Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / Command.cs / 1 / Command.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; ////// internal class Command : WeakReference { private static Command[] cmds; private static int icmdTry; private static object internalSyncObject = new object(); private const int idMin = 0x00100; private const int idLim = 0x10000; internal int id; public Command(ICommandExecutor target) : base(target, false) { AssignID(this); } public virtual int ID { get { return id; } } [SuppressMessage("Microsoft.Reliability", "CA2001:AvoidCallingProblematicMethods")] protected static void AssignID(Command cmd) { lock(internalSyncObject) { int icmd; if (null == cmds) { cmds = new Command[20]; icmd = 0; } else { Debug.Assert(cmds.Length > 0, "why is cmds.Length zero?"); Debug.Assert(icmdTry >= 0, "why is icmdTry negative?"); int icmdLim = cmds.Length; if (icmdTry >= icmdLim) icmdTry = 0; // First look for an empty slot (starting at icmdTry). for (icmd = icmdTry; icmd < icmdLim; icmd++) if (null == cmds[icmd]) goto FindSlotComplete; for (icmd = 0; icmd < icmdTry; icmd++) if (null == cmds[icmd]) goto FindSlotComplete; // All slots have Command objects in them. Look for a command // with a null referent. for (icmd = 0; icmd < icmdLim; icmd++) if (null == cmds[icmd].Target) goto FindSlotComplete; // Grow the array. icmd = cmds.Length; icmdLim = Math.Min(idLim - idMin, 2 * icmd); if (icmdLim <= icmd) { // Already at maximal size. Do a garbage collect and look again. GC.Collect(); for (icmd = 0; icmd < icmdLim; icmd++) { if (null == cmds[icmd] || null == cmds[icmd].Target) goto FindSlotComplete; } throw new ArgumentException(SR.GetString(SR.CommandIdNotAllocated)); } else { Command[] newCmds = new Command[icmdLim]; Array.Copy(cmds, 0, newCmds, 0, icmd); cmds = newCmds; } } FindSlotComplete: cmd.id = icmd + idMin; Debug.Assert(cmd.id >= idMin && cmd.id < idLim, "generated command id out of range"); cmds[icmd] = cmd; icmdTry = icmd + 1; } } public static bool DispatchID(int id) { Command cmd = GetCommandFromID(id); if (null == cmd) return false; return cmd.Invoke(); } protected static void Dispose(Command cmd) { lock (internalSyncObject) { if (cmd.id >= idMin) { cmd.Target = null; if (cmds[cmd.id - idMin] == cmd) cmds[cmd.id - idMin] = null; cmd.id = 0; } } } public virtual void Dispose() { if (id >= idMin) Dispose(this); } public static Command GetCommandFromID(int id) { lock (internalSyncObject) { if (null == cmds) return null; int i = id - idMin; if (i < 0 || i >= cmds.Length) return null; return cmds[i]; } } public virtual bool Invoke() { object target = Target; if (!(target is ICommandExecutor)) return false; ((ICommandExecutor)target).Execute(); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; ////// internal class Command : WeakReference { private static Command[] cmds; private static int icmdTry; private static object internalSyncObject = new object(); private const int idMin = 0x00100; private const int idLim = 0x10000; internal int id; public Command(ICommandExecutor target) : base(target, false) { AssignID(this); } public virtual int ID { get { return id; } } [SuppressMessage("Microsoft.Reliability", "CA2001:AvoidCallingProblematicMethods")] protected static void AssignID(Command cmd) { lock(internalSyncObject) { int icmd; if (null == cmds) { cmds = new Command[20]; icmd = 0; } else { Debug.Assert(cmds.Length > 0, "why is cmds.Length zero?"); Debug.Assert(icmdTry >= 0, "why is icmdTry negative?"); int icmdLim = cmds.Length; if (icmdTry >= icmdLim) icmdTry = 0; // First look for an empty slot (starting at icmdTry). for (icmd = icmdTry; icmd < icmdLim; icmd++) if (null == cmds[icmd]) goto FindSlotComplete; for (icmd = 0; icmd < icmdTry; icmd++) if (null == cmds[icmd]) goto FindSlotComplete; // All slots have Command objects in them. Look for a command // with a null referent. for (icmd = 0; icmd < icmdLim; icmd++) if (null == cmds[icmd].Target) goto