Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Effects / BitmapEffectRenderDataResource.cs / 1 / BitmapEffectRenderDataResource.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: BitmapEffectRenderDataResource.cs // // Description: This file contains the implementation of BitmapEffectRenderDataResource. // // History: // 10/28/2005 :[....] - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Media.Effects; using System.Security; using System.Security.Permissions; namespace System.Windows.Media.Effects { ////// This class is used to realize the content of the /// renderdata if a bitmap effect is applied to it /// internal class BitmapEffectRenderDataContent : IRealizationContextClient { RenderData _renderData; DUCE.Channel _channel; public BitmapEffectRenderDataContent(RenderData renderData, RealizationContext ctx) { _channel = ctx.Channel; _renderData = renderData; } public void ExecuteRealizationsUpdate() { _renderData.ExecuteBitmapEffectRealizationUpdates(_channel); } } ////// This class contains information used by RenderData /// when an effect is applied to it /// internal class BitmapEffectRenderDataResource { ////// Keeps track of the stack depth of the current effect /// public int BitmapEffectStackDepth { get { return _effectStackDepth; } set { _effectStackDepth = value; } } ////// Keeps track of the number of top level effects /// public int TopLevelEffects { get { return _topLevelEffects; } set { _topLevelEffects = value; } } ////// Return the modified renderdata size by the effects /// internal int DataSize { get { return _dataSize; } set { _dataSize = value; } } internal bool HasAnyEffects { get { return _topLevelEffects > 0; } } internal BitmapEffectDrawing BitmapEffectDrawing { get { return _bitmapEffectDrawing; } } ////// Update the data size. /// 1. If we have an effect on the stack skip the command, otherwise /// 2. If the command is PushEffect, add DrawDrawing command instead /// 3. For all other commands, add the totalSize /// /// /// internal unsafe void UpdateDataSize(MILCMD id, int totalSize) { if (id == MILCMD.MilPushEffect) { // for each push effect, we do instead // DrawDrawing: RecordHeader + MILCMD_DRAW_DRAWING _dataSize += (sizeof(MILCMD_DRAW_DRAWING) + sizeof(RenderData.RecordHeader)); } else { _dataSize += totalSize; } } ////// Create placeholders for the BitmapEffectDrawing resource for the toplevel bitmap effects /// We need to do this because the resources are marshalled during the render pass /// but the bitmap generated for the effect happens during the realization pass /// /// internal void CreateBitmapEffectResources(DUCE.Channel channel) { for (int i = 0; i < _topLevelEffects; i++) { BitmapEffectDrawing bitmapEffectDrawing = new BitmapEffectDrawing(); // Addref the drawing ((DUCE.IResource)bitmapEffectDrawing).AddRefOnChannel(channel); // add the drawing to the list of resources _effectRealizations.Add(bitmapEffectDrawing); } } ////// Update the DrawingGroup resource for each bitmap effect /// internal void UpdateBitmapEffectResources(RenderData renderData, DUCE.Channel channel) { // if we don't have an effect, there is nothing to do if (_topLevelEffects <= 0) { return; } // clean up the previous realizations for (int i = 0; i < _effectRealizations.Count; i++) { BitmapEffectDrawing drawing = ((BitmapEffectDrawing)_effectRealizations[i]); drawing.Clear(channel); } BitmapEffectDrawing.UpdateTransformAndDrawingLists(false); TransformCollection worldTransforms = BitmapEffectDrawing.WorldTransforms; // Update the realizations foreach (Transform worldTransform in worldTransforms) { // render everything below the top level effects BitmapEffectDrawingContextWalker ctxWalker = new BitmapEffectDrawingContextWalker(worldTransform.Value, BitmapEffectDrawing.WindowClip); renderData.DrawingContextWalk(ctxWalker); BitmapEffectDrawing bitmapEffectDrawing = ctxWalker.BitmapEffectDrawing; TransformCollection vWorldTransforms = bitmapEffectDrawing.WorldTransforms; DrawingCollection vDrawings = bitmapEffectDrawing.Drawings; Debug.Assert(vDrawings.Count == vWorldTransforms.Count, "We should