Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ProcessMonitor.cs / 1 / ProcessMonitor.cs
namespace Microsoft.InfoCards { using System; using System.ComponentModel; using System.Collections.Generic; using System.Diagnostics; using System.Runtime.InteropServices; internal class ProcessMonitor { static ProcessMonitor s_current = new ProcessMonitor(); Dictionarym_cache; object m_sync; private ProcessMonitor() { m_sync = new object(); m_cache = new Dictionary (); } public static Process GetProcessById( int id ) { return s_current.InnerGetProcessById( id ); } Process InnerGetProcessById( int id ) { Process process = null; lock( m_sync ) { if( !m_cache.TryGetValue( id, out process ) ) { process = Process.GetProcessById( id ); if( !process.HasExited ) { InitializeProcessObject( process ); // // add the entry to the cache. // m_cache.Add( process.Id, process ); } } } return process; } void InitializeProcessObject( Process process ) { // // This call can create a thread when setting the value to true. // process.EnableRaisingEvents = true; process.Exited += new EventHandler( Process_Exited ); } void Process_Exited( object sender, EventArgs e ) { Process process = (Process)sender; lock( m_sync ) { m_cache.Remove( process.Id ); } process.Exited -= new EventHandler( Process_Exited ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
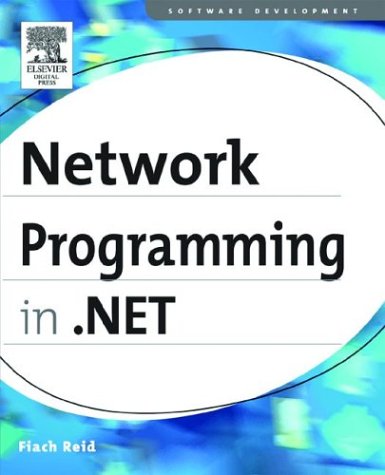
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WriterOutput.cs
- CompoundFileIOPermission.cs
- DataServiceContext.cs
- HttpResponseInternalWrapper.cs
- VersionedStream.cs
- ToolStripPanelRenderEventArgs.cs
- HwndProxyElementProvider.cs
- MarkupObject.cs
- MsmqIntegrationSecurity.cs
- XmlSignatureProperties.cs
- ReadOnlyHierarchicalDataSource.cs
- SignedInfo.cs
- ListenerElementsCollection.cs
- RefType.cs
- BasicViewGenerator.cs
- ResourcePermissionBase.cs
- TempFiles.cs
- FontCollection.cs
- CodeAttributeArgument.cs
- DataKeyCollection.cs
- UnlockInstanceCommand.cs
- SplineKeyFrames.cs
- MenuStrip.cs
- Rules.cs
- CreateUserWizardStep.cs
- KeyGestureConverter.cs
- OdbcConnectionHandle.cs
- ReferentialConstraintRoleElement.cs
- DescendentsWalker.cs
- Visual3D.cs
- DuplicateDetector.cs
- sqlser.cs
- WorkItem.cs
- PassportAuthentication.cs
- RangeEnumerable.cs
- Triplet.cs
- SqlNotificationEventArgs.cs
- WebPartDescription.cs
- EntityProviderServices.cs
- TemplateNodeContextMenu.cs
- DbCommandDefinition.cs
- TextSchema.cs
- XamlFigureLengthSerializer.cs
- TableLayoutStyle.cs
- WebBrowserEvent.cs
- DataServiceEntityAttribute.cs
- TraceData.cs
- HtmlInputRadioButton.cs
- Main.cs
- ControlPropertyNameConverter.cs
- Baml2006SchemaContext.cs
- HyperLinkStyle.cs
- TextDpi.cs
- _LazyAsyncResult.cs
- ClientScriptManager.cs
- RSAProtectedConfigurationProvider.cs
- ConfigXmlComment.cs
- ImageConverter.cs
- StandardCommands.cs
- ReadOnlyCollectionBase.cs
- CollaborationHelperFunctions.cs
- TextProviderWrapper.cs
- FilterElement.cs
- TabControlAutomationPeer.cs
- SafeSerializationManager.cs
- PhonemeConverter.cs
- ContainerAction.cs
- TableCellCollection.cs
- XamlBrushSerializer.cs
- ListManagerBindingsCollection.cs
- AdCreatedEventArgs.cs
- EventDescriptorCollection.cs
- Rectangle.cs
- DeobfuscatingStream.cs
- DependencyPropertyHelper.cs
- MonikerBuilder.cs
- SingleStorage.cs
- TextTreeObjectNode.cs
- AspNetCacheProfileAttribute.cs
- ListViewContainer.cs
- ElementAction.cs
- relpropertyhelper.cs
- OdbcConnectionHandle.cs
- CodeTypeMember.cs
- EntityDataSourceWrapperCollection.cs
- GPRECT.cs
- FrameworkObject.cs
- EtwTrace.cs
- WeakEventManager.cs
- nulltextcontainer.cs
- OpenFileDialog.cs
- XmlDocumentSerializer.cs
- BaseCAMarshaler.cs
- SystemFonts.cs
- XmlBoundElement.cs
- SizeConverter.cs
- DocumentSequenceHighlightLayer.cs
- FontDriver.cs
- AddInEnvironment.cs
- IImplicitResourceProvider.cs