ( short rcode, NativeBuffer buf ) { if (Bid.AdvancedOn){ Bid.Trace(" rcode=%d\n", (int)rcode); } int rc = UnsafeNativeMethods.oermsg(rcode, buf); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } #if ADVANCEDTRACING //---------------------------------------------------------------------- static internal string GetAttributeName ( OciHandle handle, OCI.ATTR atype ) { if (OCI.HTYPE.OCI_DTYPE_PARAM == handle.HandleType) return ((OCI.PATTR)atype).ToString(); return atype.ToString(); } #endif //ADVANCEDTRACING //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, ref IntPtr attributep, // Used to get strings; can't return a handle ref! ref uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, ref attributep, ref sizep, attrtype, errhp); if (Bid.AdvancedOn) { if (OCI.ATTR.OCI_ATTR_NAME == attrtype) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20ls sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, trgthndlp.PtrToString(attributep, checked((int)sizep)), sizep, rc ); } else { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=0x%-18Ix sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, attributep, sizep, rc ); } } return rc; } //---------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, out byte attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int attribute = 0; int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, out attribute, out sizep, attrtype, errhp); attributep = unchecked((byte)attribute); if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20d sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, (int)attributep, sizep, rc ); } return rc; } //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, out short attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int attribute = 0; int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, out attribute, out sizep, attrtype, errhp); attributep = unchecked((short)attribute); if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20d sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, (int)attributep, sizep, rc ); } return rc; } //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, out int attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, out attributep, out sizep, attrtype, errhp); if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=%-20d sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, attributep, sizep, rc ); } return rc; } //--------------------------------------------------------------------- static internal int OCIAttrGet ( OciHandle trgthndlp, OciHandle attributep, out uint sizep, OCI.ATTR attrtype, OciHandle errhp ) { // NOTE: we're updating the data that the handle points to, not the // handle value itself. int rc = UnsafeNativeMethods.OCIAttrGet(trgthndlp, trgthndlp.HandleType, attributep, out sizep, attrtype, errhp); if (Bid.AdvancedOn) { // Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attrtype=%-22ls errhp=0x%-07Ix attributep=0x%-18Ix sizep=%2d rc=%d\n", trgthndlp, trgthndlp.HandleType, attrtype, errhp, OciHandle.HandleValueToTrace(attributep), sizep, rc ); } return rc; } //---------------------------------------------------------------------- static internal int OCIAttrSet ( OciHandle trgthndlp, ref int attributep, uint size, OCI.ATTR attrtype, OciHandle errhp ) { if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attributep=%-9d size=%-2d attrtype=%-22ls errhp=0x%-07Ix\n", trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); } int rc = UnsafeNativeMethods.OCIAttrSet(trgthndlp, trgthndlp.HandleType, ref