Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / RootBuilder.cs / 2 / RootBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Implements the root builder * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web.UI { using System.Runtime.InteropServices; using System; using System.Collections; using System.IO; using System.Reflection; using System.Web; using System.Web.Util; using System.Security.Permissions; ////// /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RootBuilder : TemplateBuilder { private MainTagNameToTypeMapper _typeMapper; // Contains a mapping of all objects to their associated builders private IDictionary _builtObjects; public RootBuilder() { } ///[To be supplied.] ////// public RootBuilder(TemplateParser parser) { } public IDictionary BuiltObjects { get { // Store any objects created by this control builder // so we can properly persist items if (_builtObjects == null) { _builtObjects = new Hashtable(ReferenceKeyComparer.Default); } return _builtObjects; } } internal void SetTypeMapper(MainTagNameToTypeMapper typeMapper) { _typeMapper = typeMapper; } ///[To be supplied.] ////// public override Type GetChildControlType(string tagName, IDictionary attribs) { // Is there a type to handle this control Type type = _typeMapper.GetControlType(tagName, attribs, true /*fAllowHtmlTags*/); return type; } internal override void PrepareNoCompilePageSupport() { base.PrepareNoCompilePageSupport(); // This is needed to break any connection with the TemplateParser, allowing it // to be fully collected when the parsing is complete _typeMapper = null; } private class ReferenceKeyComparer : IComparer, IEqualityComparer { internal static readonly ReferenceKeyComparer Default = new ReferenceKeyComparer(); bool IEqualityComparer.Equals(object x, object y) { return Object.ReferenceEquals(x, y); } int IEqualityComparer.GetHashCode(object obj) { return obj.GetHashCode(); } int IComparer.Compare(object x, object y) { if (Object.ReferenceEquals(x, y)) { return 0; } if (x == null) { return -1; } if (y == null) { return 1; } return 1; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Implements the root builder * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web.UI { using System.Runtime.InteropServices; using System; using System.Collections; using System.IO; using System.Reflection; using System.Web; using System.Web.Util; using System.Security.Permissions; ////// /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RootBuilder : TemplateBuilder { private MainTagNameToTypeMapper _typeMapper; // Contains a mapping of all objects to their associated builders private IDictionary _builtObjects; public RootBuilder() { } ///[To be supplied.] ////// public RootBuilder(TemplateParser parser) { } public IDictionary BuiltObjects { get { // Store any objects created by this control builder // so we can properly persist items if (_builtObjects == null) { _builtObjects = new Hashtable(ReferenceKeyComparer.Default); } return _builtObjects; } } internal void SetTypeMapper(MainTagNameToTypeMapper typeMapper) { _typeMapper = typeMapper; } ///[To be supplied.] ////// public override Type GetChildControlType(string tagName, IDictionary attribs) { // Is there a type to handle this control Type type = _typeMapper.GetControlType(tagName, attribs, true /*fAllowHtmlTags*/); return