Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / Stylus / StylusPlugin.cs / 1 / StylusPlugin.cs
// #define TRACE using System; using System.Diagnostics; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Windows.Threading; using System.Windows; using System.Windows.Input; using System.Windows.Media; using MS.Win32; // for *NativeMethods namespace System.Windows.Input.StylusPlugIns { ///////////////////////////////////////////////////////////////////// ////// [TBS] /// public abstract class StylusPlugIn { ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected StylusPlugIn() { } ///////////////////////////////////////////////////////////////////// // (in Dispatcher) internal void Added(StylusPlugInCollection plugInCollection) { _pic = plugInCollection; OnAdded(); InvalidateIsActiveForInput(); // Make sure we fire OnIsActivateForInputChanged. } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnAdded() { } ///////////////////////////////////////////////////////////////////// // (in Dispatcher) internal void Removed() { // Make sure we fire OnIsActivateForInputChanged if we need to. if (_activeForInput) { InvalidateIsActiveForInput(); } OnRemoved(); _pic = null; } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnRemoved() { } ///////////////////////////////////////////////////////////////////// // (in RTI Dispatcher) internal void StylusEnterLeave(bool isEnter, RawStylusInput rawStylusInput, bool confirmed) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { if (isEnter) OnStylusEnter(rawStylusInput, confirmed); else OnStylusLeave(rawStylusInput, confirmed); } } ///////////////////////////////////////////////////////////////////// // (in RTI Dispatcher) internal void RawStylusInput(RawStylusInput rawStylusInput) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { switch( rawStylusInput.Report.Actions ) { case RawStylusActions.Down: OnStylusDown(rawStylusInput); break; case RawStylusActions.Move: OnStylusMove(rawStylusInput); break; case RawStylusActions.Up: OnStylusUp(rawStylusInput); break; } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusEnter(RawStylusInput rawStylusInput, bool confirmed) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusLeave(RawStylusInput rawStylusInput, bool confirmed) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusDown(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusMove(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusUp(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// // (on app Dispatcher) internal void FireCustomData(object callbackData, RawStylusActions action, bool targetVerified) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { switch (action) { case RawStylusActions.Down: OnStylusDownProcessed(callbackData, targetVerified); break; case RawStylusActions.Move: OnStylusMoveProcessed(callbackData, targetVerified); break; case RawStylusActions.Up: OnStylusUpProcessed(callbackData, targetVerified); break; } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusDownProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusMoveProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusUpProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - both Dispatchers /// public UIElement Element { get { return (_pic != null) ? _pic.Element : null; } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - both Dispatchers /// public Rect ElementBounds { get { return (_pic != null) ? _pic.Rect : new Rect(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - get - both Dispatchers, set Dispatcher /// public bool Enabled { get // both Dispatchers { return __enabled; } set // on Dispatcher { // Verify we are on the proper thread. if (_pic != null) { _pic.Element.VerifyAccess(); } if (value != __enabled) { // If we are currently active for input we need to lock before input before // changing so we don't get input coming in before event is fired. if (_pic != null && _pic.IsActiveForInput) { // Make sure lock() doesn't cause reentrancy. using(_pic.Element.Dispatcher.DisableProcessing()) { lock(_pic.PenContextsSyncRoot) { // Make sure we fire the OnEnabledChanged event in the proper order // depending on whether we are going active or inactive so you don't // get input events after going inactive or before going active. __enabled = value; if (value == false) { // Make sure we fire OnIsActivateForInputChanged if we need to. InvalidateIsActiveForInput(); OnEnabledChanged(); } else { OnEnabledChanged(); InvalidateIsActiveForInput(); } } } } else { __enabled = value; if (value == false) { // Make sure we fire OnIsActivateForInputChanged if we need to. InvalidateIsActiveForInput(); OnEnabledChanged(); } else { OnEnabledChanged(); InvalidateIsActiveForInput(); } } } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnEnabledChanged() { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - app Dispatcher /// internal void InvalidateIsActiveForInput() { bool newIsActive = (_pic != null) ? (Enabled && _pic.Contains(this) && _pic.IsActiveForInput) : false; if (newIsActive != _activeForInput) { _activeForInput = newIsActive; OnIsActiveForInputChanged(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - get - both Dispatchers /// public bool IsActiveForInput { get // both Dispatchers { return _activeForInput; } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnIsActiveForInputChanged() { } // Enabled state is local to this plugin so we just use volatile versus creating a lock // around it since we just read it from multiple thread and write from one. volatile bool __enabled = true; bool _activeForInput = false; StylusPlugInCollection _pic = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // #define TRACE using System; using System.Diagnostics; using System.Collections; using System.Collections.Specialized; using System.Security.Permissions; using System.Windows.Threading; using System.Windows; using System.Windows.Input; using System.Windows.Media; using MS.Win32; // for *NativeMethods namespace System.Windows.Input.StylusPlugIns { ///////////////////////////////////////////////////////////////////// ////// [TBS] /// public abstract class StylusPlugIn { ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected StylusPlugIn() { } ///////////////////////////////////////////////////////////////////// // (in Dispatcher) internal void Added(StylusPlugInCollection plugInCollection) { _pic = plugInCollection; OnAdded(); InvalidateIsActiveForInput(); // Make sure we fire OnIsActivateForInputChanged. } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnAdded() { } ///////////////////////////////////////////////////////////////////// // (in Dispatcher) internal void Removed() { // Make sure we fire OnIsActivateForInputChanged if we need to. if (_activeForInput) { InvalidateIsActiveForInput(); } OnRemoved(); _pic = null; } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnRemoved() { } ///////////////////////////////////////////////////////////////////// // (in RTI Dispatcher) internal void StylusEnterLeave(bool isEnter, RawStylusInput rawStylusInput, bool confirmed) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { if (isEnter) OnStylusEnter(rawStylusInput, confirmed); else OnStylusLeave(rawStylusInput, confirmed); } } ///////////////////////////////////////////////////////////////////// // (in RTI Dispatcher) internal void RawStylusInput(RawStylusInput rawStylusInput) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { switch( rawStylusInput.Report.Actions ) { case RawStylusActions.Down: OnStylusDown(rawStylusInput); break; case RawStylusActions.Move: OnStylusMove(rawStylusInput); break; case RawStylusActions.Up: OnStylusUp(rawStylusInput); break; } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusEnter(RawStylusInput rawStylusInput, bool confirmed) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusLeave(RawStylusInput rawStylusInput, bool confirmed) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusDown(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusMove(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on RTI Dispatcher /// protected virtual void OnStylusUp(RawStylusInput rawStylusInput) { } ///////////////////////////////////////////////////////////////////// // (on app Dispatcher) internal void FireCustomData(object callbackData, RawStylusActions action, bool targetVerified) { // Only fire if plugin is enabled and hooked up to plugincollection. if (__enabled && _pic != null) { switch (action) { case RawStylusActions.Down: OnStylusDownProcessed(callbackData, targetVerified); break; case RawStylusActions.Move: OnStylusMoveProcessed(callbackData, targetVerified); break; case RawStylusActions.Up: OnStylusUpProcessed(callbackData, targetVerified); break; } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusDownProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusMoveProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnStylusUpProcessed(object callbackData, bool targetVerified) { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - both Dispatchers /// public UIElement Element { get { return (_pic != null) ? _pic.Element : null; } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - both Dispatchers /// public Rect ElementBounds { get { return (_pic != null) ? _pic.Rect : new Rect(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - get - both Dispatchers, set Dispatcher /// public bool Enabled { get // both Dispatchers { return __enabled; } set // on Dispatcher { // Verify we are on the proper thread. if (_pic != null) { _pic.Element.VerifyAccess(); } if (value != __enabled) { // If we are currently active for input we need to lock before input before // changing so we don't get input coming in before event is fired. if (_pic != null && _pic.IsActiveForInput) { // Make sure lock() doesn't cause reentrancy. using(_pic.Element.Dispatcher.DisableProcessing()) { lock(_pic.PenContextsSyncRoot) { // Make sure we fire the OnEnabledChanged event in the proper order // depending on whether we are going active or inactive so you don't // get input events after going inactive or before going active. __enabled = value; if (value == false) { // Make sure we fire OnIsActivateForInputChanged if we need to. InvalidateIsActiveForInput(); OnEnabledChanged(); } else { OnEnabledChanged(); InvalidateIsActiveForInput(); } } } } else { __enabled = value; if (value == false) { // Make sure we fire OnIsActivateForInputChanged if we need to. InvalidateIsActiveForInput(); OnEnabledChanged(); } else { OnEnabledChanged(); InvalidateIsActiveForInput(); } } } } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on app Dispatcher /// protected virtual void OnEnabledChanged() { } ///////////////////////////////////////////////////////////////////// ////// [TBS] - app Dispatcher /// internal void InvalidateIsActiveForInput() { bool newIsActive = (_pic != null) ? (Enabled && _pic.Contains(this) && _pic.IsActiveForInput) : false; if (newIsActive != _activeForInput) { _activeForInput = newIsActive; OnIsActiveForInputChanged(); } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - get - both Dispatchers /// public bool IsActiveForInput { get // both Dispatchers { return _activeForInput; } } ///////////////////////////////////////////////////////////////////// ////// [TBS] - on Dispatcher /// protected virtual void OnIsActiveForInputChanged() { } // Enabled state is local to this plugin so we just use volatile versus creating a lock // around it since we just read it from multiple thread and write from one. volatile bool __enabled = true; bool _activeForInput = false; StylusPlugInCollection _pic = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
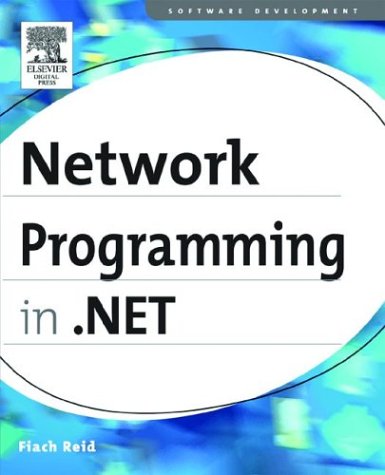
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuestionEventArgs.cs
- LinkArea.cs
- MonikerSyntaxException.cs
- Sorting.cs
- RemotingSurrogateSelector.cs
- ProxyAttribute.cs
- EdmScalarPropertyAttribute.cs
- SafeHandle.cs
- SmiRequestExecutor.cs
- WebServiceBindingAttribute.cs
- IsolatedStorageFileStream.cs
- WindowsTab.cs
- RuleSetDialog.cs
- DataRow.cs
- HtmlControlAdapter.cs
- XhtmlBasicSelectionListAdapter.cs
- BigInt.cs
- PersonalizationProviderCollection.cs
- HyperLinkStyle.cs
- ClientRolePrincipal.cs
- PropertyNames.cs
- Base64Stream.cs
- ResourceSet.cs
- ScriptReference.cs
- WebBrowserEvent.cs
- MsmqIntegrationBindingElement.cs
- XpsInterleavingPolicy.cs
- ShadowGlyph.cs
- BigInt.cs
- FontStretch.cs
- View.cs
- XmlParserContext.cs
- EntityProxyFactory.cs
- TaskHelper.cs
- ChooseAction.cs
- ConnectionInterfaceCollection.cs
- XmlProcessingInstruction.cs
- ServiceHostingEnvironment.cs
- TreeIterators.cs
- FillErrorEventArgs.cs
- GlobalizationSection.cs
- WindowShowOrOpenTracker.cs
- EmissiveMaterial.cs
- KeyManager.cs
- CreateParams.cs
- Decoder.cs
- DataObjectAttribute.cs
- WorkflowServiceBehavior.cs
- AsnEncodedData.cs
- IFlowDocumentViewer.cs
- SocketAddress.cs
- SpnEndpointIdentity.cs
- Journaling.cs
- DataGridViewComboBoxCell.cs
- ConfigurationStrings.cs
- PointAnimationClockResource.cs
- EarlyBoundInfo.cs
- LayoutEngine.cs
- GenericNameHandler.cs
- UserMapPath.cs
- StateManagedCollection.cs
- MimeMapping.cs
- WebColorConverter.cs
- Misc.cs
- MatrixTransform.cs
- CommandSet.cs
- ModuleElement.cs
- JoinSymbol.cs
- NumericUpDown.cs
- WorkflowQueueInfo.cs
- ToolStripStatusLabel.cs
- EncoderFallback.cs
- DataGridViewTopLeftHeaderCell.cs
- InstanceHandleConflictException.cs
- KnownAssembliesSet.cs
- DbModificationClause.cs
- TypeEnumerableViewSchema.cs
- ExpressionLexer.cs
- CodeArrayCreateExpression.cs
- _KerberosClient.cs
- BaseCodePageEncoding.cs
- SortableBindingList.cs
- PersonalizablePropertyEntry.cs
- HtmlTableCell.cs
- TextAnchor.cs
- _CookieModule.cs
- PreparingEnlistment.cs
- Tag.cs
- MessageBox.cs
- EntityStoreSchemaFilterEntry.cs
- XmlQualifiedNameTest.cs
- IndexedDataBuffer.cs
- Signature.cs
- ToolBarOverflowPanel.cs
- Repeater.cs
- DesignColumnCollection.cs
- ResourceManager.cs
- Timer.cs
- SecurityTokenParameters.cs
- DataKeyArray.cs