attributep, size, attrtype, errhp); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //--------------------------------------------------------------------- static internal int OCIAttrSet ( OciHandle trgthndlp, OciHandle attributep, uint size, OCI.ATTR attrtype, OciHandle errhp ) { if (Bid.AdvancedOn) { Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attributep=0x%-07Ix size=%d attrtype=%-22ls errhp=0x%-07Ix\n", trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); } int rc = UnsafeNativeMethods.OCIAttrSet(trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- static internal int OCIAttrSet ( OciHandle trgthndlp, byte[] attributep, uint size, OCI.ATTR attrtype, OciHandle errhp ) { if (Bid.AdvancedOn) { string attributes; if (OCI.ATTR.OCI_ATTR_EXTERNAL_NAME == attrtype || OCI.ATTR.OCI_ATTR_INTERNAL_NAME == attrtype) { char[] temp = System.Text.Encoding.UTF8.GetChars(attributep, 0, checked((int)size)); attributes = new string(temp); } else { attributes = attributep.ToString(); } Bid.Trace(" trgthndlp=0x%-07Ix trghndltyp=%-18ls attributep='%ls' size=%d attrtype=%-22ls errhp=0x%-07Ix\n", trgthndlp, trgthndlp.HandleType, attributes, size, attrtype, errhp); } int rc = UnsafeNativeMethods.OCIAttrSet(trgthndlp, trgthndlp.HandleType, attributep, size, attrtype, errhp); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- static internal int OCIBindByName ( OciHandle stmtp, out IntPtr bindpp, // can't return a handle ref! OciHandle errhp, string placeholder, int placeh_len, IntPtr valuep, int value_sz, OCI.DATATYPE dty, IntPtr indp, IntPtr alenp, //ub2* OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix placeholder=%-20ls placeh_len=%-2d valuep=0x%-07Ix value_sz=%-4d dty=%d{OCI.DATATYPE} indp=0x%-07Ix *indp=%-3d alenp=0x%-07Ix *alenp=%-4d rcodep=0x%-07Ix maxarr_len=%-4d curelap=0x%-07Ix mode=0x%x{OCI.MODE}\n", OciHandle.HandleValueToTrace(stmtp), OciHandle.HandleValueToTrace(errhp), placeholder, placeh_len, valuep, value_sz, (int)dty, indp, (int)(IntPtr.Zero == (IntPtr)indp ? (short)0 : Marshal.ReadInt16((IntPtr)indp)), alenp, (int)(IntPtr.Zero == (IntPtr)alenp? (short)0 : Marshal.ReadInt16((IntPtr)alenp)), IntPtr.Zero, 0, IntPtr.Zero, (int)mode ); } byte[] placeholderName = stmtp.GetBytes(placeholder); int placeholderNameLength = placeholderName.Length; int rc = UnsafeNativeMethods.OCIBindByName(stmtp, out bindpp, errhp, placeholderName, placeholderNameLength, valuep, value_sz, dty, indp, alenp, IntPtr.Zero, 0, IntPtr.Zero, mode); if (Bid.AdvancedOn){ Bid.Trace(" bindpp=0x%-07Ix rc=%d\n", bindpp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIDefineByPos ( OciHandle stmtp, out IntPtr hndlpp, // can't return a handle ref! OciHandle errhp, uint position, IntPtr valuep, int value_sz, OCI.DATATYPE dty, IntPtr indp, IntPtr rlenp, //ub2* IntPtr rcodep, //ub2* OCI.MODE mode ) { int rc = UnsafeNativeMethods.OCIDefineByPos(stmtp, out hndlpp, errhp, position, valuep, value_sz, dty, indp, rlenp, rcodep, mode); if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix position=%-2d valuep=0x%-07Ix value_sz=%-4d dty=%-3d %-14s indp=0x%-07Ix rlenp=0x%-07Ix rcodep=0x%-07Ix mode=0x%x{OCI.MODE} hndlpp=0x%-07Ix rc=%d\n", stmtp, errhp, position, valuep, value_sz, (int)dty, dty, indp, rlenp, rcodep, (int)mode, hndlpp, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIDefineArrayOfStruct ( OciHandle defnp, OciHandle errhp, uint pvskip, uint indskip, uint rlskip, uint rcskip ) { if (Bid.AdvancedOn){ Bid.Trace(" defnp=0x%-07Ix errhp=0x%-07Ix pvskip=%-4d indskip=%-4d rlskip=%-4d rcskip=%-4d\n", defnp, errhp, pvskip, indskip, rlskip, rcskip ); } int rc = UnsafeNativeMethods.OCIDefineArrayOfStruct(defnp, errhp, pvskip, indskip, rlskip, rcskip); if (0 != rc && Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIDefineDynamic ( OciHandle defnp, OciHandle errhp, IntPtr octxp, OCI.Callback.OCICallbackDefine ocbfp ) { if (Bid.AdvancedOn){ Bid.Trace(" defnp=0x%-07Ix errhp=0x%-07Ix octxp=0x%-07Ix ocbfp=...