Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / ClientSideQueueItem.cs / 1 / ClientSideQueueItem.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class to create a queue on its own thread. // // History: // 06/17/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Automation; using System.Windows.Automation.Provider; using System; using System.Collections; using System.ComponentModel; using MS.Internal.Automation; using MS.Win32; namespace MS.Internal.Automation { // Worker class used to queue events that originated on the client side (e.g. // used by focus and top-level window tracking to queue WinEvent information). internal class ClientSideQueueItem : QueueItem { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal ClientSideQueueItem(Delegate clientCallback, AutomationElement srcEl, UiaCoreApi.UiaCacheRequest request, AutomationEventArgs e) { _clientCallback = clientCallback; _srcEl = srcEl; _request = request; _e = e; } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void Process() { // Grab properties for cache request here... AutomationElement src; if (_srcEl == null) { src = null; } else { UiaCoreApi.UiaCacheResponse response = UiaCoreApi.UiaGetUpdatedCache(_srcEl.RawNode, _request, UiaCoreApi.NormalizeState.View, null); src = CacheHelper.BuildAutomationElementsFromResponse(_request, response); } // if (!(src == null && _e.EventId == AutomationElement.AutomationFocusChangedEvent)) InvokeHandlers.InvokeClientHandler(_clientCallback, src, _e); } #endregion Internal Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private Delegate _clientCallback; // a client callback delegate private AutomationElement _srcEl; // the source element private UiaCoreApi.UiaCacheRequest _request; // shopping list for prefetch private AutomationEventArgs _e; // the event args for the callback #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class to create a queue on its own thread. // // History: // 06/17/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Automation; using System.Windows.Automation.Provider; using System; using System.Collections; using System.ComponentModel; using MS.Internal.Automation; using MS.Win32; namespace MS.Internal.Automation { // Worker class used to queue events that originated on the client side (e.g. // used by focus and top-level window tracking to queue WinEvent information). internal class ClientSideQueueItem : QueueItem { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal ClientSideQueueItem(Delegate clientCallback, AutomationElement srcEl, UiaCoreApi.UiaCacheRequest request, AutomationEventArgs e) { _clientCallback = clientCallback; _srcEl = srcEl; _request = request; _e = e; } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void Process() { // Grab properties for cache request here... AutomationElement src; if (_srcEl == null) { src = null; } else { UiaCoreApi.UiaCacheResponse response = UiaCoreApi.UiaGetUpdatedCache(_srcEl.RawNode, _request, UiaCoreApi.NormalizeState.View, null); src = CacheHelper.BuildAutomationElementsFromResponse(_request, response); } // if (!(src == null && _e.EventId == AutomationElement.AutomationFocusChangedEvent)) InvokeHandlers.InvokeClientHandler(_clientCallback, src, _e); } #endregion Internal Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private Delegate _clientCallback; // a client callback delegate private AutomationElement _srcEl; // the source element private UiaCoreApi.UiaCacheRequest _request; // shopping list for prefetch private AutomationEventArgs _e; // the event args for the callback #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
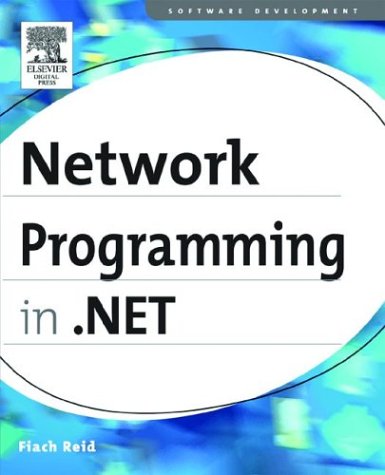
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataRecordInternal.cs
- MemberCollection.cs
- ContentFilePart.cs
- VectorAnimationBase.cs
- StateElementCollection.cs
- TimeIntervalCollection.cs
- DataGridViewLinkColumn.cs
- AsymmetricSignatureFormatter.cs
- TagMapInfo.cs
- Validator.cs
- InputBuffer.cs
- SequenceFullException.cs
- TextProperties.cs
- EntityExpressionVisitor.cs
- FixedSOMImage.cs
- MetadataItem.cs
- HostedBindingBehavior.cs
- RuntimeCompatibilityAttribute.cs
- ClientConfigurationHost.cs
- Asn1IntegerConverter.cs
- ContainerFilterService.cs
- ProfilePropertySettings.cs
- OdbcInfoMessageEvent.cs
- CategoryAttribute.cs
- x509store.cs
- TextBoxDesigner.cs
- PropertyStore.cs
- FacetValues.cs
- RSAPKCS1KeyExchangeFormatter.cs
- ButtonFieldBase.cs
- MenuEventArgs.cs
- JsonMessageEncoderFactory.cs
- BridgeDataReader.cs
- ContentControl.cs
- NamespaceMapping.cs
- CollectionEditor.cs
- BuiltInExpr.cs
- StreamReader.cs
- NativeMethods.cs
- InteropDesigner.xaml.cs
- MemberInfoSerializationHolder.cs
- WebBrowserSiteBase.cs
- SecureStringHasher.cs
- SqlDataSourceQueryConverter.cs
- HostedTransportConfigurationManager.cs
- RoleGroupCollection.cs
- _AutoWebProxyScriptHelper.cs
- DragStartedEventArgs.cs
- RadialGradientBrush.cs
- base64Transforms.cs
- CertificateElement.cs
- HealthMonitoringSectionHelper.cs
- TerminateDesigner.cs
- PolygonHotSpot.cs
- BamlTreeNode.cs
- TranslateTransform3D.cs
- UIElementPropertyUndoUnit.cs
- DataTableNameHandler.cs
- ElementHostAutomationPeer.cs
- FixedDSBuilder.cs
- UnsafeNativeMethods.cs
- TableCellAutomationPeer.cs
- Receive.cs
- DetailsViewInsertEventArgs.cs
- BasicHttpMessageSecurity.cs
- DecoderFallback.cs
- CanonicalXml.cs
- WmlControlAdapter.cs
- MenuEventArgs.cs
- SqlDataAdapter.cs
- CommunicationException.cs
- MemoryRecordBuffer.cs
- SqlFactory.cs
- BindingBase.cs
- BaseProcessor.cs
- EUCJPEncoding.cs
- XmlSchemaIdentityConstraint.cs
- BufferedReadStream.cs
- ICspAsymmetricAlgorithm.cs
- DataServiceRequest.cs
- XpsSerializationException.cs
- SynchronizedDispatch.cs
- ObjectHelper.cs
- WebScriptMetadataMessageEncoderFactory.cs
- WindowsFormsSectionHandler.cs
- WebException.cs
- ComplexPropertyEntry.cs
- IdentityReference.cs
- ChangeNode.cs
- XmlSchemaFacet.cs
- LineInfo.cs
- CategoryEditor.cs
- NGCPageContentSerializerAsync.cs
- Model3DCollection.cs
- HtmlTitle.cs
- CompoundFileStreamReference.cs
- ScrollBarAutomationPeer.cs
- QilValidationVisitor.cs
- DBDataPermissionAttribute.cs
- EncodingInfo.cs