Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / ObjectPersistData.cs / 1 / ObjectPersistData.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.Collections.Specialized; using System.Web.Util; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ObjectPersistData { private Type _objectType; private bool _isCollection; private ArrayList _collectionItems; private bool _localize; private string _resourceKey; private IDictionary _propertyTableByFilter; private IDictionary _propertyTableByProperty; private ArrayList _allPropertyEntries; private ArrayList _eventEntries; private IDictionary _builtObjects; public ObjectPersistData(ControlBuilder builder, IDictionary builtObjects) { _objectType = builder.ControlType; _localize = builder.Localize; _resourceKey = builder.GetResourceKey(); _builtObjects = builtObjects; if (typeof(ICollection).IsAssignableFrom(_objectType)) { _isCollection = true; } _collectionItems = new ArrayList(); _propertyTableByFilter = new HybridDictionary(true); _propertyTableByProperty = new HybridDictionary(true); _allPropertyEntries = new ArrayList(); _eventEntries = new ArrayList(); foreach (PropertyEntry entry in builder.SimplePropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.ComplexPropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.TemplatePropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.BoundPropertyEntries) { AddPropertyEntry(entry); } foreach (EventEntry entry in builder.EventEntries) { AddEventEntry(entry); } } ////// Get all property entries /// public ICollection AllPropertyEntries { get { return _allPropertyEntries; } } public IDictionary BuiltObjects { get { return _builtObjects; } } public ICollection CollectionItems { get { return _collectionItems; } } public ICollection EventEntries { get { return _eventEntries; } } ////// True if this persistence data is for a collection /// public bool IsCollection { get { return _isCollection; } } public bool Localize { get { return _localize; } } ////// The type of the object with these properties. /// public Type ObjectType { get { return _objectType; } } public string ResourceKey { get { return _resourceKey; } } ////// Adds a property to this persistence data, adding it to all necessary /// data structures. /// private void AddPropertyEntry(PropertyEntry entry) { if (_isCollection && (entry is ComplexPropertyEntry && ((ComplexPropertyEntry)entry).IsCollectionItem)) { _collectionItems.Add(entry); } else { IDictionary filteredProperties = (IDictionary)_propertyTableByFilter[entry.Filter]; if (filteredProperties == null) { filteredProperties = new HybridDictionary(true); _propertyTableByFilter[entry.Filter] = filteredProperties; } Debug.Assert((entry.Name != null) && (entry.Name.Length > 0)); filteredProperties[entry.Name] = entry; ArrayList properties = (ArrayList)_propertyTableByProperty[entry.Name]; if (properties == null) { properties = new ArrayList(); _propertyTableByProperty[entry.Name] = properties; } properties.Add(entry); } _allPropertyEntries.Add(entry); } private void AddEventEntry(EventEntry entry) { _eventEntries.Add(entry); } ////// public void AddToObjectControlBuilderTable(IDictionary table) { if (_builtObjects != null) { foreach (DictionaryEntry entry in _builtObjects) { table[entry.Key] = entry.Value; } } } /// /// Gets a PropertyEntry for the specified filter and property name /// public PropertyEntry GetFilteredProperty(string filter, string name) { IDictionary filteredProperties = GetFilteredProperties(filter); if (filteredProperties != null) { return (PropertyEntry)filteredProperties[name]; } return null; } ////// Gets all PropertyEntries for the specified filter /// public IDictionary GetFilteredProperties(string filter) { return (IDictionary)_propertyTableByFilter[filter]; } ////// Gets all filtered PropertiesEntries for a specified property (name uses dot-syntax e.g. Font.Bold) /// public ICollection GetPropertyAllFilters(string name) { ICollection properties = (ICollection)_propertyTableByProperty[name]; if (properties == null) { return new ArrayList(); } return properties; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.Collections.Specialized; using System.Web.Util; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ObjectPersistData { private Type _objectType; private bool _isCollection; private ArrayList _collectionItems; private bool _localize; private string _resourceKey; private IDictionary _propertyTableByFilter; private IDictionary _propertyTableByProperty; private ArrayList _allPropertyEntries; private ArrayList _eventEntries; private IDictionary _builtObjects; public ObjectPersistData(ControlBuilder builder, IDictionary builtObjects) { _objectType = builder.ControlType; _localize = builder.Localize; _resourceKey = builder.GetResourceKey(); _builtObjects = builtObjects; if (typeof(ICollection).IsAssignableFrom(_objectType)) { _isCollection = true; } _collectionItems = new ArrayList(); _propertyTableByFilter = new HybridDictionary(true); _propertyTableByProperty = new HybridDictionary(true); _allPropertyEntries = new ArrayList(); _eventEntries = new ArrayList(); foreach (PropertyEntry entry in builder.SimplePropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.ComplexPropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.TemplatePropertyEntries) { AddPropertyEntry(entry); } foreach (PropertyEntry entry in builder.BoundPropertyEntries) { AddPropertyEntry(entry); } foreach (EventEntry entry in builder.EventEntries) { AddEventEntry(entry); } } ////// Get all property entries /// public ICollection AllPropertyEntries { get { return _allPropertyEntries; } } public IDictionary BuiltObjects { get { return _builtObjects; } } public ICollection CollectionItems { get { return _collectionItems; } } public ICollection EventEntries { get { return _eventEntries; } } ////// True if this persistence data is for a collection /// public bool IsCollection { get { return _isCollection; } } public bool Localize { get { return _localize; } } ////// The type of the object with these properties. /// public Type ObjectType { get { return _objectType; } } public string ResourceKey { get { return _resourceKey; } } ////// Adds a property to this persistence data, adding it to all necessary /// data structures. /// private void AddPropertyEntry(PropertyEntry entry) { if (_isCollection && (entry is ComplexPropertyEntry && ((ComplexPropertyEntry)entry).IsCollectionItem)) { _collectionItems.Add(entry); } else { IDictionary filteredProperties = (IDictionary)_propertyTableByFilter[entry.Filter]; if (filteredProperties == null) { filteredProperties = new HybridDictionary(true); _propertyTableByFilter[entry.Filter] = filteredProperties; } Debug.Assert((entry.Name != null) && (entry.Name.Length > 0)); filteredProperties[entry.Name] = entry; ArrayList properties = (ArrayList)_propertyTableByProperty[entry.Name]; if (properties == null) { properties = new ArrayList(); _propertyTableByProperty[entry.Name] = properties; } properties.Add(entry); } _allPropertyEntries.Add(entry); } private void AddEventEntry(EventEntry entry) { _eventEntries.Add(entry); } ////// public