\n", OciHandle.HandleValueToTrace(defnp), OciHandle.HandleValueToTrace(errhp), octxp); // note: can't trace OCI.Callback.OCICallbackDefine } int rc = UnsafeNativeMethods.OCIDefineDynamic(defnp, errhp, octxp, ocbfp); if (0 != rc && Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIDescriptorAlloc ( OciHandle parenth, out IntPtr hndlpp, // can't return a handle ref! OCI.HTYPE type ) { int rc = UnsafeNativeMethods.OCIDescriptorAlloc(parenth, out hndlpp, type, 0, IntPtr.Zero); if (Bid.AdvancedOn) { Bid.Trace(" parenth=0x%-07Ix type=%3d xtramemsz=%d usrmempp=0x%-07Ix hndlpp=0x%-07Ix rc=%d\n", parenth, (int)type, 0, IntPtr.Zero, hndlpp, rc ); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OCIDescriptorFree ( IntPtr hndlp, OCI.HTYPE type ) { if (Bid.AdvancedOn) { Bid.Trace(" hndlp=0x%Id type=%3d\n", hndlp, (int)type ); } int rc = UnsafeNativeMethods.OCIDescriptorFree(hndlp, type); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIEnvCreate ( out IntPtr envhpp, // can't return a handle ref! OCI.MODE mode ) { if (Bid.AdvancedOn) { Bid.Trace(" mode=0x%x{OCI.MODE} ctxp=0x%-07Ix malocfp=0x%-07Ix ralocfp=0x%-07Ix mfreefp=0x%-07Ix xtramemsz=%d usrmempp=0x%-07Ix", (int)mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero ); } int rc = UnsafeNativeMethods.OCIEnvCreate(out envhpp, mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero); if (Bid.AdvancedOn) { Bid.Trace(" envhpp=0x%-07Ix, rc=%d\n", envhpp, rc ); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIEnvNlsCreate ( out IntPtr envhpp, // can't return a handle ref! OCI.MODE mode, ushort charset, ushort ncharset ) { if (Bid.AdvancedOn) { Bid.Trace(" mode=0x%x{OCI.MODE} ctxp=0x%-07Ix malocfp=0x%-07Ix ralocfp=0x%-07Ix mfreefp=0x%-07Ix xtramemsz=%d usrmempp=0x%-07Ix charset=%d ncharset=%d", (int)mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero, (int)charset, (int)ncharset ); } int rc = UnsafeNativeMethods.OCIEnvNlsCreate(out envhpp, mode, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, IntPtr.Zero, 0, IntPtr.Zero, charset, ncharset); if (Bid.AdvancedOn) { Bid.Trace(" envhpp=0x%-07Ix rc=%d\n", envhpp, rc ); } return rc; } //---------------------------------------------------------------------- static internal int OCIErrorGet ( OciHandle hndlp, int recordno, out int errcodep, NativeBuffer bufp ) { if (Bid.AdvancedOn){ Bid.Trace(" hndlp=0x%-07Ix recordno=%d sqlstate=0x%-07Ix bufp=0x%-07Ix bufsiz=%d type=%d{OCI.HTYPE}\n", OciHandle.HandleValueToTrace(hndlp), recordno, IntPtr.Zero, NativeBuffer.HandleValueToTrace(bufp), bufp.Length, (int)hndlp.HandleType ); } int rc = UnsafeNativeMethods.OCIErrorGet(hndlp, checked((uint)recordno), IntPtr.Zero, out errcodep, bufp, unchecked((uint)bufp.Length), hndlp.HandleType); if (Bid.AdvancedOn){ Bid.Trace(" errcodep=%d rc=%d\n\t%ls\n\n", errcodep, rc, hndlp.PtrToString(bufp)); } return rc; } //---------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] static internal int OCIHandleAlloc ( OciHandle parenth, out IntPtr hndlpp, // can't return a handle ref! OCI.HTYPE type ) { int rc = UnsafeNativeMethods.OCIHandleAlloc(parenth, out hndlpp, type, 0, IntPtr.Zero); if (Bid.AdvancedOn) { Bid.Trace(" parenth=0x%-07Ix type=%3d xtramemsz=%d usrmempp=0x%-07Ix hndlpp=0x%-07Ix rc=%d\n", parenth, (int)type, 0, IntPtr.Zero, hndlpp, rc ); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OCIHandleFree ( IntPtr hndlp, OCI.HTYPE type ) { if (Bid.AdvancedOn) { Bid.Trace(" hndlp=0x%-07Ix type=%3d\n", hndlp, (int)type); } int rc = UnsafeNativeMethods.OCIHandleFree(hndlp, type); //if (Bid.AdvancedOn) { // Bid.Trace(" rc=%d\n", rc); //} return rc; } //---------------------------------------------------------------------- static internal int OCILobAppend ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix dst_locp=0x%-07Ix src_locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(dst_locp), OciHandle.HandleValueToTrace(src_locp) ); } int rc = UnsafeNativeMethods.OCILobAppend(svchp, errhp, dst_locp, src_locp); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobClose ( OciHandle svchp, OciHandle errhp, OciHandle locp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobClose(svchp, errhp, locp); if (Bid.AdvancedOn){ Bid.Trace(" %d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobCopy ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ) { if (Bid.AdvancedOn){ Bid.Trace(" " +" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" dst_locp=0x%-07Ix" +" src_locp=0x%-07Ix" +" amount=%u" +" dst_offset=%u" +" src_offset=%u\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(dst_locp), OciHandle.HandleValueToTrace(src_locp), amount, dst_offset, src_offset ); } int rc = UnsafeNativeMethods.OCILobCopy(svchp, errhp, dst_locp, src_locp, amount, dst_offset, src_offset); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobCreateTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp, [In, MarshalAs(UnmanagedType.U2)] ushort csid, [In, MarshalAs(UnmanagedType.U1)] OCI.CHARSETFORM csfrm, [In, MarshalAs(UnmanagedType.U1)] OCI.LOB_TYPE lobtype, int cache, [In, MarshalAs(UnmanagedType.U2)] OCI.DURATION duration ) { if (Bid.AdvancedOn){ Bid.Trace(" " +" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" locp=0x%-07Ix" +" csid=%d" +" csfrm=%d{OCI.CHARSETFORM}" +" lobtype=%d{OCI.LOB_TYPE}" +" cache=%d" +" duration=%d{OCI.DURATION}\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), (int)csid, (int)csfrm, (int)lobtype, cache, (int)duration ); } int rc = UnsafeNativeMethods.OCILobCreateTemporary(svchp, errhp, locp, csid, csfrm, lobtype, cache, duration); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobErase ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref uint amount, uint offset ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=0x%-07Ix amount=%d, offset=%d\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), amount, offset ); } int rc = UnsafeNativeMethods.OCILobErase(svchp, errhp, locp, ref amount, offset); if (Bid.AdvancedOn){ Bid.Trace(" amount=%u, rc=%d\n", amount, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobFileExists ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobFileExists(svchp, errhp, locp, out flag); if (Bid.AdvancedOn){ Bid.Trace(" flag=%u, rc=%d\n", flag, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobFileGetName ( OciHandle envhp, OciHandle errhp, OciHandle filep, IntPtr dir_alias, ref ushort d_length, IntPtr filename, ref ushort f_length ) { if (Bid.AdvancedOn){ Bid.Trace(" envhp=0x%-07Ix errhp=0x%-07Ix filep=%Id\n", OciHandle.HandleValueToTrace(envhp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(filep) ); } int rc = UnsafeNativeMethods.OCILobFileGetName(envhp, errhp, filep, dir_alias, ref