Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / SqlClient / SqlGen / SqlBuilder.cs / 1 / SqlBuilder.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Text; using System.Data.SqlClient; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.SqlClient.SqlGen { ////// This class is like StringBuilder. While traversing the tree for the first time, /// we do not know all the strings that need to be appended e.g. things that need to be /// renamed, nested select statements etc. So, we use a builder that can collect /// all kinds of sql fragments. /// internal sealed class SqlBuilder : ISqlFragment { private List
Link Menu
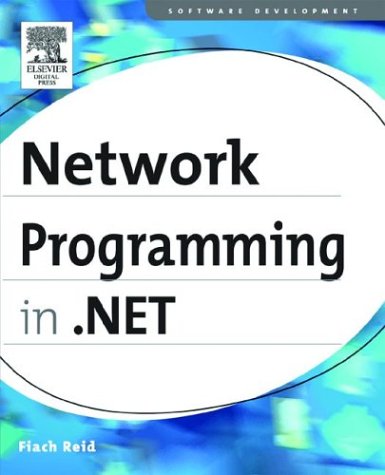
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreeRootTextBlock.cs
- TextInfo.cs
- TraceSection.cs
- Math.cs
- BitmapPalette.cs
- _LocalDataStoreMgr.cs
- Utils.cs
- TraceLog.cs
- Size3D.cs
- ServiceModelConfigurationSectionGroup.cs
- GridItemPatternIdentifiers.cs
- Pair.cs
- AsymmetricCryptoHandle.cs
- DocumentApplicationState.cs
- ObjectReaderCompiler.cs
- TemplateManager.cs
- Rect3D.cs
- CacheRequest.cs
- ErrorEventArgs.cs
- Model3DGroup.cs
- LogPolicy.cs
- CriticalExceptions.cs
- List.cs
- OpenFileDialog.cs
- _DomainName.cs
- _LazyAsyncResult.cs
- Label.cs
- ProfileServiceManager.cs
- XamlToRtfWriter.cs
- XmlTypeMapping.cs
- BuildManager.cs
- ModuleElement.cs
- log.cs
- _ConnectOverlappedAsyncResult.cs
- SplitterCancelEvent.cs
- CommonProperties.cs
- RotateTransform3D.cs
- XmlUtil.cs
- DataGridViewLinkCell.cs
- Schema.cs
- DataGridColumnCollection.cs
- Privilege.cs
- GridItemCollection.cs
- TableLayoutPanelCodeDomSerializer.cs
- EmptyCollection.cs
- CodeTypeParameter.cs
- ScrollableControl.cs
- TextSchema.cs
- autovalidator.cs
- LoginAutoFormat.cs
- ObfuscateAssemblyAttribute.cs
- NodeFunctions.cs
- CreateParams.cs
- QilTypeChecker.cs
- CommunicationException.cs
- MultiBinding.cs
- PointConverter.cs
- Utilities.cs
- FileDialogCustomPlacesCollection.cs
- RowVisual.cs
- DesignerOptionService.cs
- DocumentOutline.cs
- SimpleHandlerFactory.cs
- StringUtil.cs
- ConnectionManagementElement.cs
- SafeFileMapViewHandle.cs
- MailBnfHelper.cs
- AppDomainShutdownMonitor.cs
- Stroke2.cs
- LoadItemsEventArgs.cs
- sqlnorm.cs
- PeerNodeAddress.cs
- handlecollector.cs
- WhitespaceReader.cs
- StaticSiteMapProvider.cs
- DataServiceProviderWrapper.cs
- Object.cs
- TemplateLookupAction.cs
- GeneratedCodeAttribute.cs
- ToolTipService.cs
- StringExpressionSet.cs
- ListManagerBindingsCollection.cs
- SqlProfileProvider.cs
- WriteTimeStream.cs
- ToolBarPanel.cs
- JsonQueryStringConverter.cs
- SystemWebCachingSectionGroup.cs
- IEnumerable.cs
- EdmFunctionAttribute.cs
- _ListenerRequestStream.cs
- DesignerAttribute.cs
- HyperLink.cs
- DocumentsTrace.cs
- UTF7Encoding.cs
- RotateTransform3D.cs
- DeferredSelectedIndexReference.cs
- DesigntimeLicenseContext.cs
- RegexCompiler.cs
- SynchronizedInputPattern.cs
- OLEDB_Enum.cs