Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Validation / ExtentKey.cs / 1 / ExtentKey.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.Structures { // This class represents the key of constraint on values that a relation slot may have internal class ExtentKey : InternalBase { #region Constructors // effects: Creates a key object for an extent (present in each MemberPath) // with the fields corresponding to keyFields internal ExtentKey(IEnumerablekeyFields) { m_keyFields = new List (keyFields); } #endregion #region Fields // All the key fields in an entity set private List m_keyFields; #endregion #region Properties internal IEnumerable KeyFields { get { return m_keyFields; } } #endregion #region Methods // effects: Determines all the keys (unique and primary for // entityType) for entityType and returns a key. "prefix" gives the // path of the extent or end of a relationship in a relationship set // -- prefix is prepended to the entity's key fields to get the full memberpath internal static List GetKeysForEntityType(MemberPath prefix, EntityType entityType) { // CHANGE_[....]_MULTIPLE_KEYS: currently there is a single key only. Need to support // keys inside complex types + unique keys ExtentKey key = GetPrimaryKeyForEntityType(prefix, entityType); List keys = new List (); keys.Add(key); return keys; } // effects: Returns the key for entityType prefixed with prefix (for // its memberPath) internal static ExtentKey GetPrimaryKeyForEntityType(MemberPath prefix, EntityType entityType) { List keyFields = new List (); foreach (EdmMember keyMember in entityType.KeyMembers) { Debug.Assert(keyMember != null, "Bogus key member in metadata"); keyFields.Add(new MemberPath(prefix, keyMember)); } // Just have one key for now ExtentKey key = new ExtentKey(keyFields); return key; } // effects: Returns a key correspnding to all the fields in different // ends of relationtype prefixed with "prefix" internal static ExtentKey GetKeyForRelationType(MemberPath prefix, AssociationType relationType) { List keyFields = new List (); foreach (AssociationEndMember endMember in relationType.AssociationEndMembers) { MemberPath endPrefix = new MemberPath(prefix, endMember); EntityType entityType = MetadataHelper.GetEntityTypeForEnd(endMember); ExtentKey primaryKey = GetPrimaryKeyForEntityType(endPrefix, entityType); keyFields.AddRange(primaryKey.KeyFields); } ExtentKey key = new ExtentKey(keyFields); return key; } internal string ToUserString() { string result = StringUtil.ToCommaSeparatedStringSorted(m_keyFields); return result; } internal override void ToCompactString(StringBuilder builder) { StringUtil.ToCommaSeparatedStringSorted(builder, m_keyFields); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Metadata.Edm; namespace System.Data.Mapping.ViewGeneration.Structures { // This class represents the key of constraint on values that a relation slot may have internal class ExtentKey : InternalBase { #region Constructors // effects: Creates a key object for an extent (present in each MemberPath) // with the fields corresponding to keyFields internal ExtentKey(IEnumerablekeyFields) { m_keyFields = new List (keyFields); } #endregion #region Fields // All the key fields in an entity set private List m_keyFields; #endregion #region Properties internal IEnumerable KeyFields { get { return m_keyFields; } } #endregion #region Methods // effects: Determines all the keys (unique and primary for // entityType) for entityType and returns a key. "prefix" gives the // path of the extent or end of a relationship in a relationship set // -- prefix is prepended to the entity's key fields to get the full memberpath internal static List GetKeysForEntityType(MemberPath prefix, EntityType entityType) { // CHANGE_[....]