d_length, filename, ref f_length); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d, dir_alias='%ls', d_lenght=%d, filename='%ls', f_length=%d\n", rc, envhp.PtrToString(dir_alias, d_length), (int)d_length, envhp.PtrToString(filename, f_length), (int)f_length ); } return rc; } //---------------------------------------------------------------------- static internal int OCILobFileSetName ( OciHandle envhp, OciHandle errhp, OciFileDescriptor filep, string dir_alias, string filename ) { if (Bid.AdvancedOn){ Bid.Trace(" envhp=0x%-07Ix errhp=0x%-07Ix filep=0x%-07Ix dir_alias='%ls', d_length=%d, filename='%ls', f_length=%d\n", OciHandle.HandleValueToTrace(envhp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(filep), dir_alias, dir_alias.Length, filename, filename.Length ); } byte[] dirAlias = envhp.GetBytes(dir_alias); ushort dirAliasLength = checked((ushort)dirAlias.Length); byte[] fileName = envhp.GetBytes(filename); ushort fileNameLength = checked((ushort)fileName.Length); int rc = filep.OCILobFileSetNameWrapper(envhp, errhp, dirAlias, dirAliasLength, fileName, fileNameLength); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobFreeTemporary ( OciHandle svchp, OciHandle errhp, OciHandle locp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobFreeTemporary(svchp, errhp, locp); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobGetChunkSize ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobGetChunkSize(svchp, errhp, locp, out lenp); if (Bid.AdvancedOn){ Bid.Trace(" len=%u, rc=%d\n", lenp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobGetLength ( OciHandle svchp, OciHandle errhp, OciHandle locp, out uint lenp ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobGetLength(svchp, errhp, locp, out lenp); if (Bid.AdvancedOn){ Bid.Trace(" len=%u, rc=%d\n", lenp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobIsOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, out int flag ) { if (Bid.AdvancedOn) Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); int rc = UnsafeNativeMethods.OCILobIsOpen(svchp, errhp, locp, out flag); if (Bid.AdvancedOn) Bid.Trace(" flag=%d, rc=%d\n", flag, rc); return rc; } //--------------------------------------------------------------------- static internal int OCILobIsTemporary ( OciHandle envhp, OciHandle errhp, OciHandle locp, out int flag ) { if (Bid.AdvancedOn){ Bid.Trace(" envhp=0x%-07Ix errhp=0x%-07Ix locp=%Id\n", OciHandle.HandleValueToTrace(envhp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp) ); } int rc = UnsafeNativeMethods.OCILobIsTemporary(envhp, errhp, locp, out flag); if (Bid.AdvancedOn){ Bid.Trace(" flag=%d, rc=%d\n", flag, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobLoadFromFile ( OciHandle svchp, OciHandle errhp, OciHandle dst_locp, OciHandle src_locp, uint amount, uint dst_offset, uint src_offset ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix dst_locp=0x%-07Ix src_locp=0x%-07Ix amount=%u dst_offset=%u src_offset=%u\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(dst_locp), OciHandle.HandleValueToTrace(src_locp), amount, dst_offset, src_offset ); } int rc = UnsafeNativeMethods.OCILobLoadFromFile(svchp, errhp, dst_locp, src_locp, amount, dst_offset, src_offset); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobOpen ( OciHandle svchp, OciHandle errhp, OciHandle locp, byte mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=0x%-07Ix mode=%d\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), (int)mode ); } int rc = UnsafeNativeMethods.OCILobOpen(svchp, errhp, locp, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCILobRead ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref int amtp, uint offset, IntPtr bufp, // using pinned memory, IntPtr is OK uint bufl, ushort csid, OCI.CHARSETFORM csfrm ) { uint uintAmtp = checked((uint)amtp); if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" locp=0x%-07Ix" +" amt=%-4d" +" offset=%-6u" +" bufp=0x%-07Ix" +" bufl=%-4d" +" ctxp=0x%-07Ix" +" cbfp=0x%-07Ix" +" csid=%-4d" +" csfrm=%d{OCI.CHARSETFORM}\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), amtp, offset, bufp, unchecked((int)bufl), IntPtr.Zero, IntPtr.Zero, (int)csid, (int)csfrm ); } int rc = UnsafeNativeMethods.OCILobRead(svchp, errhp, locp, ref uintAmtp, offset, bufp, bufl, IntPtr.Zero, IntPtr.Zero, csid, csfrm); amtp = checked((int)uintAmtp); if (Bid.AdvancedOn) Bid.Trace(" amt=%-4d rc=%d\n", amtp, rc); return rc; } //---------------------------------------------------------------------- static internal int OCILobTrim ( OciHandle svchp, OciHandle errhp, OciHandle locp, uint newlen ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix locp=0x%-07Ix newlen=%d\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), newlen ); } int rc = UnsafeNativeMethods.OCILobTrim(svchp, errhp, locp, newlen); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCILobWrite ( OciHandle svchp, OciHandle errhp, OciHandle locp, ref int amtp, uint offset, IntPtr bufp, // using pinned memory, IntPtr is OK uint buflen, byte piece, ushort csid, OCI.CHARSETFORM csfrm ) { uint uintAmtp = checked((uint)amtp); if (Bid.AdvancedOn){ Bid.Trace(" " +" svchp=0x%-07Ix" +" errhp=0x%-07Ix" +" locp=0x%-07Ix" +" amt=%d" +" offset=%u" +" bufp=0x%-07Ix" +" buflen=%d" +" piece=%d{Byte}" +" ctxp=0x%-07Ix" +" cbfp=0x%-07Ix" +" csid=%d" +" csfrm=%d{OCI.CHARSETFORM}\n", OciHandle.HandleValueToTrace(svchp), OciHandle.HandleValueToTrace(errhp), OciHandle.HandleValueToTrace(locp), amtp, offset, bufp, unchecked((int)buflen), (int)piece, IntPtr.Zero, IntPtr.Zero, (int)csid, (int)csfrm ); } int rc = UnsafeNativeMethods.OCILobWrite(svchp, errhp, locp, ref uintAmtp, offset, bufp, buflen, piece, IntPtr.Zero, IntPtr.Zero, csid, csfrm); amtp = checked((int)uintAmtp); if (Bid.AdvancedOn){ Bid.Trace(" amt=%d, rc=%d\n", amtp, rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIParamGet ( OciHandle hndlp, OCI.HTYPE hType, OciHandle errhp, out IntPtr paramdpp, // can't return a handle ref! int pos ) { int rc = UnsafeNativeMethods.OCIParamGet(hndlp, hType, errhp, out paramdpp, checked((uint)pos)); if (Bid.AdvancedOn){ Bid.Trace(" hndlp=0x%-07Ix htype=%-18ls errhp=0x%-07Ix pos=%d paramdpp=0x%-07Ix rc=%d\n", hndlp, hType, errhp, pos, paramdpp, rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIRowidToChar ( OciHandle rowidDesc, NativeBuffer outbfp, ref int bufferLength, OciHandle errhp ) { ushort outbflp = checked((ushort)bufferLength); if (Bid.AdvancedOn){ Bid.Trace(" rowidDesc=0x%-07Ix outbfp=0x%-07Ix outbflp=%d, errhp=0x%-07Ix\n", OciHandle.HandleValueToTrace(rowidDesc), NativeBuffer.HandleValueToTrace(outbfp), outbfp.Length, OciHandle.HandleValueToTrace(errhp) ); } int rc = UnsafeNativeMethods.OCIRowidToChar(rowidDesc, outbfp, ref outbflp, errhp); bufferLength = outbflp; if (Bid.AdvancedOn){ Bid.Trace(" outbfp='%ls' rc=%d\n", outbfp.PtrToStringAnsi(0, outbflp), rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIServerAttach ( OciHandle srvhp, OciHandle errhp, string dblink, int dblink_len, OCI.MODE mode // Must always be OCI_DEFAULT ) { if (Bid.AdvancedOn){ Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix dblink='%ls' dblink_len=%d mode=0x%x{OCI.MODE}\n", srvhp, errhp, dblink, dblink_len, (int)mode); } byte[] dblinkValue = srvhp.GetBytes(dblink); int dblinkLen = dblinkValue.Length; int rc = UnsafeNativeMethods.OCIServerAttach(srvhp, errhp, dblinkValue, dblinkLen, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] static internal int OCIServerDetach ( IntPtr srvhp, IntPtr errhp, OCI.MODE mode ) { if (Bid.AdvancedOn) { Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", srvhp, errhp, (int)mode); } int rc = UnsafeNativeMethods.OCIServerDetach(srvhp, errhp, mode); if (Bid.AdvancedOn) { Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIServerVersion ( OciHandle hndlp, OciHandle errhp, NativeBuffer bufp ) { if (Bid.AdvancedOn){ Bid.Trace(" hndlp=0x%-07Ix errhp=0x%-07Ix bufp=0x%-07Ix bufsz=%d hndltype=%d{OCI.HTYPE}\n", OciHandle.HandleValueToTrace(hndlp), OciHandle.HandleValueToTrace(errhp), NativeBuffer.HandleValueToTrace(bufp), bufp.Length, (int)hndlp.HandleType ); } int rc = UnsafeNativeMethods.OCIServerVersion(hndlp, errhp, bufp, unchecked((uint)bufp.Length), (byte)hndlp.HandleType); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n%ls\n\n", rc, hndlp.PtrToString(bufp)); } return rc; } //--------------------------------------------------------------------- static internal int OCISessionBegin ( OciHandle svchp, OciHandle errhp, OciHandle usrhp, OCI.CRED credt, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix usrhp=0x%-07Ix credt=%s mode=0x%x{OCI.MODE}\n", svchp, errhp, usrhp, credt, (int)mode ); } int rc = UnsafeNativeMethods.OCISessionBegin(svchp, errhp, usrhp, credt, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCISessionEnd ( IntPtr svchp, IntPtr errhp, IntPtr usrhp, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix errhp=0x%-07Ix usrhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", svchp, errhp, usrhp, (int)mode ); } int rc = UnsafeNativeMethods.OCISessionEnd(svchp, errhp, usrhp, mode); if (Bid.AdvancedOn) Bid.Trace(" rc=%d\n", rc); return rc; } //---------------------------------------------------------------------- static internal int OCIStmtExecute ( OciHandle svchp, OciHandle stmtp, OciHandle errhp, int iters, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" svchp=0x%-07Ix stmtp=0x%-07Ix errhp=0x%-07Ix iters=%d rowoff=%d snap_in=0x%-07Ix snap_out=0x%-07Ix mode=0x%x{OCI.MODE}\n", svchp, stmtp, errhp, iters, 0, IntPtr.Zero, IntPtr.Zero, (int)mode ); } int rc = UnsafeNativeMethods.OCIStmtExecute(svchp, stmtp, errhp, checked((uint)iters), 0, IntPtr.Zero, IntPtr.Zero, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCIStmtFetch ( OciHandle stmtp, OciHandle errhp, int nrows, OCI.FETCH orientation, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix nrows=%d orientation=%d{OCI.FETCH}, mode=0x%x{OCI.MODE}\n", stmtp, errhp, nrows, (int)orientation, (int)mode ); } int rc = UnsafeNativeMethods.OCIStmtFetch(stmtp, errhp, checked((uint)nrows), orientation, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //---------------------------------------------------------------------- static internal int OCIStmtPrepare ( OciHandle stmtp, OciHandle errhp, string stmt, OCI.SYNTAX language, OCI.MODE mode, OracleConnection connection ) { if (Bid.AdvancedOn){ Bid.Trace(" stmtp=0x%-07Ix errhp=0x%-07Ix stmt_len=%d language=%d{OCI.SYNTAX} mode=0x%x{OCI.MODE}\n\t\t%ls\n\n", stmtp, errhp, stmt.Length, (int)language, (int)mode, stmt ); } byte[] statementText = connection.GetBytes(stmt, false); uint statementTextLength = unchecked((uint)statementText.Length); int rc = UnsafeNativeMethods.OCIStmtPrepare(stmtp, errhp, statementText, statementTextLength, language, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCITransCommit ( OciHandle srvhp, OciHandle errhp, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", OciHandle.HandleValueToTrace(srvhp), OciHandle.HandleValueToTrace(errhp), (int)mode ); } int rc = UnsafeNativeMethods.OCITransCommit(srvhp, errhp, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } //--------------------------------------------------------------------- static internal int OCITransRollback ( OciHandle srvhp, OciHandle errhp, OCI.MODE mode ) { if (Bid.AdvancedOn){ Bid.Trace(" srvhp=0x%-07Ix errhp=0x%-07Ix mode=0x%x{OCI.MODE}\n", OciHandle.HandleValueToTrace(srvhp), OciHandle.HandleValueToTrace(errhp), (int)mode ); } int rc = UnsafeNativeMethods.OCITransRollback(srvhp, errhp, mode); if (Bid.AdvancedOn){ Bid.Trace(" rc=%d\n", rc); } return rc; } }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
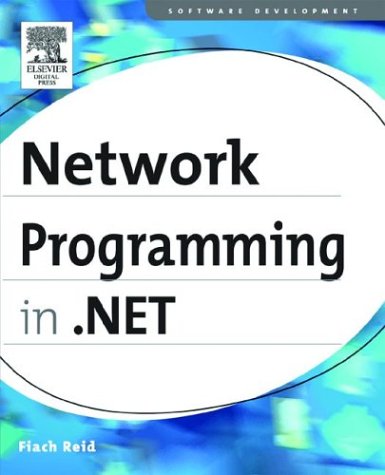
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeValidationEventArgs.cs
- CodeNamespaceImport.cs
- Zone.cs
- WmpBitmapEncoder.cs
- JsonQNameDataContract.cs
- _NegotiateClient.cs
- StylusPointPropertyId.cs
- ToolStripMenuItem.cs
- SuppressIldasmAttribute.cs
- Stacktrace.cs
- StringDictionaryCodeDomSerializer.cs
- RC2CryptoServiceProvider.cs
- BaseTemplateBuildProvider.cs
- Oci.cs
- TwoPhaseCommit.cs
- CommandConverter.cs
- WmfPlaceableFileHeader.cs
- FormsAuthenticationUserCollection.cs
- BaseAppDomainProtocolHandler.cs
- NewArray.cs
- OutOfProcStateClientManager.cs
- NativeMethods.cs
- contentDescriptor.cs
- mansign.cs
- UniqueIdentifierService.cs
- AdjustableArrowCap.cs
- OraclePermission.cs
- TrustManager.cs
- SQLMembershipProvider.cs
- BevelBitmapEffect.cs
- Collection.cs
- ImageCodecInfo.cs
- Speller.cs
- SqlUserDefinedTypeAttribute.cs
- SqlProvider.cs
- UserValidatedEventArgs.cs
- FilterException.cs
- PEFileReader.cs
- WebPartUserCapability.cs
- DispatcherExceptionFilterEventArgs.cs
- XmlChildNodes.cs
- Types.cs
- HtmlWindow.cs
- DataControlFieldHeaderCell.cs
- ImageDrawing.cs
- SqlDataSourceSelectingEventArgs.cs
- FilterQueryOptionExpression.cs
- PngBitmapDecoder.cs
- TabControlEvent.cs
- SqlDataAdapter.cs
- ProfileSettingsCollection.cs
- XmlDataSourceView.cs
- ToolStripOverflowButton.cs
- FlowNode.cs
- AttributeCollection.cs
- UnsafeNativeMethods.cs
- HtmlUtf8RawTextWriter.cs
- MsmqBindingBase.cs
- HttpServerVarsCollection.cs
- XmlSubtreeReader.cs
- ManagementScope.cs
- SimpleHandlerBuildProvider.cs
- MaterializeFromAtom.cs
- IpcChannel.cs
- QilPatternFactory.cs
- MLangCodePageEncoding.cs
- WindowsStatusBar.cs
- OutputCacheProfileCollection.cs
- CodeEntryPointMethod.cs
- CacheDependency.cs
- OdbcParameter.cs
- RestClientProxyHandler.cs
- AsyncCompletedEventArgs.cs
- StopRoutingHandler.cs
- PackageProperties.cs
- XmlUnspecifiedAttribute.cs
- GraphicsPath.cs
- ProviderSettings.cs
- NativeRecognizer.cs
- BitmapCacheBrush.cs
- TypeForwardedToAttribute.cs
- TdsValueSetter.cs
- OleDbPermission.cs
- ArgumentValue.cs
- RootBrowserWindowAutomationPeer.cs
- InkPresenterAutomationPeer.cs
- MailAddress.cs
- DiscoveryClientReferences.cs
- ExpandableObjectConverter.cs
- JapaneseCalendar.cs
- ModelPropertyImpl.cs
- RealProxy.cs
- BindingUtils.cs
- FocusTracker.cs
- TypeUnloadedException.cs
- HyperLink.cs
- TreeNodeCollection.cs
- TimeIntervalCollection.cs
- ToolTip.cs
- SQLBoolean.cs