Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Principal / WindowsPrincipal.cs / 1 / WindowsPrincipal.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // WindowsPrincipal.cs // // Group membership checks. // namespace System.Security.Principal { using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using Hashtable = System.Collections.Hashtable; [Serializable] [ComVisible(true)] public enum WindowsBuiltInRole { Administrator = 0x220, User = 0x221, Guest = 0x222, PowerUser = 0x223, AccountOperator = 0x224, SystemOperator = 0x225, PrintOperator = 0x226, BackupOperator = 0x227, Replicator = 0x228 } [Serializable()] [HostProtection(SecurityInfrastructure=true)] [ComVisible(true)] public class WindowsPrincipal : IPrincipal { private WindowsIdentity m_identity = null; // Following 3 fields are present purely for serialization compatability with Everett: not used in Whidbey #pragma warning disable 169 private String[] m_roles; private Hashtable m_rolesTable; private bool m_rolesLoaded; #pragma warning restore 169 // // Constructors. // private WindowsPrincipal () {} public WindowsPrincipal (WindowsIdentity ntIdentity) { if (ntIdentity == null) throw new ArgumentNullException("ntIdentity"); m_identity = ntIdentity; } // // Properties. // public virtual IIdentity Identity { get { return m_identity; } } // // Public methods. // public virtual bool IsInRole (string role) { if (role == null || role.Length == 0) return false; NTAccount ntAccount = new NTAccount(role); IdentityReferenceCollection source = new IdentityReferenceCollection(1); source.Add(ntAccount); IdentityReferenceCollection target = NTAccount.Translate(source, typeof(SecurityIdentifier), false); SecurityIdentifier sid = target[0] as SecurityIdentifier; if (sid == null) return false; return IsInRole(sid); } public virtual bool IsInRole (WindowsBuiltInRole role) { if (role < WindowsBuiltInRole.Administrator || role > WindowsBuiltInRole.Replicator) throw new ArgumentException(Environment.GetResourceString("Arg_EnumIllegalVal", (int)role), "role"); return IsInRole((int) role); } public virtual bool IsInRole (int rid) { SecurityIdentifier sid = new SecurityIdentifier(IdentifierAuthority.NTAuthority, new int[] {Win32Native.SECURITY_BUILTIN_DOMAIN_RID, rid}); return IsInRole(sid); } // This methods (with a SID parameter) is more general than the 2 overloads that accept a WindowsBuiltInRole or // a rid (as an int). It is also better from a performance standpoint than the overload that accepts a string. // The aformentioned overloads remain in this class since we do not want to introduce a // breaking change. However, this method should be used in all new applications and we should document this. [ComVisible(false)] public virtual bool IsInRole (SecurityIdentifier sid) { if (sid == null) throw new ArgumentNullException("sid"); // special case the anonymous identity. if (m_identity.TokenHandle.IsInvalid) return false; // CheckTokenMembership expects an impersonation token SafeTokenHandle token = SafeTokenHandle.InvalidHandle; if (m_identity.ImpersonationLevel == TokenImpersonationLevel.None) { if (!Win32Native.DuplicateTokenEx(m_identity.TokenHandle, (uint) TokenAccessLevels.Query, IntPtr.Zero, (uint) TokenImpersonationLevel.Identification, (uint) TokenType.TokenImpersonation, ref token)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); } bool isMember = false; // CheckTokenMembership will check if the SID is both present and enabled in the access token. if (!Win32Native.CheckTokenMembership((m_identity.ImpersonationLevel != TokenImpersonationLevel.None ? m_identity.TokenHandle : token), sid.BinaryForm, ref isMember)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); token.Dispose(); return isMember; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // WindowsPrincipal.cs // // Group membership checks. // namespace System.Security.Principal { using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using Hashtable = System.Collections.Hashtable; [Serializable] [ComVisible(true)] public enum WindowsBuiltInRole { Administrator = 0x220, User = 0x221, Guest = 0x222, PowerUser = 0x223, AccountOperator = 0x224, SystemOperator = 0x225, PrintOperator = 0x226, BackupOperator = 0x227, Replicator = 0x228 } [Serializable()] [HostProtection(SecurityInfrastructure=true)] [ComVisible(true)] public class WindowsPrincipal : IPrincipal { private WindowsIdentity m_identity = null; // Following 3 fields are present purely for serialization compatability with Everett: not used in Whidbey #pragma warning disable 169 private String[] m_roles; private Hashtable m_rolesTable; private bool m_rolesLoaded; #pragma warning restore 169 // // Constructors. // private WindowsPrincipal () {} public WindowsPrincipal (WindowsIdentity ntIdentity) { if (ntIdentity == null) throw new ArgumentNullException("ntIdentity"); m_identity = ntIdentity; } // // Properties. // public virtual IIdentity Identity { get { return m_identity; } } // // Public methods. // public virtual