Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / schema / SchemaAttDef.cs / 1 / SchemaAttDef.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Diagnostics; /* * This class describes an attribute type and potential values. * This encapsulates the information for one Attdef * in an * Attlist in a DTD as described below: */ internal sealed class SchemaAttDef : SchemaDeclBase { public enum Reserve { None, XmlSpace, XmlLang }; private Reserve reserved; // indicate the attribute type, such as xml:lang or xml:space private String defExpanded; // default value in its expanded form private bool hasEntityRef; // whether there is any entity reference in the default value XmlSchemaAttribute schemaAttribute; private bool defaultValueChecked; int lineNum; int linePos; int valueLineNum; int valueLinePos; public static readonly SchemaAttDef Empty = new SchemaAttDef(); public SchemaAttDef(XmlQualifiedName name, String prefix) : base(name, prefix) { reserved = Reserve.None; } private SchemaAttDef() {} public SchemaAttDef Clone() { return (SchemaAttDef) MemberwiseClone(); } internal int LinePos { get { return linePos; } set { linePos = value; } } internal int LineNum { get { return lineNum; } set { lineNum = value; } } internal int ValueLinePos { get { return valueLinePos; } set { valueLinePos = value; } } internal int ValueLineNum { get { return valueLineNum; } set { valueLineNum = value; } } internal bool DefaultValueChecked { get { return defaultValueChecked; } } public String DefaultValueExpanded { get { return(defExpanded != null) ? defExpanded : String.Empty;} set { defExpanded = value;} } public Reserve Reserved { get { return reserved;} set { reserved = value;} } public bool HasEntityRef { get { return hasEntityRef;} set { hasEntityRef = value;} } public XmlSchemaAttribute SchemaAttribute { get { return schemaAttribute; } set { schemaAttribute = value; } } public void CheckXmlSpace(ValidationEventHandler eventhandler) { if (datatype.TokenizedType == XmlTokenizedType.ENUMERATION && (values != null) && (values.Count <= 2)) { String s1 = values[0].ToString(); if (values.Count == 2) { String s2 = values[1].ToString(); if ((s1 == "default" || s2 == "default") && (s1 == "preserve" || s2 == "preserve")) { return; } } else { if (s1 == "default" || s1 == "preserve") { return; } } } eventhandler(this, new ValidationEventArgs(new XmlSchemaException(Res.Sch_XmlSpace, string.Empty))); } internal void CheckDefaultValue( SchemaInfo schemaInfo, IDtdParserAdapter readerAdapter ) { DtdValidator.CheckDefaultValue( this, schemaInfo, readerAdapter ); defaultValueChecked = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
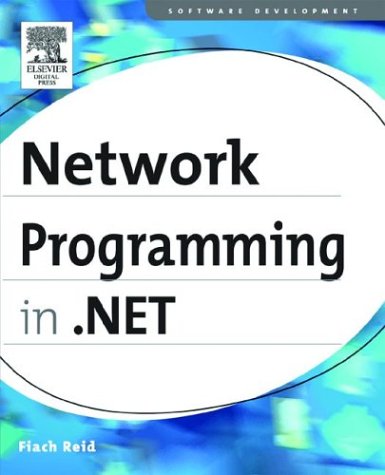
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidatingPropertiesEventArgs.cs
- RoutedUICommand.cs
- CancellableEnumerable.cs
- SqlStatistics.cs
- SafeFindHandle.cs
- StylusButtonEventArgs.cs
- FloatMinMaxAggregationOperator.cs
- MediaPlayerState.cs
- __FastResourceComparer.cs
- PolyQuadraticBezierSegment.cs
- CommentEmitter.cs
- HwndSourceKeyboardInputSite.cs
- AutomationPropertyInfo.cs
- TextEffectResolver.cs
- AssemblyBuilder.cs
- XsltSettings.cs
- LayoutTable.cs
- TypeDescriptionProvider.cs
- WizardStepBase.cs
- CookielessHelper.cs
- Type.cs
- Hashtable.cs
- FormsAuthenticationConfiguration.cs
- IisTraceListener.cs
- ResourceIDHelper.cs
- RuntimeConfig.cs
- SiteMapHierarchicalDataSourceView.cs
- WorkflowInstanceContextProvider.cs
- IndexedString.cs
- xml.cs
- StringToken.cs
- DataControlFieldCell.cs
- SafeNativeMethods.cs
- RunInstallerAttribute.cs
- TextBox.cs
- ToolbarAUtomationPeer.cs
- XPathAncestorIterator.cs
- DataSourceDesigner.cs
- SubclassTypeValidator.cs
- HostedBindingBehavior.cs
- ConfigurationPropertyAttribute.cs
- CompilationSection.cs
- ProjectionCamera.cs
- CodeSubDirectory.cs
- Config.cs
- SequentialOutput.cs
- ActionMessageFilter.cs
- XmlSchemaChoice.cs
- DataGridViewTextBoxEditingControl.cs
- Monitor.cs
- MenuCommand.cs
- SubclassTypeValidator.cs
- DataSourceCache.cs
- SQLInt32.cs
- ExpressionBuilderContext.cs
- OdbcException.cs
- SQLMoneyStorage.cs
- SortDescriptionCollection.cs
- AttachmentCollection.cs
- CodeMemberEvent.cs
- DescendentsWalker.cs
- MemberInfoSerializationHolder.cs
- Divide.cs
- EdmConstants.cs
- FieldToken.cs
- Component.cs
- TCPClient.cs
- KnowledgeBase.cs
- FrameworkName.cs
- FieldTemplateFactory.cs
- ApplicationProxyInternal.cs
- PersonalizationProvider.cs
- CatalogZoneBase.cs
- SQLUtility.cs
- IndexedEnumerable.cs
- FullTrustAssembliesSection.cs
- MessageTraceRecord.cs
- ServiceDescriptionImporter.cs
- SystemWebExtensionsSectionGroup.cs
- WizardForm.cs
- EditorPart.cs
- XmlIlTypeHelper.cs
- EventRecordWrittenEventArgs.cs
- BlurEffect.cs
- VarInfo.cs
- BitmapEffectDrawing.cs
- DesignBindingEditor.cs
- SerTrace.cs
- SafeProcessHandle.cs
- ListBoxDesigner.cs
- OracleRowUpdatedEventArgs.cs
- WebBaseEventKeyComparer.cs
- WebPartMovingEventArgs.cs
- LinqExpressionNormalizer.cs
- WeakReference.cs
- ChannelPoolSettingsElement.cs
- Vector3DAnimationBase.cs
- InstanceCreationEditor.cs
- EnumBuilder.cs
- PartitionedStreamMerger.cs