have the same number of images and transforms"); int count = vDrawings.Count; for (int i = 0; i < count; i++) { // if we rendered something update the resources if (vDrawings.Internal_GetItem(i) != null) { BitmapEffectDrawing drawing = ((BitmapEffectDrawing)_effectRealizations[i]); // if the drawing is already on channel, it will addref the items // when they are added to the collections. // otherwise, we need to addref them if (!drawing.IsOnChannel) { vWorldTransforms.Internal_GetItem(i).AddRefOnChannelCore(channel); vDrawings.Internal_GetItem(i).AddRefOnChannelCore(channel); } drawing.WorldTransforms.Add(vWorldTransforms.Internal_GetItem(i)); drawing.Drawings.Add(vDrawings.Internal_GetItem(i)); } } } for (int i = 0; i < _effectRealizations.Count; i++) { BitmapEffectDrawing drawing = ((BitmapEffectDrawing)_effectRealizations[i]); drawing.UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } BitmapEffectDrawing.ScheduleForUpdates = true; } ////// Marshal the DrawingGroup resource for each bitmap effect /// /// /// ////// This transparent method has unverifiable code. Added demand for UnmanagedCode on 64bit to mimic /// Security Transparency behavior on 64 bit. /// Workaround: VSWhidbey #577422 /// The #if WIN64 block below should be removed when the above issue is resolved on downlevel CLR bits. /// internal void MarshalBitmapEffectResources(DUCE.Channel channel, int topLevelEffectIndex) { Debug.Assert(topLevelEffectIndex >= 0, "We should not have a negative index"); // if we don't have an effect, there is nothing to do if (HasAnyEffects) { unsafe { #if WIN64 SecurityHelper.DemandUnmanagedCode(); #endif //Win64 // append drawing RenderData.RecordHeader recordImage = new RenderData.RecordHeader(); recordImage.Id = MILCMD.MilDrawDrawing; recordImage.Size = sizeof(MILCMD_DRAW_DRAWING) + sizeof(RenderData.RecordHeader); channel.AppendCommandData( (byte*)&recordImage, sizeof(RenderData.RecordHeader) ); MILCMD_DRAW_DRAWING dataImage = new MILCMD_DRAW_DRAWING( (uint)(((DUCE.IResource)_effectRealizations[topLevelEffectIndex]).GetHandle(channel))); channel.AppendCommandData( (byte*)&dataImage, sizeof(MILCMD_DRAW_DRAWING) ); } } } ////// Releases all the resources on the given channel /// /// internal void ReleaseBitmapEffectResources(DUCE.Channel channel) { for (int i = 0; i < _effectRealizations.Count; i++) { DUCE.IResource resource = (DUCE.IResource)_effectRealizations[i]; resource.ReleaseOnChannel(channel); } _effectRealizations.Clear(); BitmapEffectDrawing.WorldTransforms.Clear(); } #region Private Fields private int _dataSize; private int _topLevelEffects; ////// Record the stack depth at which the effect was applied /// This will let us know when it is time to remove the effect during the Pop /// instruction /// private int _effectStackDepth; private FrugalStructList_effectRealizations = new FrugalStructList (); private BitmapEffectDrawing _bitmapEffectDrawing = new BitmapEffectDrawing(); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // File: BitmapEffectRenderDataResource.cs // // Description: This file contains the implementation of BitmapEffectRenderDataResource. // // History: // 10/28/2005 :[....] - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Media.Effects; using System.Security; using System.Security.Permissions; namespace System.Windows.Media.Effects { /// /// This class is used to realize the content of the /// renderdata if a bitmap effect is applied to it /// internal class BitmapEffectRenderDataContent : IRealizationContextClient { RenderData _renderData; DUCE.Channel _channel; public BitmapEffectRenderDataContent(RenderData renderData, RealizationContext ctx) { _channel = ctx.Channel; _renderData = renderData; } public void ExecuteRealizationsUpdate() { _renderData.ExecuteBitmapEffectRealizationUpdates(_channel); } } ////// This class contains information used by RenderData /// when an effect is applied to it /// internal class BitmapEffectRenderDataResource { ////// Keeps track of the stack depth of the current effect /// public int BitmapEffectStackDepth { get { return _effectStackDepth; } set { _effectStackDepth = value; } } ////// Keeps track of the number of top level effects /// public int TopLevelEffects { get { return _topLevelEffects; } set { _topLevelEffects = value; } } ////// Return the modified renderdata size by the effects /// internal int DataSize { get { return _dataSize; } set { _dataSize = value; } } internal bool HasAnyEffects { get { return _topLevelEffects > 0; } } internal BitmapEffectDrawing BitmapEffectDrawing { get { return _bitmapEffectDrawing; } } ////// Update the data size. /// 1. If we have an effect on the stack skip the command, otherwise /// 2. If the command is PushEffect, add DrawDrawing command instead /// 3. For all other commands, add the totalSize /// /// /// internal unsafe void UpdateDataSize(MILCMD id, int totalSize) { if (id == MILCMD.MilPushEffect) { // for each push effect, we do instead // DrawDrawing: RecordHeader + MILCMD_DRAW_DRAWING _dataSize += (sizeof(MILCMD_DRAW_DRAWING) + sizeof(RenderData.RecordHeader)); } else { _dataSize += totalSize; } } ////// Create placeholders for the BitmapEffectDrawing resource for the toplevel bitmap effects /// We need to do this because the resources are marshalled during the render pass /// but the bitmap generated for the effect happens during the realization pass /// /// internal void CreateBitmapEffectResources(DUCE.Channel channel) { for (int i = 0; i < _topLevelEffects; i++) { BitmapEffectDrawing bitmapEffectDrawing = new BitmapEffectDrawing(); // Addref the drawing ((DUCE.IResource)bitmapEffectDrawing).AddRefOnChannel(channel); // add the drawing to the list of resources _effectRealizations.Add(bitmapEffectDrawing); } } ////// Update the DrawingGroup resource for each bitmap effect /// internal void UpdateBitmapEffectResources(RenderData renderData, DUCE.Channel channel) { // if we don't have an effect, there is nothing to do if (_topLevelEffects <= 0) { return; } // clean up the previous realizations for (int i = 0; i < _effectRealizations.Count; i++) { BitmapEffectDrawing drawing = ((BitmapEffectDrawing)_effectRealizations[i]); drawing.Clear(channel); } BitmapEffectDrawing.UpdateTransformAndDrawingLists(false); TransformCollection worldTransforms = BitmapEffectDrawing.WorldTransforms; // Update the realizations foreach (Transform worldTransform in worldTransforms) { // render everything below the top level effects BitmapEffectDrawingContextWalker ctxWalker = new BitmapEffectDrawingContextWalker(worldTransform.Value, BitmapEffectDrawing.WindowClip); renderData.DrawingContextWalk(ctxWalker); BitmapEffectDrawing bitmapEffectDrawing = ctxWalker.BitmapEffectDrawing; TransformCollection vWorldTransforms = bitmapEffectDrawing.WorldTransforms; DrawingCollection vDrawings = bitmapEffectDrawing.Drawings; Debug.Assert(vDrawings.Count == vWorldTransforms.Count, "We should have the same number of images and transforms"); int count = vDrawings.Count; for (int i = 0; i < count; i++) { // if we rendered something update the resources if (vDrawings.Internal_GetItem(i) != null) { BitmapEffectDrawing drawing = ((BitmapEffectDrawing)_effectRealizations[i]); // if the drawing is already on channel, it will addref the items // when they are added to the collections. // otherwise, we need to addref them if (!drawing.IsOnChannel) { vWorldTransforms.Internal_GetItem(i).AddRefOnChannelCore(channel); vDrawings.Internal_GetItem(i).AddRefOnChannelCore(channel); } drawing.WorldTransforms.Add(vWorldTransforms.Internal_GetItem(i)); drawing.Drawings.Add(vDrawings.Internal_GetItem(i)); } } } for (int i = 0; i < _effectRealizations.Count; i++) { BitmapEffectDrawing drawing = ((BitmapEffectDrawing)_effectRealizations[i]); drawing.UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } BitmapEffectDrawing.ScheduleForUpdates = true; } ////// Marshal the DrawingGroup resource for each bitmap effect /// /// /// ////// This transparent method has unverifiable code. Added demand for UnmanagedCode on 64bit to mimic /// Security Transparency behavior on 64 bit. /// Workaround: VSWhidbey #577422 /// The #if WIN64 block below should be removed when the