Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / CodeGenerator.cs / 1 / CodeGenerator.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // ***NOTE*** If this code is changed, make corresponding changes in System.Runtime.Serialization.CodeGenerator also namespace System.ServiceModel.Dispatcher { using System; using System.Collections; using System.Globalization; using System.Xml; using System.Reflection; using System.Reflection.Emit; using System.IO; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.ServiceModel.Diagnostics; ////// Critical - generates IL into an ILGenerator that was created under an Assert /// generated IL must be correct and must not subvert the type system /// [SecurityCritical(SecurityCriticalScope.Everything)] internal class CodeGenerator { static MethodInfo getTypeFromHandle; static MethodInfo stringConcat2; static MethodInfo objectToString; static MethodInfo boxPointer; static MethodInfo unboxPointer; #if USE_REFEMIT AssemblyBuilder assemblyBuilder; ModuleBuilder moduleBuilder; TypeBuilder typeBuilder; static int typeCounter; MethodBuilder methodBuilder; #else static Module SerializationModule = typeof(CodeGenerator).Module; // Can be replaced by different assembly with SkipVerification set to false DynamicMethod dynamicMethod; #if DEBUG bool allowPrivateMemberAccess; #endif #endif Type delegateType; ILGenerator ilGen; ArrayList argList; Stack blockStack; Label methodEndLabel; Hashtable localNames; int lineNo = 1; enum CodeGenTrace {None, Save, Tron}; CodeGenTrace codeGenTrace; internal CodeGenerator() { SourceSwitch codeGenSwitch = OperationInvokerTrace.CodeGenerationSwitch; if ((codeGenSwitch.Level & SourceLevels.Verbose) == SourceLevels.Verbose) codeGenTrace = CodeGenTrace.Tron; else if ((codeGenSwitch.Level & SourceLevels.Information) == SourceLevels.Information) codeGenTrace = CodeGenTrace.Save; else codeGenTrace = CodeGenTrace.None; } static MethodInfo GetTypeFromHandle { get { if (getTypeFromHandle == null) getTypeFromHandle = typeof(Type).GetMethod("GetTypeFromHandle"); return getTypeFromHandle; } } static MethodInfo StringConcat2 { get { if (stringConcat2 == null) stringConcat2 = typeof(string).GetMethod("Concat", new Type[] { typeof(string), typeof(string) }); return stringConcat2; } } static MethodInfo ObjectToString { get { if (objectToString == null) objectToString = typeof(object).GetMethod("ToString", new Type[0]); return objectToString; } } static MethodInfo BoxPointer { get { if (boxPointer == null) boxPointer = typeof(Pointer).GetMethod("Box"); return boxPointer; } } static MethodInfo UnboxPointer { get { if (unboxPointer == null) unboxPointer = typeof(Pointer).GetMethod("Unbox"); return unboxPointer; } } internal void BeginMethod(string methodName, Type delegateType, bool allowPrivateMemberAccess) { MethodInfo signature = delegateType.GetMethod("Invoke"); ParameterInfo[] parameters = signature.GetParameters(); Type[] paramTypes = new Type[parameters.Length]; for (int i = 0; i < parameters.Length; i++) paramTypes[i] = parameters[i].ParameterType; BeginMethod(signature.ReturnType, methodName, paramTypes, allowPrivateMemberAccess); this.delegateType = delegateType; } void BeginMethod(Type returnType, string methodName, Type[] argTypes, bool allowPrivateMemberAccess) { #if USE_REFEMIT string typeName = "Type" + (typeCounter++); InitAssemblyBuilder(typeName + "." + methodName); this.typeBuilder = moduleBuilder.DefineType(typeName, TypeAttributes.Public); this.methodBuilder = typeBuilder.DefineMethod(methodName, MethodAttributes.Public|MethodAttributes.Static, returnType, argTypes); this.ilGen = this.methodBuilder.GetILGenerator(); #else this.dynamicMethod = new DynamicMethod(methodName, returnType, argTypes, SerializationModule, allowPrivateMemberAccess); this.ilGen = this.dynamicMethod.GetILGenerator(); #if DEBUG this.allowPrivateMemberAccess = allowPrivateMemberAccess; #endif #endif this.methodEndLabel = ilGen.DefineLabel(); this.blockStack = new Stack(); this.argList = new ArrayList(); for (int i=0;i"); Else(); Call(ObjectToString); EndIf(); } } internal void Concat2() { Call(StringConcat2); } internal void LoadZeroValueIntoLocal(Type type, LocalBuilder local) { if (type.IsValueType) { switch (Type.GetTypeCode(type)) { case TypeCode.Boolean: case TypeCode.Char: case TypeCode.SByte: case TypeCode.Byte: case TypeCode.Int16: case TypeCode.UInt16: case TypeCode.Int32: case TypeCode.UInt32: ilGen.Emit(OpCodes.Ldc_I4_0); Store(local); break; case TypeCode.Int64: case TypeCode.UInt64: ilGen.Emit(OpCodes.Ldc_I4_0); ilGen.Emit(OpCodes.Conv_I8); Store(local); break; case TypeCode.Single: ilGen.Emit(OpCodes.Ldc_R4, 0.0F); Store(local); break; case TypeCode.Double: ilGen.Emit(OpCodes.Ldc_R8, 0.0); Store(local); break; case TypeCode.Decimal: case TypeCode.DateTime: default: LoadAddress(local); InitObj(type); break; } } else { Load(null); Store(local); } } } internal class ArgBuilder { internal int Index; internal Type ArgType; internal ArgBuilder(int index, Type argType) { this.Index = index; this.ArgType = argType; } } internal class IfState { Label elseBegin; Label endIf; internal Label EndIf { get { return this.endIf; } set { this.endIf= value; } } internal Label ElseBegin { get { return this.elseBegin; } set { this.elseBegin= value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
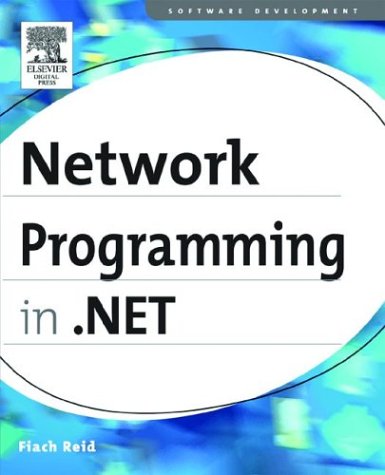
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QueryPageSettingsEventArgs.cs
- LocalsItemDescription.cs
- SqlNodeAnnotation.cs
- DeobfuscatingStream.cs
- ExceptionTranslationTable.cs
- FullTextState.cs
- GlyphsSerializer.cs
- NestedContainer.cs
- PartitionResolver.cs
- HttpResponseHeader.cs
- DbModificationCommandTree.cs
- Rotation3DAnimationBase.cs
- CompilerWrapper.cs
- smtpconnection.cs
- ErrorProvider.cs
- CodePageEncoding.cs
- ValueType.cs
- SynchronizationContext.cs
- ObfuscationAttribute.cs
- DotAtomReader.cs
- TextPointerBase.cs
- ComEventsHelper.cs
- InvalidWMPVersionException.cs
- PageCatalogPartDesigner.cs
- ContentHostHelper.cs
- BitmapCache.cs
- path.cs
- RSAPKCS1SignatureDeformatter.cs
- ChildDocumentBlock.cs
- DoubleAnimation.cs
- SecurityTokenParameters.cs
- handlecollector.cs
- CounterCreationDataCollection.cs
- UiaCoreApi.cs
- ObjectFullSpanRewriter.cs
- ViewService.cs
- MeasureData.cs
- UrlAuthFailedErrorFormatter.cs
- FileAuthorizationModule.cs
- HierarchicalDataSourceControl.cs
- DataColumn.cs
- SocketElement.cs
- ContextMenu.cs
- HttpResponseMessageProperty.cs
- CommandHelper.cs
- ResourceProperty.cs
- UriScheme.cs
- Stroke.cs
- RequestCacheEntry.cs
- ListParagraph.cs
- NamespaceDisplay.xaml.cs
- _CacheStreams.cs
- UserPreference.cs
- ItemContainerPattern.cs
- DrawingImage.cs
- HostedImpersonationContext.cs
- AlphabeticalEnumConverter.cs
- DataServiceProcessingPipeline.cs
- XmlSignatureProperties.cs
- HttpCookieCollection.cs
- Normalizer.cs
- WebPartConnectionsCancelEventArgs.cs
- IssuedSecurityTokenParameters.cs
- DrawingImage.cs
- OracleMonthSpan.cs
- Figure.cs
- ValidationErrorCollection.cs
- Dynamic.cs
- SecurityChannelListener.cs
- OdbcParameterCollection.cs
- ZipIOLocalFileDataDescriptor.cs
- AssociationSetEnd.cs
- StylusButtonCollection.cs
- XmlObjectSerializerWriteContextComplex.cs
- OleDbWrapper.cs
- SqlUserDefinedTypeAttribute.cs
- ReadOnlyHierarchicalDataSource.cs
- DispatcherTimer.cs
- ObjectDataSourceDisposingEventArgs.cs
- FileIOPermission.cs
- StorageAssociationTypeMapping.cs
- ReceiveActivityDesignerTheme.cs
- EdmItemCollection.cs
- AQNBuilder.cs
- ExtensionQuery.cs
- PreservationFileReader.cs
- BlockCollection.cs
- EditingCoordinator.cs
- Quack.cs
- OleDbReferenceCollection.cs
- GridViewHeaderRowPresenter.cs
- MarginsConverter.cs
- DetailsViewRowCollection.cs
- Vector3DConverter.cs
- XPathConvert.cs
- DirectoryObjectSecurity.cs
- ArrayList.cs
- KeyedHashAlgorithm.cs
- PropertyHelper.cs
- DecimalStorage.cs