_MULTIPLE_KEYS: currently there is a single key only. Need to support // keys inside complex types + unique keys ExtentKey key = GetPrimaryKeyForEntityType(prefix, entityType); List keys = new List (); keys.Add(key); return keys; } // effects: Returns the key for entityType prefixed with prefix (for // its memberPath) internal static ExtentKey GetPrimaryKeyForEntityType(MemberPath prefix, EntityType entityType) { List keyFields = new List (); foreach (EdmMember keyMember in entityType.KeyMembers) { Debug.Assert(keyMember != null, "Bogus key member in metadata"); keyFields.Add(new MemberPath(prefix, keyMember)); } // Just have one key for now ExtentKey key = new ExtentKey(keyFields); return key; } // effects: Returns a key correspnding to all the fields in different // ends of relationtype prefixed with "prefix" internal static ExtentKey GetKeyForRelationType(MemberPath prefix, AssociationType relationType) { List keyFields = new List (); foreach (AssociationEndMember endMember in relationType.AssociationEndMembers) { MemberPath endPrefix = new MemberPath(prefix, endMember); EntityType entityType = MetadataHelper.GetEntityTypeForEnd(endMember); ExtentKey primaryKey = GetPrimaryKeyForEntityType(endPrefix, entityType); keyFields.AddRange(primaryKey.KeyFields); } ExtentKey key = new ExtentKey(keyFields); return key; } internal string ToUserString() { string result = StringUtil.ToCommaSeparatedStringSorted(m_keyFields); return result; } internal override void ToCompactString(StringBuilder builder) { StringUtil.ToCommaSeparatedStringSorted(builder, m_keyFields); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
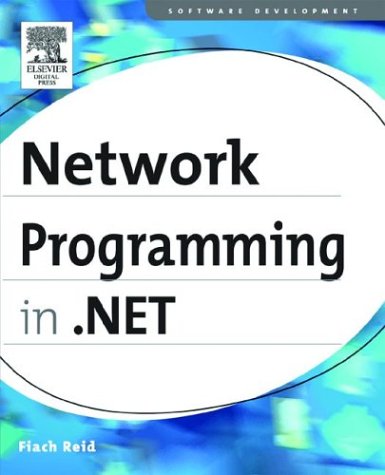
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeNodeMouseHoverEvent.cs
- TextClipboardData.cs
- TrustLevel.cs
- MsmqIntegrationChannelFactory.cs
- SystemIcons.cs
- SrgsDocumentParser.cs
- SamlAuthorizationDecisionStatement.cs
- ObjectIDGenerator.cs
- TemplateEditingService.cs
- WorkflowInstanceExtensionManager.cs
- Timeline.cs
- XPathNodeHelper.cs
- CompiledQuery.cs
- TcpSocketManager.cs
- MemoryRecordBuffer.cs
- UserNameSecurityToken.cs
- SqlParameter.cs
- StateRuntime.cs
- DataStorage.cs
- StringExpressionSet.cs
- DataRowComparer.cs
- ProfilePropertySettings.cs
- TextBox.cs
- PerformanceCountersElement.cs
- LogLogRecordEnumerator.cs
- ReadOnlyPropertyMetadata.cs
- BehaviorEditorPart.cs
- XmlSchemaIdentityConstraint.cs
- Win32.cs
- GeneralTransform3D.cs
- Lease.cs
- SymmetricAlgorithm.cs
- KeyInfo.cs
- GeometryDrawing.cs
- TextRunCacheImp.cs
- FileRegion.cs
- wgx_commands.cs
- SplitterCancelEvent.cs
- DefaultMemberAttribute.cs
- TreeNodeEventArgs.cs
- OptimalTextSource.cs
- securitymgrsite.cs
- DataGridViewButtonColumn.cs
- IODescriptionAttribute.cs
- ContextBase.cs
- HashCodeCombiner.cs
- wmiprovider.cs
- MouseEvent.cs
- ImageMapEventArgs.cs
- Point4DValueSerializer.cs
- PropertyValue.cs
- StringUtil.cs
- HttpRuntimeSection.cs
- SiteMapProvider.cs
- ClientOptions.cs
- ListComponentEditorPage.cs
- _FtpDataStream.cs
- CheckBoxAutomationPeer.cs
- SecurityCookieModeValidator.cs
- PerformanceCounters.cs
- AssociationSetMetadata.cs
- SplitterCancelEvent.cs
- ToolStripStatusLabel.cs
- List.cs
- SqlGatherProducedAliases.cs
- SocketInformation.cs
- MobileUITypeEditor.cs
- securitycriticaldataClass.cs
- ControlEvent.cs
- PenCursorManager.cs
- LingerOption.cs
- UiaCoreTypesApi.cs
- RelatedPropertyManager.cs
- Message.cs
- basenumberconverter.cs
- LocatorBase.cs
- StylusPointProperty.cs
- PrimitiveType.cs
- CaseStatementSlot.cs
- TextMarkerSource.cs
- ViewUtilities.cs
- ChtmlTextWriter.cs
- HttpCookiesSection.cs
- Token.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- CacheSection.cs
- WindowsProgressbar.cs
- SqlDataRecord.cs
- EditorPartChrome.cs
- LocatorBase.cs
- ContainerControl.cs
- Stack.cs
- OdbcFactory.cs
- TabletDeviceInfo.cs
- ReflectEventDescriptor.cs
- ExpressionBindingCollection.cs
- ObjectListField.cs
- ParamArrayAttribute.cs
- PropertyGridView.cs
- ProcessHostMapPath.cs