above issue is resolved on downlevel CLR bits. /// internal void MarshalBitmapEffectResources(DUCE.Channel channel, int topLevelEffectIndex) { Debug.Assert(topLevelEffectIndex >= 0, "We should not have a negative index"); // if we don't have an effect, there is nothing to do if (HasAnyEffects) { unsafe { #if WIN64 SecurityHelper.DemandUnmanagedCode(); #endif //Win64 // append drawing RenderData.RecordHeader recordImage = new RenderData.RecordHeader(); recordImage.Id = MILCMD.MilDrawDrawing; recordImage.Size = sizeof(MILCMD_DRAW_DRAWING) + sizeof(RenderData.RecordHeader); channel.AppendCommandData( (byte*)&recordImage, sizeof(RenderData.RecordHeader) ); MILCMD_DRAW_DRAWING dataImage = new MILCMD_DRAW_DRAWING( (uint)(((DUCE.IResource)_effectRealizations[topLevelEffectIndex]).GetHandle(channel))); channel.AppendCommandData( (byte*)&dataImage, sizeof(MILCMD_DRAW_DRAWING) ); } } } ////// Releases all the resources on the given channel /// /// internal void ReleaseBitmapEffectResources(DUCE.Channel channel) { for (int i = 0; i < _effectRealizations.Count; i++) { DUCE.IResource resource = (DUCE.IResource)_effectRealizations[i]; resource.ReleaseOnChannel(channel); } _effectRealizations.Clear(); BitmapEffectDrawing.WorldTransforms.Clear(); } #region Private Fields private int _dataSize; private int _topLevelEffects; ////// Record the stack depth at which the effect was applied /// This will let us know when it is time to remove the effect during the Pop /// instruction /// private int _effectStackDepth; private FrugalStructList_effectRealizations = new FrugalStructList (); private BitmapEffectDrawing _bitmapEffectDrawing = new BitmapEffectDrawing(); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
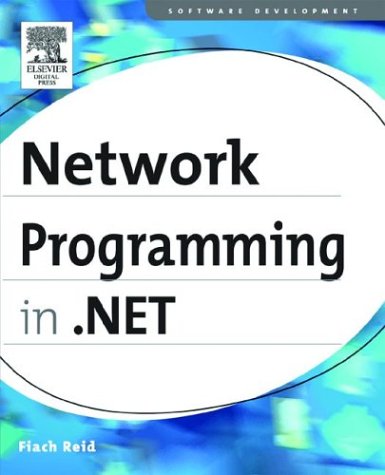
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlRoutingHandler.cs
- GroupAggregateExpr.cs
- TemplateInstanceAttribute.cs
- PageTheme.cs
- DataViewSettingCollection.cs
- CommandSet.cs
- RegexCode.cs
- SignedXmlDebugLog.cs
- ConfigurationErrorsException.cs
- HttpException.cs
- DateTimeConstantAttribute.cs
- AffineTransform3D.cs
- ConfigurationProperty.cs
- LocalizableResourceBuilder.cs
- Block.cs
- EditCommandColumn.cs
- LoginDesigner.cs
- XmlSignificantWhitespace.cs
- XmlFormatWriterGenerator.cs
- ModelItemImpl.cs
- XmlPropertyBag.cs
- Literal.cs
- FlagsAttribute.cs
- ErasingStroke.cs
- EventlogProvider.cs
- SqlNamer.cs
- PointLight.cs
- PropertyOverridesTypeEditor.cs
- StringAnimationBase.cs
- xml.cs
- MappingMetadataHelper.cs
- EventLogWatcher.cs
- Button.cs
- Point.cs
- DesignColumnCollection.cs
- PropertyCondition.cs
- tooltip.cs
- PackageRelationshipSelector.cs
- Utils.cs
- ZipIOCentralDirectoryFileHeader.cs
- ConfigurationPropertyCollection.cs
- XmlDesigner.cs
- VisualBasicHelper.cs
- MethodImplAttribute.cs
- SparseMemoryStream.cs
- BlobPersonalizationState.cs
- PingOptions.cs
- Vector3DValueSerializer.cs
- XmlBindingWorker.cs
- MetadataItemSerializer.cs
- CodeTypeReferenceSerializer.cs
- CollectionMarkupSerializer.cs
- WebContext.cs
- CompilationUtil.cs
- Range.cs
- BidirectionalDictionary.cs
- WriteLineDesigner.xaml.cs
- NestPullup.cs
- SamlAdvice.cs
- ThreadStateException.cs
- Lease.cs
- CollectionBase.cs
- SqlNotificationEventArgs.cs
- MouseEvent.cs
- PersonalizablePropertyEntry.cs
- Mappings.cs
- FieldToken.cs
- QuaternionRotation3D.cs
- XmlCodeExporter.cs
- PropertyToken.cs
- MgmtConfigurationRecord.cs
- StringValidatorAttribute.cs
- MultipleViewPattern.cs
- TextChange.cs
- ProfileManager.cs
- X509CertificateCollection.cs
- Scene3D.cs
- CancellationScope.cs
- ServiceBusyException.cs
- EntityCollection.cs
- PenThread.cs
- NullNotAllowedCollection.cs
- XmlException.cs
- WindowsListViewItem.cs
- _KerberosClient.cs
- PlainXmlWriter.cs
- ReturnType.cs
- TextPointer.cs
- SiteMapProvider.cs
- RecognizerInfo.cs
- ThousandthOfEmRealDoubles.cs
- ValidationResults.cs
- WindowPatternIdentifiers.cs
- EditingCoordinator.cs
- AsyncOperationManager.cs
- NamedPipeTransportSecurityElement.cs
- figurelength.cs
- MarshalByValueComponent.cs
- ToolStripMenuItem.cs
- EnumerableCollectionView.cs