Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Security / RightsManagement / UseLicense.cs / 1 / UseLicense.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents the Use Lciense which enables end users to // consume protected content. // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Windows; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; using MS.Internal.Security.RightsManagement; using MS.Internal.Utility; // Disable message about unknown message numbers so as to allow the suppression // of PreSharp warnings (whose numbers are unknown to the compiler). #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// This class represents the Use Lciense which enables end users to consume protected content. /// ////// Critical: This class expose access to methods that eventually do one or more of the the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attribute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class UseLicense { ////// This constructor accepts the serialized form of a use license, and builds an instance of the classs based on that. /// public UseLicense(string useLicense) { SecurityHelper.DemandRightsManagementPermission(); if (useLicense == null) { throw new ArgumentNullException("useLicense"); } _serializedUseLicense = useLicense; ///////////////// // parse out the Content Id GUID ///////////////// string contentId; string contentIdType; ClientSession.GetContentIdFromLicense(_serializedUseLicense, out contentId, out contentIdType); if (contentId == null) { throw new RightsManagementException(RightsManagementFailureCode.InvalidLicense); } else { _contentId = new Guid(contentId); } ///////////////// // Get Owner information from the license ///////////////// _owner = ClientSession.ExtractUserFromCertificateChain(_serializedUseLicense); ///////////////// // Get ApplicationSpecific Data Dictionary ///////////////// _applicationSpecificDataDictionary = new ReadOnlyDictionary(ClientSession.ExtractApplicationSpecificDataFromLicense(_serializedUseLicense)); } /// /// This constructor accepts the serialized form of a use license, and builds an instance of the classs based on that. /// public ContentUser Owner { get { SecurityHelper.DemandRightsManagementPermission(); return _owner; } } ////// The ContentId is created by the publisher and can be used to match content to UseLicense and PublishLicenses. /// public Guid ContentId { get { SecurityHelper.DemandRightsManagementPermission(); return _contentId; } } ////// Returns the original XrML string that was used to deserialize the Use License /// public override string ToString() { SecurityHelper.DemandRightsManagementPermission(); return _serializedUseLicense; } ////// This function allows an application to examine or exercise the rights on a locally stored license. /// public CryptoProvider Bind (SecureEnvironment secureEnvironment) { SecurityHelper.DemandRightsManagementPermission(); if (secureEnvironment == null) { throw new ArgumentNullException("secureEnvironment"); } // The SecureEnvironment constructor makes sure ClientSession cannot be null. // Accordingly suppressing preSharp warning about having to validate ClientSession. #pragma warning suppress 6506 return secureEnvironment.ClientSession.TryBindUseLicenseToAllIdentites(_serializedUseLicense); } ////// ApplicationData data dictionary contains values that are passed from publishing /// application to a consuming application. One data pair that is processed by a Rights /// Management Services (RMS) server is the string pair "Allow_Server_Editing"/"True". /// When an issuance license has this value pair, it will allow the service, or any trusted /// service, to reuse the content key. The pair "NOLICCACHE" / "1" is expected to control /// Use License embedding policy of the consuming applications. If it is set to 1, applications /// are expected not to embed the Use License into the document. /// public IDictionaryApplicationData { get { SecurityHelper.DemandRightsManagementPermission(); return _applicationSpecificDataDictionary; } } /// /// Test for equality. /// public override bool Equals(object x) { SecurityHelper.DemandRightsManagementPermission(); if (x == null) return