Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / InputProviderSite.cs / 1 / InputProviderSite.cs
using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using MS.Win32; using System.Windows.Threading; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// The object which input providers use to report input to the input /// manager. /// internal class InputProviderSite : IDisposable { ////// Critical: This code creates critical data in the form of InputManager and InputProvider /// [SecurityCritical] internal InputProviderSite(InputManager inputManager, IInputProvider inputProvider) { _inputManager = new SecurityCriticalDataClass(inputManager); _inputProvider = new SecurityCriticalDataClass (inputProvider); } /// /// Returns the input manager that this site is attached to. /// ////// Critical: We do not want to expose the Input manager in the SEE /// TreatAsSafe: This code has a demand in it /// public InputManager InputManager { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnrestrictedUIPermission(); return CriticalInputManager; } } ////// Returns the input manager that this site is attached to. /// ////// Critical: We do not want to expose the Input manager in the SEE /// internal InputManager CriticalInputManager { [SecurityCritical] get { return _inputManager.Value; } } ////// Unregisters this input provider. /// ////// Critical: This code accesses critical data (InputManager and InputProvider). /// TreatAsSafe: The critical data is not exposed outside this call /// [SecurityCritical,SecurityTreatAsSafe] public void Dispose() { if (!_isDisposed) { _isDisposed = true; if (_inputManager != null && _inputProvider != null) { _inputManager.Value.UnregisterInputProvider(_inputProvider.Value); } _inputManager = null; _inputProvider = null; } } ////// Returns true if the CompositionTarget is disposed. /// public bool IsDisposed { get { return _isDisposed; } } ////// Reports input to the input manager. /// ////// Whether or not any event generated as a consequence of this /// event was handled. /// ////// Critical:This code is critical and can be used in event spoofing. It also accesses /// InputManager and calls into ProcessInput which is critical. /// // [SecurityCritical ] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted = true)] public bool ReportInput(InputReport inputReport) { if(IsDisposed) { throw new ObjectDisposedException(SR.Get(SRID.InputProviderSiteDisposed)); } bool handled = false; InputReportEventArgs input = new InputReportEventArgs(null, inputReport); input.RoutedEvent=InputManager.PreviewInputReportEvent; if(_inputManager != null) { handled = _inputManager.Value.ProcessInput(input); } return handled; } private bool _isDisposed; ////// Critical: This object should not be exposed in the SEE as it can be /// used for input spoofing /// private SecurityCriticalDataClass_inputManager; /// /// Critical: This object should not be exposed in the SEE as it can be /// used for input spoofing /// private SecurityCriticalDataClass_inputProvider; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; // SecurityHelper using MS.Win32; using System.Windows.Threading; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { /// /// The object which input providers use to report input to the input /// manager. /// internal class InputProviderSite : IDisposable { ////// Critical: This code creates critical data in the form of InputManager and InputProvider /// [SecurityCritical] internal InputProviderSite(InputManager inputManager, IInputProvider inputProvider) { _inputManager = new SecurityCriticalDataClass(inputManager); _inputProvider = new SecurityCriticalDataClass (inputProvider); } /// /// Returns the input manager that this site is attached to. /// ////// Critical: We do not want to expose the Input manager in the SEE /// TreatAsSafe: This code has a demand in it /// public InputManager InputManager { [SecurityCritical,SecurityTreatAsSafe] get { SecurityHelper.DemandUnrestrictedUIPermission(); return CriticalInputManager; } } ////// Returns the input manager that this site is attached to. /// ////// Critical: We do not want to expose the Input manager in the SEE /// internal InputManager CriticalInputManager { [SecurityCritical] get { return _inputManager.Value; } } ////// Unregisters this input provider. /// ////// Critical: This code accesses critical data (InputManager and InputProvider). /// TreatAsSafe: The critical data is not exposed outside this call /// [SecurityCritical,SecurityTreatAsSafe] public void Dispose() { if (!