FindSlotComplete; // Grow the array. icmd = cmds.Length; icmdLim = Math.Min(idLim - idMin, 2 * icmd); if (icmdLim <= icmd) { // Already at maximal size. Do a garbage collect and look again. GC.Collect(); for (icmd = 0; icmd < icmdLim; icmd++) { if (null == cmds[icmd] || null == cmds[icmd].Target) goto FindSlotComplete; } throw new ArgumentException(SR.GetString(SR.CommandIdNotAllocated)); } else { Command[] newCmds = new Command[icmdLim]; Array.Copy(cmds, 0, newCmds, 0, icmd); cmds = newCmds; } } FindSlotComplete: cmd.id = icmd + idMin; Debug.Assert(cmd.id >= idMin && cmd.id < idLim, "generated command id out of range"); cmds[icmd] = cmd; icmdTry = icmd + 1; } } public static bool DispatchID(int id) { Command cmd = GetCommandFromID(id); if (null == cmd) return false; return cmd.Invoke(); } protected static void Dispose(Command cmd) { lock (internalSyncObject) { if (cmd.id >= idMin) { cmd.Target = null; if (cmds[cmd.id - idMin] == cmd) cmds[cmd.id - idMin] = null; cmd.id = 0; } } } public virtual void Dispose() { if (id >= idMin) Dispose(this); } public static Command GetCommandFromID(int id) { lock (internalSyncObject) { if (null == cmds) return null; int i = id - idMin; if (i < 0 || i >= cmds.Length) return null; return cmds[i]; } } public virtual bool Invoke() { object target = Target; if (!(target is ICommandExecutor)) return false; ((ICommandExecutor)target).Execute(); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
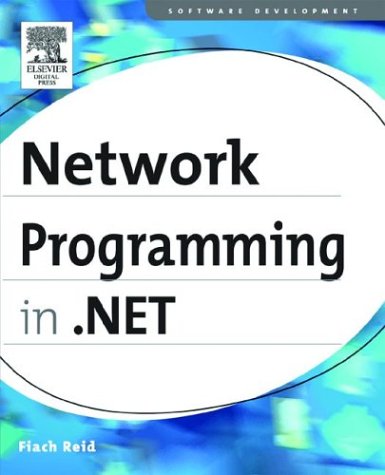
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AggregationMinMaxHelpers.cs
- Rect3DConverter.cs
- BrowserCapabilitiesCodeGenerator.cs
- FixUp.cs
- ToolZone.cs
- DataSourceControlBuilder.cs
- UseAttributeSetsAction.cs
- NamedPermissionSet.cs
- SizeFConverter.cs
- DiscoveryClientChannelBase.cs
- SimplePropertyEntry.cs
- CellParagraph.cs
- SplitContainerDesigner.cs
- OleDbPropertySetGuid.cs
- QilLiteral.cs
- TextServicesDisplayAttributePropertyRanges.cs
- DecimalStorage.cs
- WindowsFormsHostPropertyMap.cs
- DPTypeDescriptorContext.cs
- PathSegmentCollection.cs
- TextEndOfParagraph.cs
- DataMisalignedException.cs
- ProfileProvider.cs
- PaginationProgressEventArgs.cs
- BlockCollection.cs
- HTMLTagNameToTypeMapper.cs
- WebBaseEventKeyComparer.cs
- coordinator.cs
- DbCommandDefinition.cs
- ListParaClient.cs
- TargetControlTypeCache.cs
- FatalException.cs
- FileStream.cs
- SymbolTable.cs
- StringCollection.cs
- SapiRecognizer.cs
- StylusPointPropertyId.cs
- RadioButtonPopupAdapter.cs
- XmlSerializationGeneratedCode.cs
- XmlSerializerNamespaces.cs
- TableSectionStyle.cs
- TextContainerHelper.cs
- ResolveCompletedEventArgs.cs
- TCPListener.cs
- SQLDecimal.cs
- ChangeBlockUndoRecord.cs
- BindingSource.cs
- SettingsBase.cs
- WindowExtensionMethods.cs
- PropertyStore.cs
- SqlBulkCopy.cs
- DnsEndpointIdentity.cs
- LocalBuilder.cs
- EmptyEnumerator.cs
- JapaneseCalendar.cs
- ListViewDataItem.cs
- DesignTimeParseData.cs
- SQLUtility.cs
- AbstractExpressions.cs
- TransformValueSerializer.cs
- Int32Storage.cs
- MultiPartWriter.cs
- EventlogProvider.cs
- ListenerServiceInstallComponent.cs
- BrowserCapabilitiesFactoryBase.cs
- URI.cs
- MiniParameterInfo.cs
- TransformGroup.cs
- HttpProfileBase.cs
- ConstraintStruct.cs
- Scene3D.cs
- MembershipSection.cs
- DataBoundLiteralControl.cs
- LazyInitializer.cs
- DiagnosticTrace.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ElementsClipboardData.cs
- DataRelationCollection.cs
- isolationinterop.cs
- SqlDataSourceCache.cs
- XmlValidatingReaderImpl.cs
- GlobalizationAssembly.cs
- SqlNode.cs
- WSTrustFeb2005.cs
- OpCopier.cs
- SpecularMaterial.cs
- DataSourceProvider.cs
- FontTypeConverter.cs
- WpfMemberInvoker.cs
- SafeSystemMetrics.cs
- IItemContainerGenerator.cs
- UpdatePanelTrigger.cs
- CellIdBoolean.cs
- activationcontext.cs
- TextServicesManager.cs
- DecoderExceptionFallback.cs
- PermissionSetTriple.cs
- TextDecorationCollection.cs
- Form.cs
- PanelContainerDesigner.cs