false; // Standard behavior. if (x.GetType() != GetType()) return false; // Not the same type. // Note that because of the GetType() checking above, the casting must be valid. UseLicense obj = (UseLicense)x; return (String.CompareOrdinal(_serializedUseLicense, obj._serializedUseLicense) == 0); } ////// Compute hash code. /// public override int GetHashCode() { SecurityHelper.DemandRightsManagementPermission(); return _serializedUseLicense.GetHashCode(); } private string _serializedUseLicense; private Guid _contentId; private ContentUser _owner = null; private IDictionary_applicationSpecificDataDictionary = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: This class represents the Use Lciense which enables end users to // consume protected content. // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Windows; using SecurityHelper=MS.Internal.WindowsBase.SecurityHelper; using MS.Internal.Security.RightsManagement; using MS.Internal.Utility; // Disable message about unknown message numbers so as to allow the suppression // of PreSharp warnings (whose numbers are unknown to the compiler). #pragma warning disable 1634, 1691 namespace System.Security.RightsManagement { ////// This class represents the Use Lciense which enables end users to consume protected content. /// ////// Critical: This class expose access to methods that eventually do one or more of the the following /// 1. call into unmanaged code /// 2. affects state/data that will eventually cross over unmanaged code boundary /// 3. Return some RM related information which is considered private /// /// TreatAsSafe: This attribute automatically applied to all public entry points. All the public entry points have /// Demands for RightsManagementPermission at entry to counter the possible attacks that do /// not lead to the unamanged code directly(which is protected by another Demand there) but rather leave /// some status/data behind which eventually might cross the unamanaged boundary. /// [SecurityCritical(SecurityCriticalScope.Everything)] public class UseLicense { ////// This constructor accepts the serialized form of a use license, and builds an instance of the classs based on that. /// public UseLicense(string useLicense) { SecurityHelper.DemandRightsManagementPermission(); if (useLicense == null) { throw new ArgumentNullException("useLicense"); } _serializedUseLicense = useLicense; ///////////////// // parse out the Content Id GUID ///////////////// string contentId; string contentIdType; ClientSession.GetContentIdFromLicense(_serializedUseLicense, out contentId, out contentIdType); if (contentId == null) { throw new RightsManagementException(RightsManagementFailureCode.InvalidLicense); } else { _contentId = new Guid(contentId); } ///////////////// // Get Owner information from the license ///////////////// _owner = ClientSession.ExtractUserFromCertificateChain(_serializedUseLicense); ///////////////// // Get ApplicationSpecific Data Dictionary ///////////////// _applicationSpecificDataDictionary = new ReadOnlyDictionary(ClientSession.ExtractApplicationSpecificDataFromLicense(_serializedUseLicense)); } /// /// This constructor accepts the serialized form of a use license, and builds an instance of the classs based on that. /// public ContentUser Owner { get { SecurityHelper.DemandRightsManagementPermission(); return _owner; } } ////// The ContentId is created by the publisher and can be used to match content to UseLicense and PublishLicenses. /// public Guid ContentId { get { SecurityHelper.DemandRightsManagementPermission(); return _contentId; } } ////// Returns the original XrML string that was used to deserialize the Use License /// public override string ToString() { SecurityHelper.DemandRightsManagementPermission(); return _serializedUseLicense; } ////// This function allows an application to examine or exercise the rights on a locally stored license. /// public CryptoProvider Bind (SecureEnvironment secureEnvironment) { SecurityHelper.DemandRightsManagementPermission(); if (secureEnvironment == null) { throw new ArgumentNullException("secureEnvironment"); } // The SecureEnvironment constructor makes sure ClientSession cannot be