bool IsInRole (string role) { if (role == null || role.Length == 0) return false; NTAccount ntAccount = new NTAccount(role); IdentityReferenceCollection source = new IdentityReferenceCollection(1); source.Add(ntAccount); IdentityReferenceCollection target = NTAccount.Translate(source, typeof(SecurityIdentifier), false); SecurityIdentifier sid = target[0] as SecurityIdentifier; if (sid == null) return false; return IsInRole(sid); } public virtual bool IsInRole (WindowsBuiltInRole role) { if (role < WindowsBuiltInRole.Administrator || role > WindowsBuiltInRole.Replicator) throw new ArgumentException(Environment.GetResourceString("Arg_EnumIllegalVal", (int)role), "role"); return IsInRole((int) role); } public virtual bool IsInRole (int rid) { SecurityIdentifier sid = new SecurityIdentifier(IdentifierAuthority.NTAuthority, new int[] {Win32Native.SECURITY_BUILTIN_DOMAIN_RID, rid}); return IsInRole(sid); } // This methods (with a SID parameter) is more general than the 2 overloads that accept a WindowsBuiltInRole or // a rid (as an int). It is also better from a performance standpoint than the overload that accepts a string. // The aformentioned overloads remain in this class since we do not want to introduce a // breaking change. However, this method should be used in all new applications and we should document this. [ComVisible(false)] public virtual bool IsInRole (SecurityIdentifier sid) { if (sid == null) throw new ArgumentNullException("sid"); // special case the anonymous identity. if (m_identity.TokenHandle.IsInvalid) return false; // CheckTokenMembership expects an impersonation token SafeTokenHandle token = SafeTokenHandle.InvalidHandle; if (m_identity.ImpersonationLevel == TokenImpersonationLevel.None) { if (!Win32Native.DuplicateTokenEx(m_identity.TokenHandle, (uint) TokenAccessLevels.Query, IntPtr.Zero, (uint) TokenImpersonationLevel.Identification, (uint) TokenType.TokenImpersonation, ref token)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); } bool isMember = false; // CheckTokenMembership will check if the SID is both present and enabled in the access token. if (!Win32Native.CheckTokenMembership((m_identity.ImpersonationLevel != TokenImpersonationLevel.None ? m_identity.TokenHandle : token), sid.BinaryForm, ref isMember)) throw new SecurityException(Win32Native.GetMessage(Marshal.GetLastWin32Error())); token.Dispose(); return isMember; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
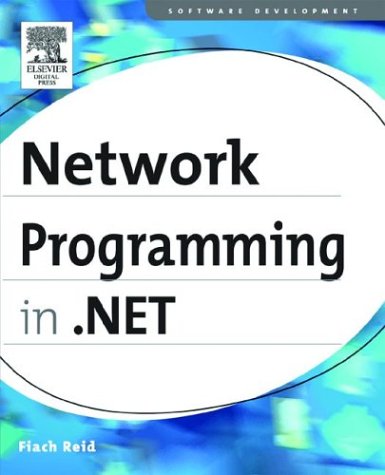
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SoapCodeExporter.cs
- SqlMetaData.cs
- NamespaceInfo.cs
- DataGridItem.cs
- DatatypeImplementation.cs
- ValidationPropertyAttribute.cs
- ServiceSettingsResponseInfo.cs
- IChannel.cs
- ScriptingScriptResourceHandlerSection.cs
- DataRow.cs
- ViewGenResults.cs
- SessionPageStatePersister.cs
- DataGridCaption.cs
- BrowserCapabilitiesFactoryBase.cs
- PixelFormatConverter.cs
- RecordBuilder.cs
- WebPageTraceListener.cs
- ImageSource.cs
- CodeDirectiveCollection.cs
- InOutArgument.cs
- Substitution.cs
- SqlTopReducer.cs
- NestedContainer.cs
- DetailsViewPageEventArgs.cs
- EdmError.cs
- XmlSchemas.cs
- RNGCryptoServiceProvider.cs
- MarshalByValueComponent.cs
- ClientOperation.cs
- ContentPlaceHolder.cs
- TreeBuilderBamlTranslator.cs
- ParenthesizePropertyNameAttribute.cs
- AutoGeneratedField.cs
- Stack.cs
- DesignerVerbCollection.cs
- JulianCalendar.cs
- followingquery.cs
- AnonymousIdentificationModule.cs
- StructuredTypeInfo.cs
- SiteMapNodeCollection.cs
- _FtpControlStream.cs
- TabItem.cs
- CatchBlock.cs
- TerminatorSinks.cs
- Form.cs
- XmlCharType.cs
- PtsHost.cs
- WebPartConnectionsConfigureVerb.cs
- FixedStringLookup.cs
- GetParentChain.cs
- LocationChangedEventArgs.cs
- ObjectAnimationBase.cs
- TemplateBindingExtensionConverter.cs
- Size.cs
- WebPartTransformer.cs
- TickBar.cs
- WebBrowserPermission.cs
- ScrollPatternIdentifiers.cs
- UxThemeWrapper.cs
- CompiledQueryCacheEntry.cs
- CallbackTimeoutsElement.cs
- ComponentEvent.cs
- Win32PrintDialog.cs
- ReadOnlyHierarchicalDataSourceView.cs
- WebPartDescriptionCollection.cs
- ReferencedCollectionType.cs
- FileDialog_Vista_Interop.cs
- Int16KeyFrameCollection.cs
- WebPartMovingEventArgs.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- ListViewItem.cs
- HttpHandler.cs
- TextDecorations.cs
- HyperLinkDataBindingHandler.cs
- FixedPage.cs
- GridItemCollection.cs
- Misc.cs
- WebConfigurationFileMap.cs
- CustomAttributeSerializer.cs
- InputLanguageSource.cs
- BuildProvidersCompiler.cs
- FixUpCollection.cs
- ScriptReference.cs
- MULTI_QI.cs
- PackWebRequest.cs
- RepeaterItemCollection.cs
- ServiceMetadataContractBehavior.cs
- ObjectDataProvider.cs
- XsdBuildProvider.cs
- RelOps.cs
- ParsedAttributeCollection.cs
- Bitmap.cs
- XNodeNavigator.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- MessageDecoder.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- CollectionChangedEventManager.cs
- XPathDocumentIterator.cs
- WorkflowExecutor.cs
- ProfileParameter.cs