void AddToObjectControlBuilderTable(IDictionary table) { if (_builtObjects != null) { foreach (DictionaryEntry entry in _builtObjects) { table[entry.Key] = entry.Value; } } } /// /// Gets a PropertyEntry for the specified filter and property name /// public PropertyEntry GetFilteredProperty(string filter, string name) { IDictionary filteredProperties = GetFilteredProperties(filter); if (filteredProperties != null) { return (PropertyEntry)filteredProperties[name]; } return null; } ////// Gets all PropertyEntries for the specified filter /// public IDictionary GetFilteredProperties(string filter) { return (IDictionary)_propertyTableByFilter[filter]; } ////// Gets all filtered PropertiesEntries for a specified property (name uses dot-syntax e.g. Font.Bold) /// public ICollection GetPropertyAllFilters(string name) { ICollection properties = (ICollection)_propertyTableByProperty[name]; if (properties == null) { return new ArrayList(); } return properties; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
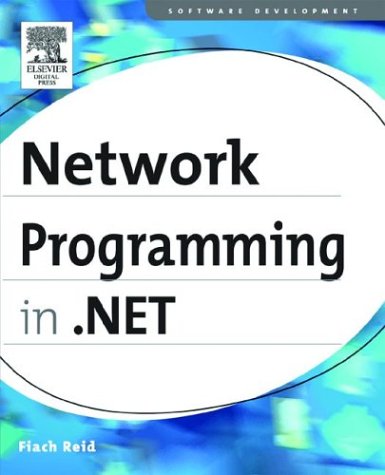
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _HelperAsyncResults.cs
- ComplexBindingPropertiesAttribute.cs
- NewArray.cs
- SqlWebEventProvider.cs
- FacetValueContainer.cs
- ApplicationServicesHostFactory.cs
- LostFocusEventManager.cs
- RoutedUICommand.cs
- Types.cs
- FragmentQueryKB.cs
- EditorPartDesigner.cs
- TextEditorSpelling.cs
- DataGridViewBindingCompleteEventArgs.cs
- FontStretches.cs
- WrappedReader.cs
- DataGridViewCellPaintingEventArgs.cs
- CacheRequest.cs
- SmtpSection.cs
- WebContext.cs
- configsystem.cs
- TrackingServices.cs
- SiteMap.cs
- TableLayoutCellPaintEventArgs.cs
- DbMetaDataFactory.cs
- TextServicesDisplayAttribute.cs
- DefaultBinder.cs
- PixelShader.cs
- DiscoveryClientDuplexChannel.cs
- DynamicPropertyHolder.cs
- ToolstripProfessionalRenderer.cs
- TransformCollection.cs
- ZipIOLocalFileDataDescriptor.cs
- StaticFileHandler.cs
- ListContractAdapter.cs
- MatrixAnimationBase.cs
- CounterCreationData.cs
- SelectedCellsCollection.cs
- ListViewAutomationPeer.cs
- NamedPipeConnectionPoolSettingsElement.cs
- MessageEncoderFactory.cs
- XmlValidatingReader.cs
- Speller.cs
- EllipseGeometry.cs
- ConnectionStringsExpressionBuilder.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- _emptywebproxy.cs
- BooleanConverter.cs
- SamlSecurityTokenAuthenticator.cs
- MimeWriter.cs
- SoapRpcMethodAttribute.cs
- AlternateViewCollection.cs
- EditorPartChrome.cs
- WebPartVerb.cs
- _SafeNetHandles.cs
- TextServicesDisplayAttribute.cs
- DataSourceXmlSerializer.cs
- ToolStripGrip.cs
- MyContact.cs
- TaskCanceledException.cs
- GenericXmlSecurityToken.cs
- XslAstAnalyzer.cs
- NonParentingControl.cs
- ColumnBinding.cs
- shaperfactoryquerycacheentry.cs
- HtmlAnchor.cs
- DataTableNewRowEvent.cs
- RadialGradientBrush.cs
- InternalResources.cs
- RenamedEventArgs.cs
- SurrogateEncoder.cs
- XmlQuerySequence.cs
- MetadataCache.cs
- EncodingNLS.cs
- Label.cs
- GridToolTip.cs
- BitArray.cs
- TreeNode.cs
- PointValueSerializer.cs
- SystemIPGlobalStatistics.cs
- bidPrivateBase.cs
- LayoutUtils.cs
- DecimalAnimationUsingKeyFrames.cs
- ReachFixedPageSerializer.cs
- CqlBlock.cs
- SchemaTypeEmitter.cs
- TextEndOfSegment.cs
- DbConnectionPoolGroupProviderInfo.cs
- XmlIgnoreAttribute.cs
- Metafile.cs
- RegionData.cs
- DayRenderEvent.cs
- SafeTimerHandle.cs
- TimeSpan.cs
- XmlSchemaSimpleTypeUnion.cs
- OletxTransactionHeader.cs
- Resources.Designer.cs
- NameValuePermission.cs
- LocatorPartList.cs
- TextElementEditingBehaviorAttribute.cs
- ListControl.cs