type; } internal override void PrepareNoCompilePageSupport() { base.PrepareNoCompilePageSupport(); // This is needed to break any connection with the TemplateParser, allowing it // to be fully collected when the parsing is complete _typeMapper = null; } private class ReferenceKeyComparer : IComparer, IEqualityComparer { internal static readonly ReferenceKeyComparer Default = new ReferenceKeyComparer(); bool IEqualityComparer.Equals(object x, object y) { return Object.ReferenceEquals(x, y); } int IEqualityComparer.GetHashCode(object obj) { return obj.GetHashCode(); } int IComparer.Compare(object x, object y) { if (Object.ReferenceEquals(x, y)) { return 0; } if (x == null) { return -1; } if (y == null) { return 1; } return 1; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
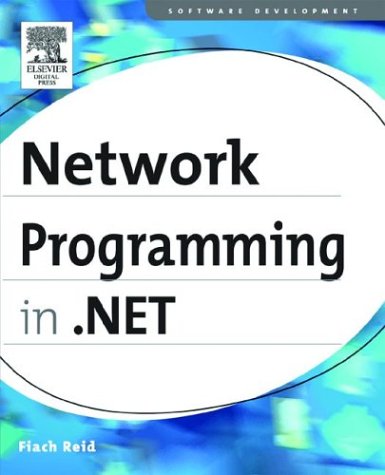
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QilPatternFactory.cs
- StorageInfo.cs
- PermissionRequestEvidence.cs
- MessageQueuePermissionAttribute.cs
- FontUnitConverter.cs
- PropertyValue.cs
- ELinqQueryState.cs
- ModelItem.cs
- SqlCacheDependency.cs
- ViewManager.cs
- TemplateKey.cs
- SqlFacetAttribute.cs
- TaskResultSetter.cs
- ForEachAction.cs
- CodeTypeMember.cs
- EmbeddedMailObject.cs
- BooleanAnimationUsingKeyFrames.cs
- ReliableOutputSessionChannel.cs
- shaperfactoryquerycachekey.cs
- WebPartConnectVerb.cs
- RootBrowserWindowAutomationPeer.cs
- EventWaitHandleSecurity.cs
- WrappedReader.cs
- SymbolDocumentGenerator.cs
- DeviceContexts.cs
- WebEvents.cs
- FormsAuthentication.cs
- DataMemberAttribute.cs
- VerificationException.cs
- FillBehavior.cs
- ZipIOExtraFieldZip64Element.cs
- ZipIORawDataFileBlock.cs
- NaturalLanguageHyphenator.cs
- HttpPostedFile.cs
- EndpointConfigContainer.cs
- SQLSingle.cs
- Activator.cs
- OuterGlowBitmapEffect.cs
- ObjectStateFormatter.cs
- EditCommandColumn.cs
- DisplayMemberTemplateSelector.cs
- UserNameSecurityToken.cs
- RSACryptoServiceProvider.cs
- WebPartConnectionsCancelVerb.cs
- WindowVisualStateTracker.cs
- OdbcStatementHandle.cs
- XPathArrayIterator.cs
- DateTimeFormatInfoScanner.cs
- DllHostInitializer.cs
- bindurihelper.cs
- IntPtr.cs
- VectorCollectionValueSerializer.cs
- FilterException.cs
- DataColumnPropertyDescriptor.cs
- MDIControlStrip.cs
- InkCollectionBehavior.cs
- _HeaderInfo.cs
- RefExpr.cs
- FormViewRow.cs
- ExceptionList.cs
- SingleBodyParameterMessageFormatter.cs
- TargetConverter.cs
- ProxyWebPart.cs
- StylusPointPropertyId.cs
- ResourceContainer.cs
- CompiledIdentityConstraint.cs
- ObjectReaderCompiler.cs
- CqlGenerator.cs
- CriticalHandle.cs
- InputProcessorProfilesLoader.cs
- ContainsSearchOperator.cs
- TreeNodeBinding.cs
- IIS7UserPrincipal.cs
- RawStylusInput.cs
- StoreItemCollection.cs
- EntityConnection.cs
- UnsafeNativeMethods.cs
- PolicyManager.cs
- AppDomainResourcePerfCounters.cs
- DataRecordInfo.cs
- EndGetFileNameFromUserRequest.cs
- BuildResult.cs
- PoisonMessageException.cs
- MsmqBindingFilter.cs
- BindingFormattingDialog.cs
- BasicBrowserDialog.designer.cs
- TypeForwardedToAttribute.cs
- EditableRegion.cs
- ListBindingConverter.cs
- ManagementException.cs
- PointAnimation.cs
- SafeFileMappingHandle.cs
- ClientSideProviderDescription.cs
- IdentitySection.cs
- TreeViewDesigner.cs
- GorillaCodec.cs
- Stack.cs
- UidPropertyAttribute.cs
- ListView.cs
- TypedElement.cs