Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / Expressions / DataSourceExpressionCollection.cs / 1305376 / DataSourceExpressionCollection.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { using System; #else namespace System.Web.UI.WebControls.Expressions { #endif using System.Collections; using System.Collections.ObjectModel; using System.Diagnostics.CodeAnalysis; using System.Web; using System.Web.UI; public class DataSourceExpressionCollection : StateManagedCollection { private IQueryableDataSource _dataSource; private static readonly Type[] knownTypes = new Type[] { typeof(SearchExpression), typeof(MethodExpression), typeof(OrderByExpression), typeof(RangeExpression), typeof(PropertyExpression), typeof(CustomExpression), }; public HttpContext Context { get; private set; } public Control Owner { get; private set; } public DataSourceExpression this[int index] { get { return (DataSourceExpression)((IList)this)[index]; } set { ((IList)this)[index] = value; } } // Allows for nested expression blocks to be initilaized after the fact internal void SetContext(Control owner, HttpContext context, IQueryableDataSource dataSource) { Owner = owner; Context = context; _dataSource = dataSource; foreach (DataSourceExpression expression in this) { expression.SetContext(owner, context, _dataSource); } } public void Add(DataSourceExpression expression) { ((IList)this).Add(expression); } protected override object CreateKnownType(int index) { switch (index) { case 0: return new SearchExpression(); case 1: return new MethodExpression(); case 2: return new OrderByExpression(); case 3: return new RangeExpression(); case 4: return new PropertyExpression(); case 5: return new CustomExpression(); default: throw new ArgumentOutOfRangeException("index"); } } public void CopyTo(DataSourceExpression[] expressionArray, int index) { base.CopyTo(expressionArray, index); } public void Contains(DataSourceExpression expression) { ((IList)this).Contains(expression); } protected override Type[] GetKnownTypes() { return knownTypes; } public int IndexOf(DataSourceExpression expression) { return ((IList)this).IndexOf(expression); } public void Insert(int index, DataSourceExpression expression) { ((IList)this).Insert(index, expression); } public void Remove(DataSourceExpression expression) { ((IList)this).Remove(expression); } public void RemoveAt(int index) { ((IList)this).RemoveAt(index); } protected override void SetDirtyObject(object o) { ((DataSourceExpression)o).SetDirty(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
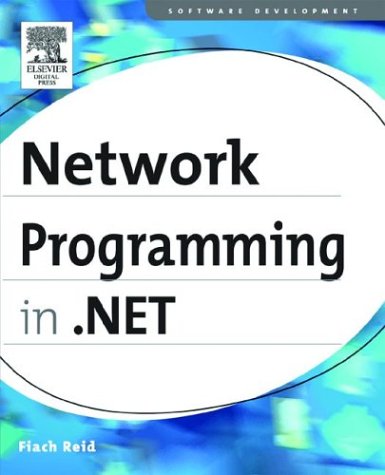
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLChars.cs
- WebPartConnectionsCloseVerb.cs
- DataServiceRequestOfT.cs
- Exceptions.cs
- CodeGroup.cs
- BaseParser.cs
- CacheAxisQuery.cs
- DocumentsTrace.cs
- SqlAggregateChecker.cs
- HttpPostedFileBase.cs
- ObjectConverter.cs
- SqlUserDefinedTypeAttribute.cs
- ContainerParaClient.cs
- EditableTreeList.cs
- XPathParser.cs
- IgnoreSection.cs
- TranslateTransform.cs
- CriticalExceptions.cs
- InputProcessorProfiles.cs
- HttpModuleActionCollection.cs
- ExtendedProperty.cs
- Permission.cs
- StyleXamlParser.cs
- RootBuilder.cs
- TabletDeviceInfo.cs
- IgnorePropertiesAttribute.cs
- WebResourceUtil.cs
- ParseChildrenAsPropertiesAttribute.cs
- Content.cs
- Select.cs
- Random.cs
- UserInitiatedNavigationPermission.cs
- DebugHandleTracker.cs
- TrackingParticipant.cs
- KeyBinding.cs
- HttpException.cs
- MessageRpc.cs
- RunClient.cs
- ReceiveSecurityHeaderEntry.cs
- XPathPatternParser.cs
- AttributeQuery.cs
- ContainerControlDesigner.cs
- CannotUnloadAppDomainException.cs
- FileStream.cs
- Point3DAnimationBase.cs
- BufferedGraphics.cs
- PassportIdentity.cs
- EpmHelper.cs
- NGCPageContentSerializerAsync.cs
- Adorner.cs
- selecteditemcollection.cs
- MarkupExtensionReturnTypeAttribute.cs
- RowVisual.cs
- SignedXml.cs
- FormViewRow.cs
- CustomPopupPlacement.cs
- ByteStack.cs
- DataTablePropertyDescriptor.cs
- HttpAsyncResult.cs
- parserscommon.cs
- DataGridViewHeaderCell.cs
- ObjectDataSourceEventArgs.cs
- _NestedSingleAsyncResult.cs
- AccessibleObject.cs
- Composition.cs
- TreeNode.cs
- RowToParametersTransformer.cs
- PostBackOptions.cs
- DynamicILGenerator.cs
- _ProxyRegBlob.cs
- ButtonChrome.cs
- DataGridViewColumnConverter.cs
- FrameworkElement.cs
- QilDataSource.cs
- Style.cs
- SimpleRecyclingCache.cs
- MultiPartWriter.cs
- newinstructionaction.cs
- DBParameter.cs
- XNameTypeConverter.cs
- ServiceProviders.cs
- HGlobalSafeHandle.cs
- InternalConfigSettingsFactory.cs
- SettingsPropertyValue.cs
- QuaternionRotation3D.cs
- NetworkInformationException.cs
- DateTimeUtil.cs
- ChannelCredentials.cs
- CultureInfo.cs
- SchemaImporter.cs
- WindowsFormsLinkLabel.cs
- PersianCalendar.cs
- ItemContainerGenerator.cs
- LassoSelectionBehavior.cs
- CapabilitiesRule.cs
- PageParserFilter.cs
- SoapServerMessage.cs
- EmptyEnumerator.cs
- SynchronizationContext.cs
- Wildcard.cs