Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / XmlUtils / System / Xml / Xsl / SourceLineInfo.cs / 1 / SourceLineInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Diagnostics; namespace System.Xml.Xsl { [DebuggerDisplay("{uriString} [{startLine},{startPos} -- {endLine},{endPos}]")] internal class SourceLineInfo : ISourceLineInfo { private string uriString; private int startLine; private int startPos; private int endLine; private int endPos; public SourceLineInfo(string uriString, int startLine, int startPos, int endLine, int endPos) { this.uriString = uriString; this.startLine = startLine; this.startPos = startPos; this.endLine = endLine; this.endPos = endPos; } public string Uri { get { return this.uriString; } } public int StartLine { get { return this.startLine; } } public int StartPos { get { return this.startPos ; } } public int EndLine { get { return this.endLine ; } } public int EndPos { get { return this.endPos ; } } internal void SetEndLinePos(int endLine, int endPos) { this.endLine = endLine; this.endPos = endPos; } ////// Magic number 0xfeefee is used in PDB to denote a section of IL that does not map to any user code. /// When VS debugger steps into IL marked with 0xfeefee, it will continue the step until it reaches /// some user code. /// private const int NoSourceMagicNumber = 0xfeefee; public static SourceLineInfo NoSource = new SourceLineInfo(string.Empty, NoSourceMagicNumber, 0, NoSourceMagicNumber, 0); public bool IsNoSource { get { return this.startLine == NoSourceMagicNumber; } } [Conditional("DEBUG")] public static void Validate(ISourceLineInfo lineInfo) { if (lineInfo.StartLine == 0 || lineInfo.StartLine == NoSourceMagicNumber) { Debug.Assert(lineInfo.StartLine == lineInfo.EndLine); Debug.Assert(lineInfo.StartPos == 0 && lineInfo.EndPos == 0); } else { Debug.Assert(0 < lineInfo.StartLine && lineInfo.StartLine <= lineInfo.EndLine); if (lineInfo.StartLine == lineInfo.EndLine) { Debug.Assert(0 < lineInfo.StartPos && lineInfo.StartPos < lineInfo.EndPos); } else { Debug.Assert(0 < lineInfo.StartPos && 0 < lineInfo.EndPos); } } } // Returns file path for local and network URIs. Used for PDB generating and error reporting. public static string GetFileName(string uriString) { Uri uri; if (uriString.Length != 0 && System.Uri.TryCreate(uriString, UriKind.Absolute, out uri) && uri.IsFile ) { return uri.LocalPath; } return uriString; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Diagnostics; namespace System.Xml.Xsl { [DebuggerDisplay("{uriString} [{startLine},{startPos} -- {endLine},{endPos}]")] internal class SourceLineInfo : ISourceLineInfo { private string uriString; private int startLine; private int startPos; private int endLine; private int endPos; public SourceLineInfo(string uriString, int startLine, int startPos, int endLine, int endPos) { this.uriString = uriString; this.startLine = startLine; this.startPos = startPos; this.endLine = endLine; this.endPos = endPos; } public string Uri { get { return this.uriString; } } public int StartLine { get { return this.startLine; } } public int StartPos { get { return this.startPos ; } } public int EndLine { get { return this.endLine ; } } public int EndPos { get { return this.endPos ; } } internal void SetEndLinePos(int endLine, int endPos) { this.endLine = endLine; this.endPos = endPos; } ////// Magic number 0xfeefee is used in PDB to denote a section of IL that does not map to any user code. /// When VS debugger steps into IL marked with 0xfeefee, it will continue the step until it reaches /// some user code. /// private const int NoSourceMagicNumber = 0xfeefee; public static SourceLineInfo NoSource = new SourceLineInfo(string.Empty, NoSourceMagicNumber, 0, NoSourceMagicNumber, 0); public bool IsNoSource { get { return this.startLine == NoSourceMagicNumber; } } [Conditional("DEBUG")] public static void Validate(ISourceLineInfo lineInfo) { if (lineInfo.StartLine == 0 || lineInfo.StartLine == NoSourceMagicNumber) { Debug.Assert(lineInfo.StartLine == lineInfo.EndLine); Debug.Assert(lineInfo.StartPos == 0 && lineInfo.EndPos == 0); } else { Debug.Assert(0 < lineInfo.StartLine && lineInfo.StartLine <= lineInfo.EndLine); if (lineInfo.StartLine == lineInfo.EndLine) { Debug.Assert(0 < lineInfo.StartPos && lineInfo.StartPos < lineInfo.EndPos); } else { Debug.Assert(0 < lineInfo.StartPos && 0 < lineInfo.EndPos); } } } // Returns file path for local and network URIs. Used for PDB generating and error reporting. public static string GetFileName(string uriString) { Uri uri; if (uriString.Length != 0 && System.Uri.TryCreate(uriString, UriKind.Absolute, out uri) && uri.IsFile ) { return uri.LocalPath; } return uriString; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
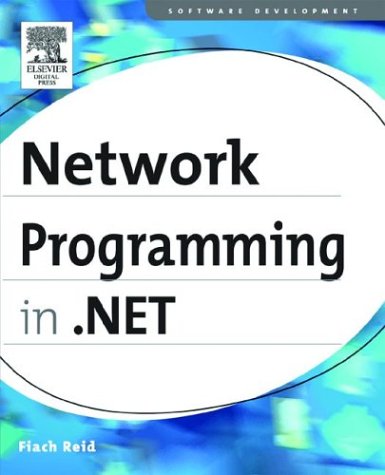
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScaleTransform.cs
- NamespaceDisplayAutomationPeer.cs
- StylusEditingBehavior.cs
- QueueNameHelper.cs
- ReadOnlyDataSource.cs
- LicFileLicenseProvider.cs
- ScrollEventArgs.cs
- XPathNodeList.cs
- SecurityCriticalDataForSet.cs
- EntitySqlQueryState.cs
- PersonalizationStateQuery.cs
- ByteStack.cs
- DetailsViewUpdateEventArgs.cs
- CompareValidator.cs
- SqlUserDefinedTypeAttribute.cs
- PowerModeChangedEventArgs.cs
- CalendarData.cs
- AutomationElementIdentifiers.cs
- ListArgumentProvider.cs
- ConnectionInterfaceCollection.cs
- SplashScreen.cs
- InlineUIContainer.cs
- WorkflowViewService.cs
- Number.cs
- SmtpFailedRecipientsException.cs
- ProcessHostConfigUtils.cs
- VectorValueSerializer.cs
- Opcode.cs
- AnnotationResource.cs
- CompilerErrorCollection.cs
- ContentTextAutomationPeer.cs
- Timer.cs
- DefaultCommandConverter.cs
- ComponentManagerBroker.cs
- State.cs
- OperationAbortedException.cs
- SwitchAttribute.cs
- CancelAsyncOperationRequest.cs
- TableCell.cs
- SizeAnimationUsingKeyFrames.cs
- SchemaMapping.cs
- DataControlButton.cs
- MenuItemAutomationPeer.cs
- PolygonHotSpot.cs
- GeometryGroup.cs
- AddToCollection.cs
- WebServiceFault.cs
- FixedPageProcessor.cs
- RolePrincipal.cs
- StrongNameUtility.cs
- WebRequestModulesSection.cs
- DirectoryInfo.cs
- SystemEvents.cs
- RtfToken.cs
- GeometryGroup.cs
- WebSysDisplayNameAttribute.cs
- PageAdapter.cs
- CubicEase.cs
- UndirectedGraph.cs
- Hash.cs
- DictionaryBase.cs
- ScriptRef.cs
- SubpageParaClient.cs
- ListItem.cs
- HtmlEmptyTagControlBuilder.cs
- DataGridPagerStyle.cs
- ChangePassword.cs
- XPathScanner.cs
- ObjectConverter.cs
- DiscardableAttribute.cs
- HttpServerUtilityBase.cs
- WorkflowInlining.cs
- Math.cs
- TrustManagerPromptUI.cs
- Convert.cs
- XmlDictionaryString.cs
- SchemaContext.cs
- XMLUtil.cs
- DateTime.cs
- Point3DKeyFrameCollection.cs
- InvalidOperationException.cs
- TextMarkerSource.cs
- PointLight.cs
- WmfPlaceableFileHeader.cs
- TypeConverterValueSerializer.cs
- AlphaSortedEnumConverter.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- BaseTemplateParser.cs
- ThreadExceptionDialog.cs
- OleDbReferenceCollection.cs
- HtmlLink.cs
- DataGridViewColumnEventArgs.cs
- SourceChangedEventArgs.cs
- SystemWebCachingSectionGroup.cs
- OdbcParameter.cs
- BuildManagerHost.cs
- AlternateViewCollection.cs
- SerializationException.cs
- StsCommunicationException.cs
- BindToObject.cs