_isDisposed) { _isDisposed = true; if (_inputManager != null && _inputProvider != null) { _inputManager.Value.UnregisterInputProvider(_inputProvider.Value); } _inputManager = null; _inputProvider = null; } } ////// Returns true if the CompositionTarget is disposed. /// public bool IsDisposed { get { return _isDisposed; } } ////// Reports input to the input manager. /// ////// Whether or not any event generated as a consequence of this /// event was handled. /// ////// Critical:This code is critical and can be used in event spoofing. It also accesses /// InputManager and calls into ProcessInput which is critical. /// // [SecurityCritical ] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted = true)] public bool ReportInput(InputReport inputReport) { if(IsDisposed) { throw new ObjectDisposedException(SR.Get(SRID.InputProviderSiteDisposed)); } bool handled = false; InputReportEventArgs input = new InputReportEventArgs(null, inputReport); input.RoutedEvent=InputManager.PreviewInputReportEvent; if(_inputManager != null) { handled = _inputManager.Value.ProcessInput(input); } return handled; } private bool _isDisposed; ////// Critical: This object should not be exposed in the SEE as it can be /// used for input spoofing /// private SecurityCriticalDataClass_inputManager; /// /// Critical: This object should not be exposed in the SEE as it can be /// used for input spoofing /// private SecurityCriticalDataClass_inputProvider; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
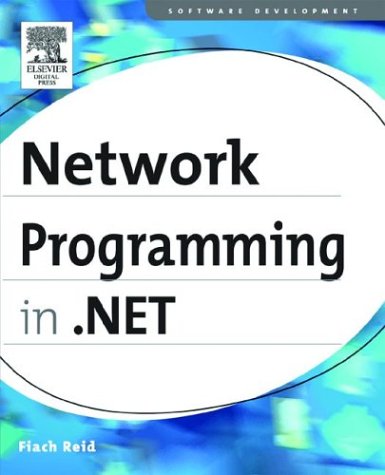
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DBCommandBuilder.cs
- HtmlControl.cs
- ViewCellSlot.cs
- DatagridviewDisplayedBandsData.cs
- ScrollBar.cs
- CapabilitiesAssignment.cs
- TypeLibConverter.cs
- CompilerCollection.cs
- Models.cs
- DocumentSequenceHighlightLayer.cs
- UnsafeNativeMethods.cs
- ObjectSecurity.cs
- StringUtil.cs
- EntityDataSourceValidationException.cs
- AppLevelCompilationSectionCache.cs
- IDQuery.cs
- IPPacketInformation.cs
- SettingsAttributes.cs
- IntegrationExceptionEventArgs.cs
- PolicyUnit.cs
- DnsPermission.cs
- MarkupExtensionReturnTypeAttribute.cs
- TakeQueryOptionExpression.cs
- SubpageParagraph.cs
- RemoteHelper.cs
- StylusOverProperty.cs
- Int16AnimationUsingKeyFrames.cs
- AmbientValueAttribute.cs
- VBCodeProvider.cs
- StylusPointPropertyId.cs
- InstanceBehavior.cs
- ObjectSet.cs
- ProbeMatchesApril2005.cs
- PageClientProxyGenerator.cs
- WebSysDisplayNameAttribute.cs
- SessionParameter.cs
- FileSystemWatcher.cs
- TextLineBreak.cs
- SiteMapNodeCollection.cs
- UncommonField.cs
- WebPartCatalogAddVerb.cs
- XmlBinaryReaderSession.cs
- MetadataException.cs
- CacheChildrenQuery.cs
- ExtensionMethods.cs
- TemplatedEditableDesignerRegion.cs
- PageCodeDomTreeGenerator.cs
- StringFormat.cs
- CustomWebEventKey.cs
- ApplyHostConfigurationBehavior.cs
- MaskDescriptor.cs
- SrgsElementFactory.cs
- Vector3DAnimationBase.cs
- NamedPipeHostedTransportConfiguration.cs
- SqlReferenceCollection.cs
- Site.cs
- XmlAnyElementAttribute.cs
- HMACMD5.cs
- ComplexPropertyEntry.cs
- SudsWriter.cs
- AnimatedTypeHelpers.cs
- CodeSubDirectory.cs
- DataAccessor.cs
- ShaderEffect.cs
- Context.cs
- OracleBinary.cs
- BindingContext.cs
- PassportPrincipal.cs
- DataGridTextBox.cs
- Visitors.cs
- ModelTreeManager.cs
- AstTree.cs
- GenericAuthenticationEventArgs.cs
- UdpReplyToBehavior.cs
- KnownColorTable.cs
- QueryResult.cs
- ItemsControlAutomationPeer.cs
- MachineKeyConverter.cs
- ObjectAssociationEndMapping.cs
- EventMappingSettingsCollection.cs
- ErrorStyle.cs
- SqlDependencyListener.cs
- Memoizer.cs
- DrawingContext.cs
- _HeaderInfoTable.cs
- SqlInternalConnection.cs
- BaseHashHelper.cs
- InputReport.cs
- DataGridViewButtonColumn.cs
- FontWeight.cs
- CodeTypeMemberCollection.cs
- wgx_exports.cs
- LineServices.cs
- Int16Storage.cs
- LayoutEvent.cs
- bindurihelper.cs
- Help.cs
- RangeBase.cs
- KeyInstance.cs
- GraphicsContainer.cs