null. // Accordingly suppressing preSharp warning about having to validate ClientSession. #pragma warning suppress 6506 return secureEnvironment.ClientSession.TryBindUseLicenseToAllIdentites(_serializedUseLicense); } ////// ApplicationData data dictionary contains values that are passed from publishing /// application to a consuming application. One data pair that is processed by a Rights /// Management Services (RMS) server is the string pair "Allow_Server_Editing"/"True". /// When an issuance license has this value pair, it will allow the service, or any trusted /// service, to reuse the content key. The pair "NOLICCACHE" / "1" is expected to control /// Use License embedding policy of the consuming applications. If it is set to 1, applications /// are expected not to embed the Use License into the document. /// public IDictionaryApplicationData { get { SecurityHelper.DemandRightsManagementPermission(); return _applicationSpecificDataDictionary; } } /// /// Test for equality. /// public override bool Equals(object x) { SecurityHelper.DemandRightsManagementPermission(); if (x == null) return false; // Standard behavior. if (x.GetType() != GetType()) return false; // Not the same type. // Note that because of the GetType() checking above, the casting must be valid. UseLicense obj = (UseLicense)x; return (String.CompareOrdinal(_serializedUseLicense, obj._serializedUseLicense) == 0); } ////// Compute hash code. /// public override int GetHashCode() { SecurityHelper.DemandRightsManagementPermission(); return _serializedUseLicense.GetHashCode(); } private string _serializedUseLicense; private Guid _contentId; private ContentUser _owner = null; private IDictionary_applicationSpecificDataDictionary = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
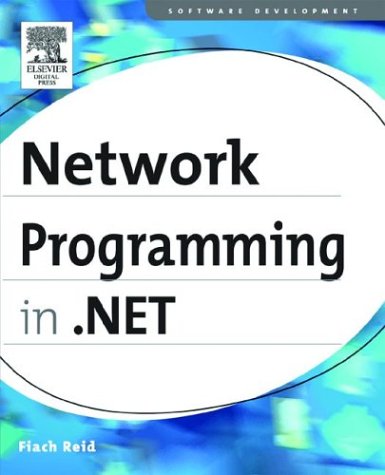
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlError.cs
- ParameterCollection.cs
- SoapHeader.cs
- VSDExceptions.cs
- WaitHandle.cs
- WindowsTreeView.cs
- NavigateUrlConverter.cs
- RequestCachingSection.cs
- ViewGenerator.cs
- EventListener.cs
- WindowsAuthenticationEventArgs.cs
- DeviceFiltersSection.cs
- Vector3DIndependentAnimationStorage.cs
- AssemblyAttributes.cs
- MultiSelector.cs
- StructuralType.cs
- ListSourceHelper.cs
- ReadOnlyPermissionSet.cs
- SecureStringHasher.cs
- DrawingVisual.cs
- XPathSingletonIterator.cs
- WmlSelectionListAdapter.cs
- DataBindingList.cs
- XmlSecureResolver.cs
- ConfigXmlElement.cs
- Codec.cs
- GlobalizationSection.cs
- VisualStyleRenderer.cs
- CommandEventArgs.cs
- RegexCompiler.cs
- PropertyConverter.cs
- CreatingCookieEventArgs.cs
- SystemIPGlobalProperties.cs
- LicenseContext.cs
- MissingSatelliteAssemblyException.cs
- Activity.cs
- UnsafeNetInfoNativeMethods.cs
- AnimationClockResource.cs
- XamlSerializationHelper.cs
- Logging.cs
- XmlEventCache.cs
- TextBlock.cs
- LinqDataSourceStatusEventArgs.cs
- ControlValuePropertyAttribute.cs
- ParagraphResult.cs
- ZipPackagePart.cs
- SourceFileInfo.cs
- TypeContext.cs
- EncryptedHeaderXml.cs
- ConfigXmlReader.cs
- Keyboard.cs
- XmlParserContext.cs
- SizeChangedInfo.cs
- cookie.cs
- WebPartsPersonalizationAuthorization.cs
- PerformanceCounterPermissionAttribute.cs
- GraphicsContainer.cs
- Processor.cs
- PermissionAttributes.cs
- PolyQuadraticBezierSegment.cs
- ToolZone.cs
- XPathBinder.cs
- ColumnHeader.cs
- Automation.cs
- UnsafeNativeMethods.cs
- WmfPlaceableFileHeader.cs
- DataSourceExpressionCollection.cs
- CodeTypeMember.cs
- SessionState.cs
- QilLiteral.cs
- ListManagerBindingsCollection.cs
- DataGridViewElement.cs
- IPCCacheManager.cs
- KeyGesture.cs
- AppDomainCompilerProxy.cs
- CodeArrayIndexerExpression.cs
- AssemblyInfo.cs
- MaterialGroup.cs
- DigitShape.cs
- ObservableCollection.cs
- IPEndPointCollection.cs
- EraserBehavior.cs
- Serializer.cs
- GridViewCellAutomationPeer.cs
- RedirectionProxy.cs
- DataPagerFieldCommandEventArgs.cs
- ByteAnimationBase.cs
- AddInDeploymentState.cs
- SettingsBindableAttribute.cs
- Configuration.cs
- DependencySource.cs
- ToolStripLabel.cs
- Flowchart.cs
- OutOfMemoryException.cs
- CopyAction.cs
- TokenBasedSetEnumerator.cs
- DbConnectionFactory.cs
- WindowsComboBox.cs
- ThreadTrace.cs
- InputDevice.cs